The JavaTM API for RESTful Web Services. For more detail, see Wikipedia.
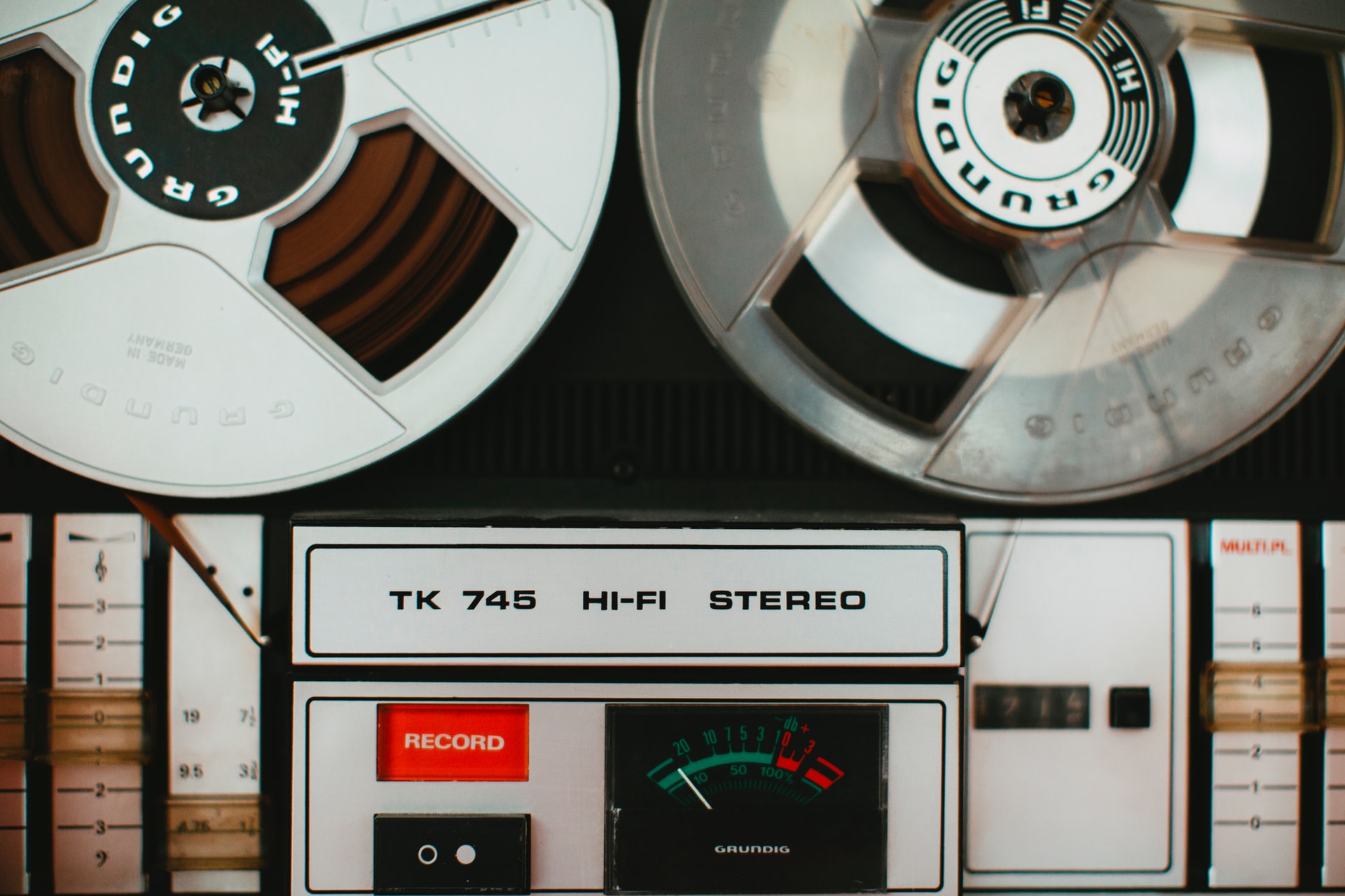
Audit Logs
This article discusses how to implement a simple audit logs solution with Java JAX-RS, including requirements, considerations for the implementation, and 3 different solutions based on Java Servlet, Jetty, and JAX-RS filter.
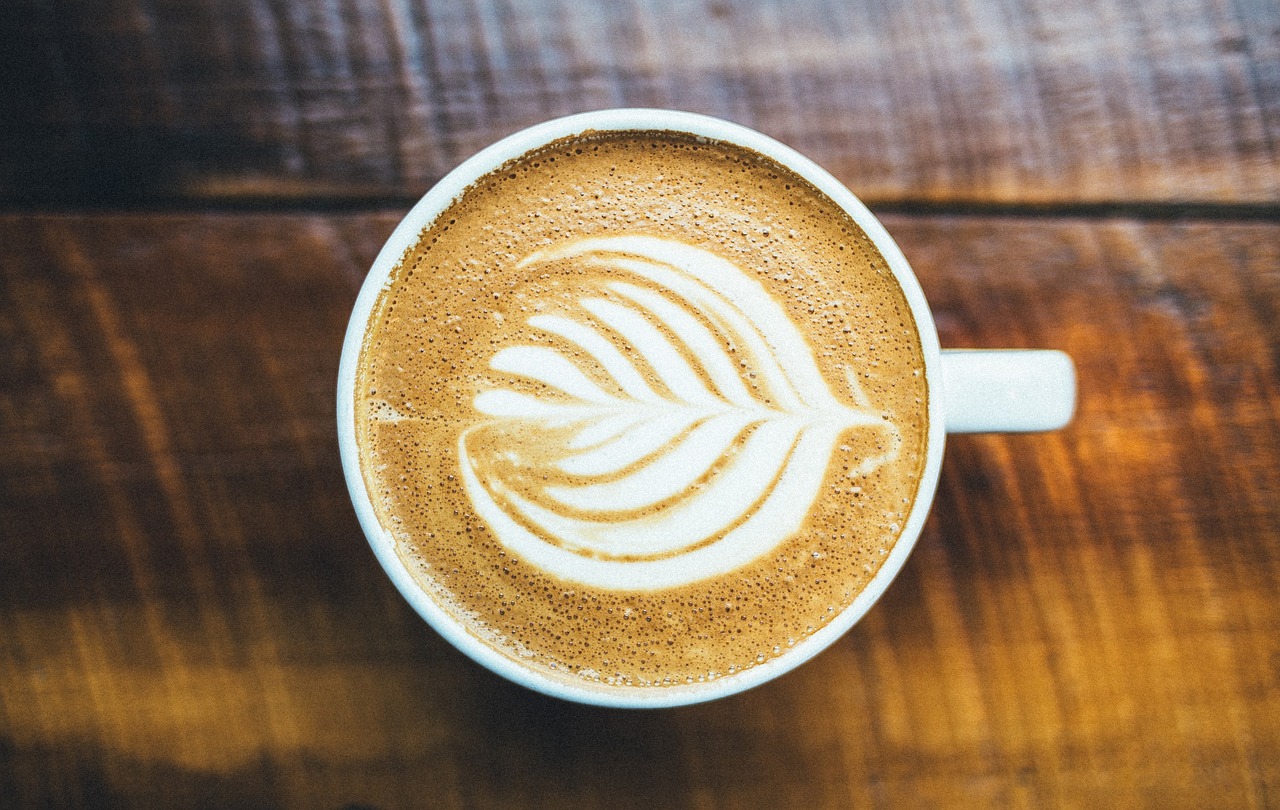
Asynchronous Processing in JAX-RS 2.x
Quick introduction of asynchrnous processing in JAX-RS 2.x on both server-side and client-side.
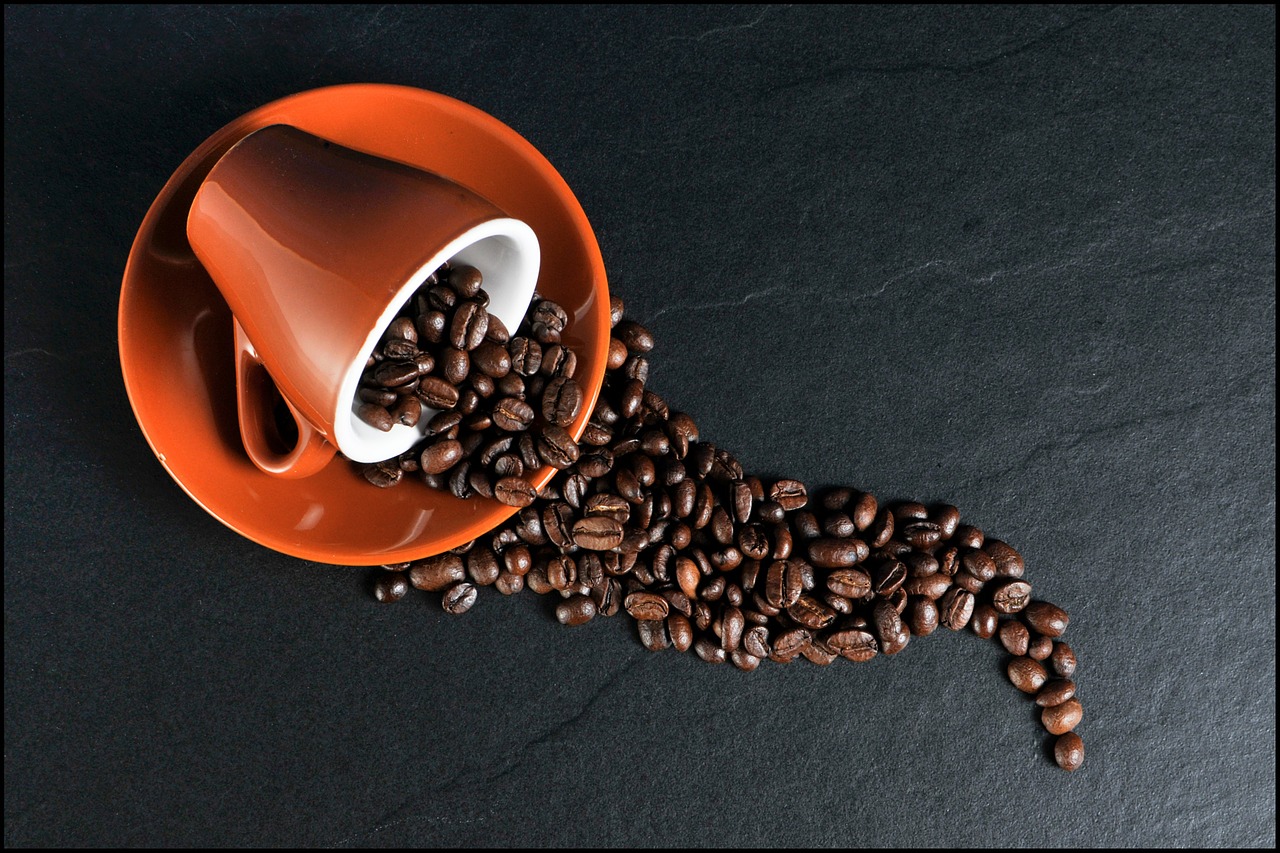
Testing JAX-RS Resources
This article explains how to set up and tear down a Grizzly Server for testing JAX-RS resources, how to create a HTTP request and assert the response using JUnit 4. And finally, the limits of testing API in reality.
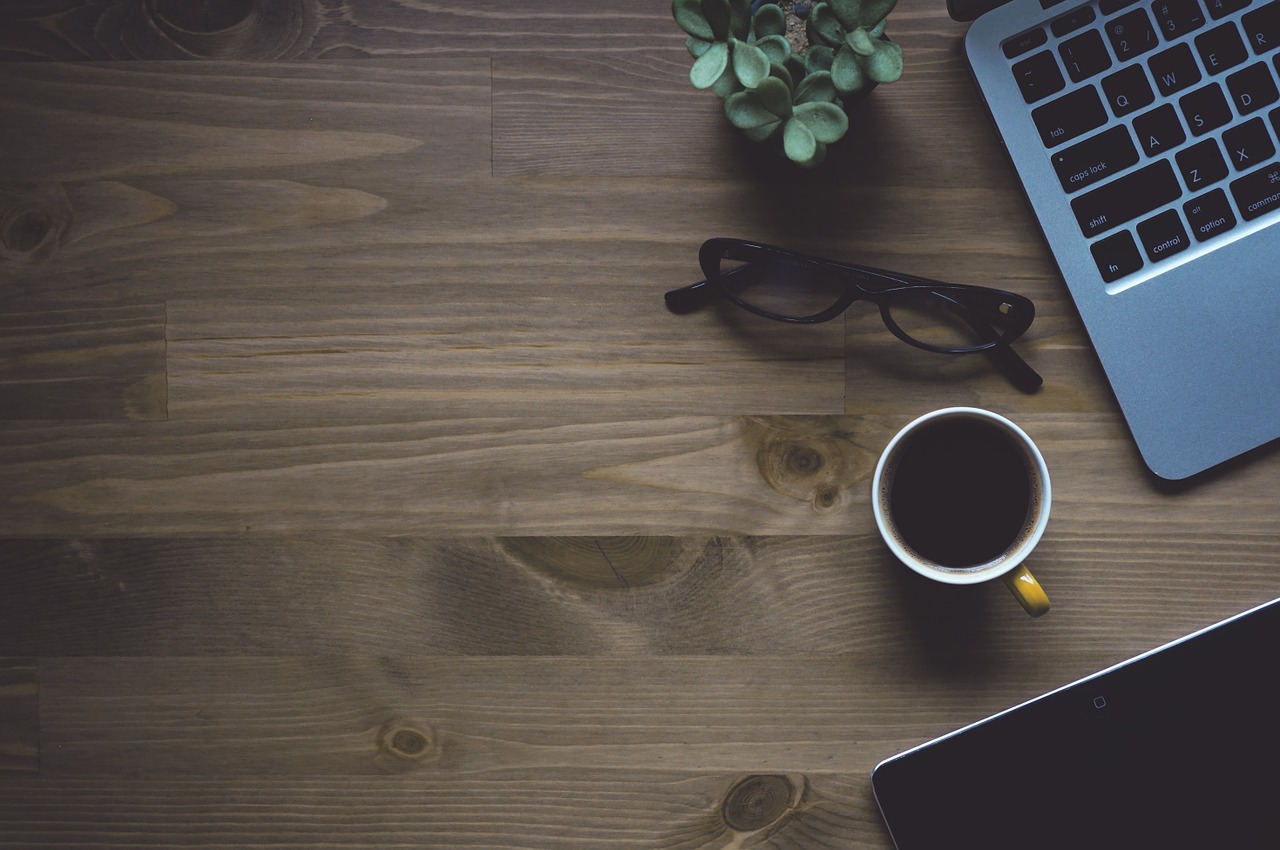
JAX-RS Client API
This post explains what is JAX-RS Client API and how to use it via Jersey Client API. We will talk about the Maven dependencies, Client, WebTarget, and HTTP response.
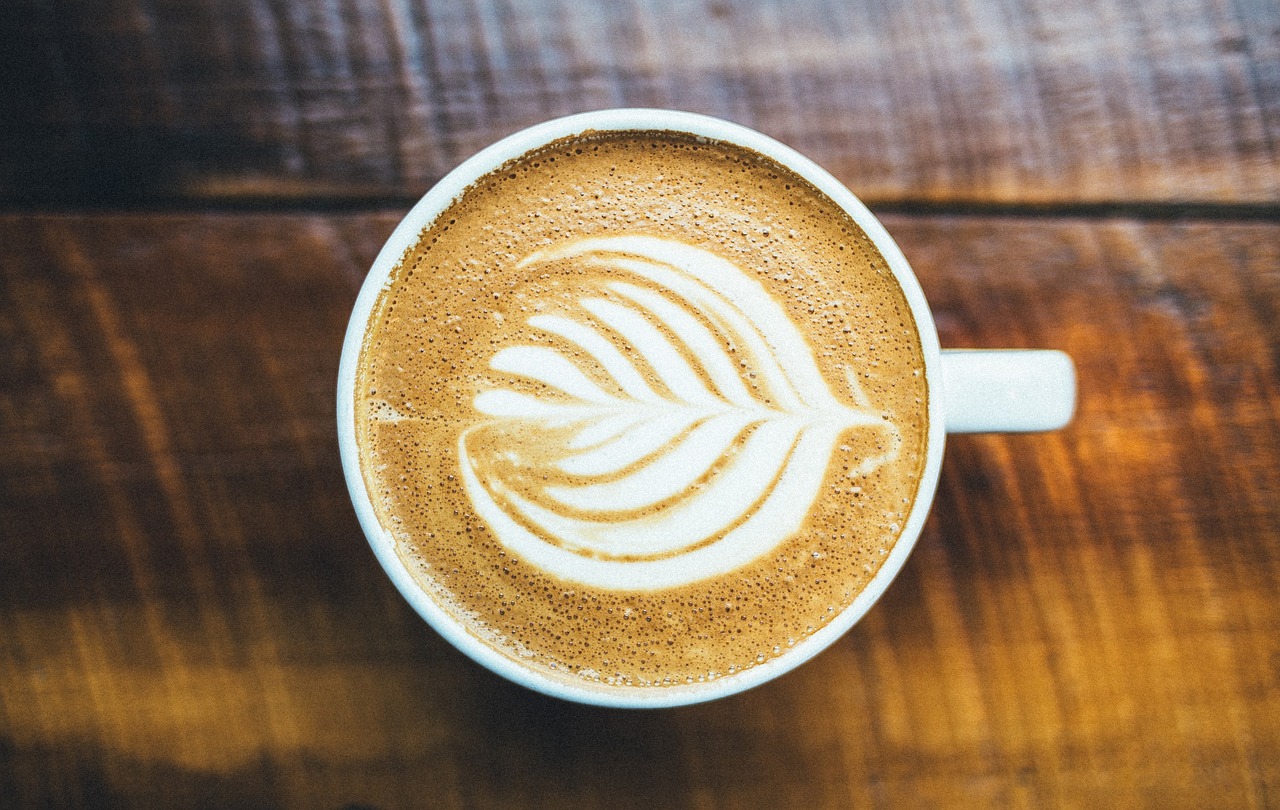
Exception Handling in JAX-RS
This post explains exception mapper, how to register it in JAX-RS application programmatically or via annotation, the exception matching mechanism (nearest-superclass), and more.
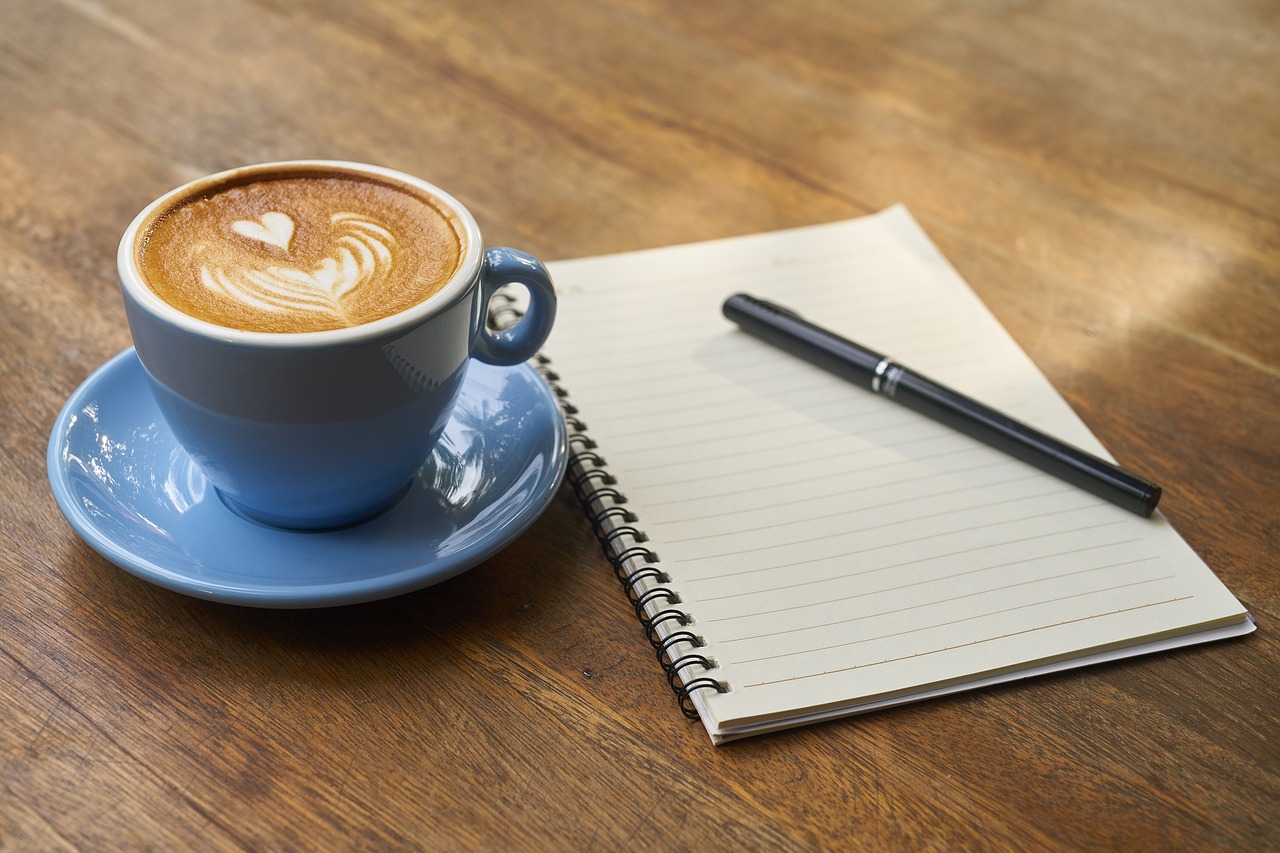
JAX-RS Param Annotations
This post explains different param annotations in JAX-RS 2.1 and their use-cases, including @QueryParam, @MatrixParam, @PathParam, @HeaderParam, @CookieParam, @FormParam and @BeanParam.
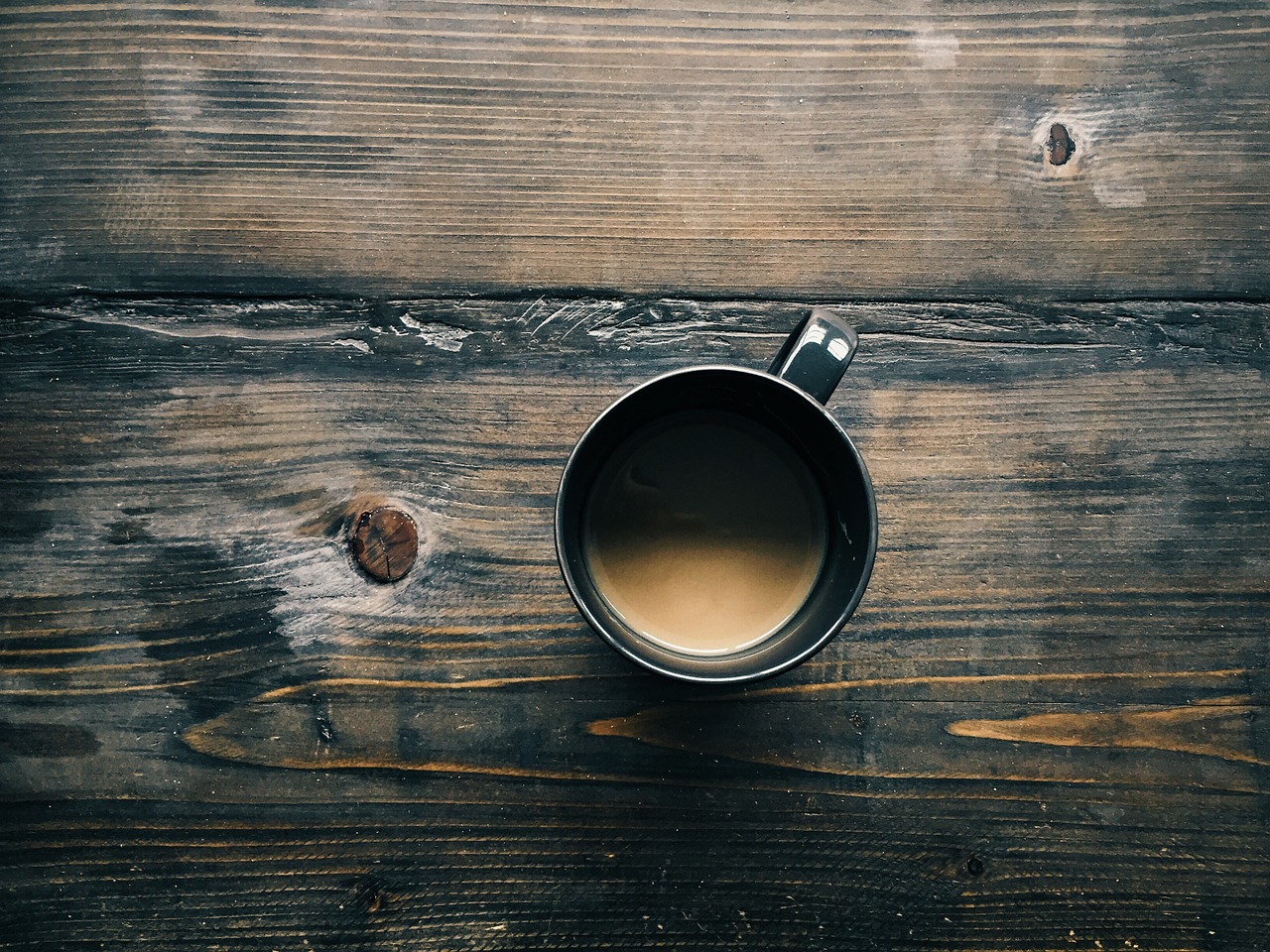
HTTP Methods in JAX-RS
This article explains the common HTTP methods in JAX-RS: annotation @GET, @POST, @PUT, and @DELETE.
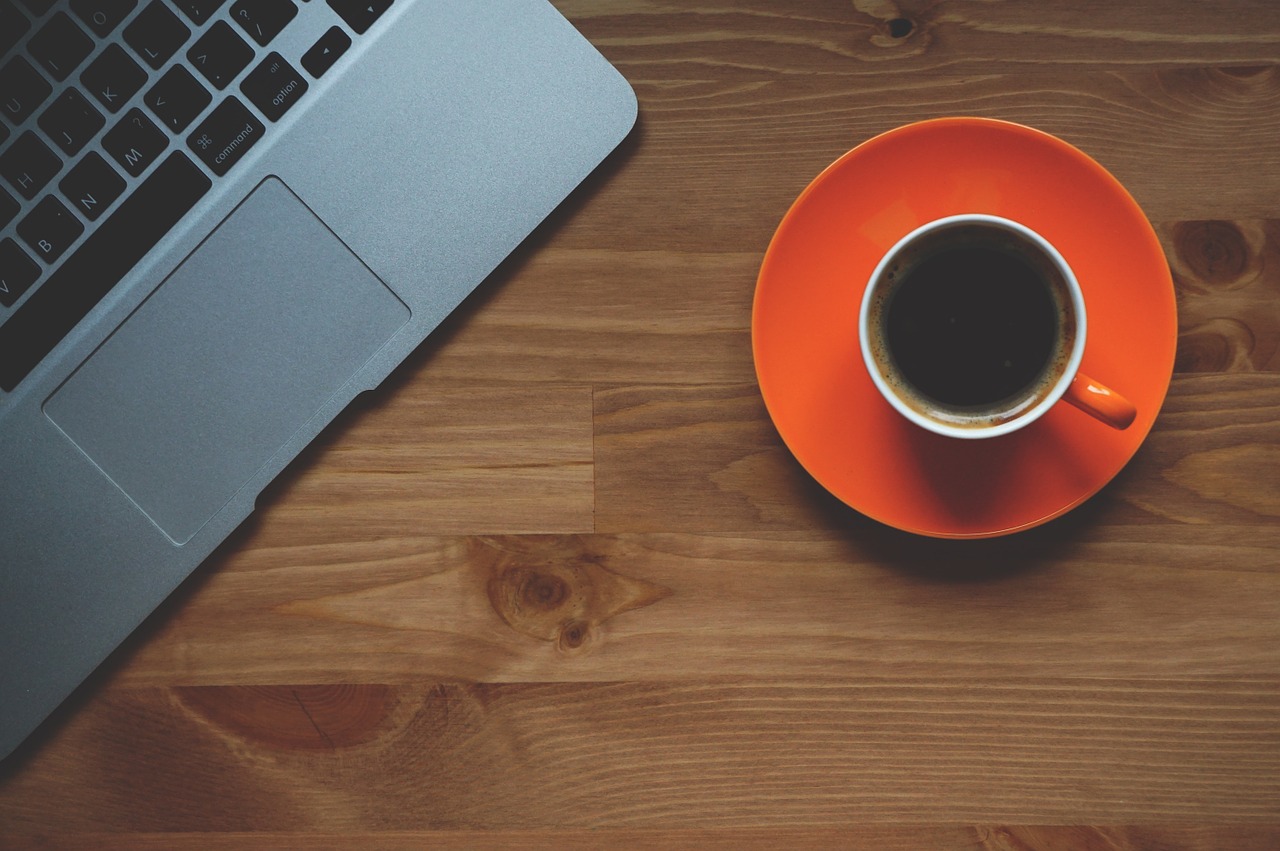
Simple REST Demo With JAX-RS
A quickstart demo for creating REST service in Java using JAX-RS 2.0. The sample is implemented by Jersey, the reference implementation of JAX-RS.