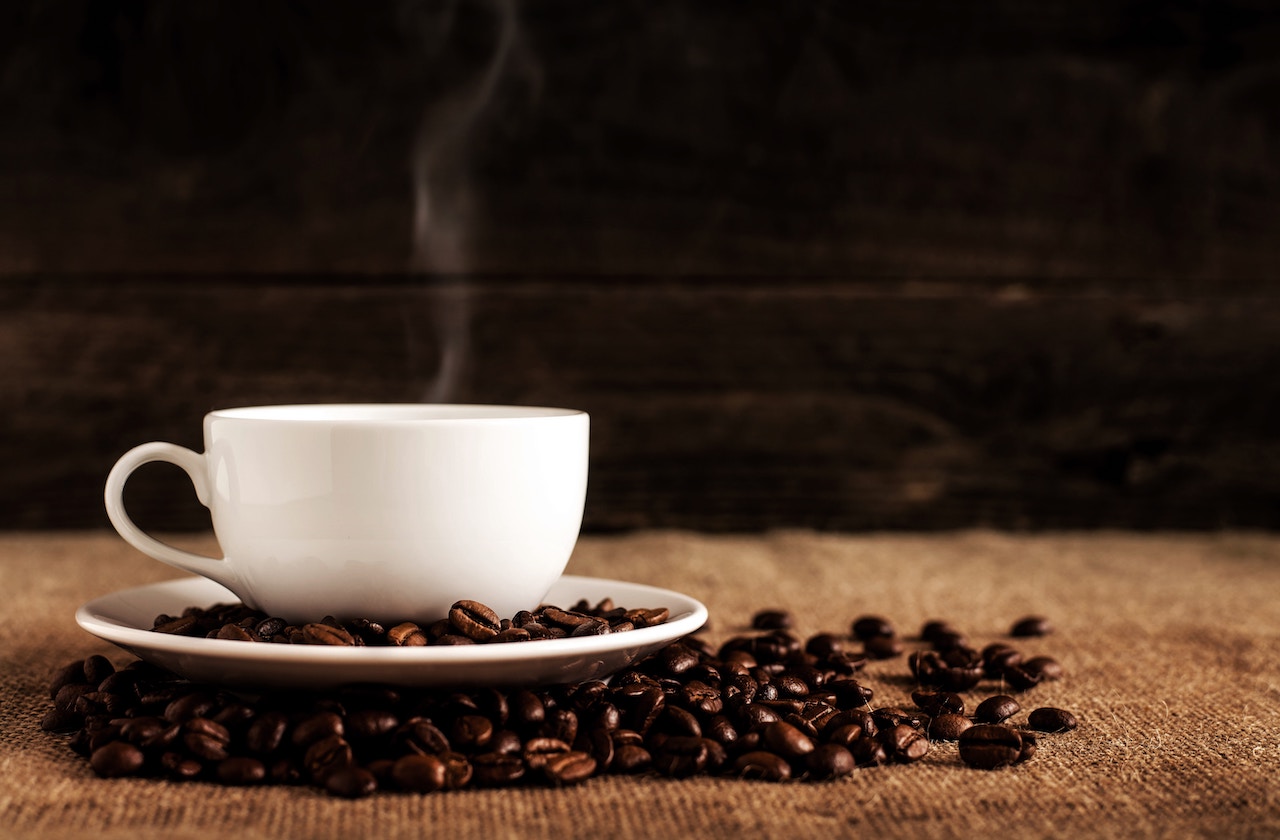
JUnit 5: Dynamic Tests with TestFactory
How to write dynamic tests using @TestFactory in JUnit 5? This article explains the syntax, different return types, the test lifecycle, and potential use-cases.
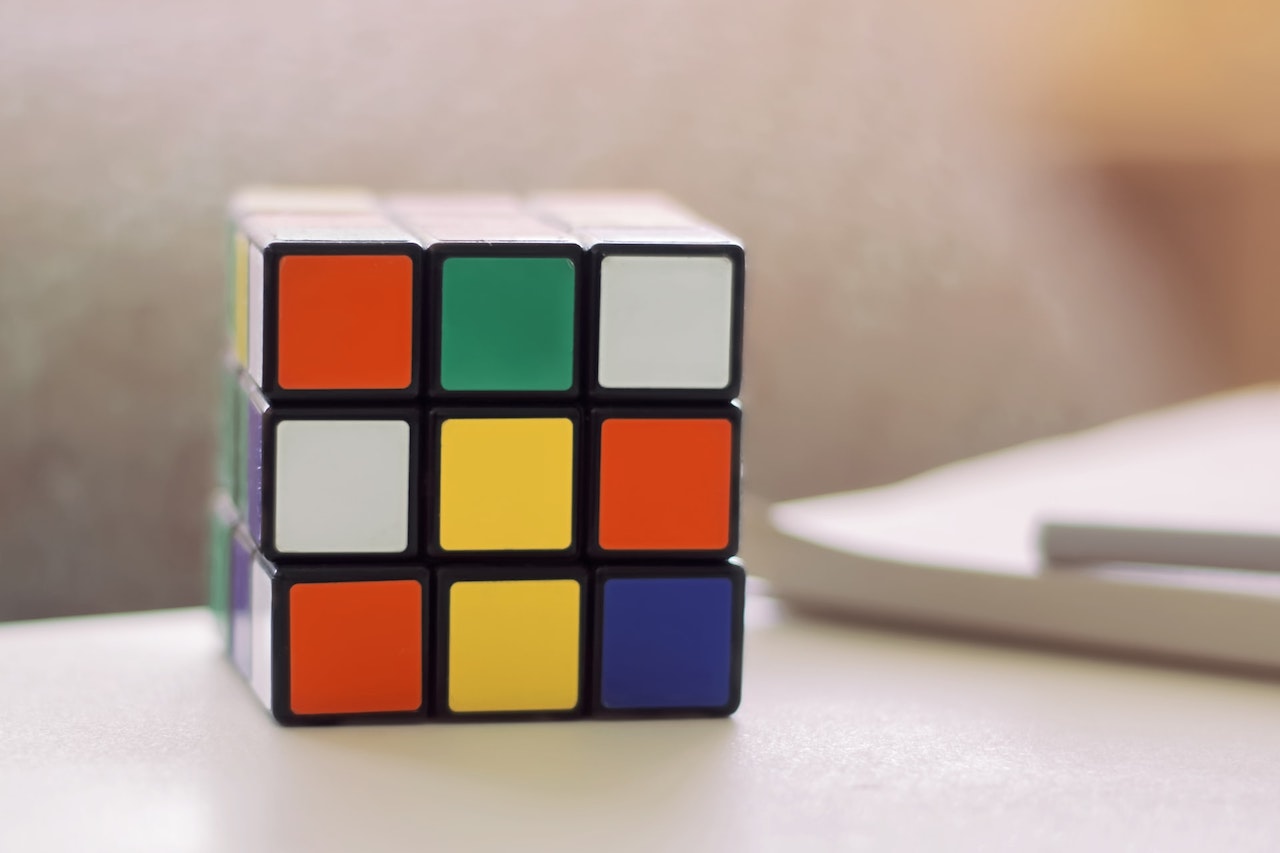
Writing Parameterized Tests in JUnit 5
Improving code quality using parameterized tests of JUnit 5! This article explains the motivation, the basic syntax, different annotations, and IDE-related actions about parameterized tests. Also, when you should or shouldn't use it and how to go further from here.
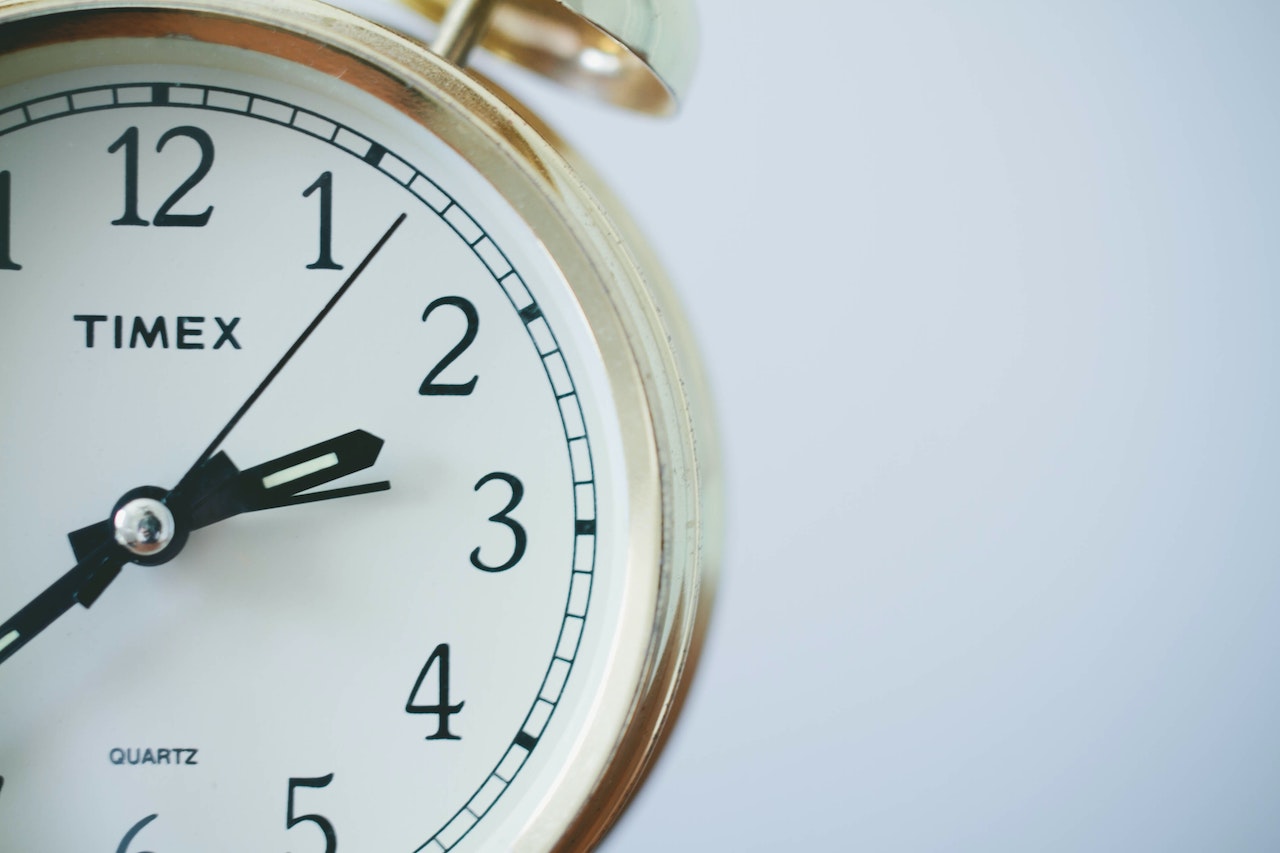
Controlling Time with Java Clock
Use java.time.Clock to control time in your unit tests. This article will mainly focus on usage of fixed clock and offset clock.
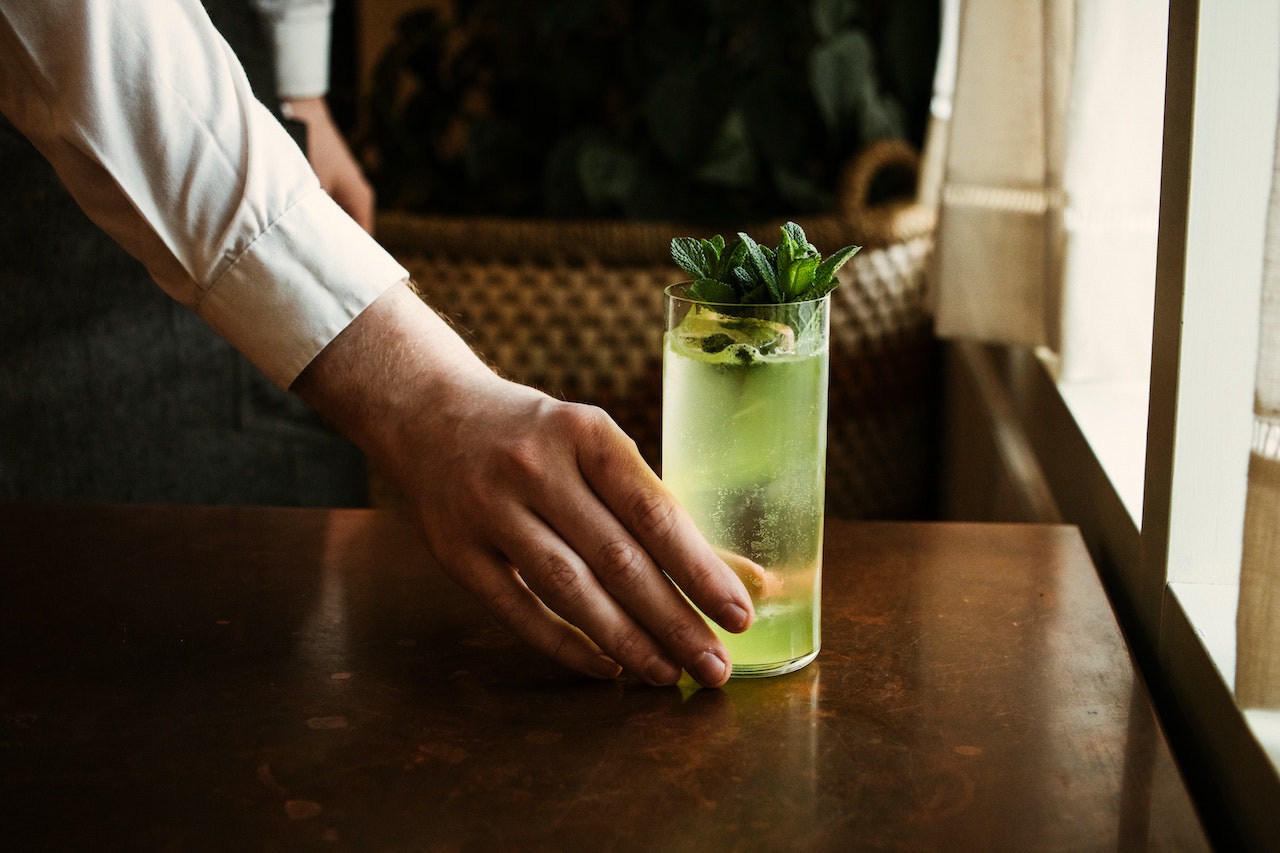
Mockito: 3 Ways to Init Mock in JUnit 5
Initialize Mockito mock objects in JUnit 5 using MockitoExtension, MockitoAnnotations#initMocks, or Mockito#mock.
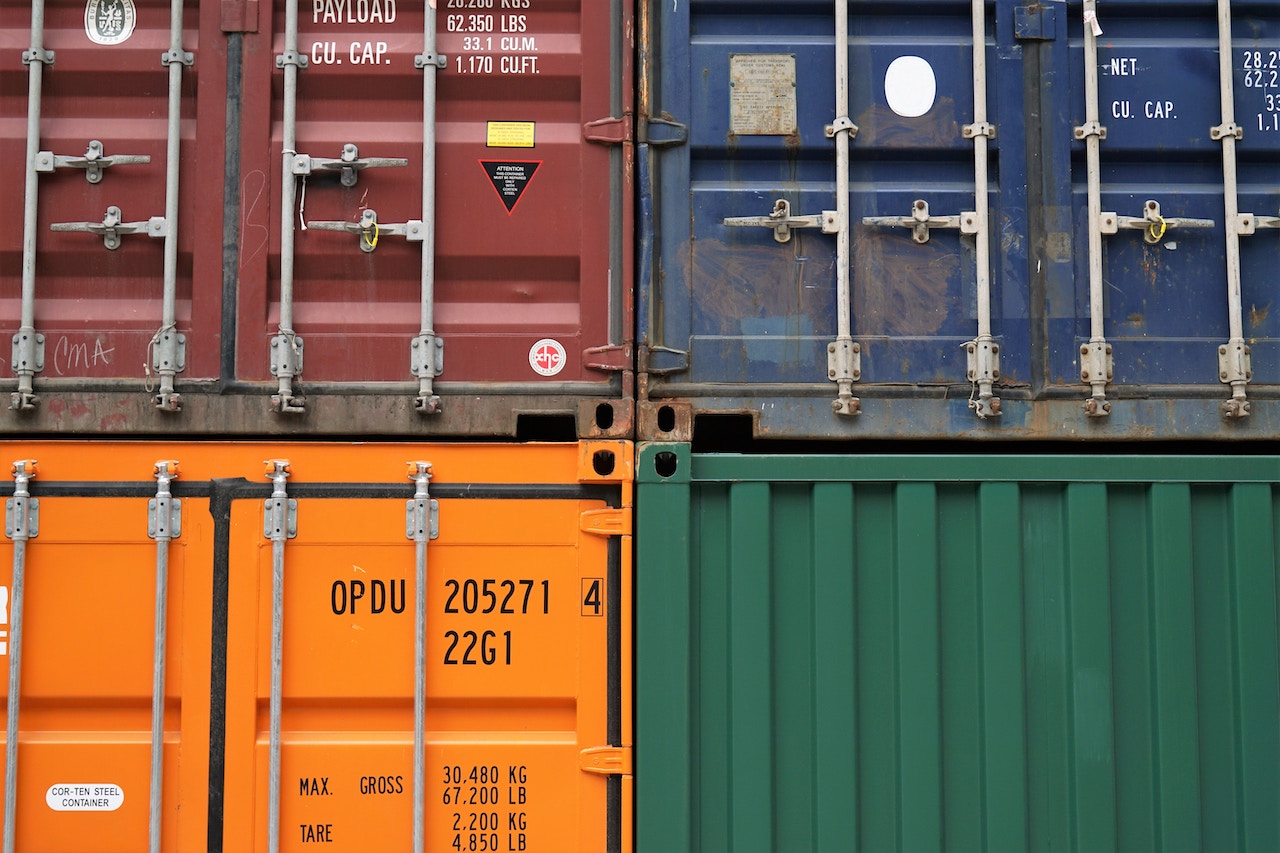
Testing Elasticsearch With Docker And Java High Level REST Client
Testing Elasticsearch with docker and Java High Level REST Client
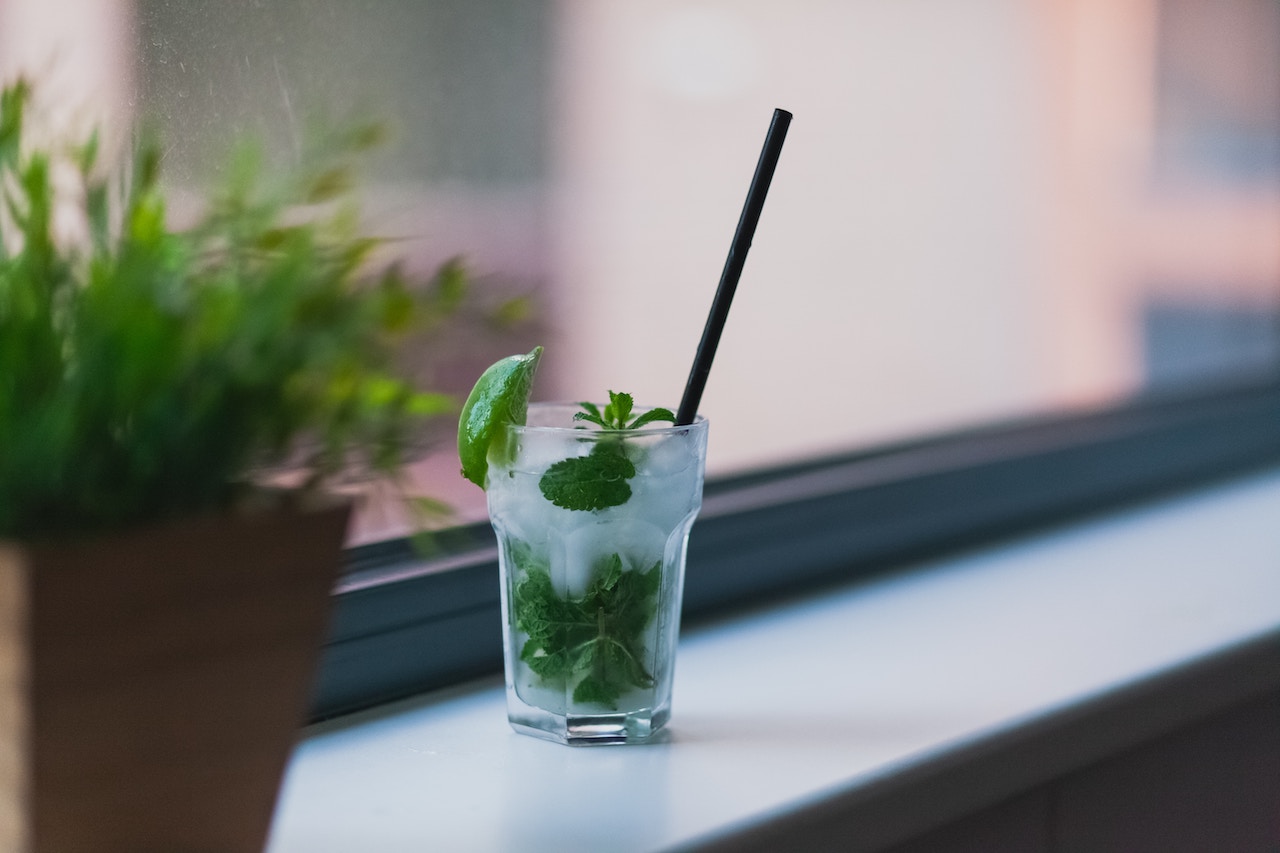
Mockito: ArgumentCaptor
Three ways to create ArgumentCaptor in unit tests (Mockito JUnit Runner, annotations, or factory method) and its different usage.
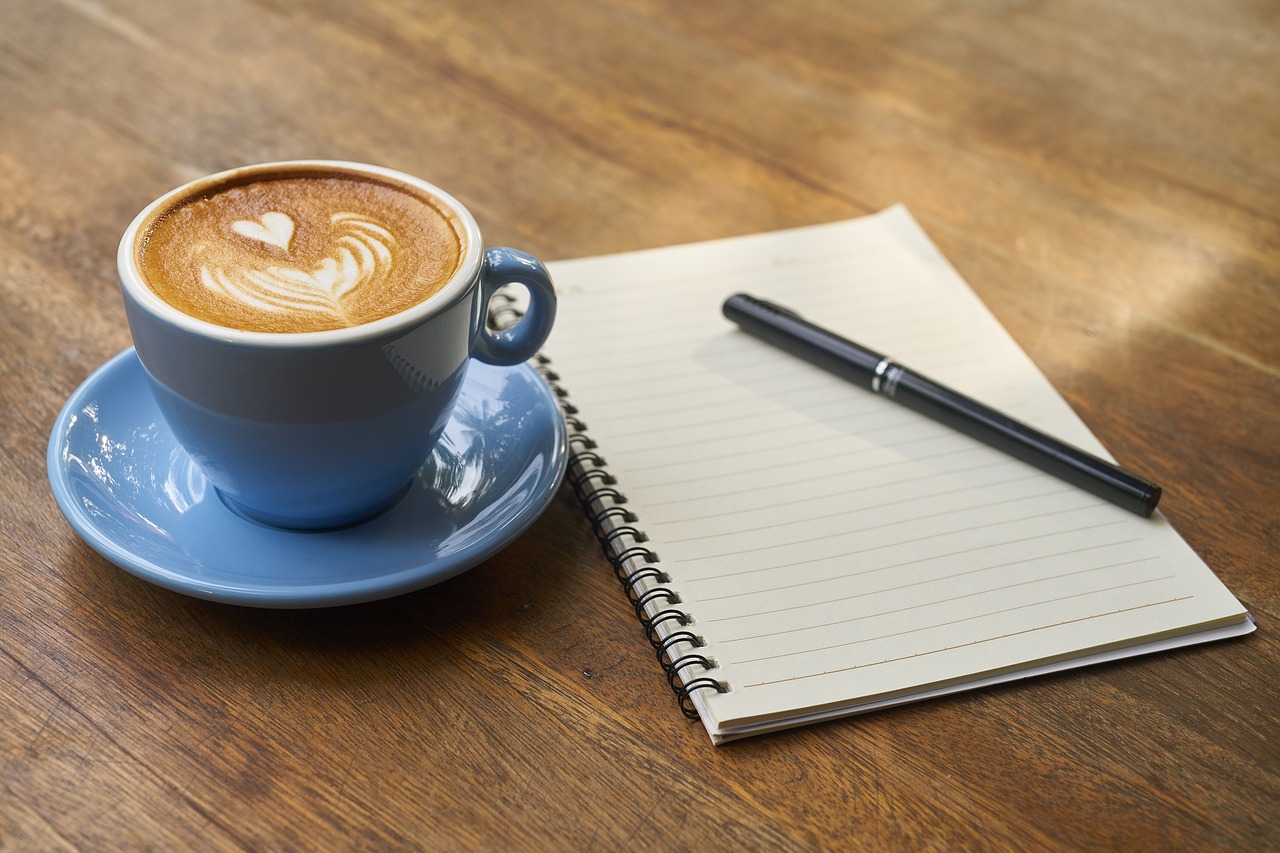
Testing Elasticsearch with ESSingleNodeTestCase
Writing unit tests for Elasticsearch using Elasticsearch Single Node Test Case (ESSingleNodeTestCase), a derived class of ESTestCase which simplifies the testing set up for you.
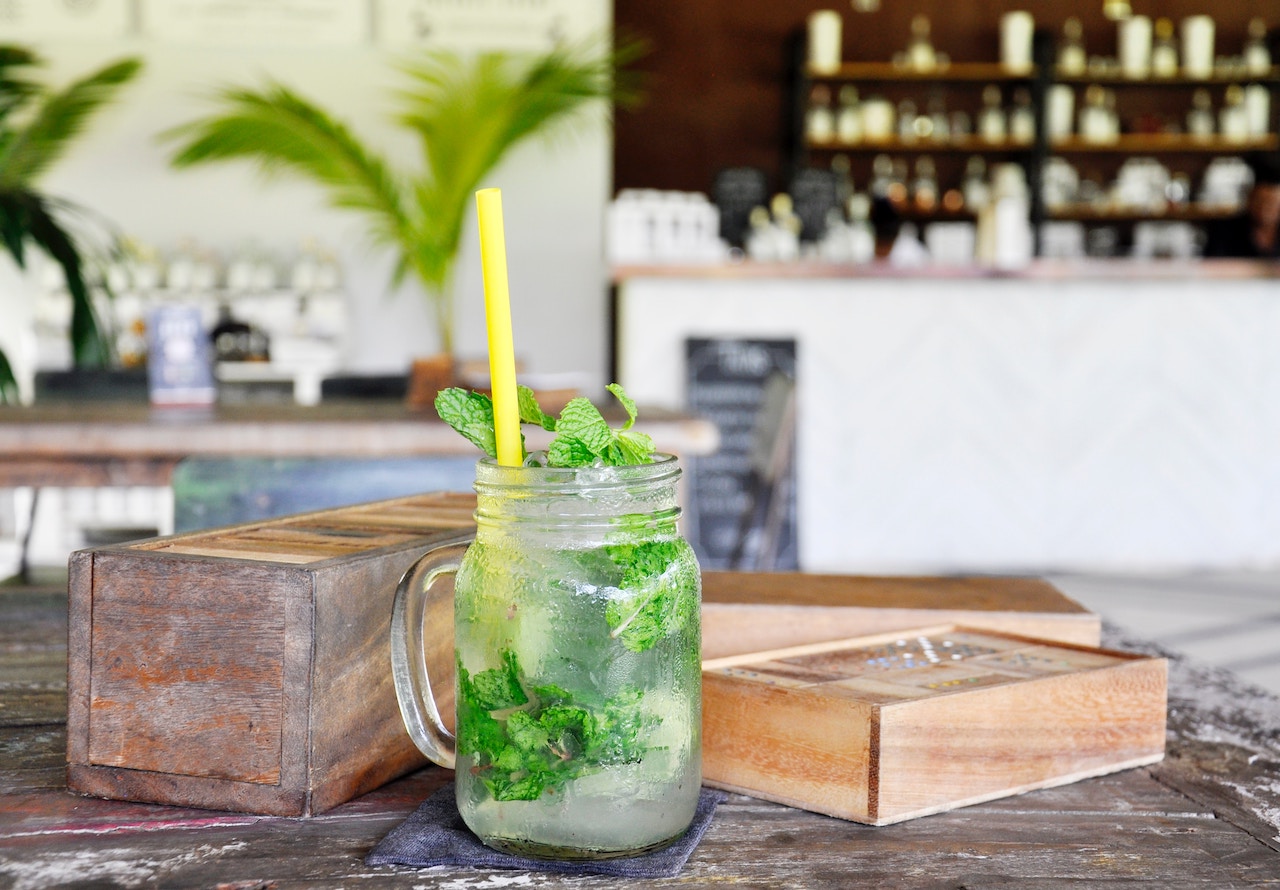
Mockito: 4 Ways to Verify Interactions
Verify interaction with mock objects with verify(), verifyZeroInteractions() verifyNoMoreInteractions(), and inOrder().
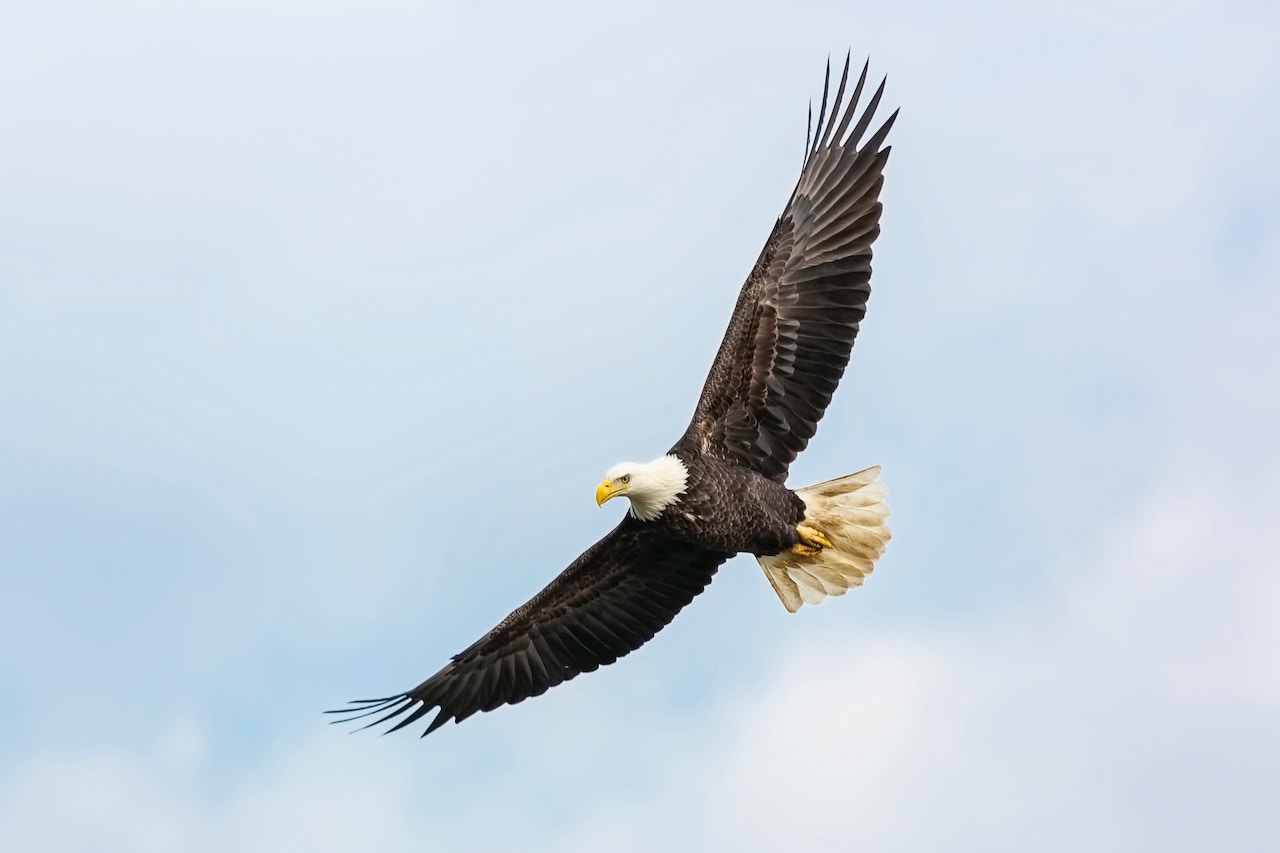
Project: NOS Test
NOS Test is a QA project consists of 3 parts: RESTful API, browser, and command line. In this article, I will explain how I implemented it.
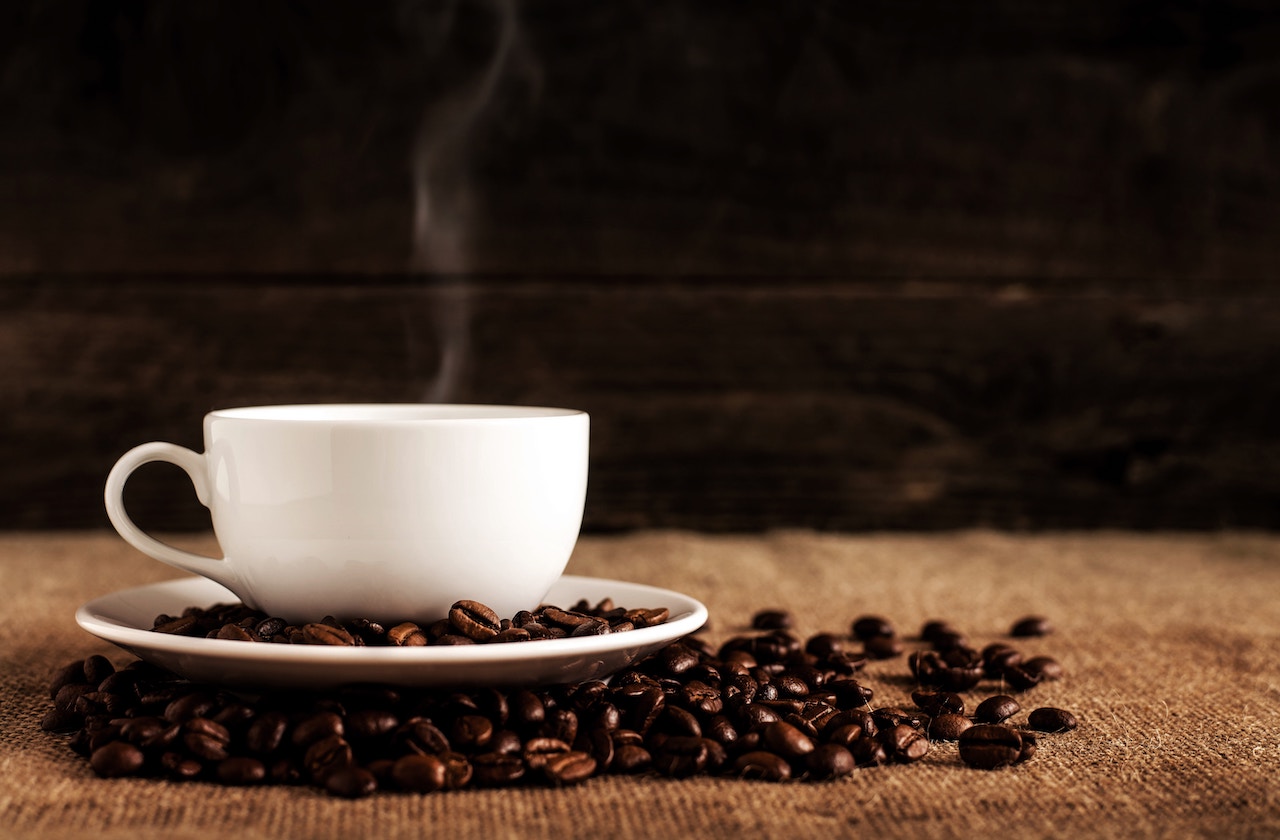
Mockito: 3 Ways to Init Mock in JUnit 4
Initialize Mockito mock objects in JUnit 4 using MockitoJUnitRunner, MockitoAnnotations#initMocks, or Mockito#mock.
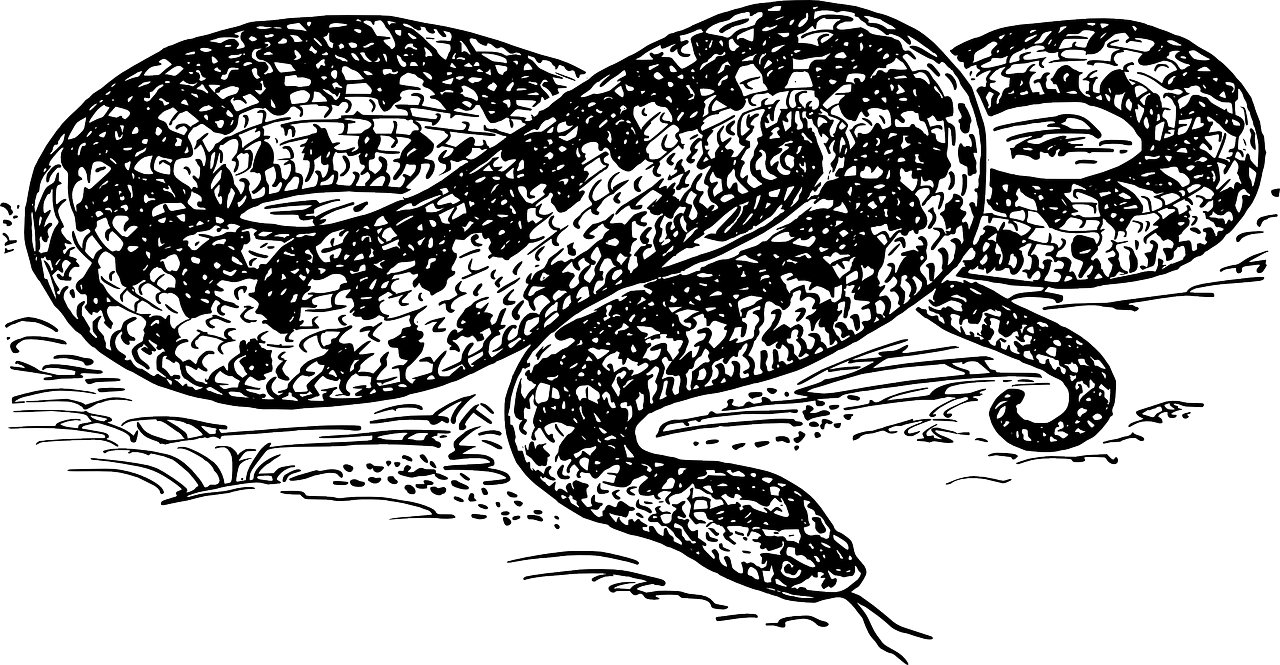
Measure Coverage with Coverage.py
Experience sharing on code coverage measurement of Python program using Coverage.py.
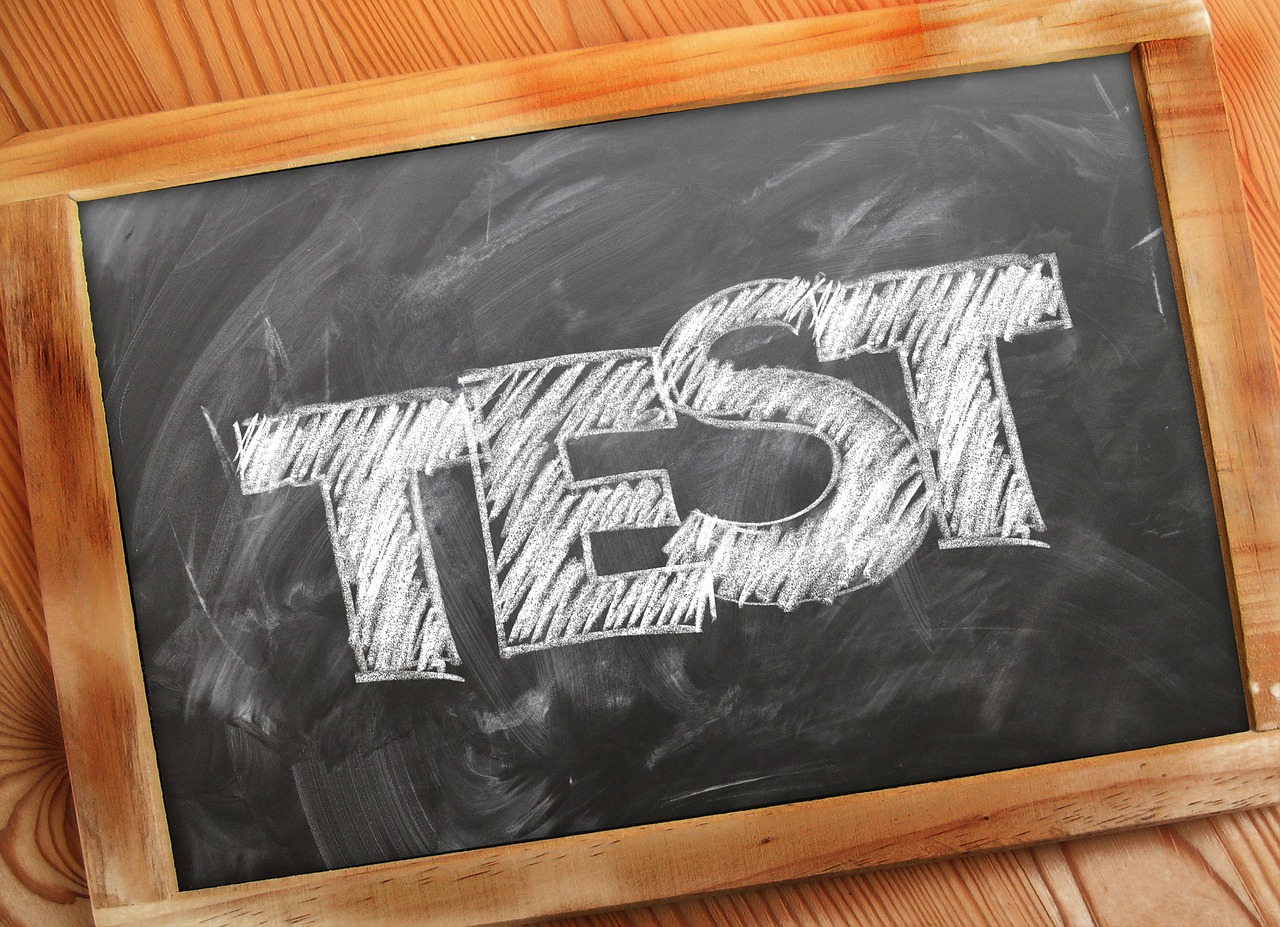
Testing with GwtMockito
My recent bug fixing experience on Google Web Kit (GWT) with GwtMockito: problem understanding, code refactoring, mocking framework preparation, testing, and comparison between GwtMockito and GWTTestCase.
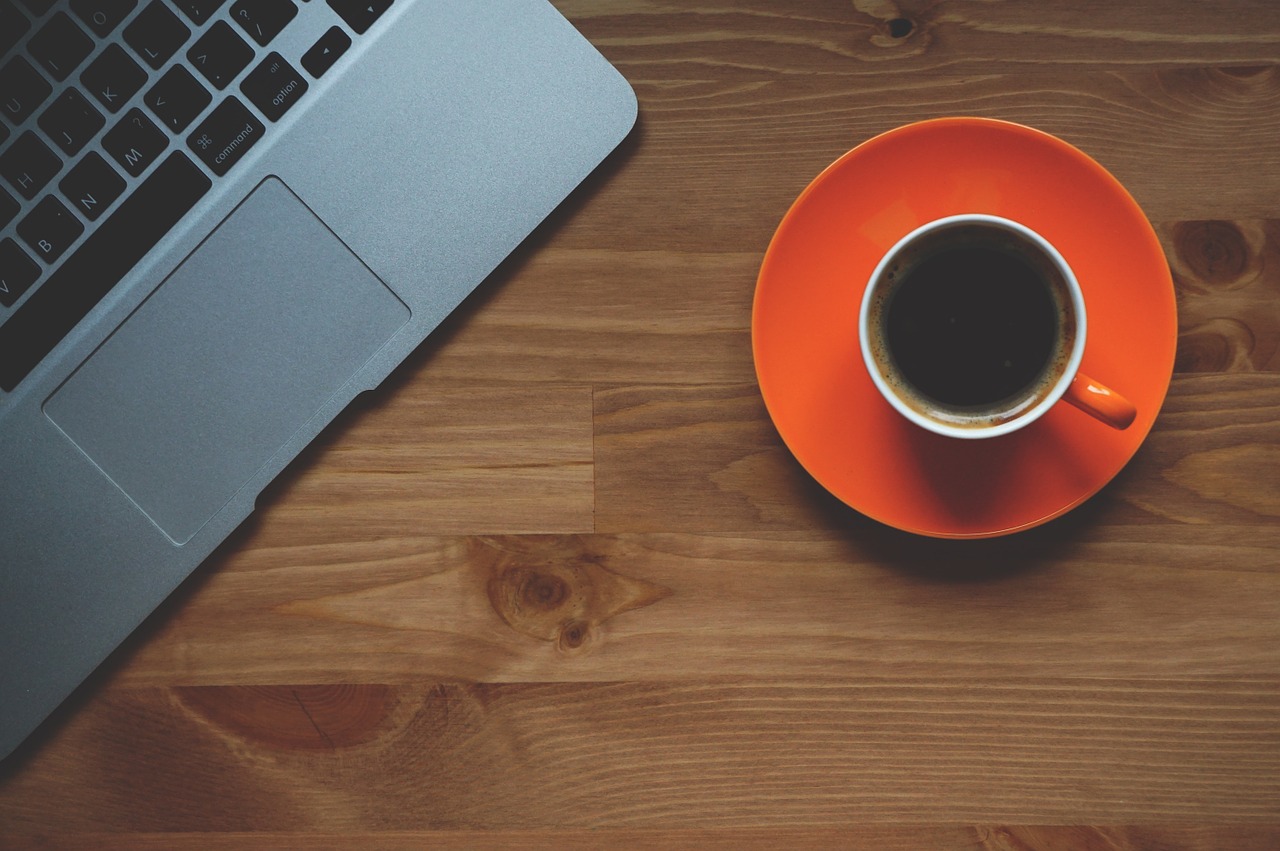
Fixing Comparator
Fun experience on fixing a custom comparator by identifying the sub-problems, testing different combinations, and finally fix it.
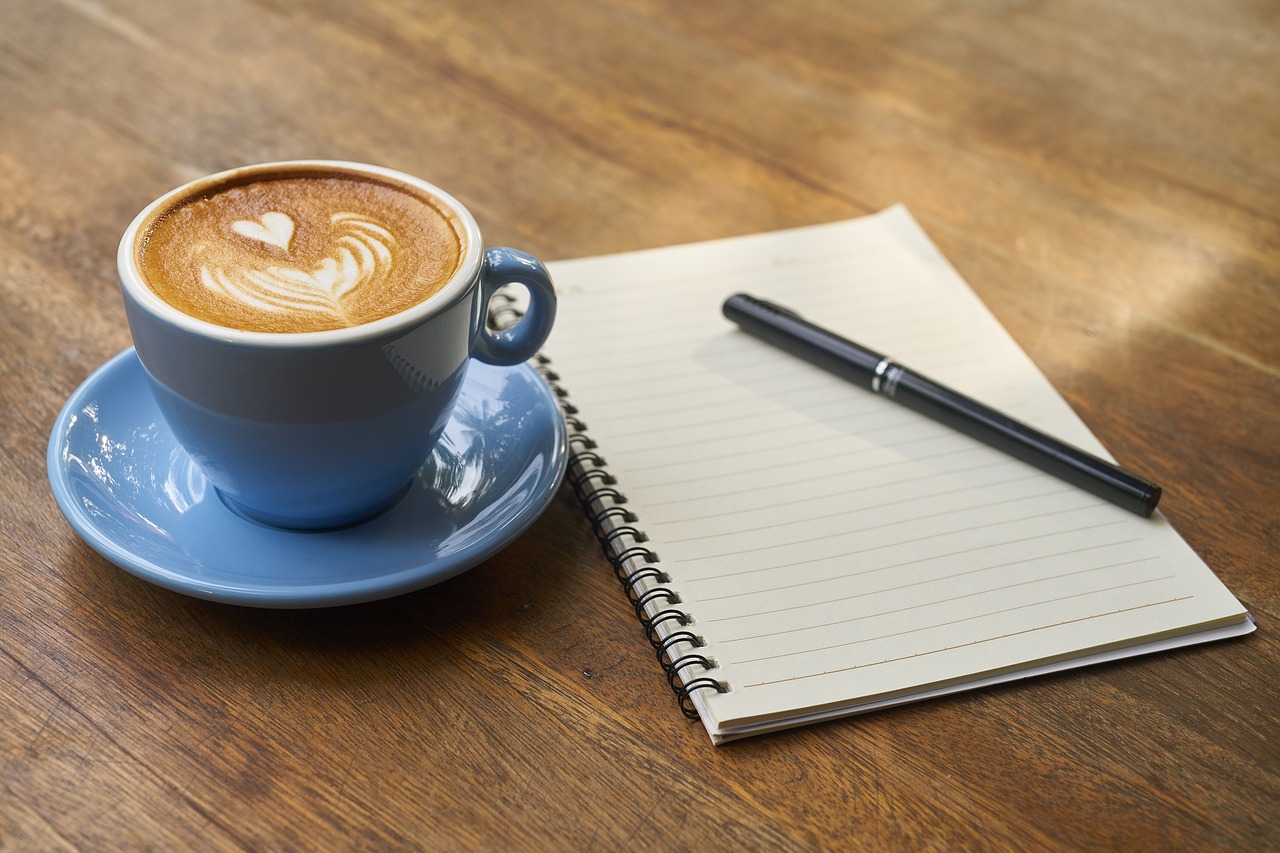
Design Pattern: Static Factory Method
Understand static factory method pattern in Java with concrete examples from Selenium WebDriver, Jackson JSON object mapper, and SAX reader for XML.
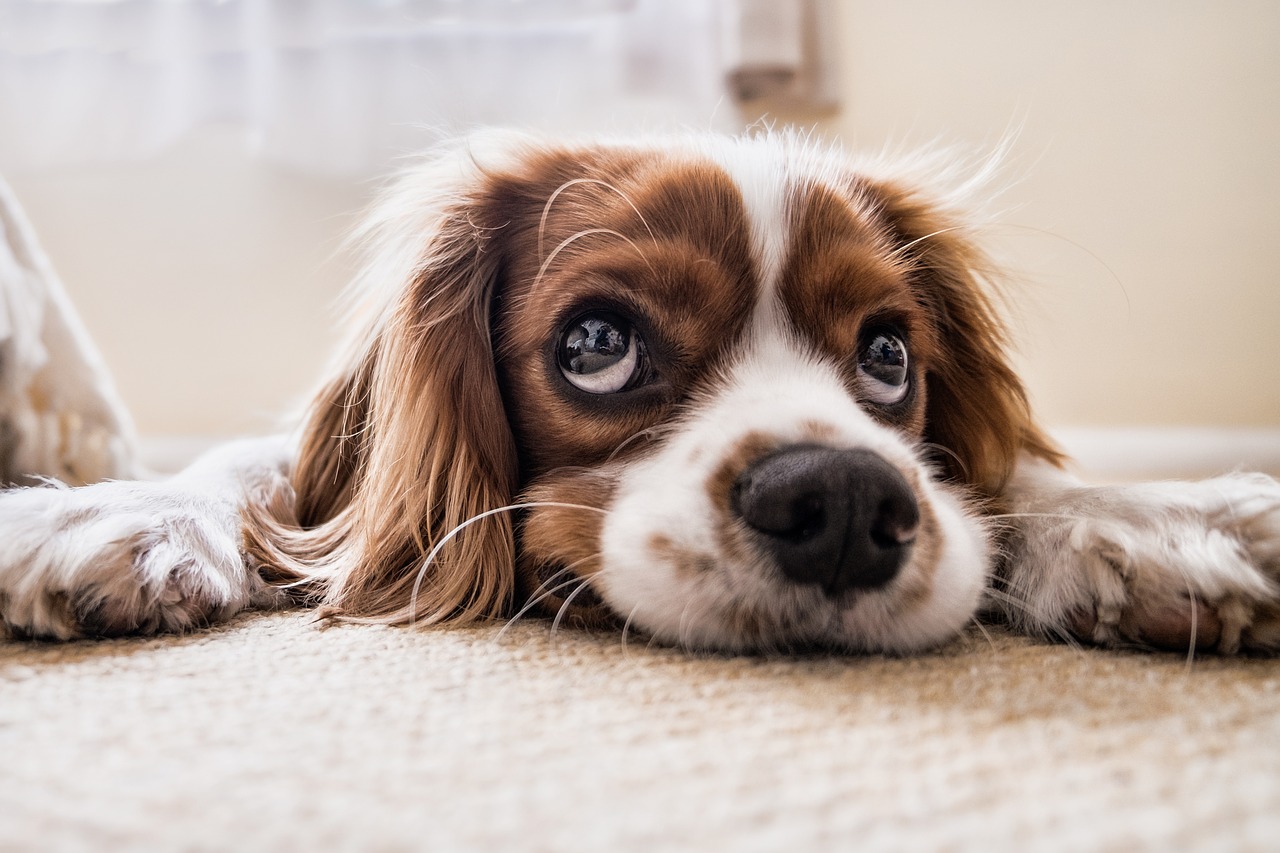
TDD: After 3 Months' Practice
I started TDD in all my personal projects 3 months ago. Here're some thoughts about it, including architecture, IDE, methodology, execution speed up, legacy code, and limits.
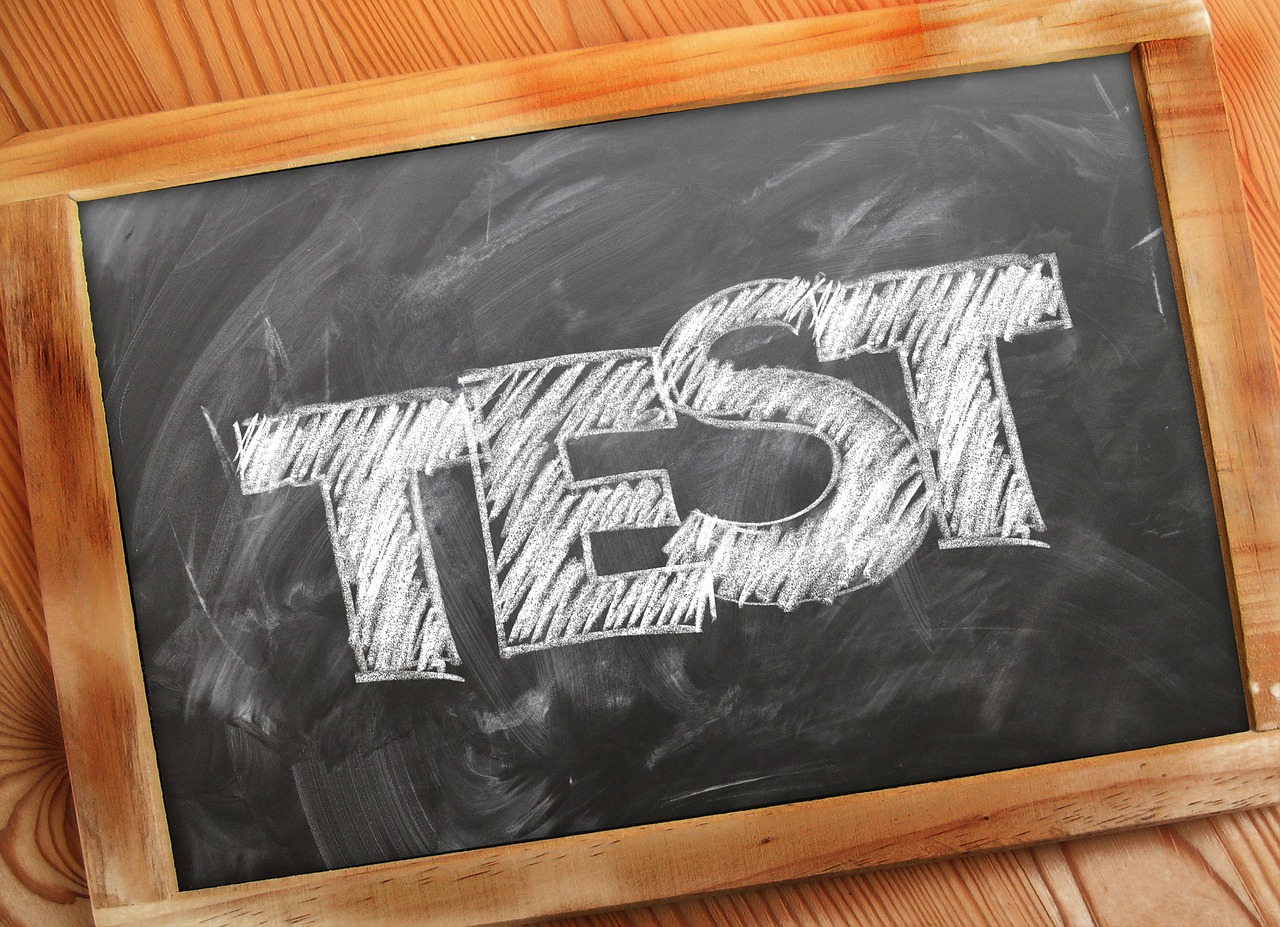
Test Polymer 2 Using WCT
Web Component Tester (WCT) allows you to test your Polymer elements easily. This article explains how to launch tests locally, set up the tests on CI, detect browsers, and write unit tests using WCT.
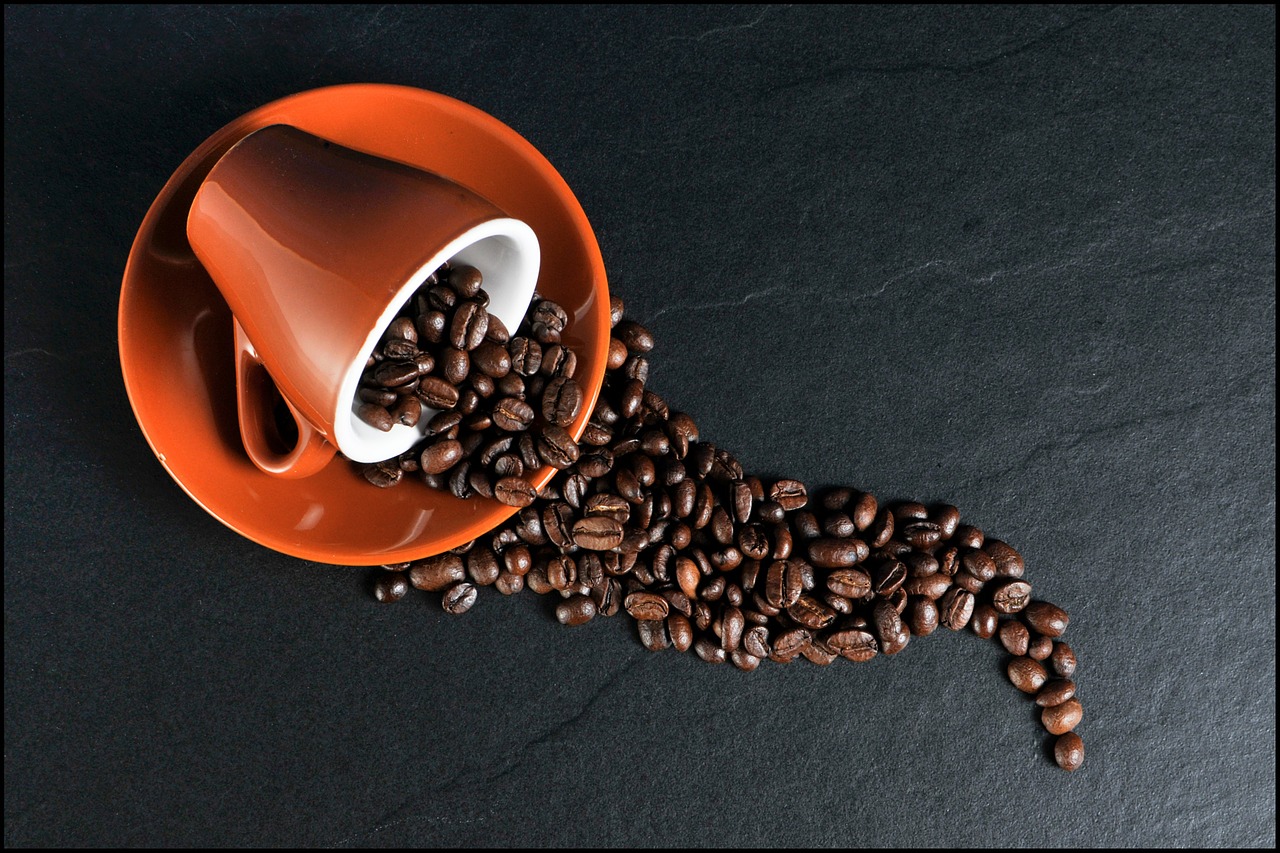
Testing JAX-RS Resources
This article explains how to set up and tear down a Grizzly Server for testing JAX-RS resources, how to create a HTTP request and assert the response using JUnit 4. And finally, the limits of testing API in reality.
Testing Haskell
This post explains how to configure your Haskell project for testing, using Cabal and Travis CI.
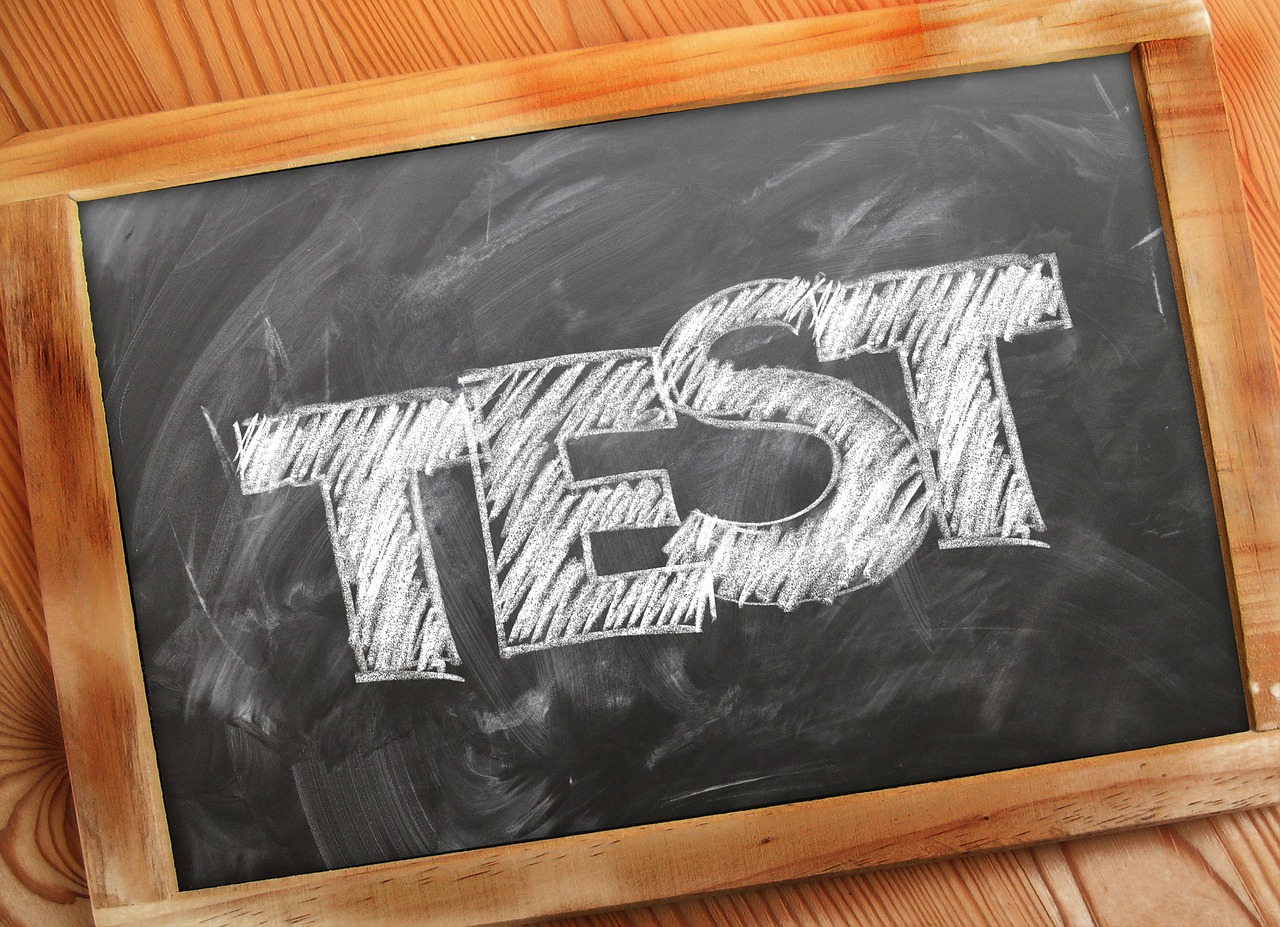
Introduction to Selenium WebDriver
A quick introduction to Selenium WebDriver, a practical tool for running functional tests and browser automation. The sample is written with Firefox 58 and GeckoDriver 0.20.