Java is a general-purpose computer-programming language that is concurrent, class-based, object-oriented, and specifically designed to have as few implementation dependencies as possible.
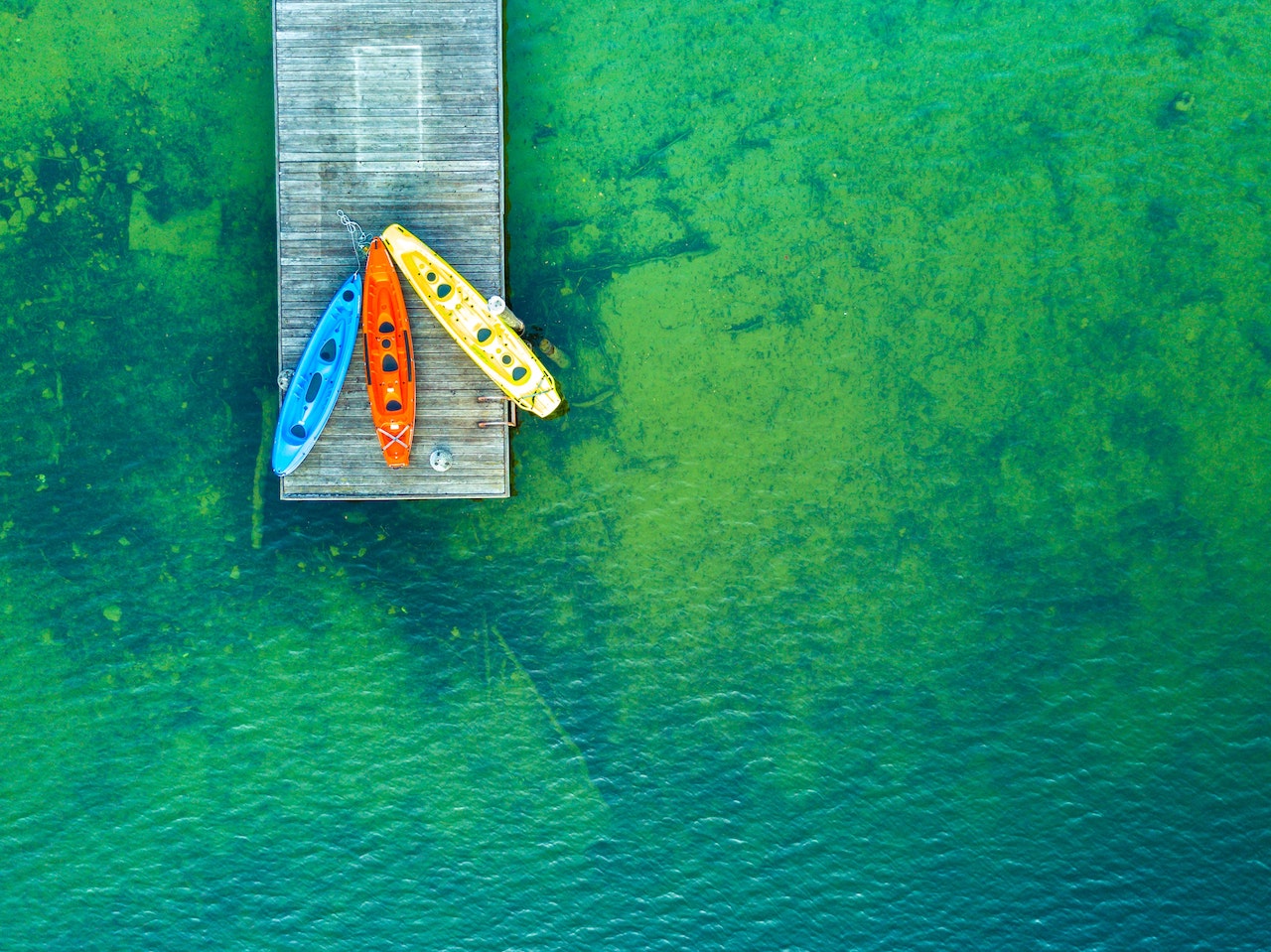
Sign in with Google
This article shares the keynotes for implementing a "Sign-in with Google" mechanism for a web application based on OAuth 2.0.
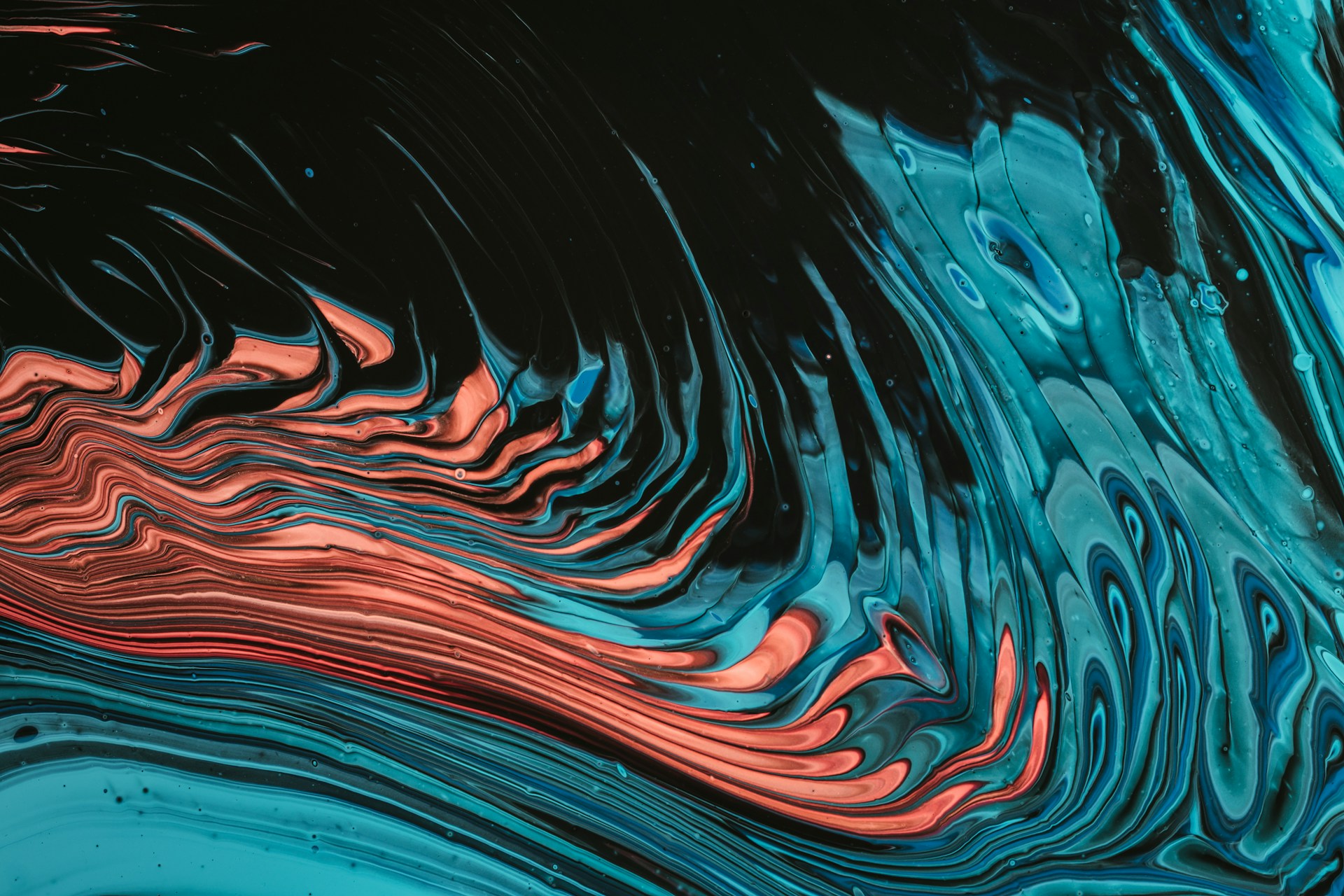
Implementing Search-as-you-type in "ChatGPT QuickSearch"
The mechanism of the search-as-you-type feature for the Chrome extension "ChatGPT QuickSearch".
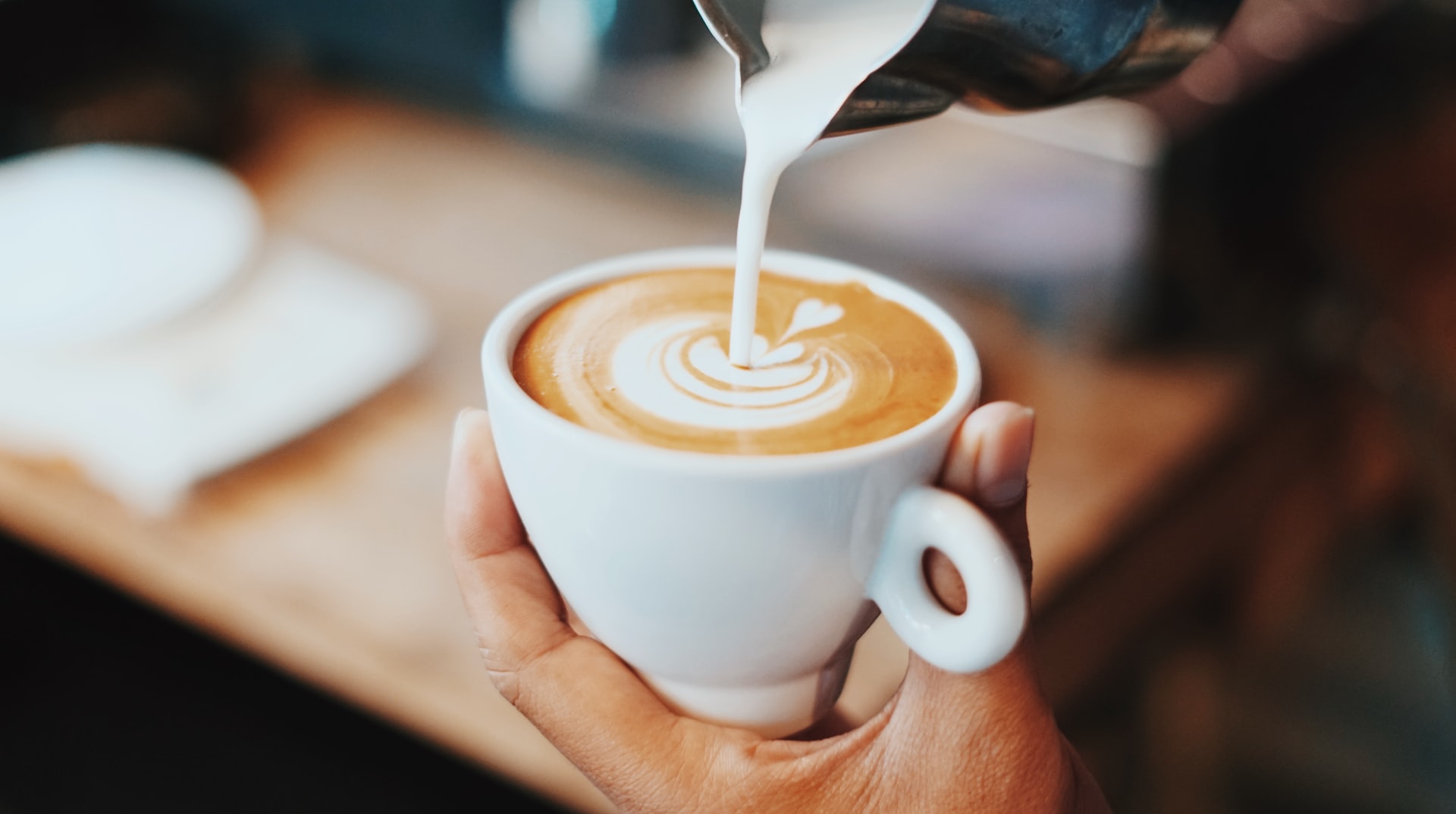
OpenAPI Overview
The key concepts provided by the OpenAPI Initiative, e.g. OpenAPI spec, code generator, schema, build, api-diff, and how they may be useful for your project.
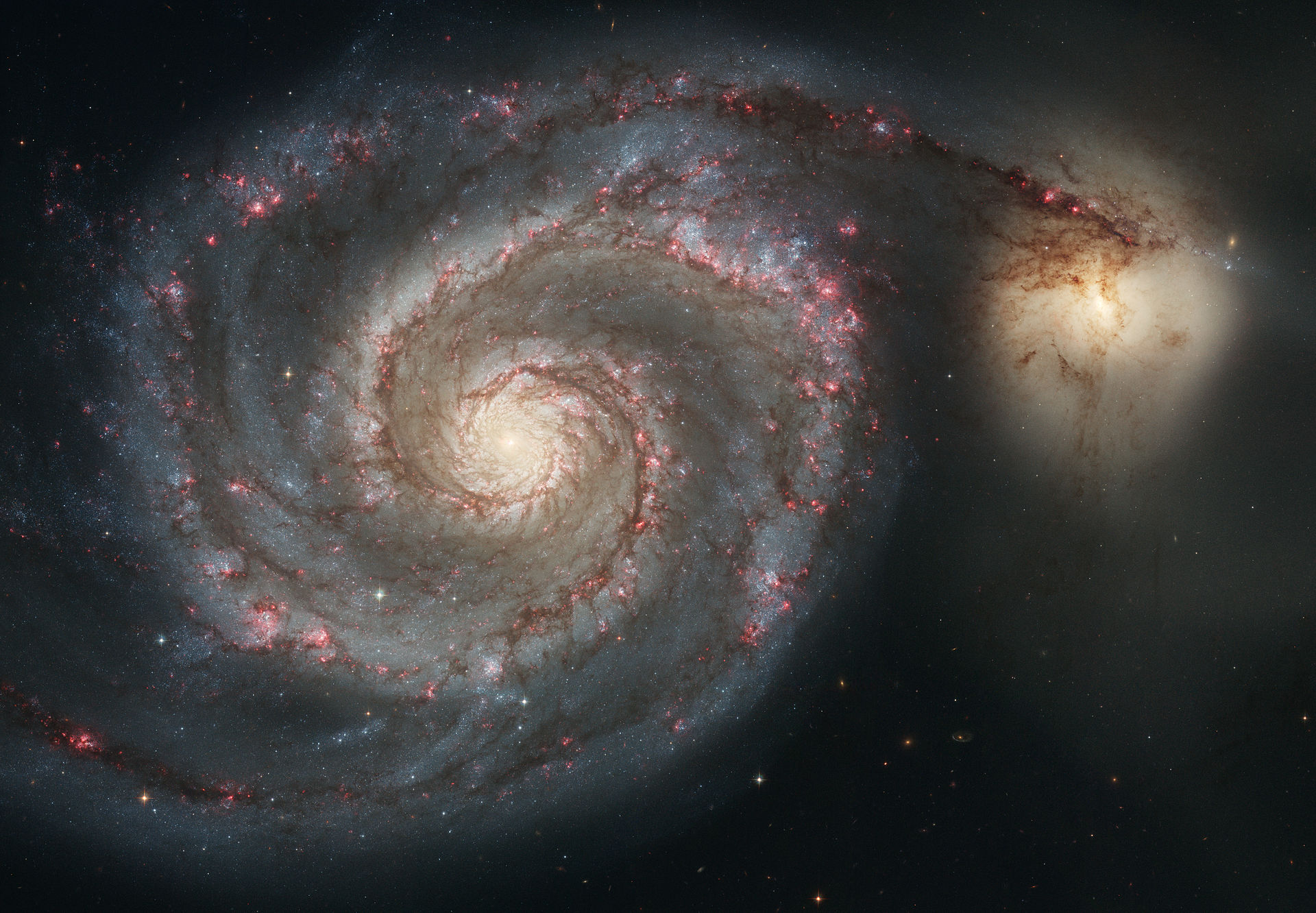
Internal Working of Temporal Java Service Client
The internal working of Temporal Java service client: module structure, protobuf messages, code generation, data conversion, authorization service stubs, and more.
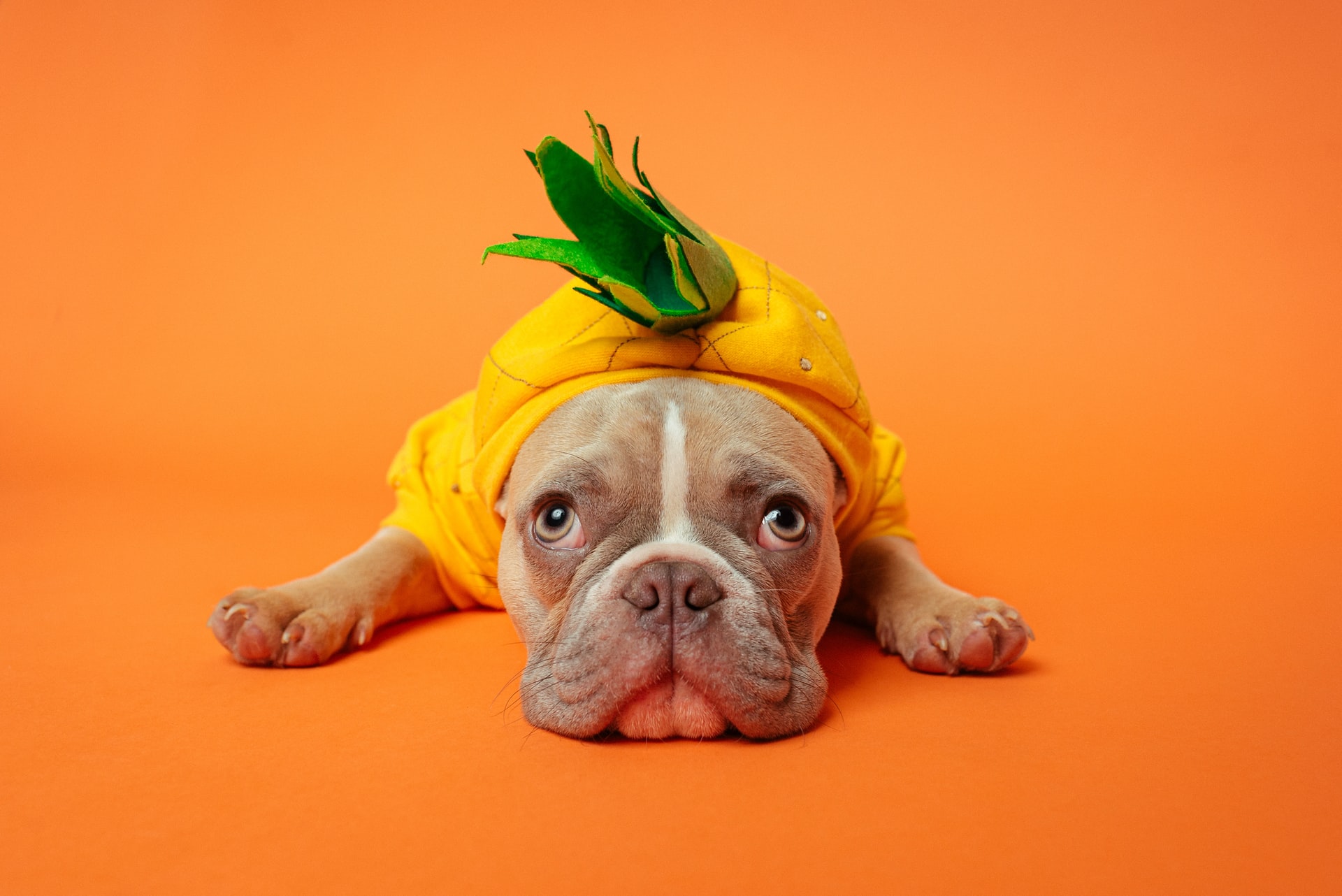
My Hackathon Projects At Datadog
This article shared the 5 projects that I did over the last hackathons in Datadog and the lessons learned from those experiences.
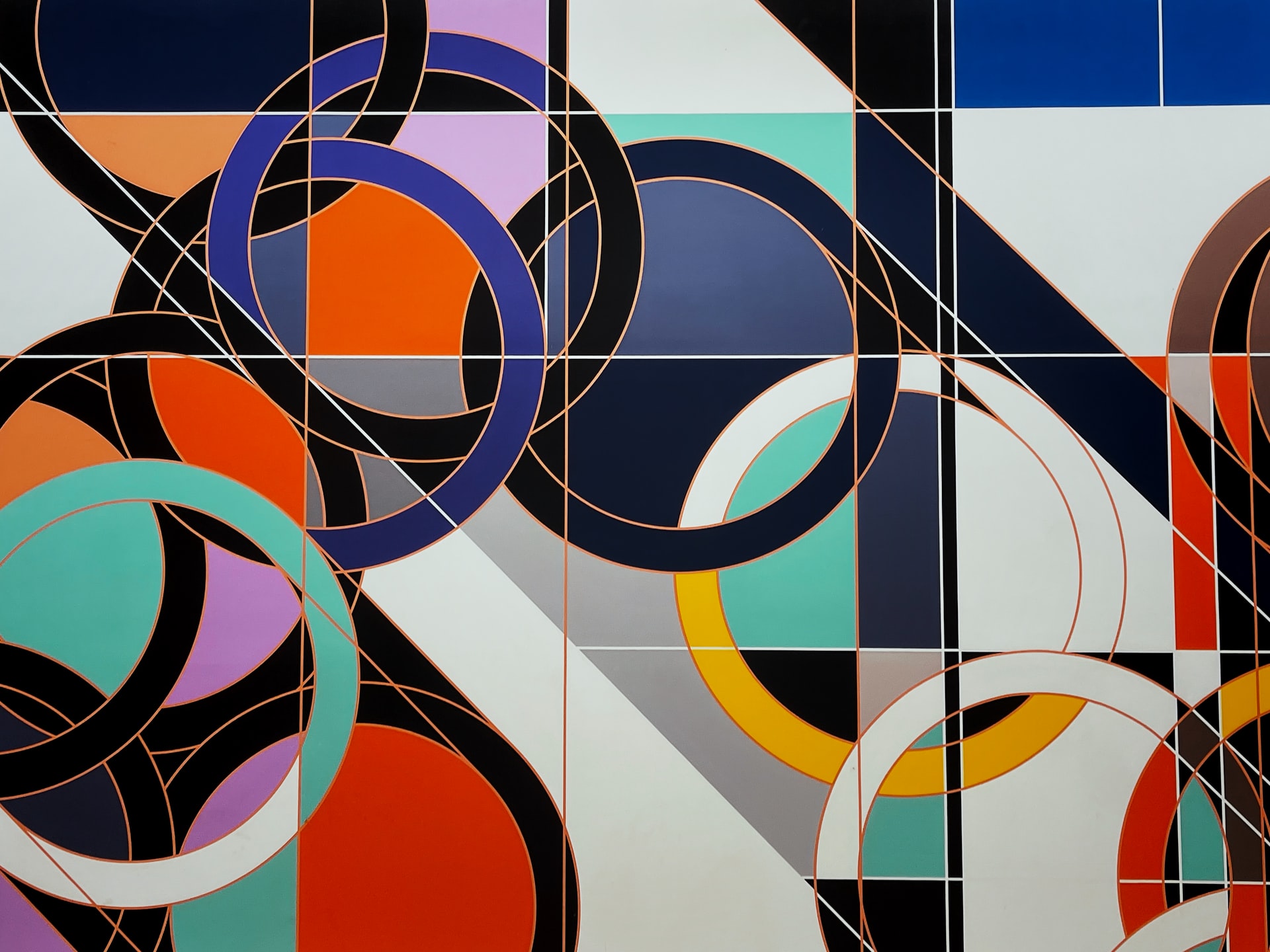
Managing Code Complexity
This article discusses how to manage code complexity when the codebase is getting more and more complex, how to refactor continuously, and what kinds of strategies you need to succeed.
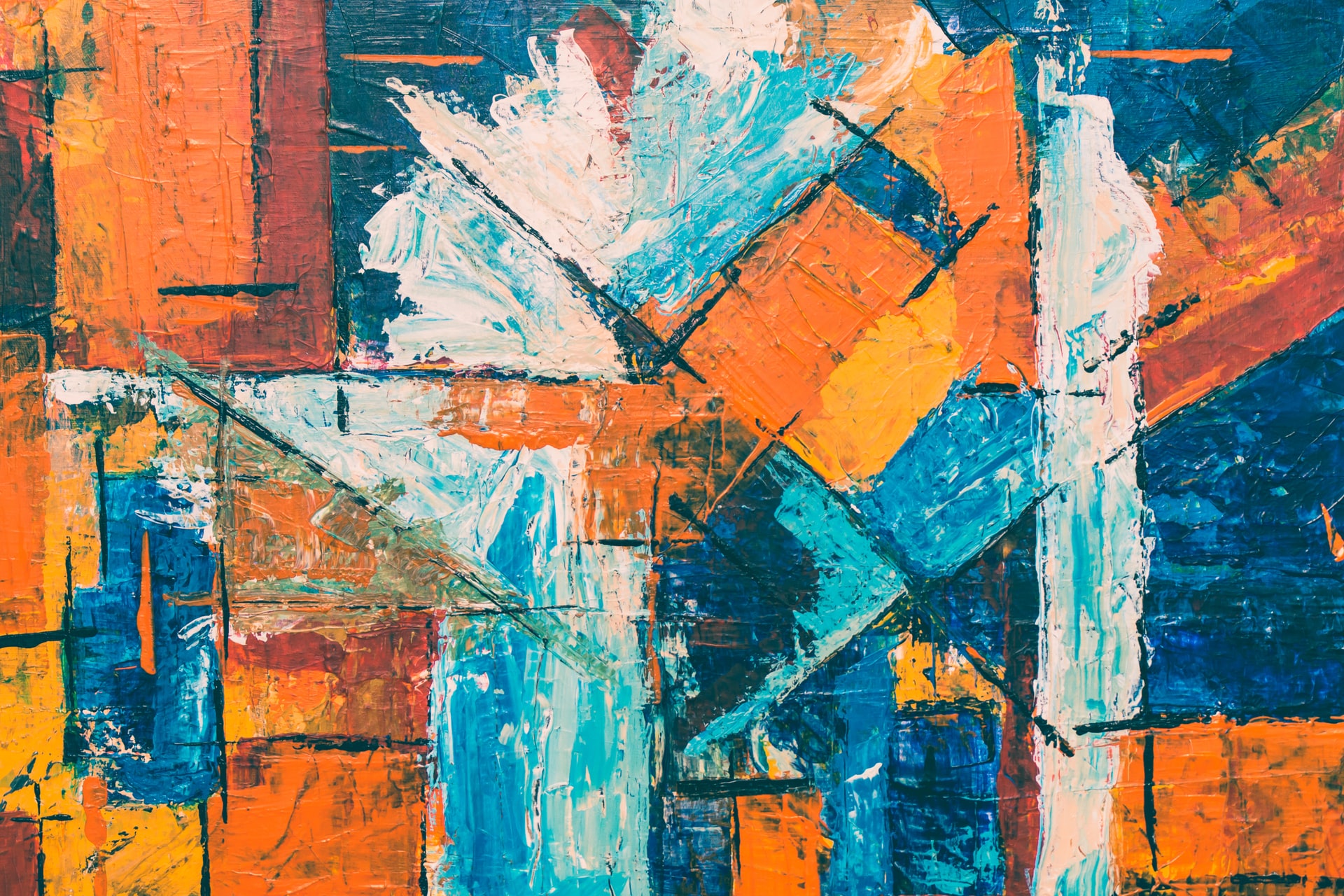
BlogSearch Architecture
The overview of the BlogSearch, a simple search service for blog posts.
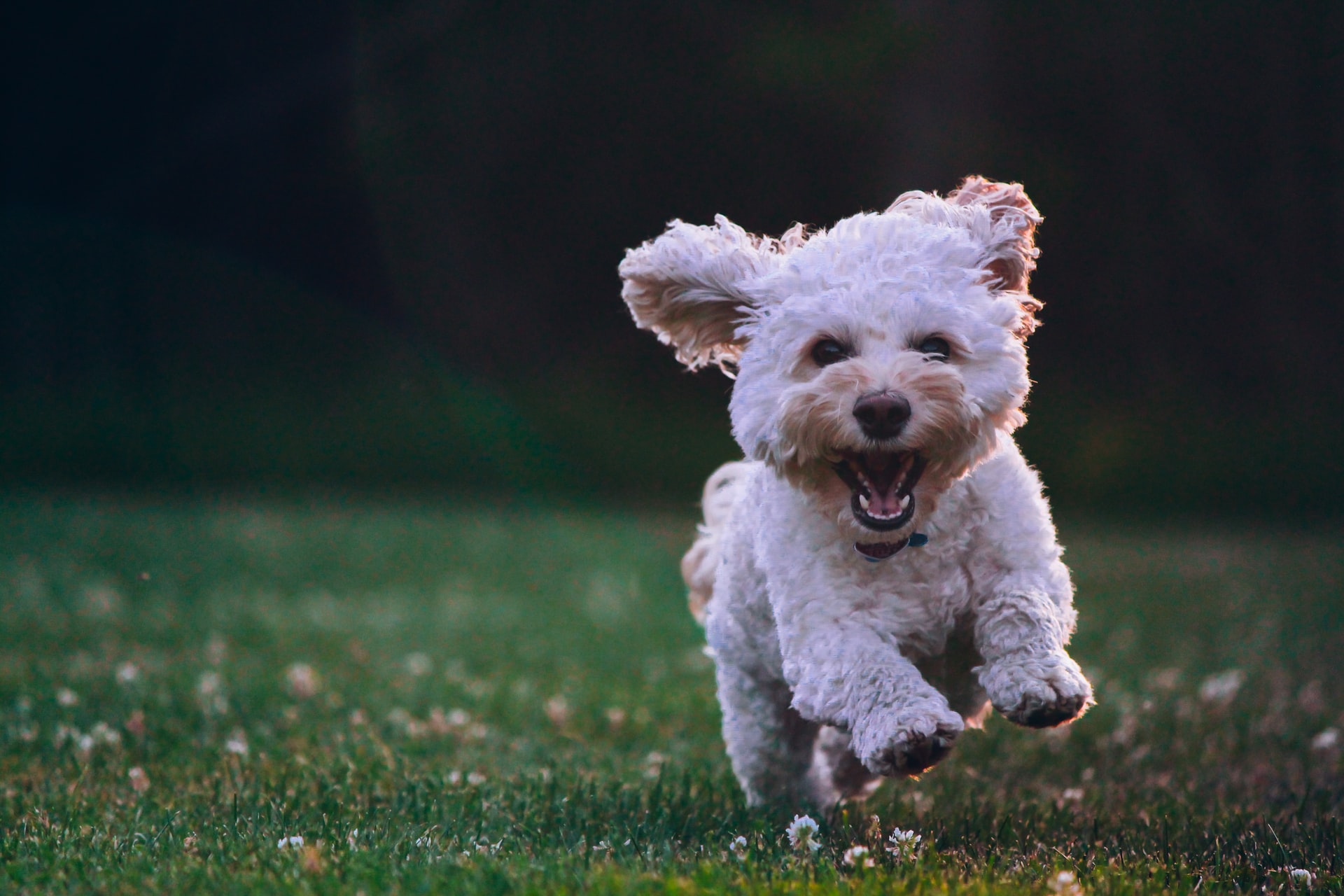
Setting Up Monitoring For Java With Datadog
This article explains how to set up Datadog monitoring for a Java application and an Elasticsearch service running in Alibaba Cloud.
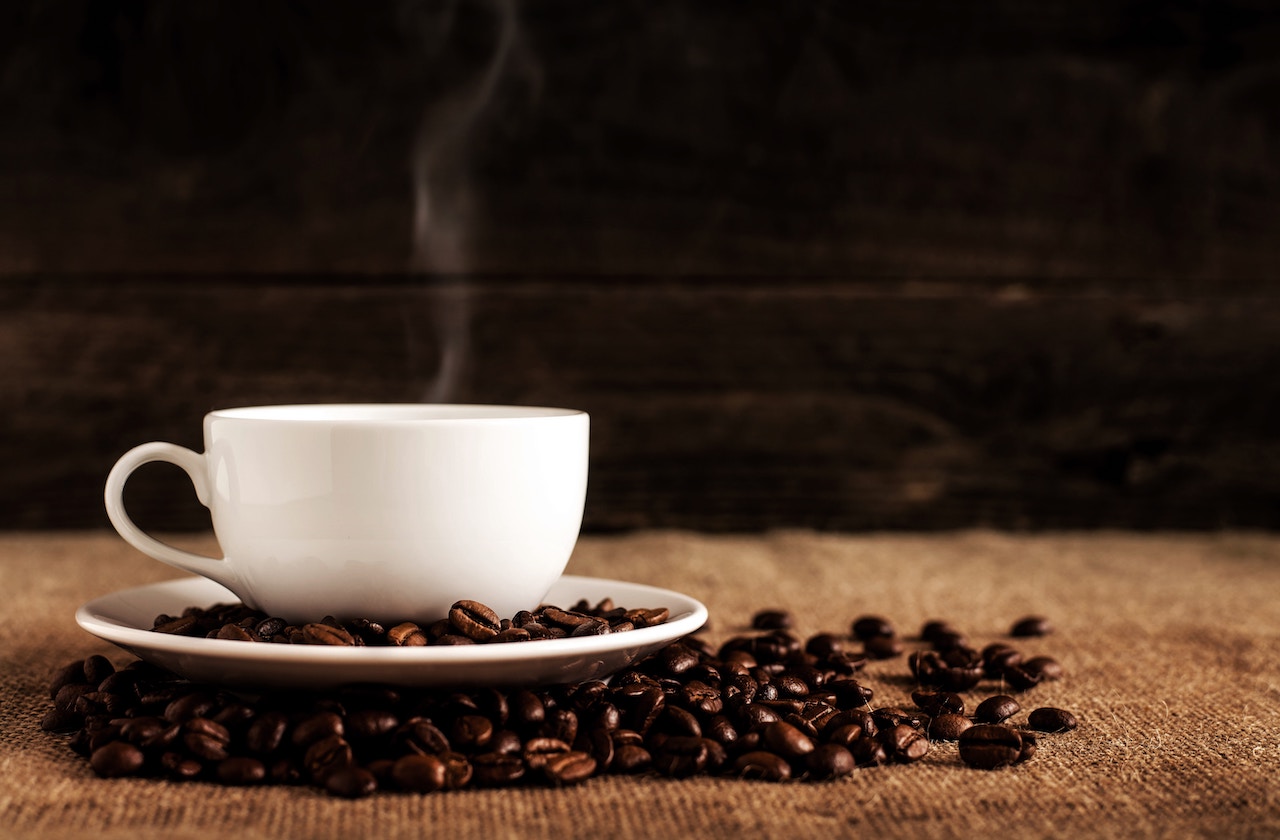
Internal Structure Of Elasticsearch Java High-Level REST Client
This article explores the implementation of Elasticsearch Java High-Level REST Client (HLRC) by analyzing the structure of the client, the dependencies, the error handling mechanism, serialization, its observability, and more.
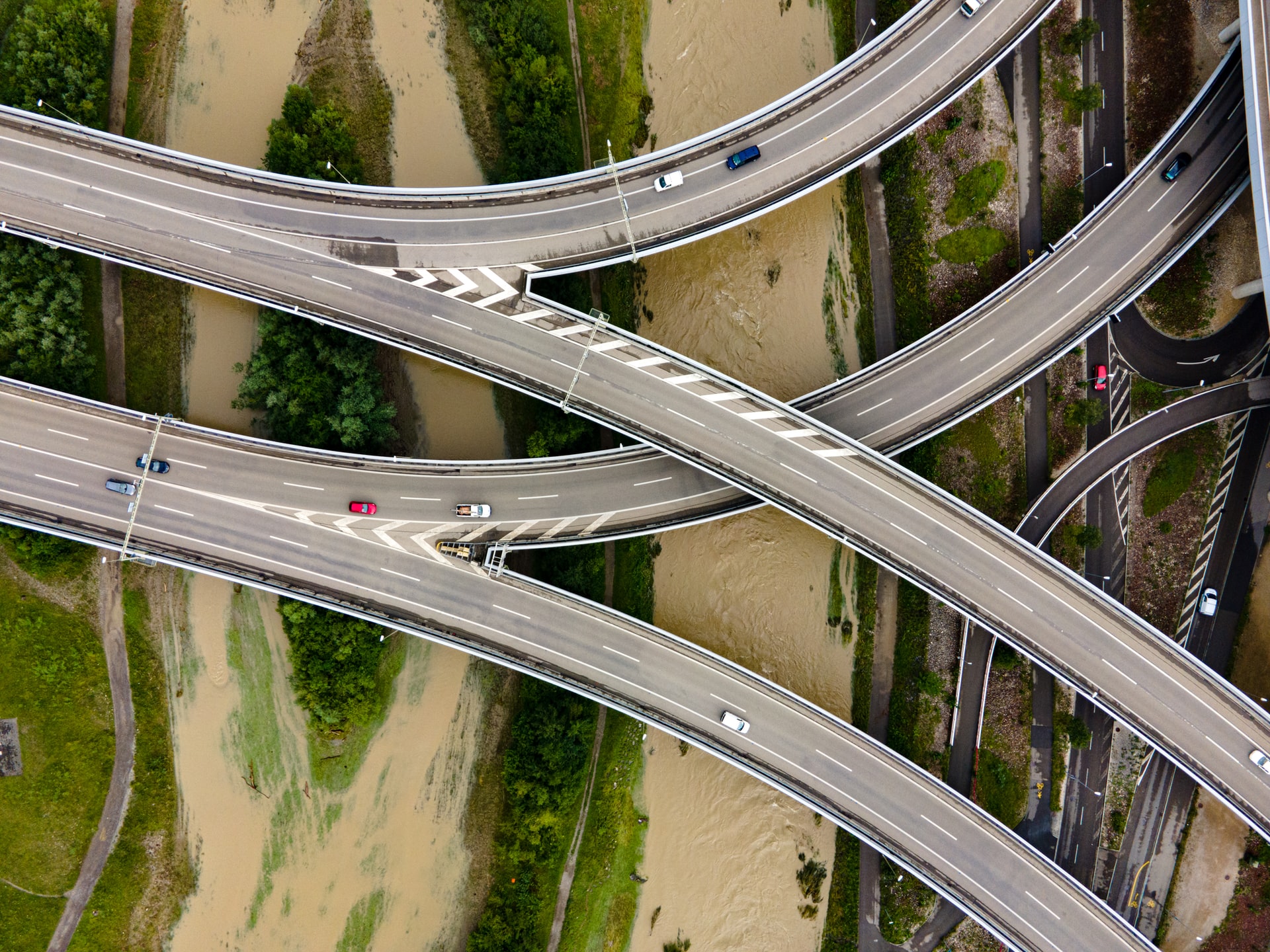
The Decision System For Shard Allocation in Elasticsearch
Curious about how does a decision system work? This article explains the deciders for shard allocation in Elasticsearch by going through their responsibility, structure, decision making, lifecycle, testing, and more.
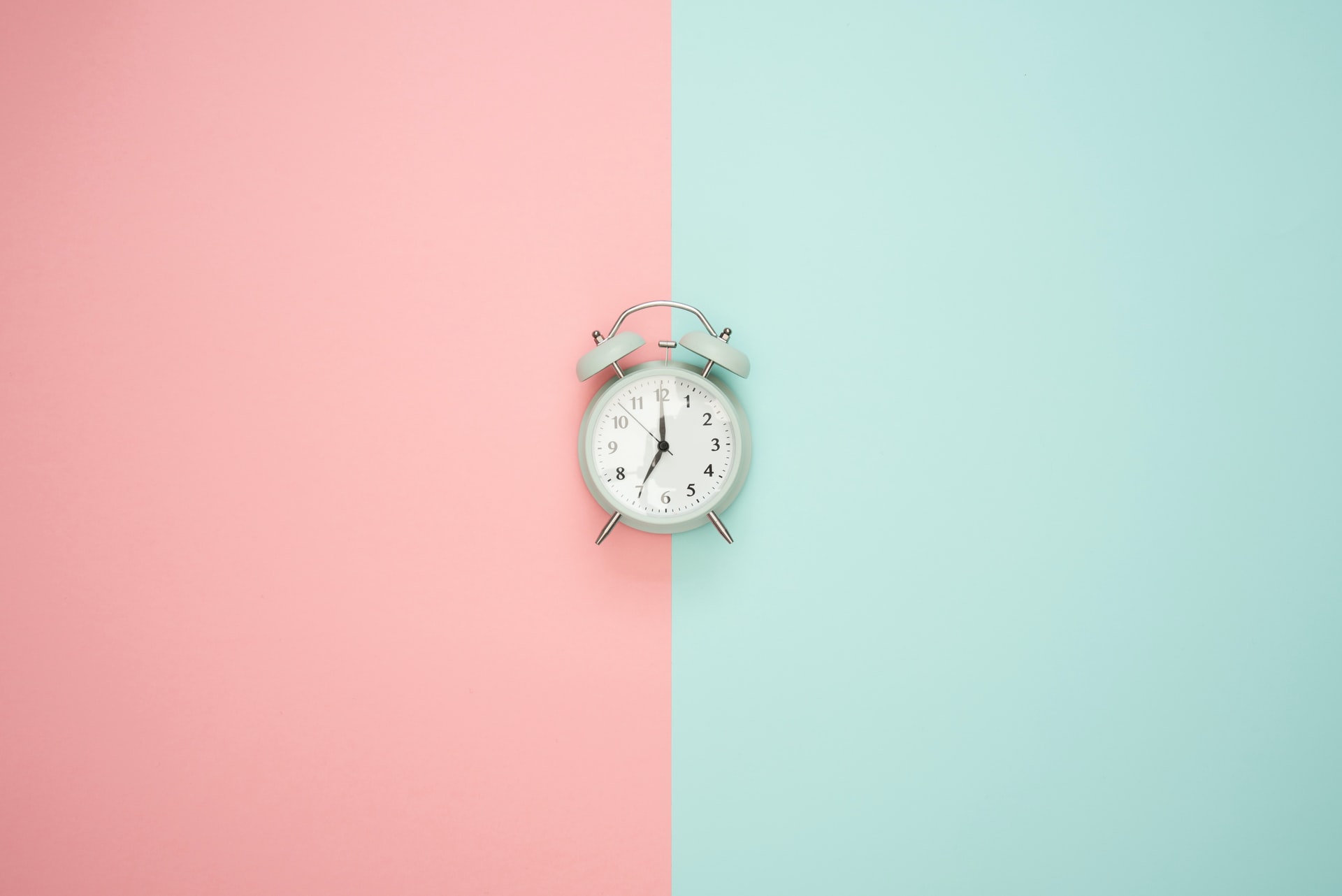
Change Log Level At Runtime in Logback
This Q&A explains how to change logger level dynamically at runtime via RESTful API for your Java application when using Logback.
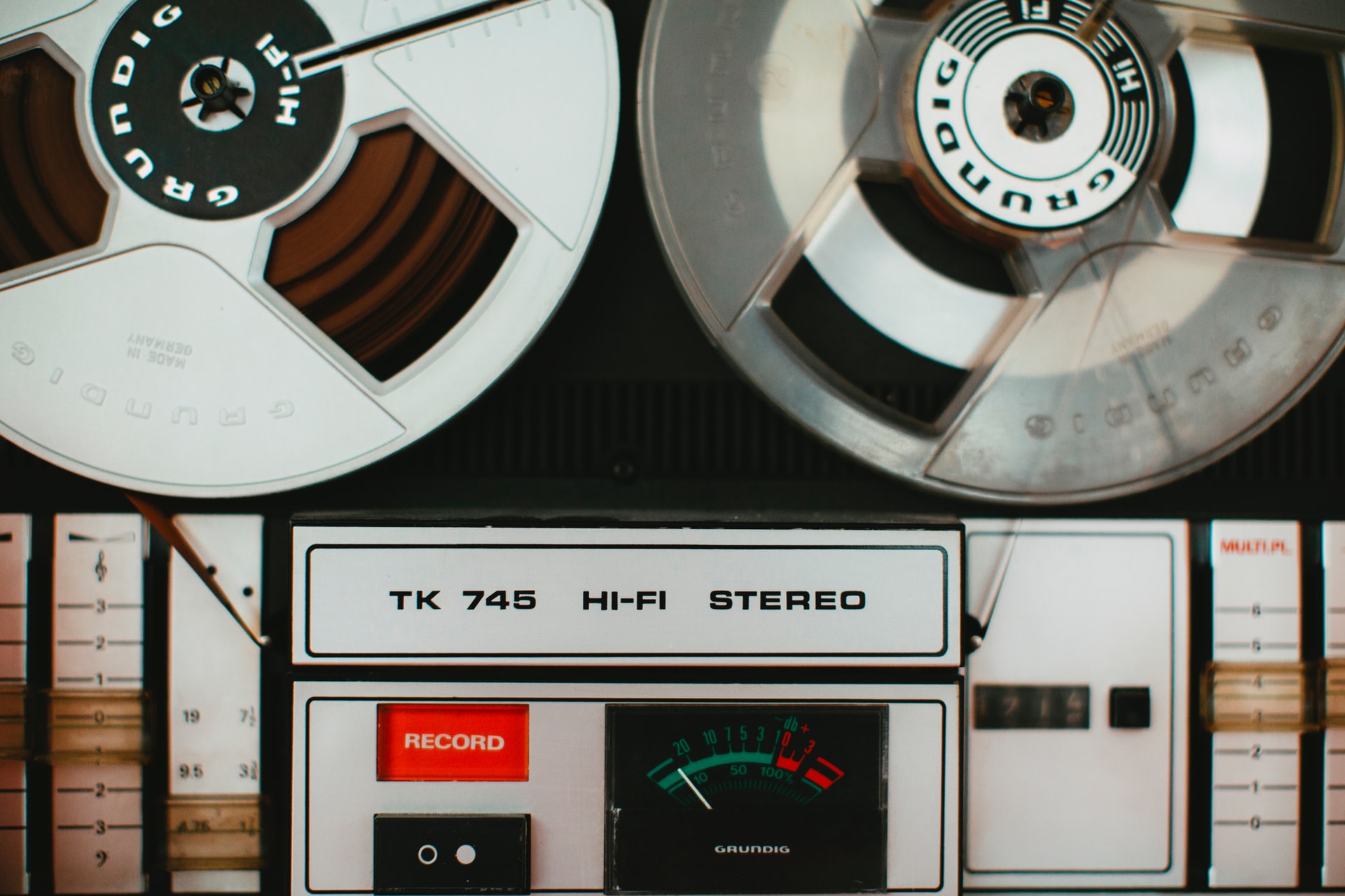
Audit Logs
This article discusses how to implement a simple audit logs solution with Java JAX-RS, including requirements, considerations for the implementation, and 3 different solutions based on Java Servlet, Jetty, and JAX-RS filter.
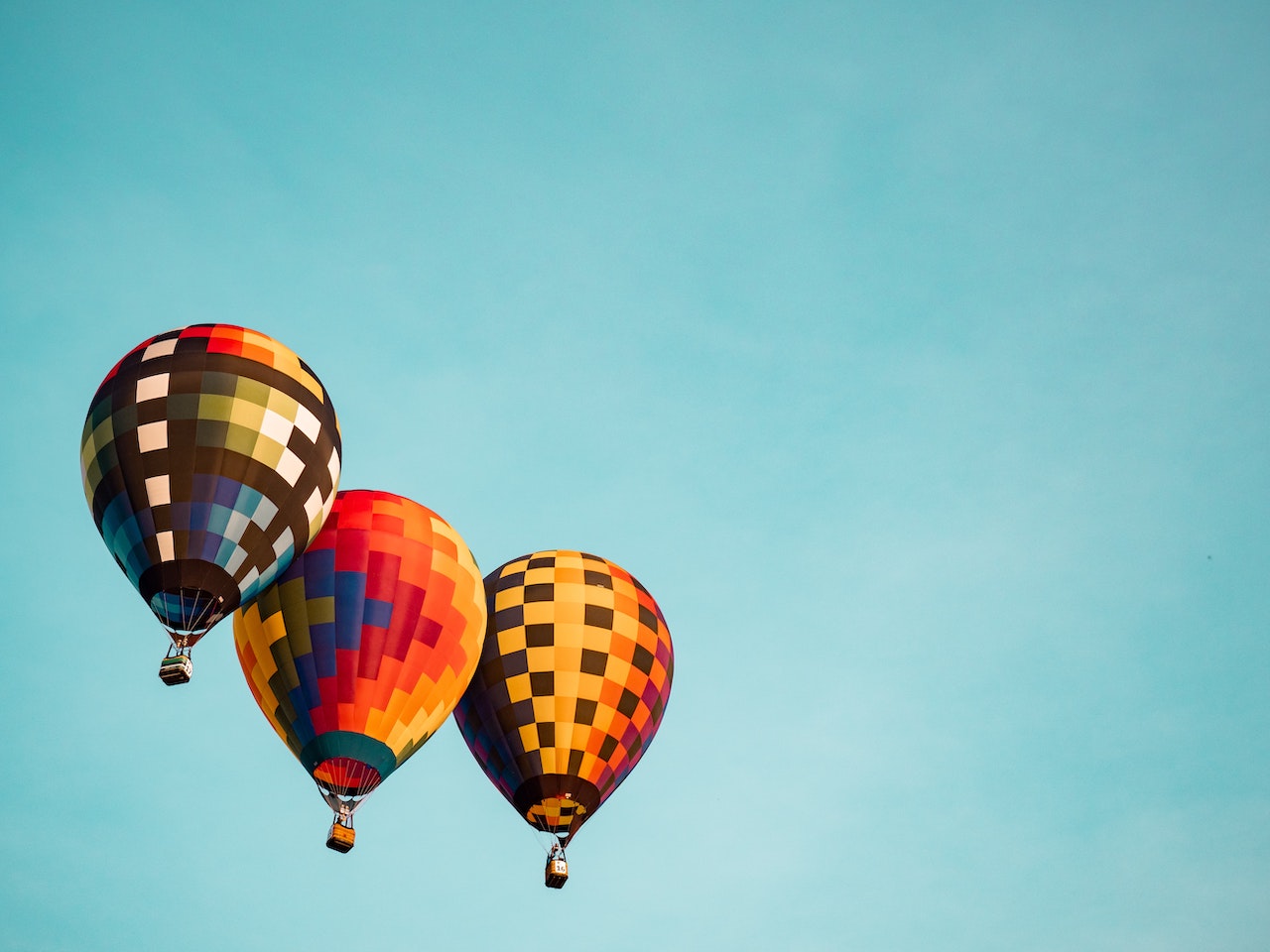
Elasticsearch Snapshot Plugins
Elasticsearch snapshot repository plugins for AWS, GCP, and Azure
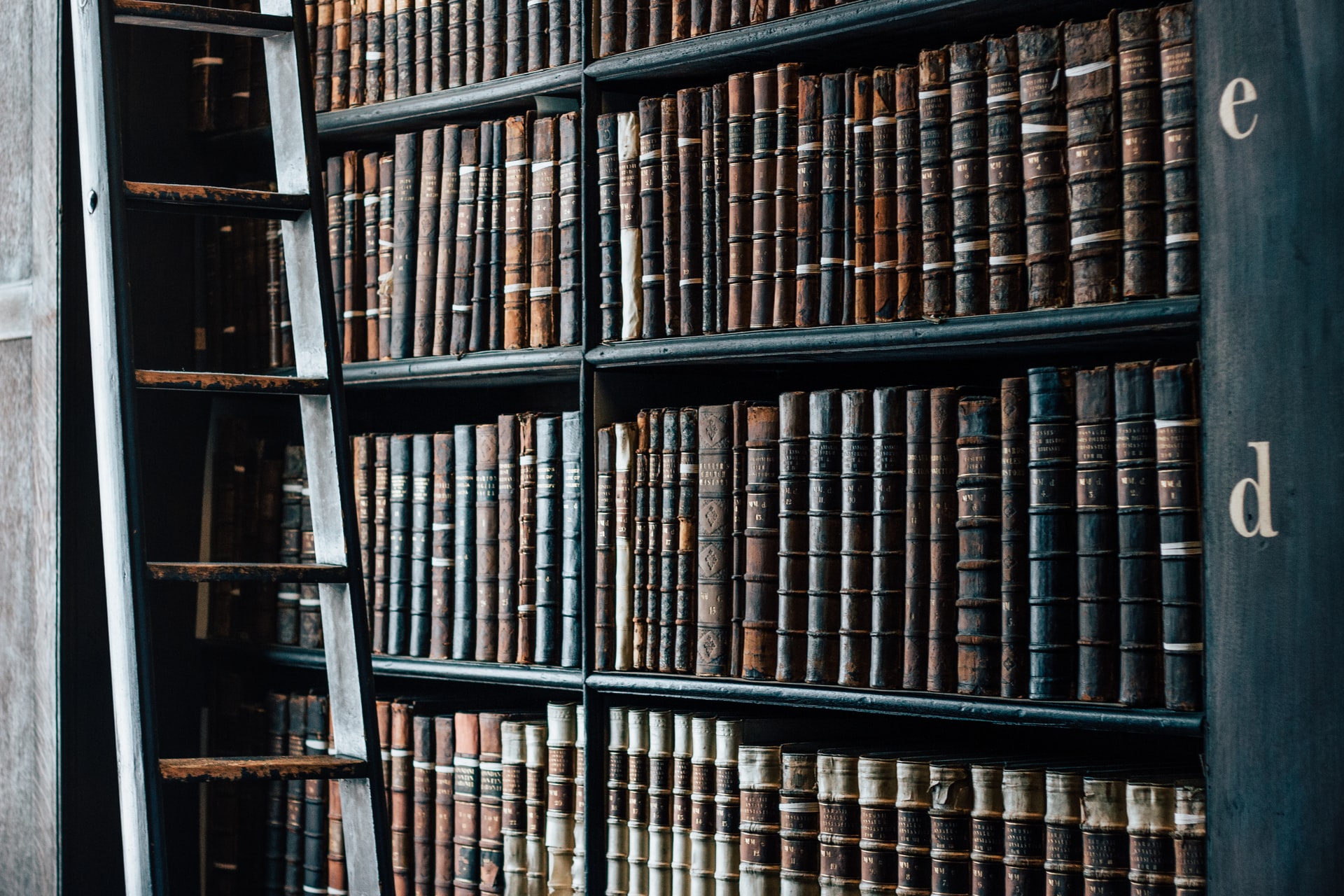
Internal Structure Of Snapshot Repository
This article takes you to the Elasticsearch snapshot repository to explore its internal structure and understand the contents and uses of different files.
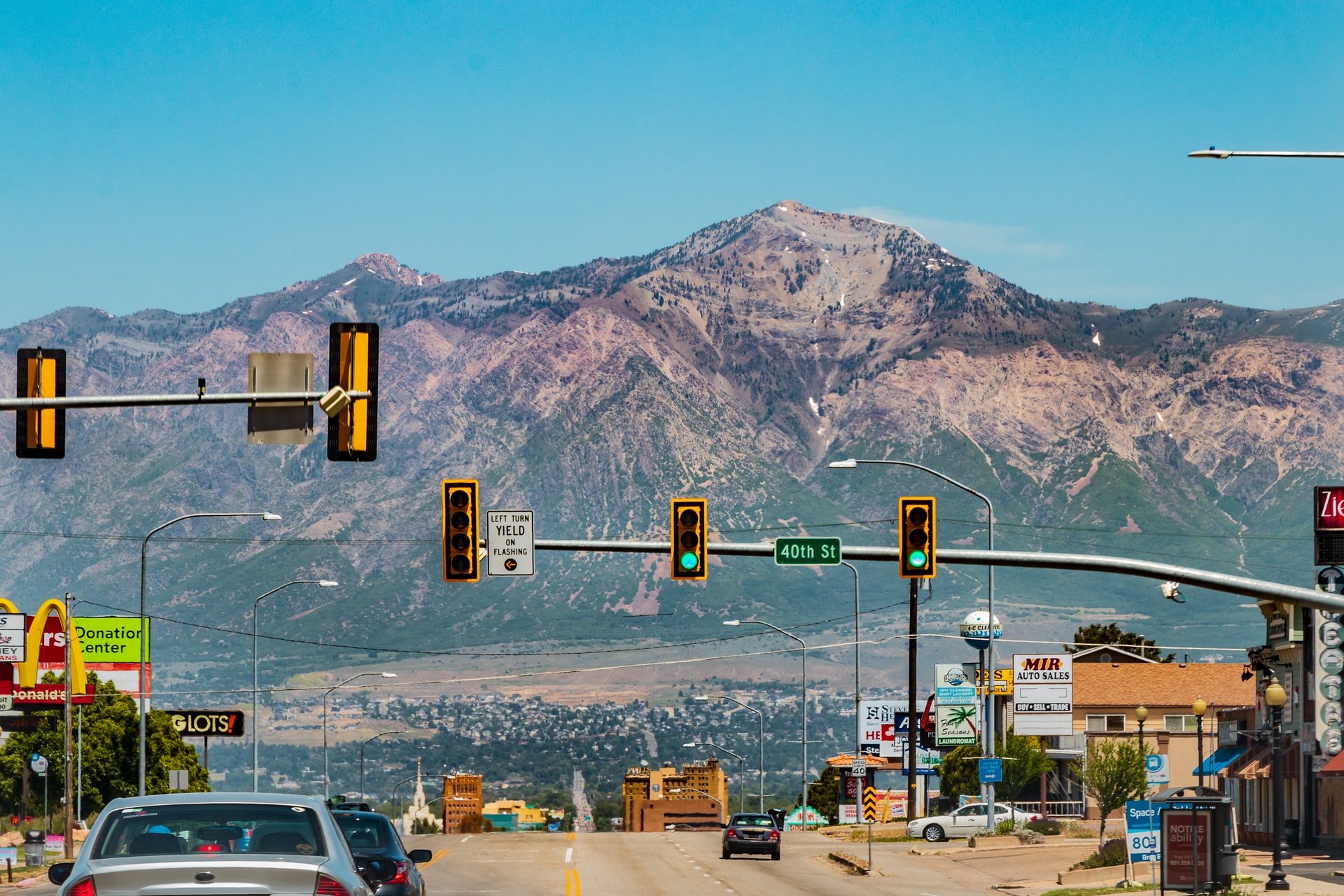
How to prevent data loss in Elasticsearch?
Six practical solutions to improve data reliability: improve number of replicas, snapshot and restore, RAID, MQ, etc.
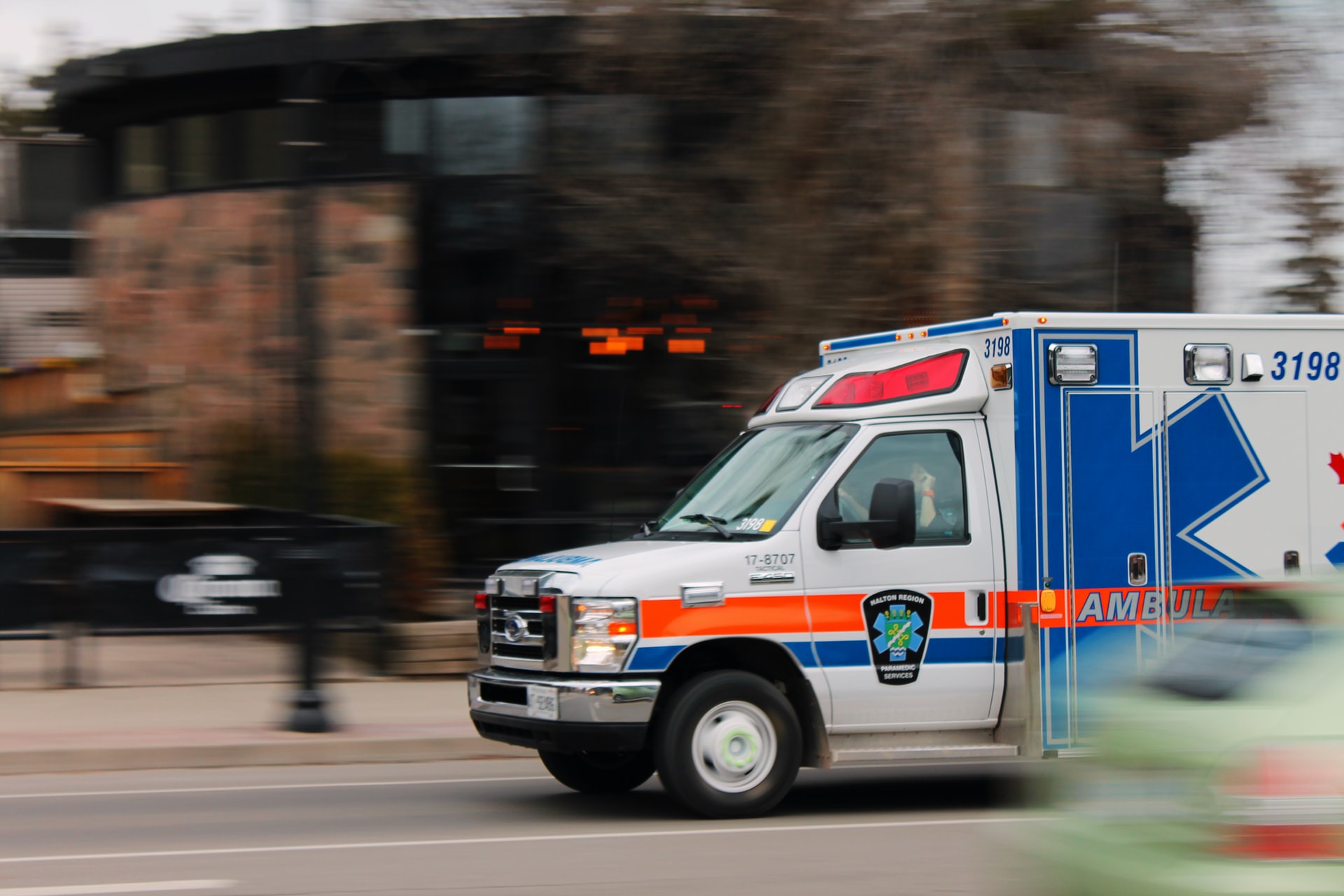
Fix Corrupted Index in Elasticsearch
How to fix corrupted index in Elasticsearch cluster without snapshot?
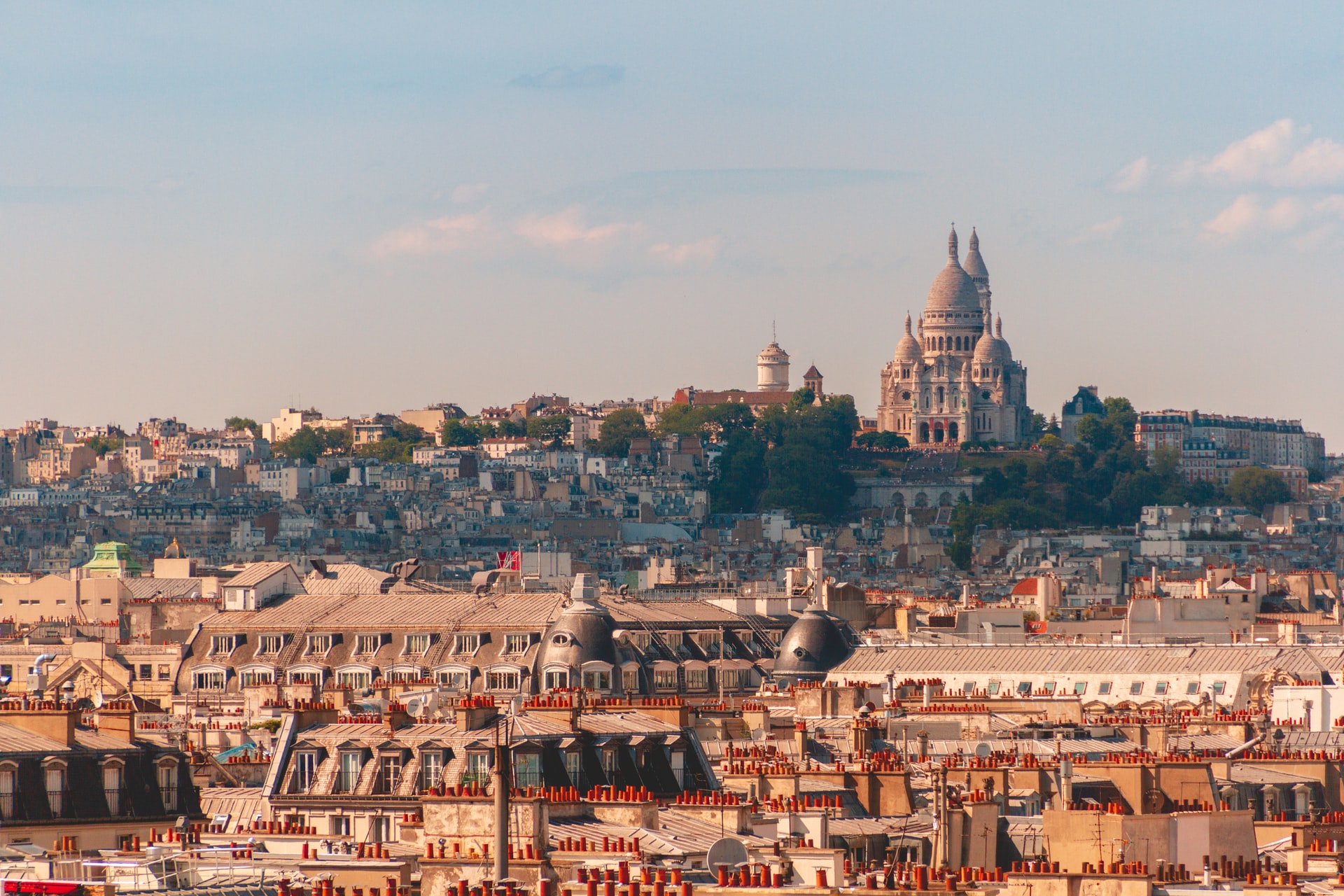
DVF: Aggregations
How to write and execute metric and bucket aggregations in Elasticsearch for dataset: Demandes de valeurs foncières (DVF) for data analytics. Also, how to execute aggregations that contain sub-aggregations.
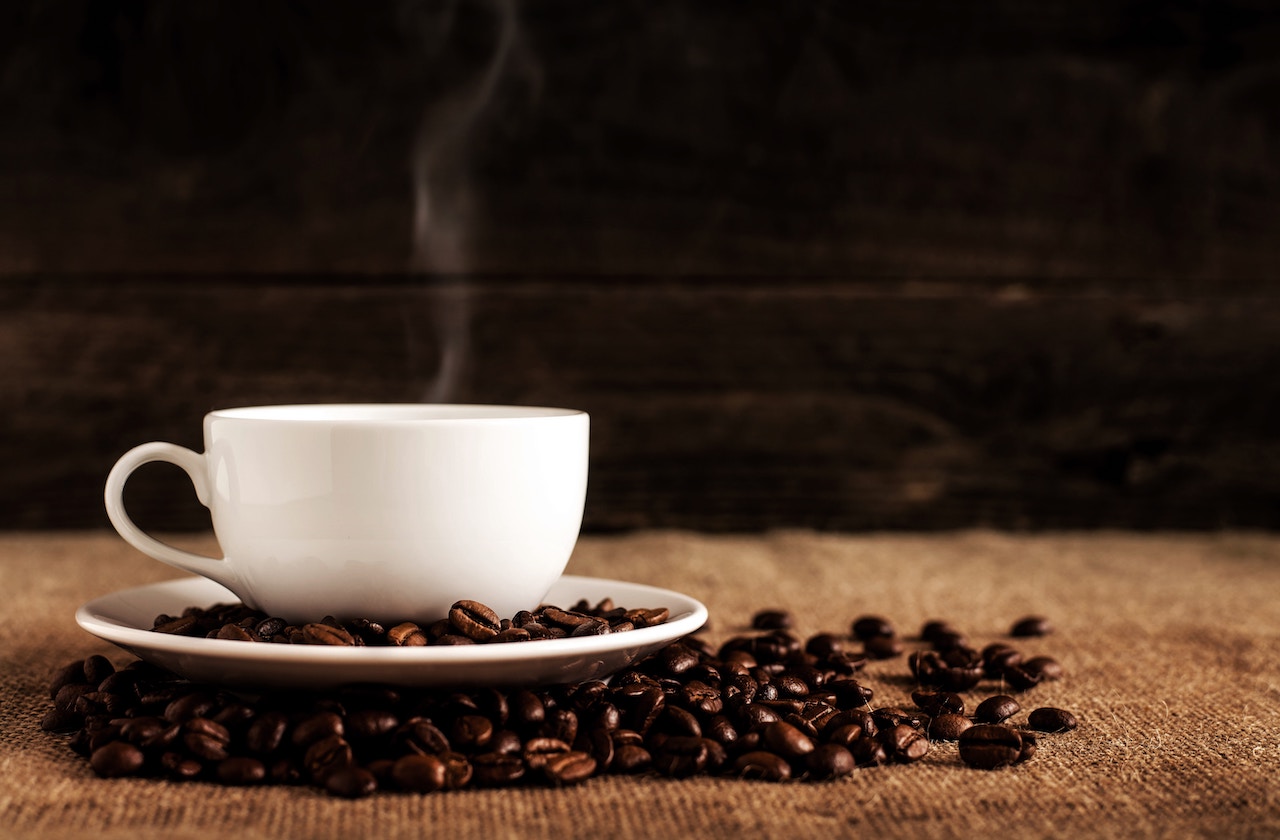
JUnit 5: Dynamic Tests with TestFactory
How to write dynamic tests using @TestFactory in JUnit 5? This article explains the syntax, different return types, the test lifecycle, and potential use-cases.
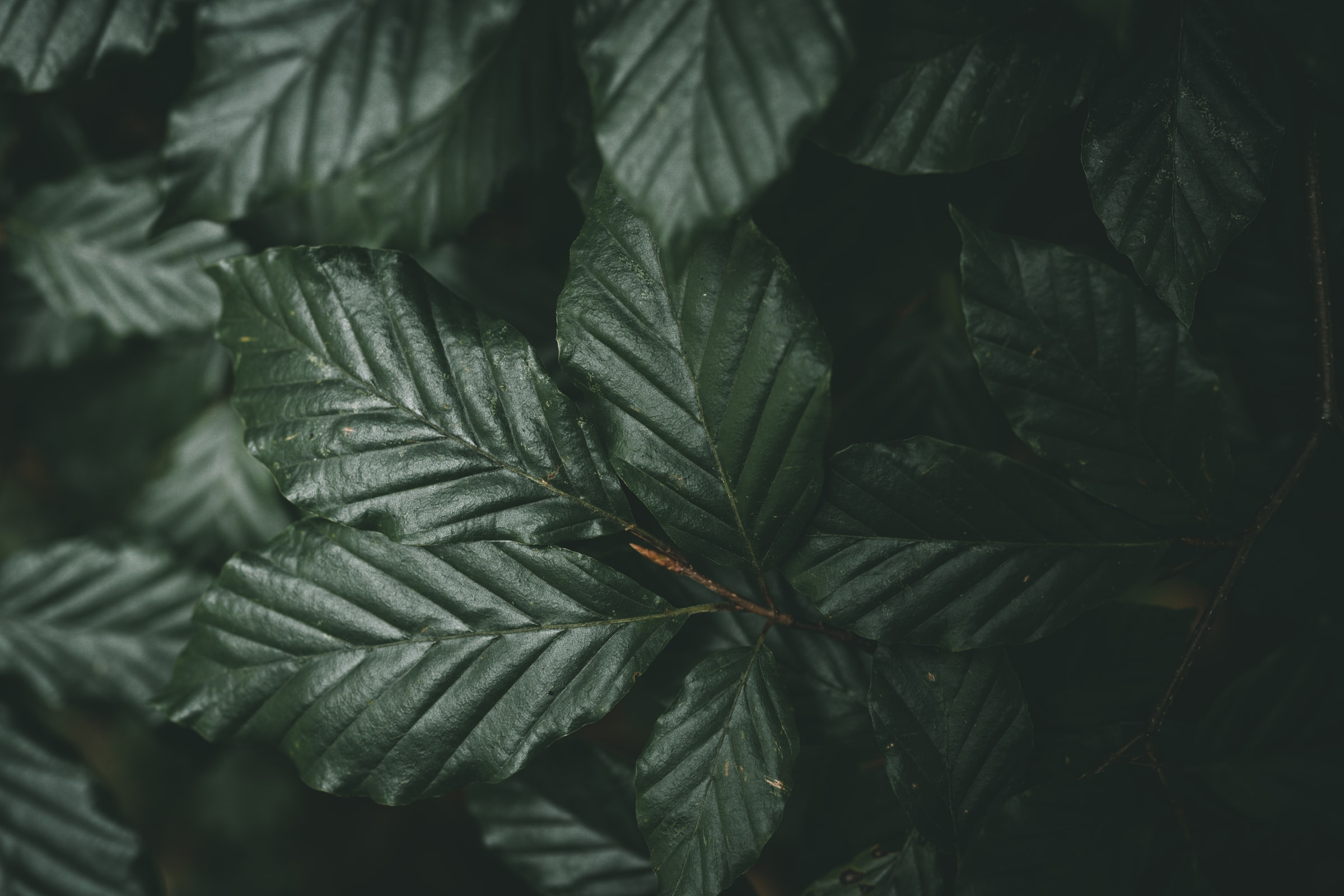
Making Backward-Compatible Schema Changes in MongoDB
How to add or remove a field from a Mongo collection without breaking the production?
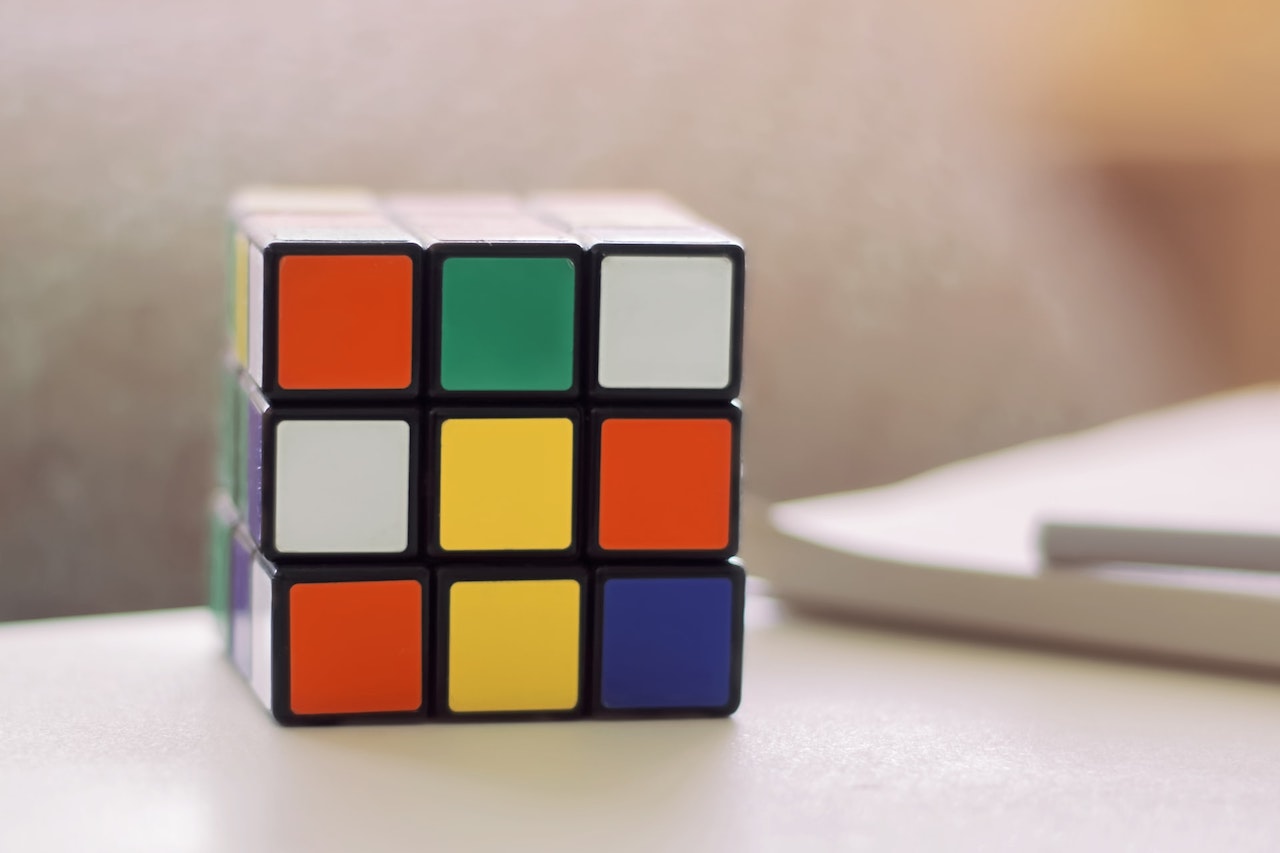
Writing Parameterized Tests in JUnit 5
Improving code quality using parameterized tests of JUnit 5! This article explains the motivation, the basic syntax, different annotations, and IDE-related actions about parameterized tests. Also, when you should or shouldn't use it and how to go further from here.
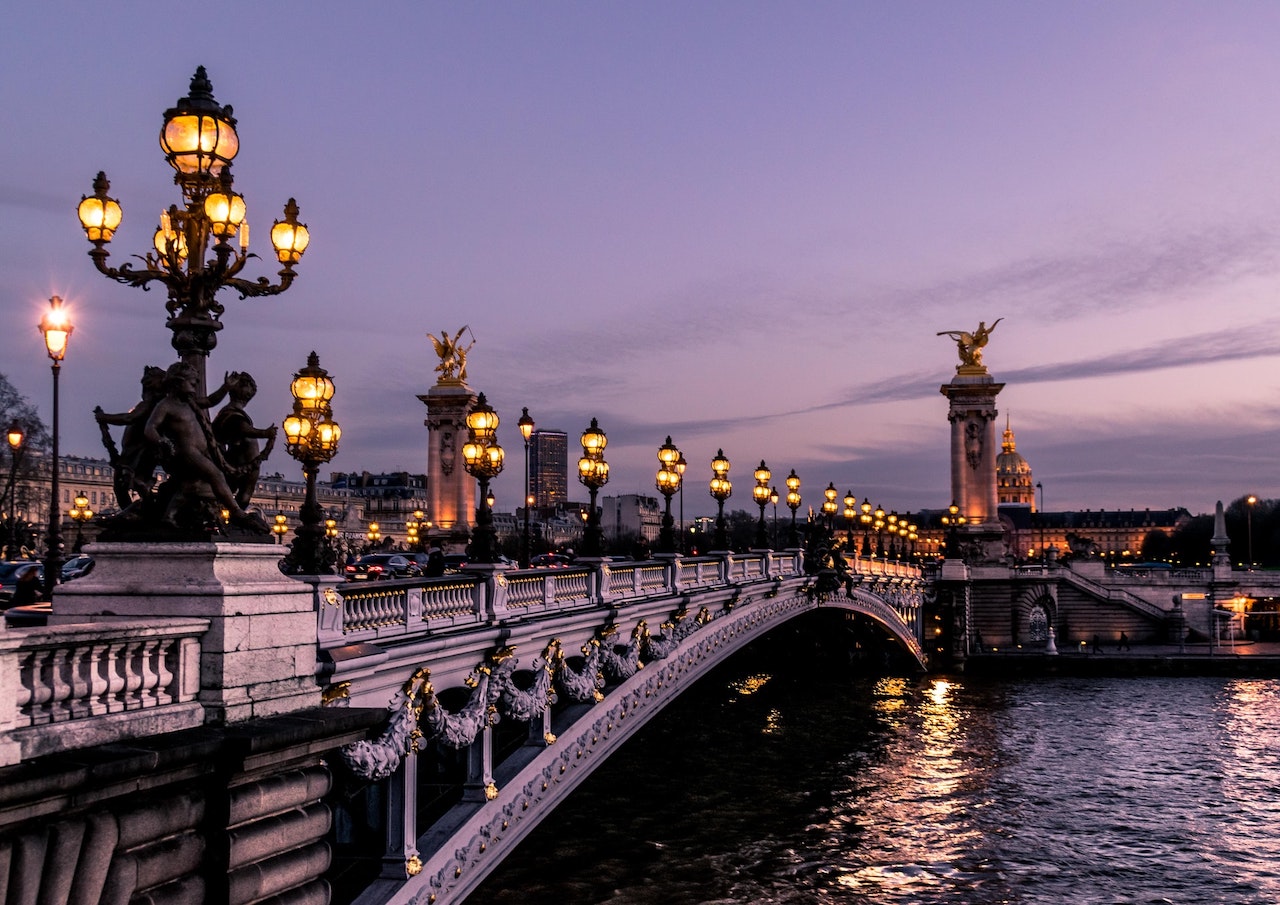
DVF: Snapshot And Restore
Part 4: How to create a snapshot for index "transactions" of DVF and restore it to an Elasticsearch cluster.
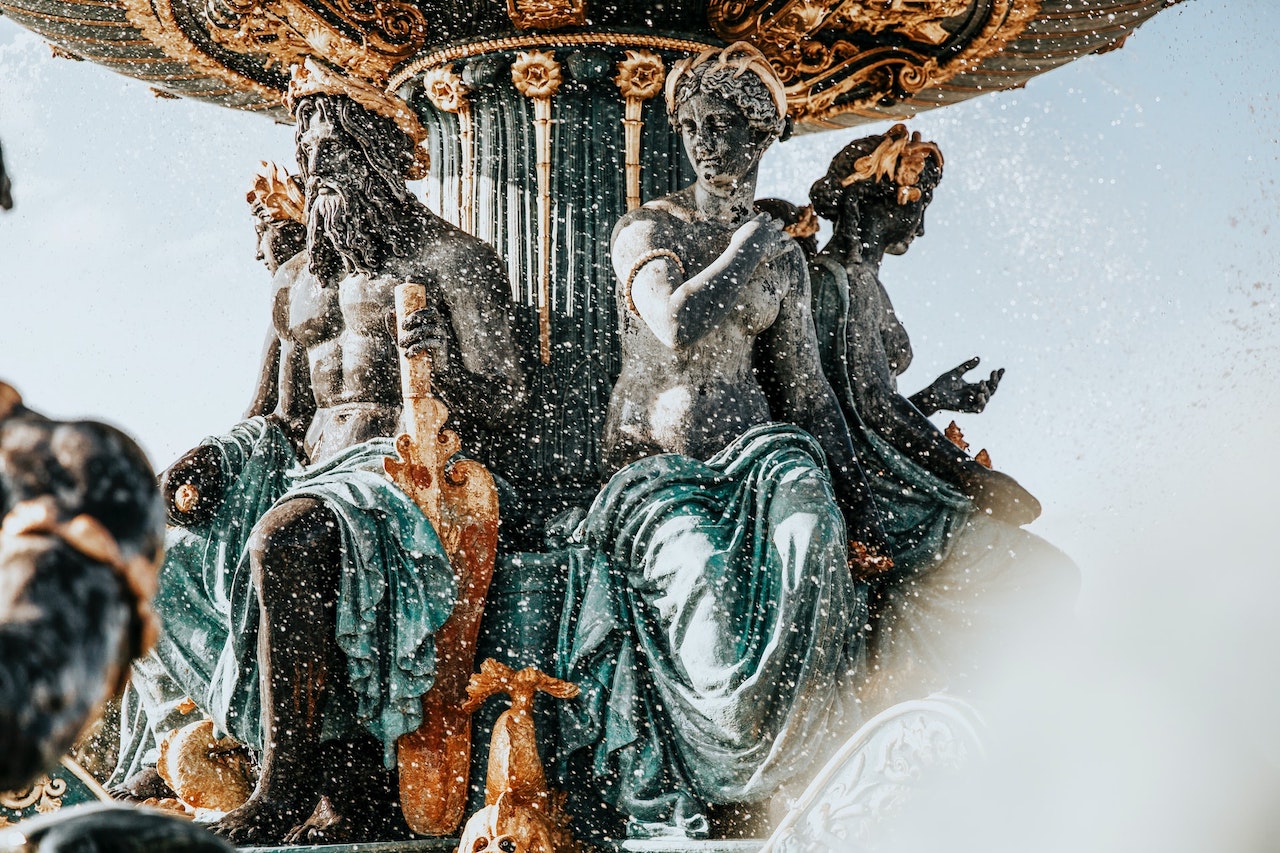
DVF: Storage Optimization
Part 3: How to optmize storage of a given index by 40% using force-merge.
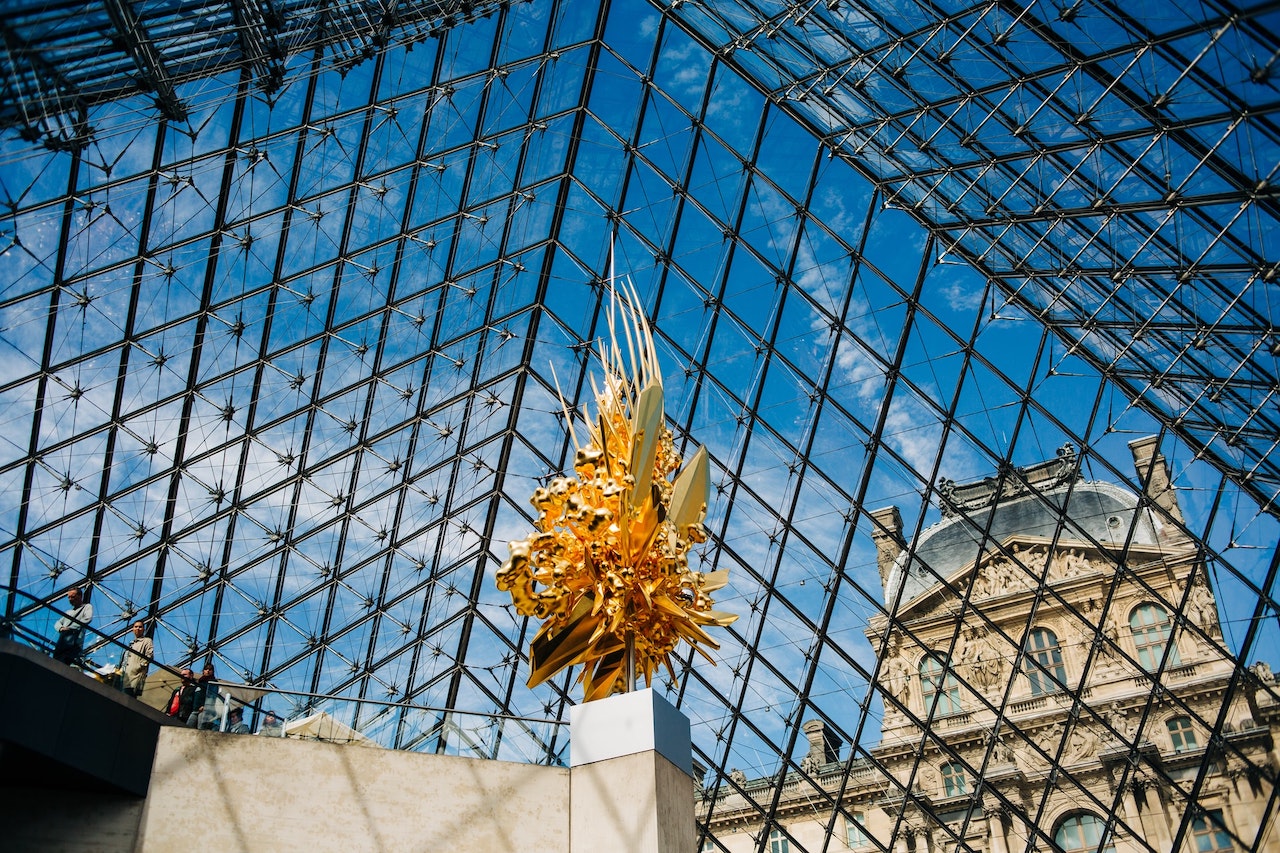
DVF: Indexing Optimization
Part 2: Optimize the indexing process using bulk index requests and multi-threading.
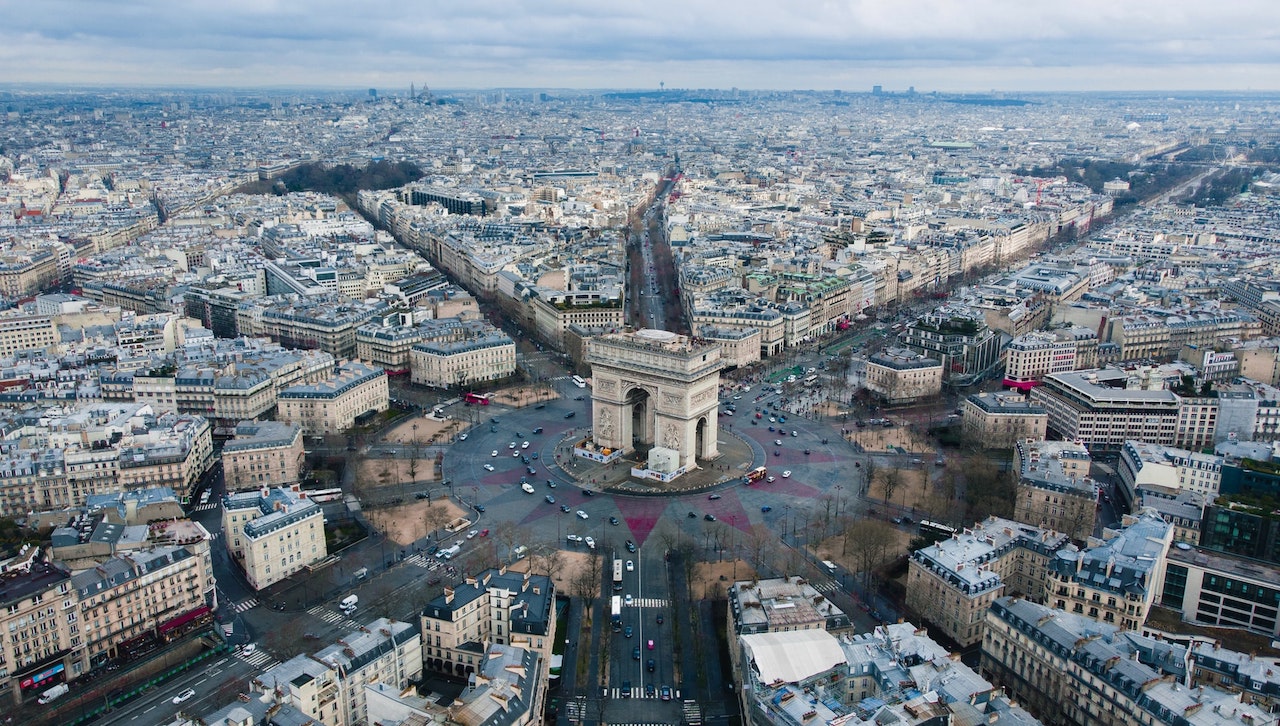
DVF: Indexing New Documents
Part 1: Indexing new documents into Elasticsearch using French government's open data "Demande de valeurs foncières (DVF)".
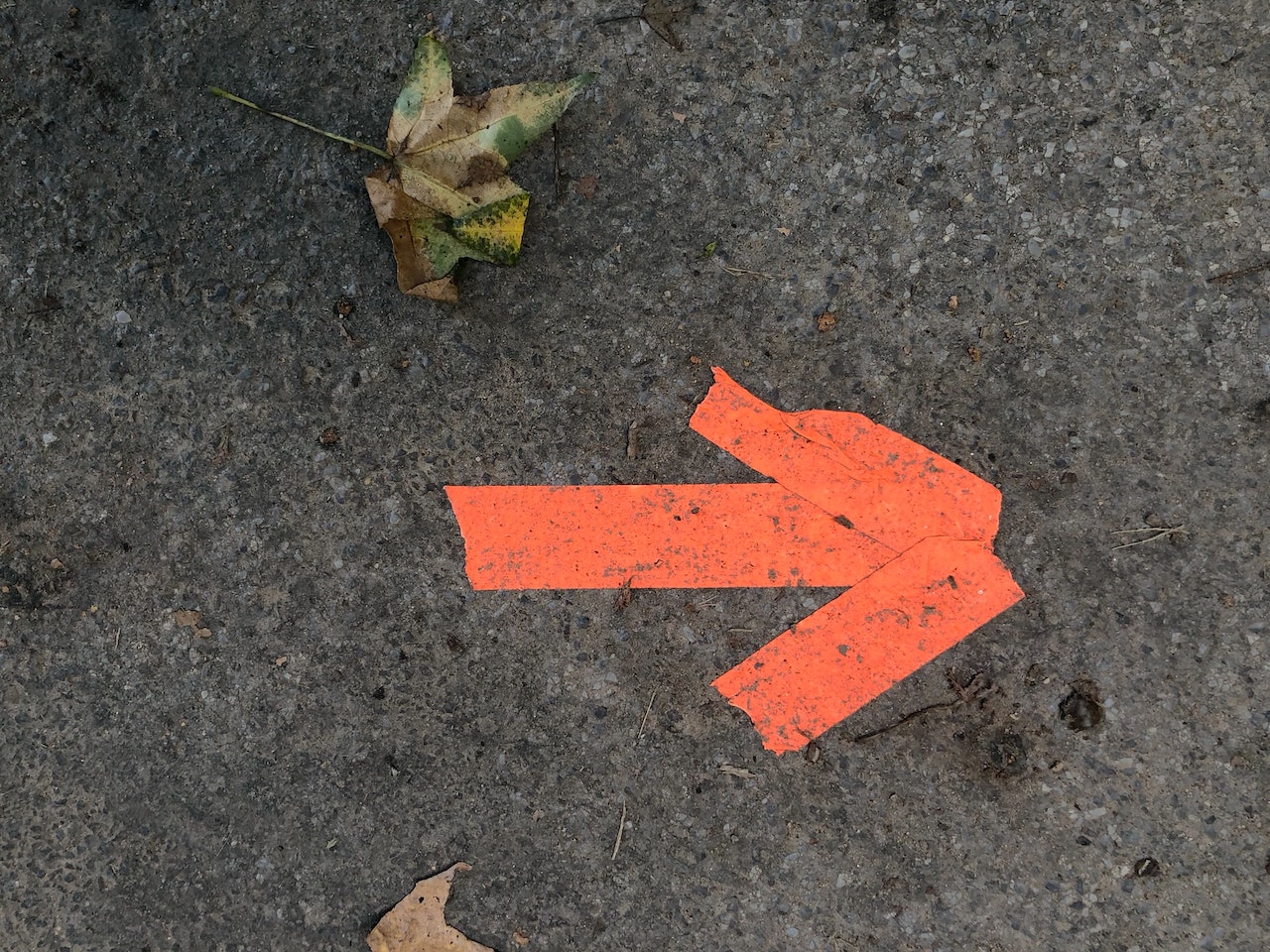
Feature Flag: Making Your Application More Reliable
How to make your web application more reliable with feature flags?
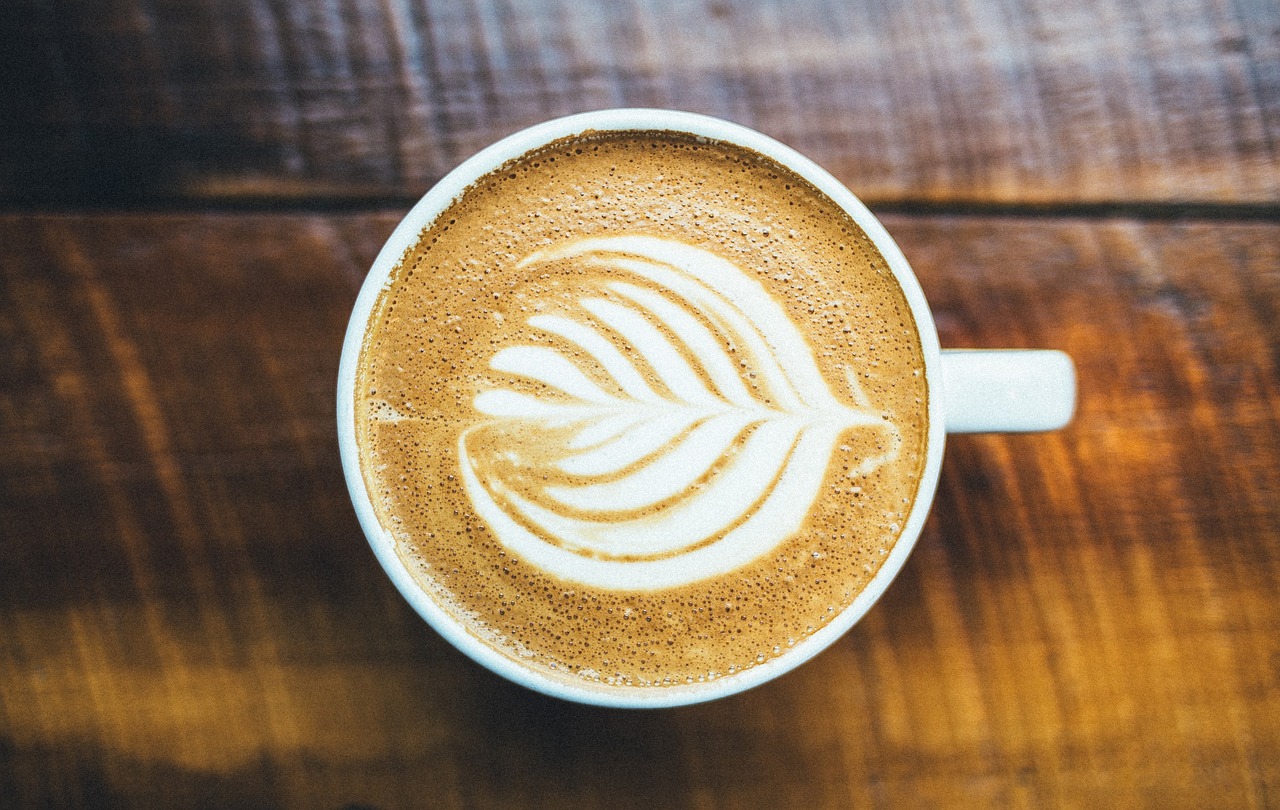
Introduction of Google Error-Prone
Introdution to Google Error-Prone in Maven, which augments the compiler's type analysis and catches more mistakes at compile time!
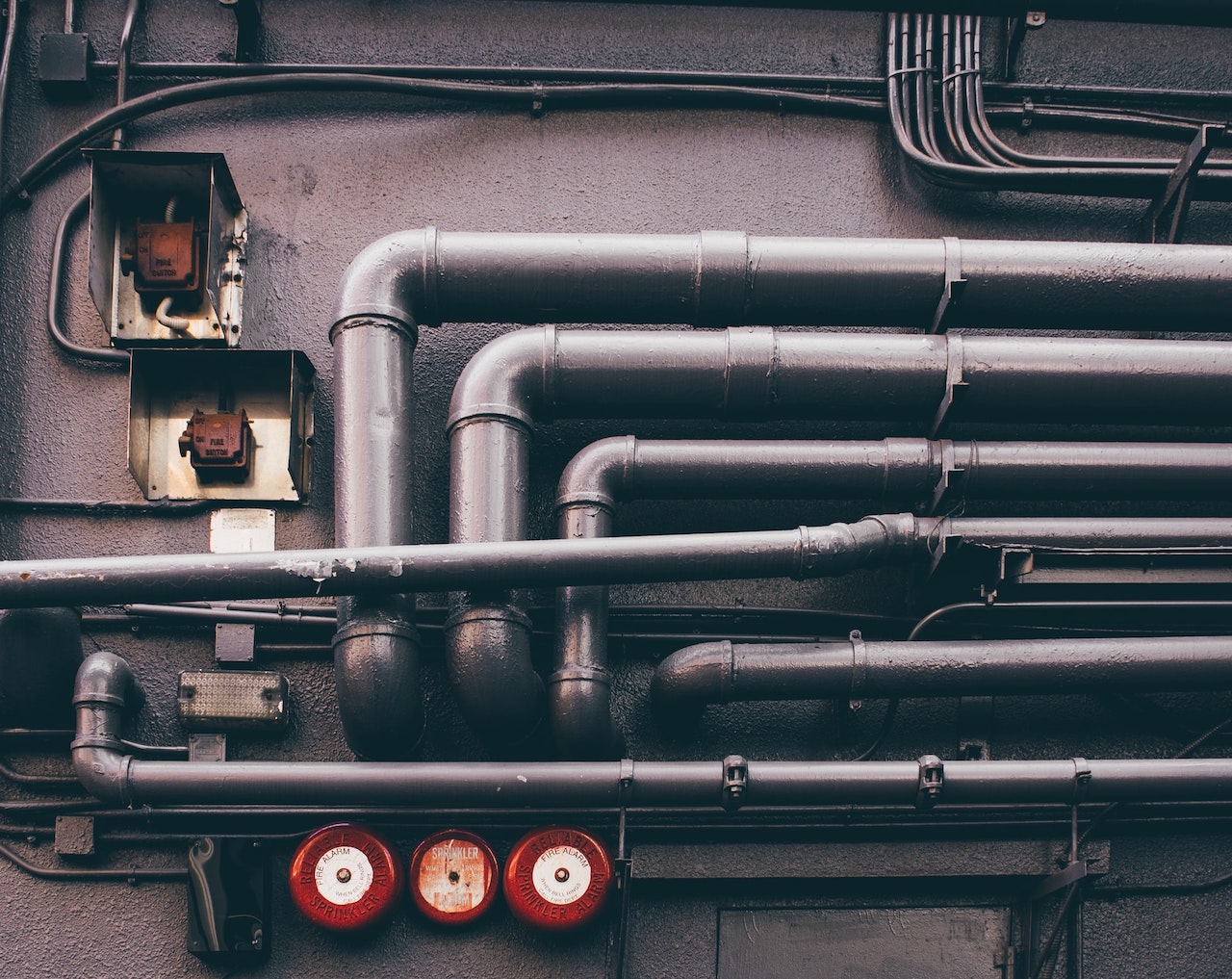
Create A Throttler In Java
What is throttling and how to write a simple throttler in Java.
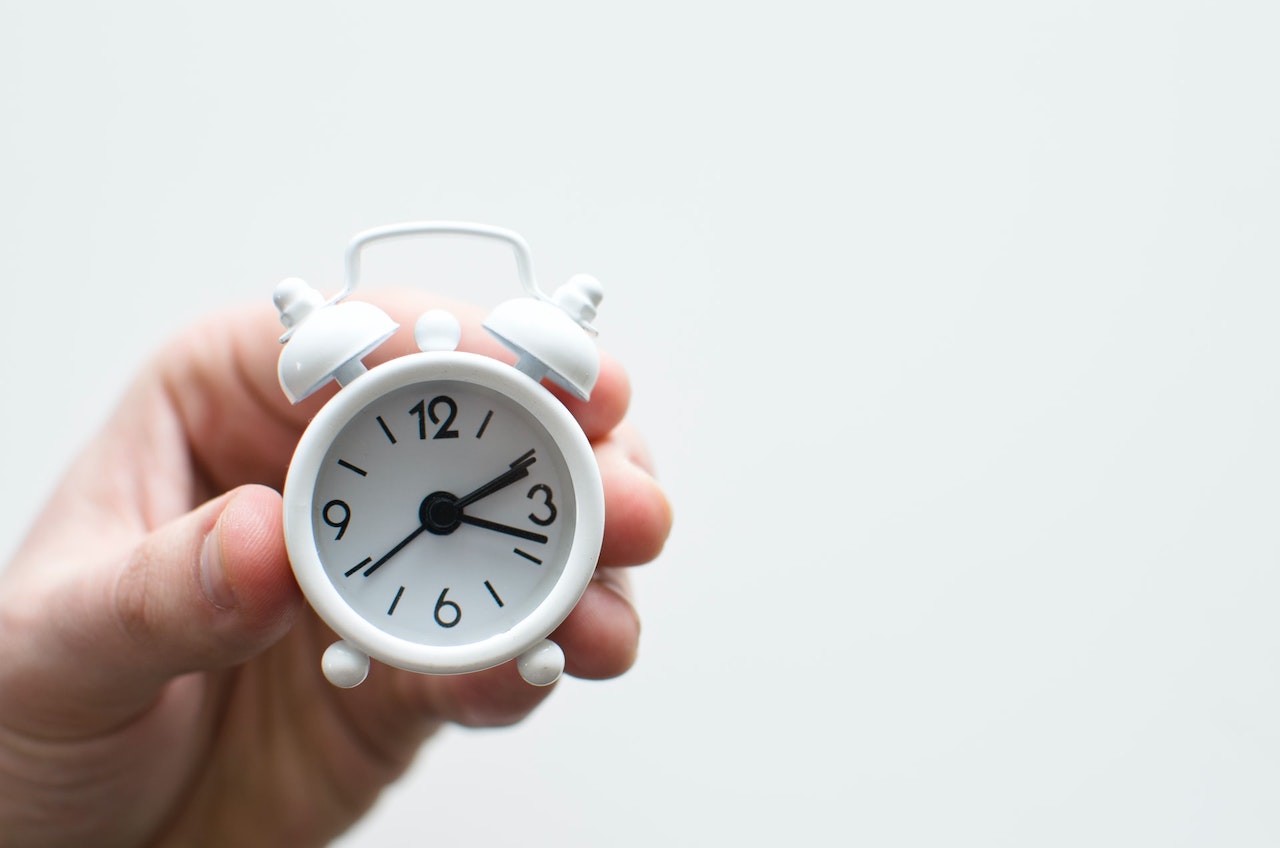
Using Java Time In Different Frameworks
How to use Java Time in different frameworks? This article shares examples in Java concurrency classes, Jackson, Akka, and Elasticsearch.
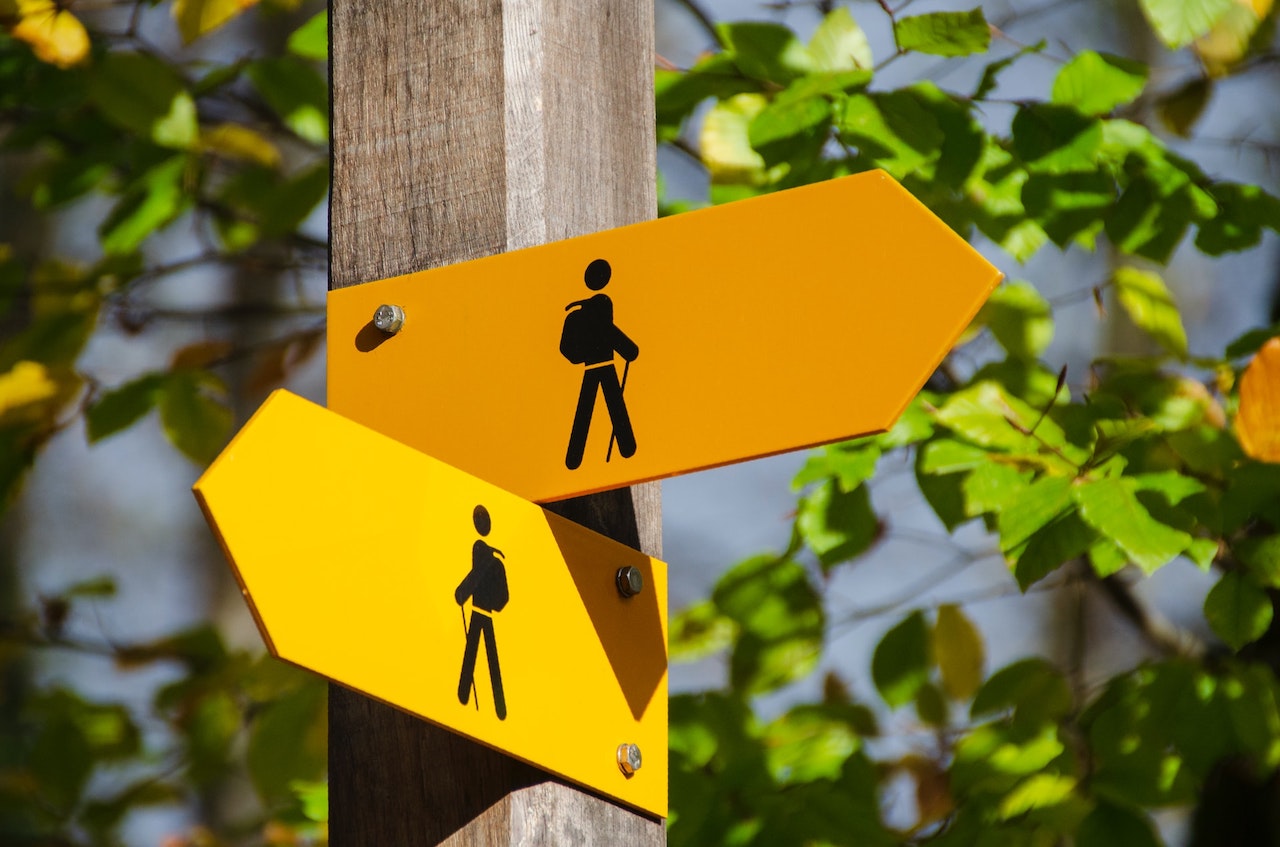
18 Allocation Deciders in Elasticsearch
This article explains the 18 allocation deciders in Elasticsearch: when they decide to allow, deny, or throttle the shard allocation under different circumstances. Also, a complete list of messages for unassigned shards.
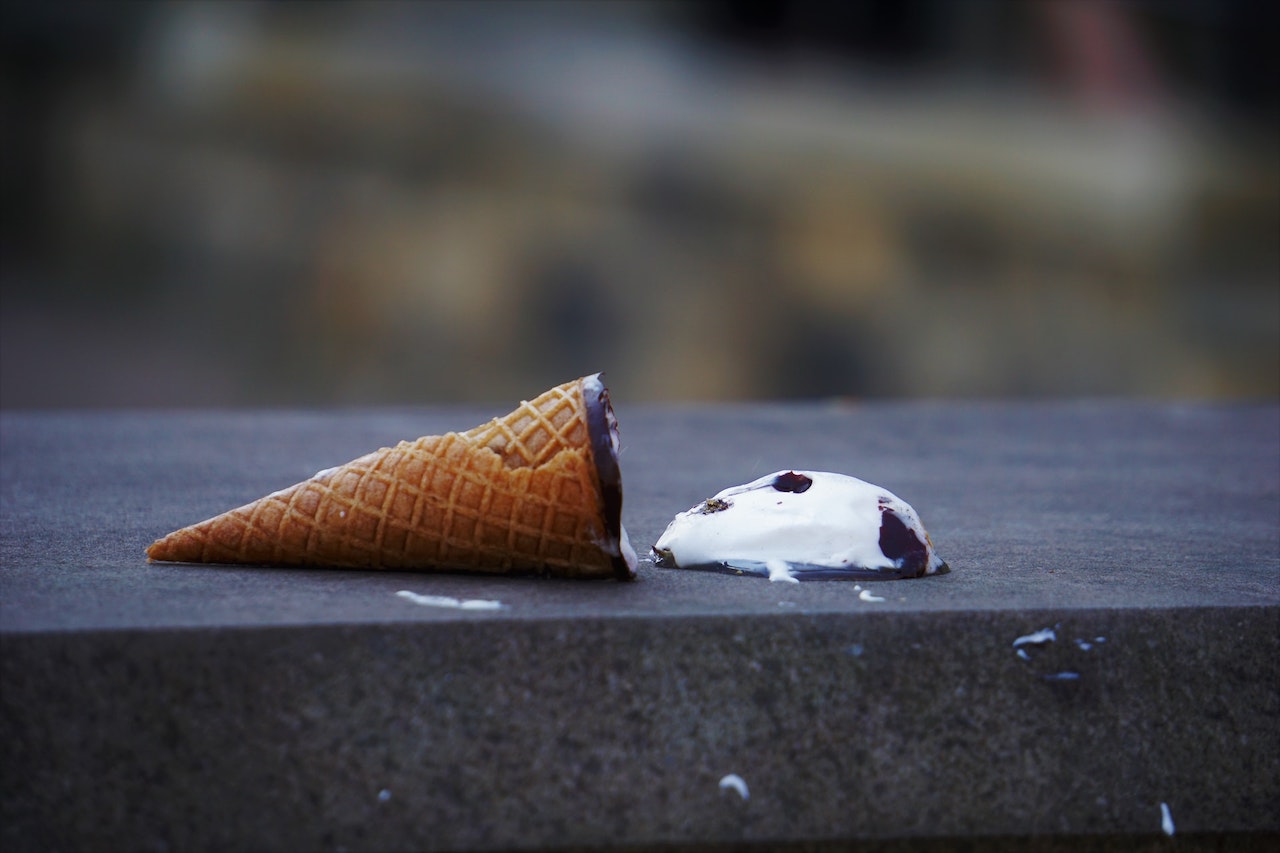
Elasticsearch: Common Index Exceptions
Explain the common Elasticsearch exceptions occurred when indexing new documents, with sample messages, analysis, suggestions and external resources for further investigation.
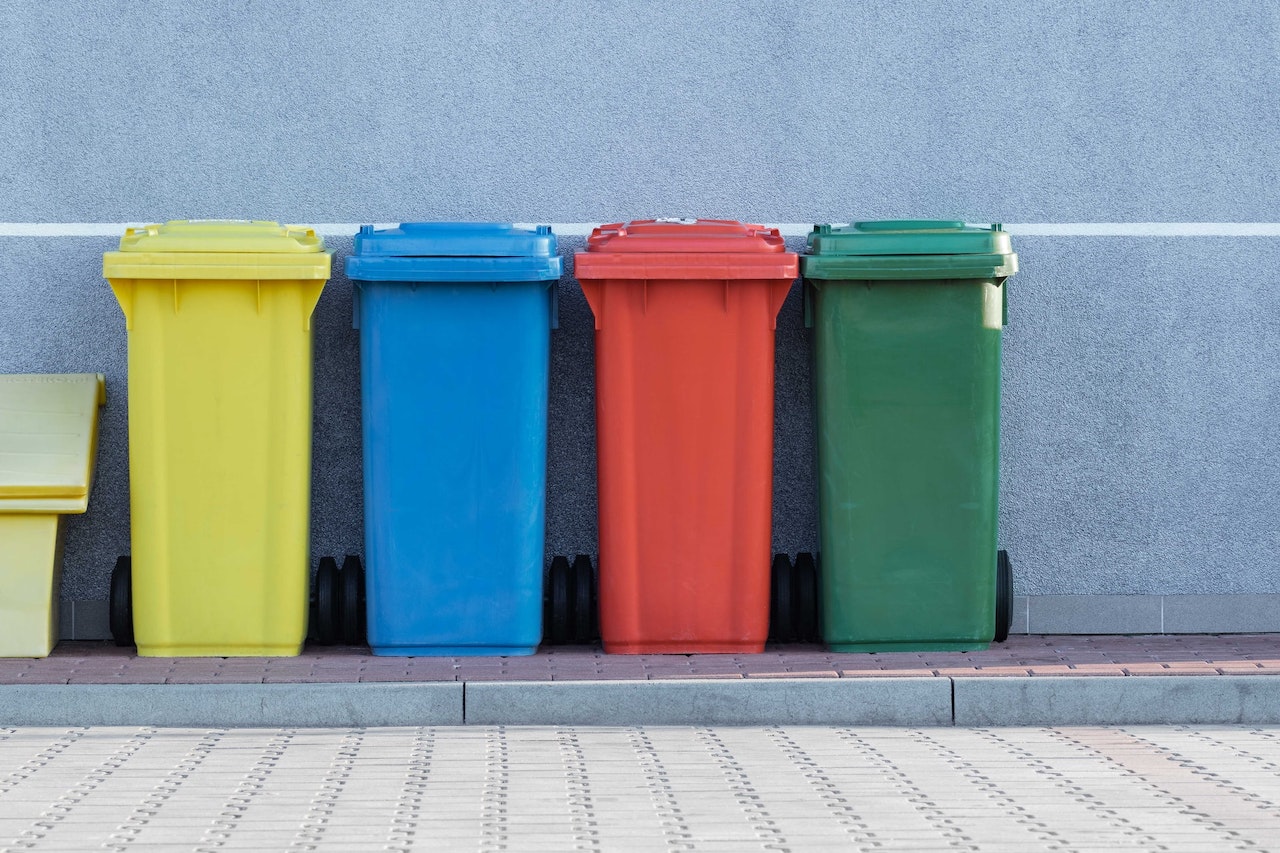
GC in Elasticsearch
Basic information about garbage collection (GC) in Elasticsearch, including the default garbage collector used, JVM options, GC logging, and more.
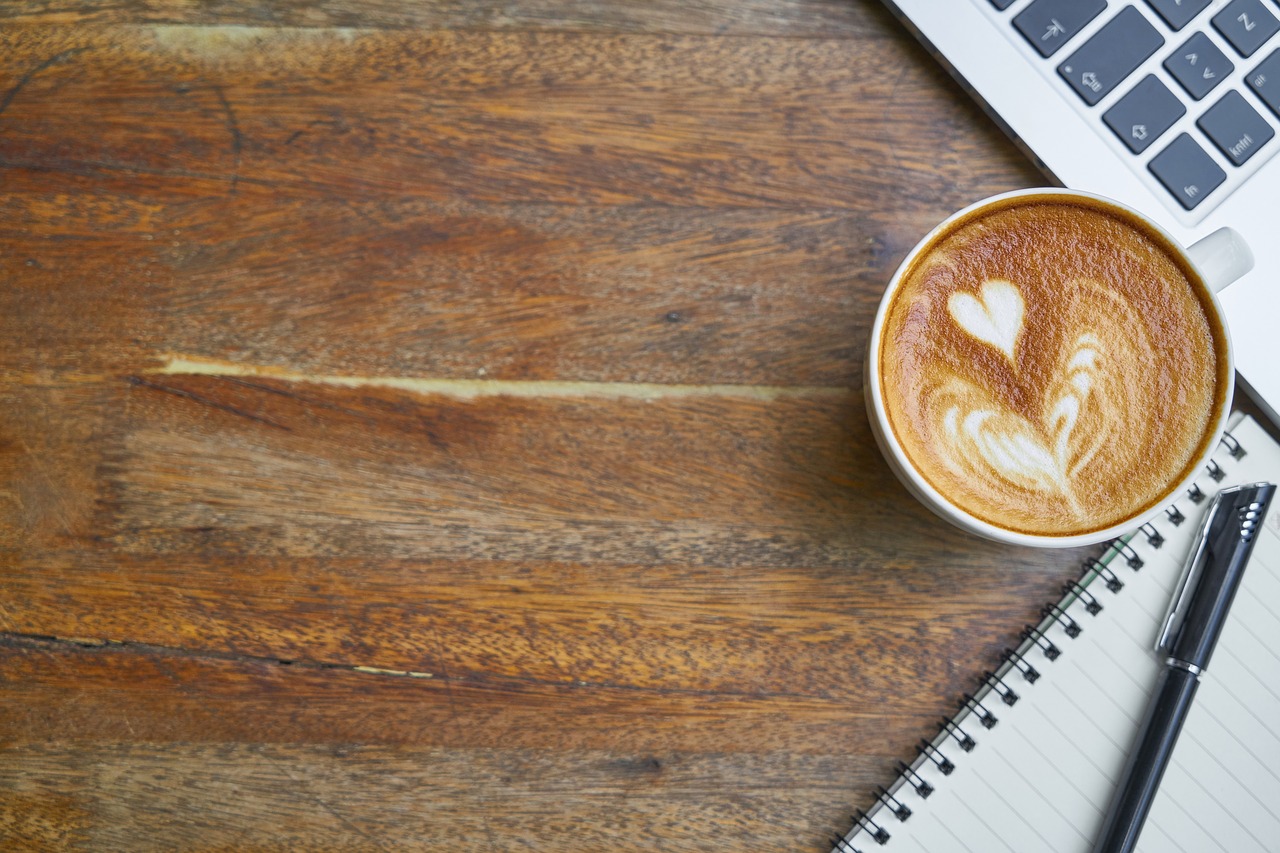
Wrap Elasticsearch Response Into CompletableFuture
Wrap Elasticsearch client response into CompletableFuture in Java for Elasticsearch transport client or Java high level REST client.
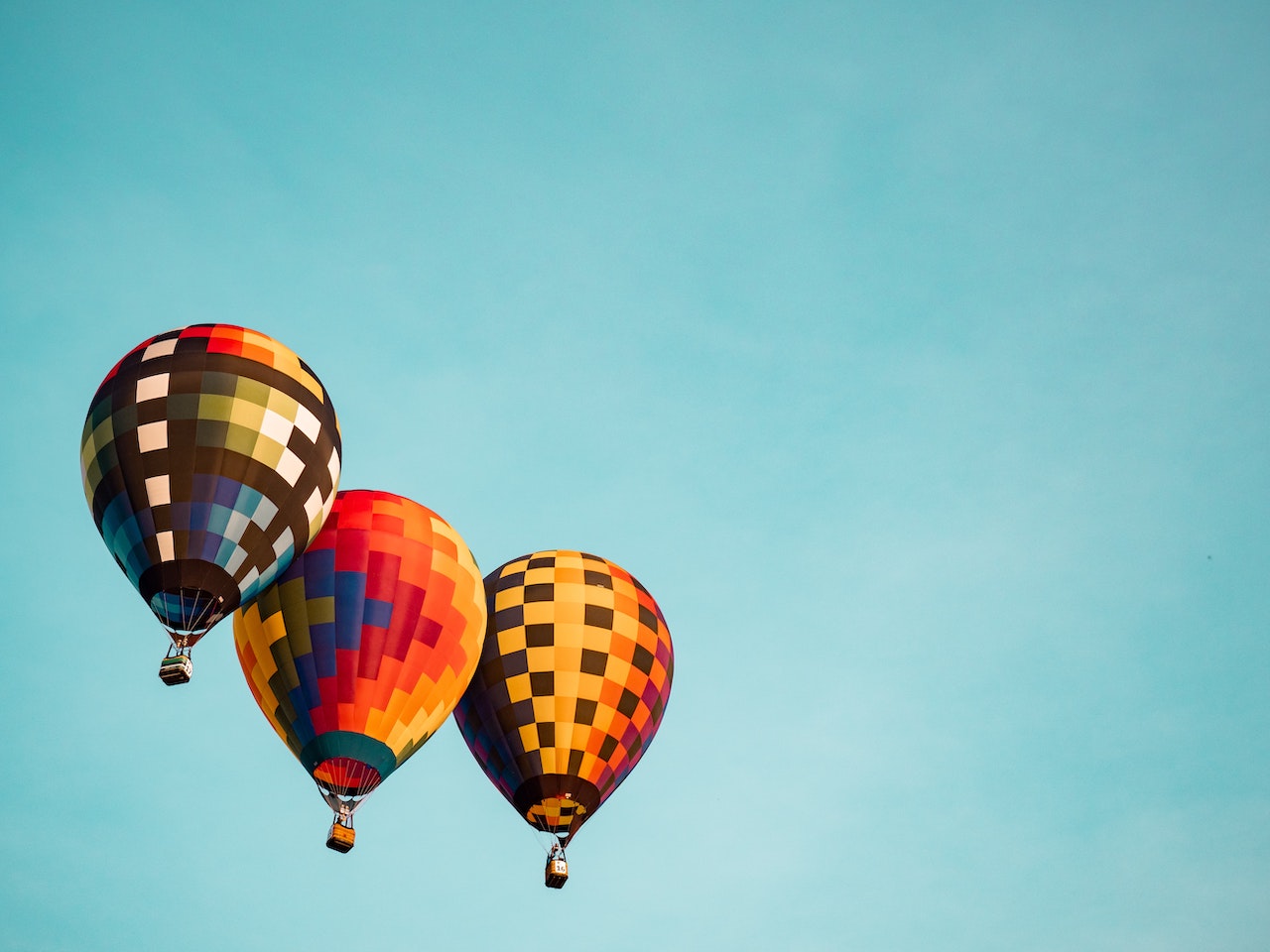
Vavr Jackson 1.0.0 Alpha 3
Release note of Vavr Jackson 1.0.0 Alpha 3.
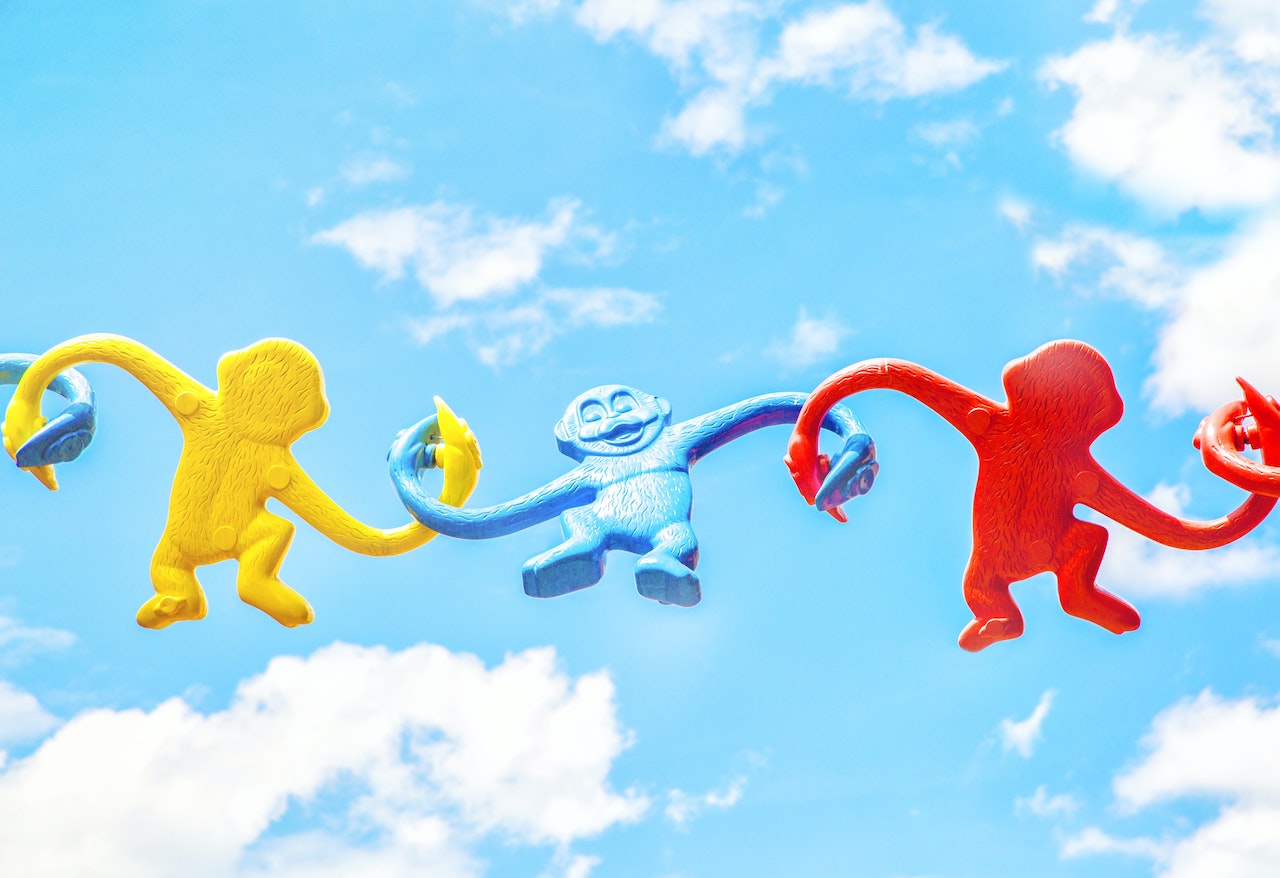
Why Do We Need Completable Future?
Why do we need CompletableFuture? What is its strength compared to synchronous code and classic future in Java? How to remember its APIs?
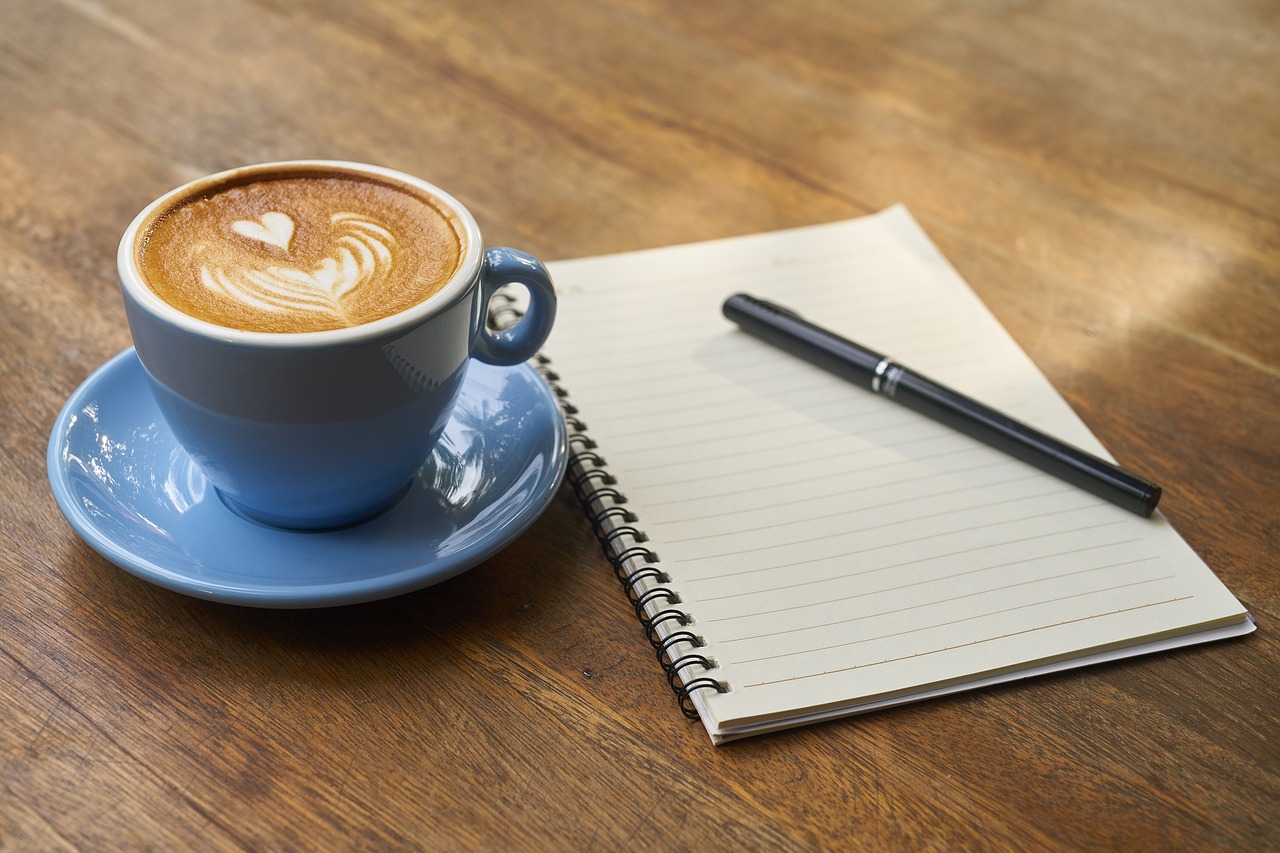
Write An Actor In Akka
How to write a new actor in Akka in Java? This post explains actor creation, message reception, and testing.
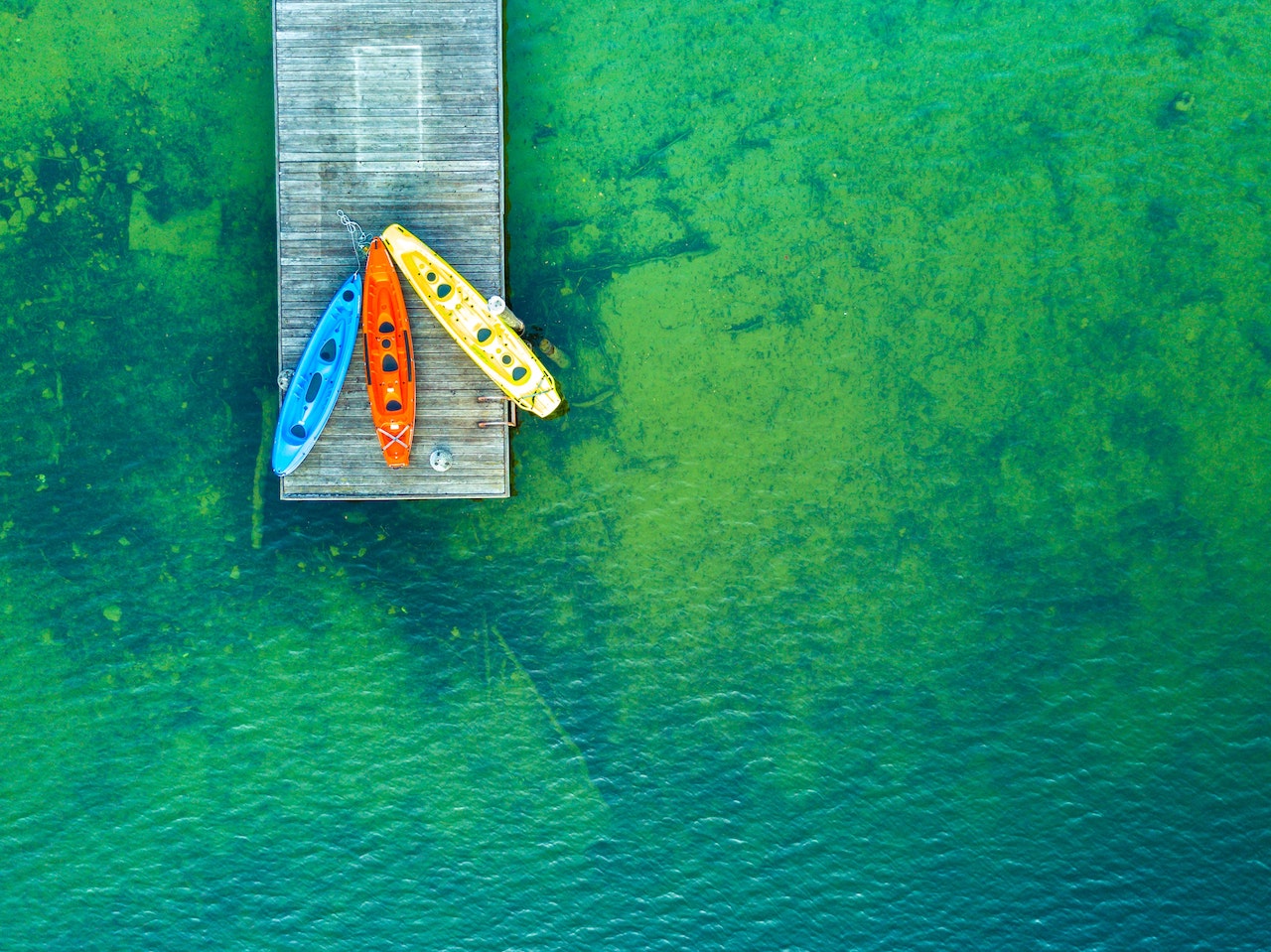
3 Ways to Handle Exception In Completable Future
How to handle exception in CompletableFuture? This article explains the difference between handle(), whenComplete(), and exceptionally().
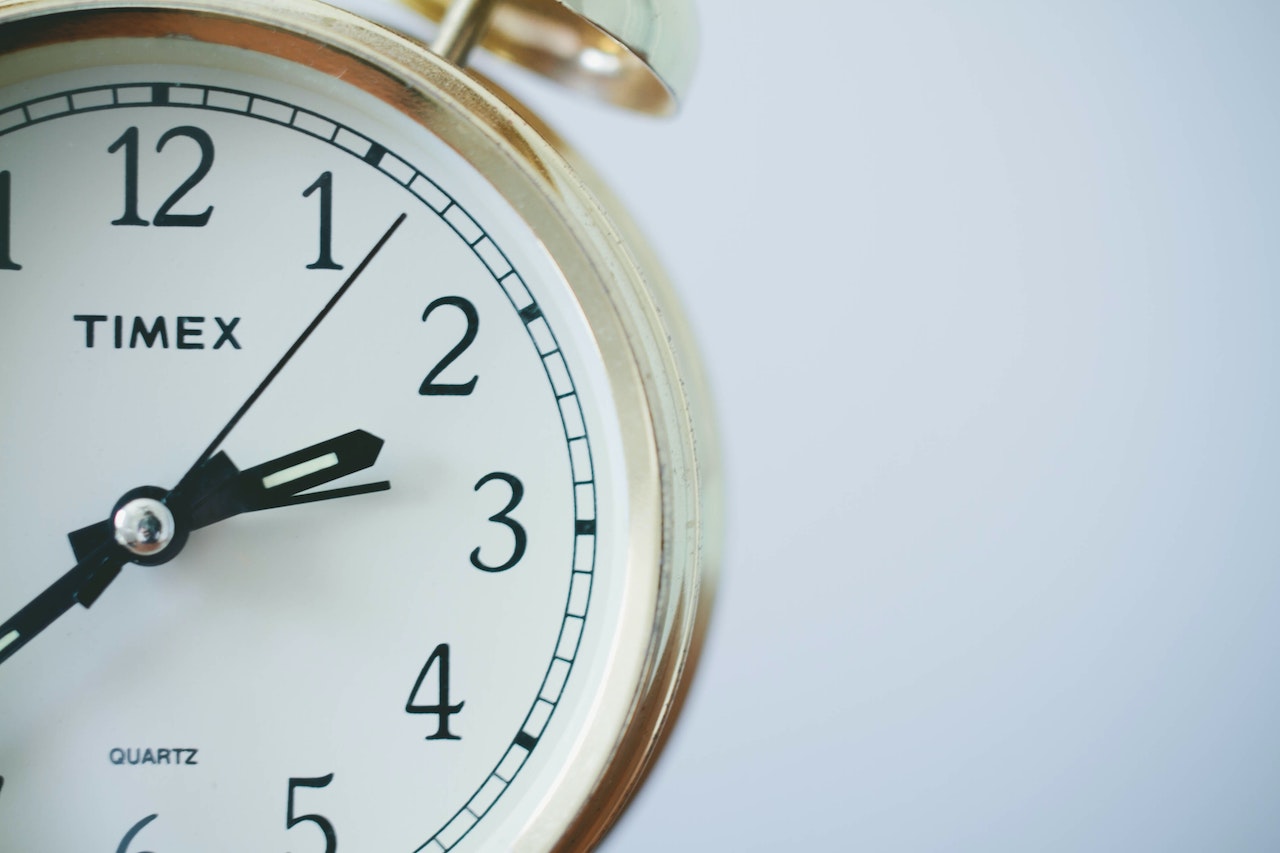
Controlling Time with Java Clock
Use java.time.Clock to control time in your unit tests. This article will mainly focus on usage of fixed clock and offset clock.
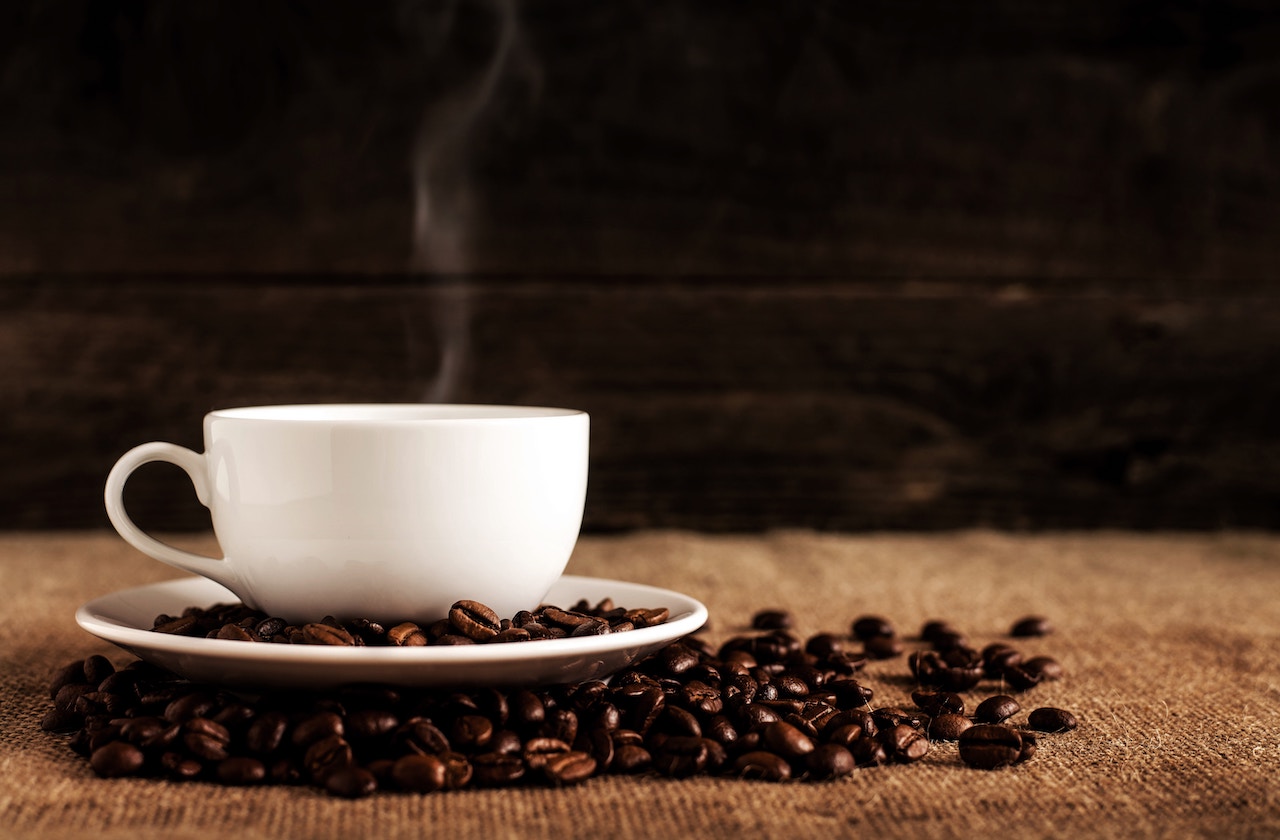
How CompletableFuture is tested in OpenJDK?
How CompletableFuture is tested in OpenJDK 14? What can we learn from it?
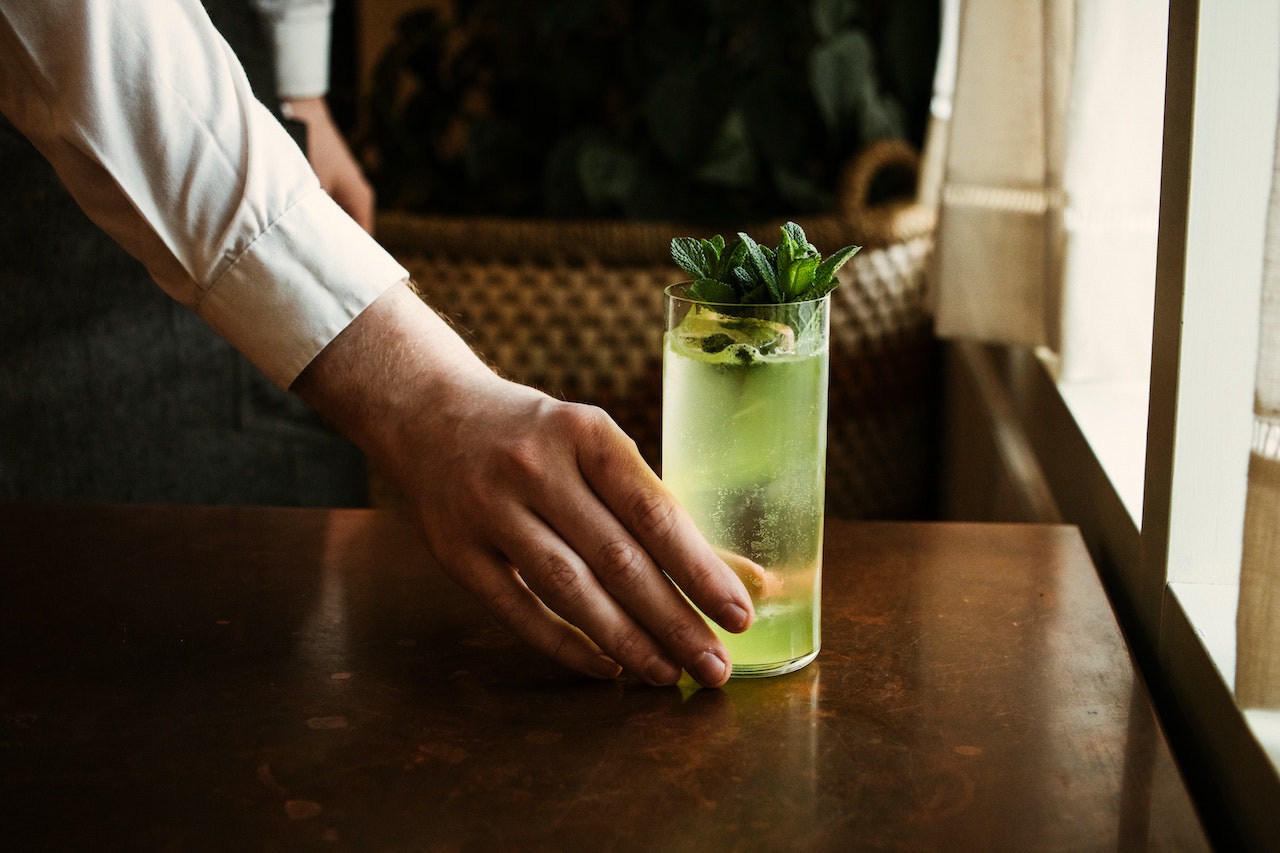
Mockito: 3 Ways to Init Mock in JUnit 5
Initialize Mockito mock objects in JUnit 5 using MockitoExtension, MockitoAnnotations#initMocks, or Mockito#mock.
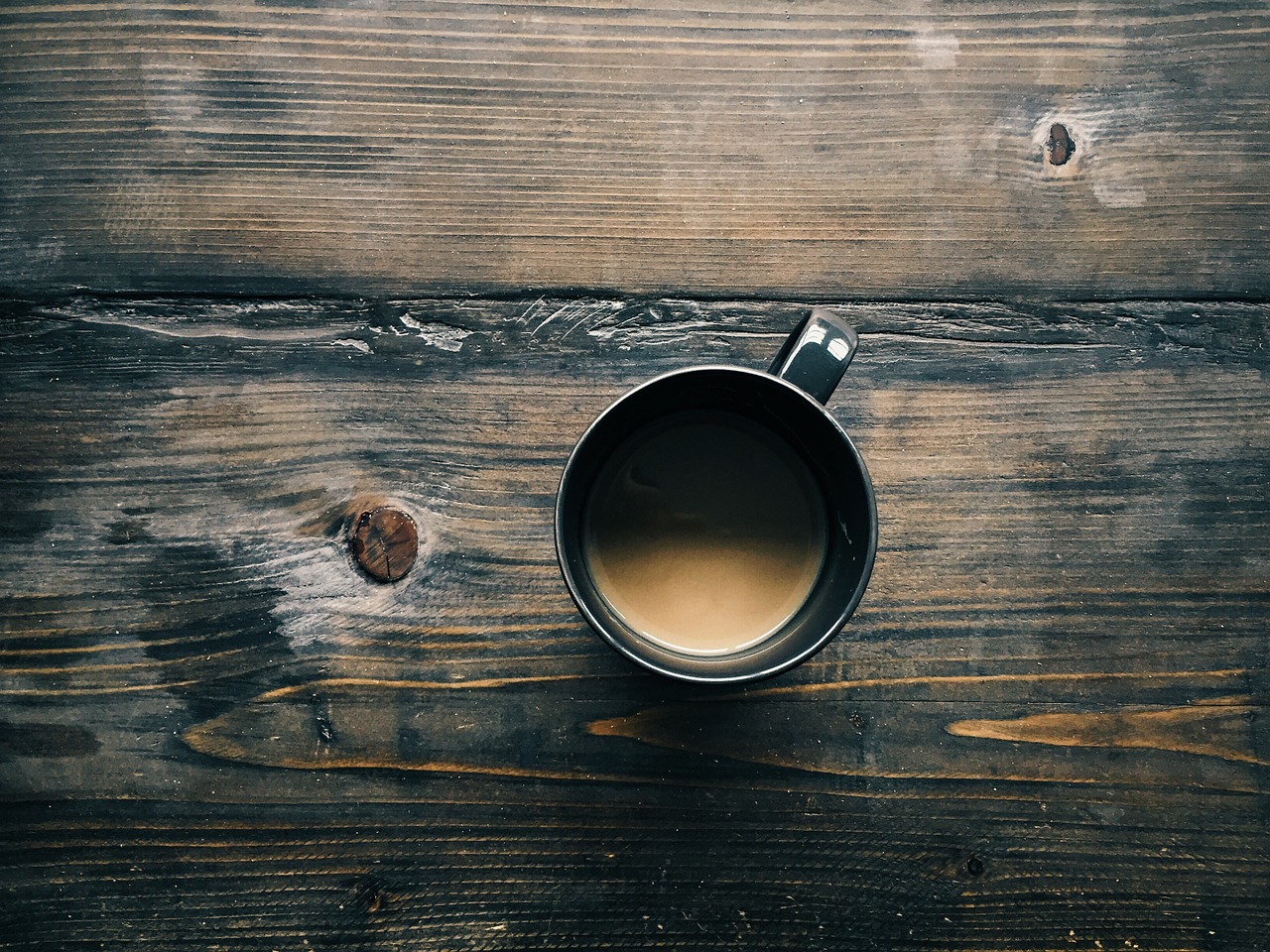
Introduction of Immutables
Introduction of Java framework Immutables which creates immutable objects and builders for you. Type-safe, null-safe and thread-safe, with no boilerplate.
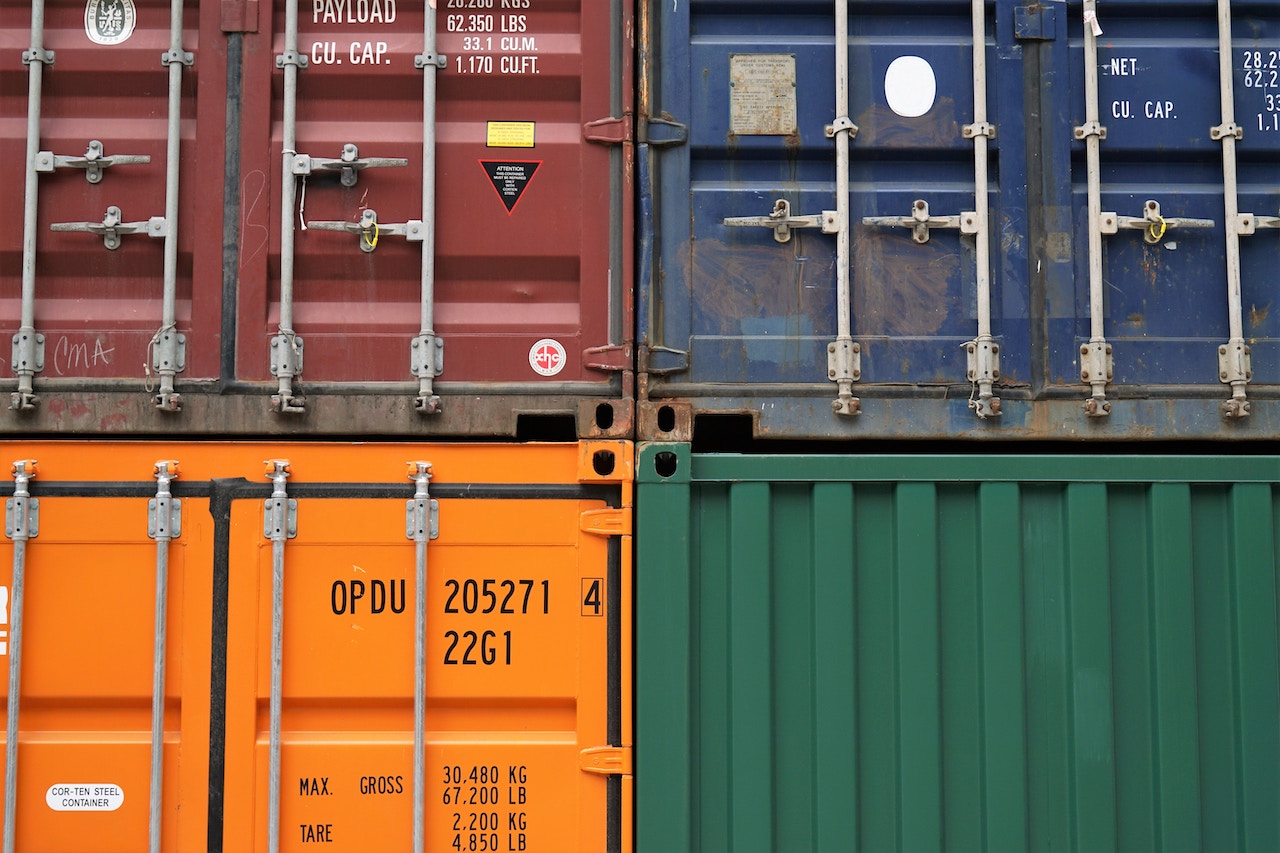
Testing Elasticsearch With Docker And Java High Level REST Client
Testing Elasticsearch with docker and Java High Level REST Client
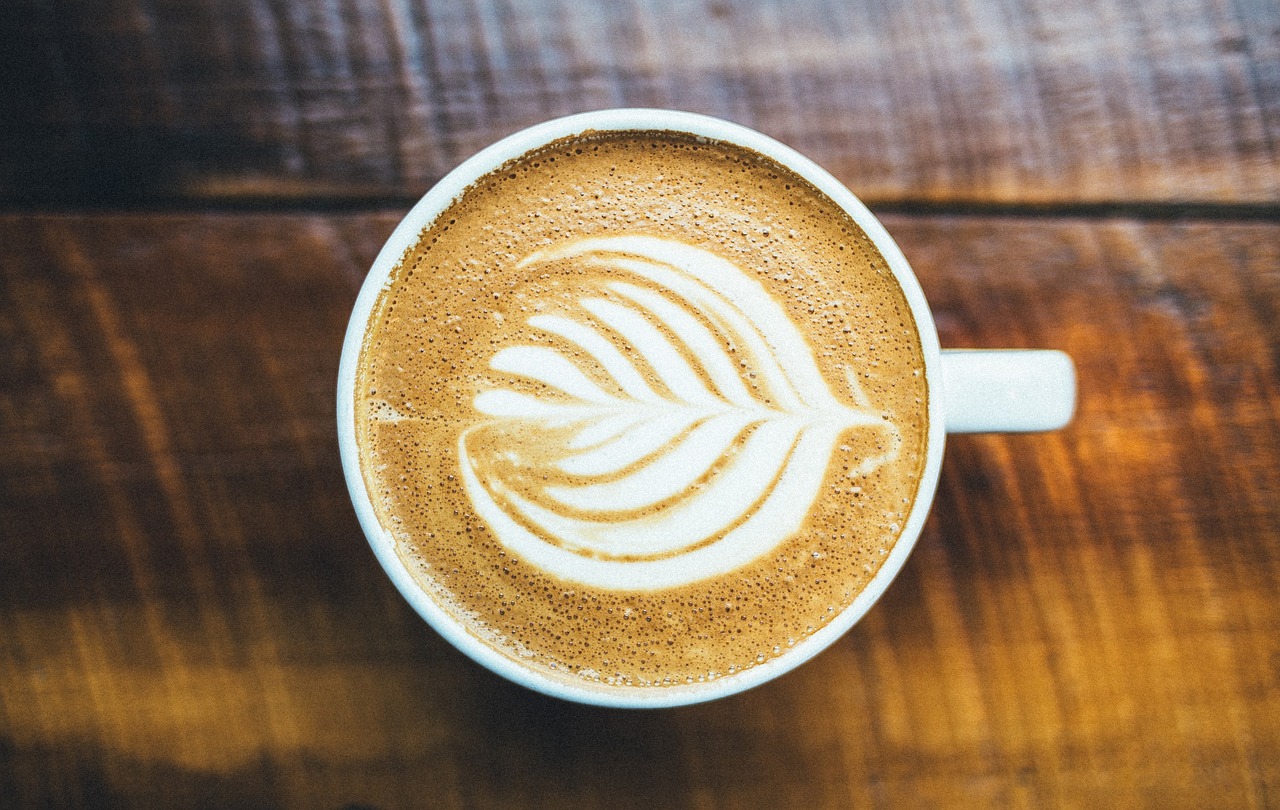
Asynchronous Processing in JAX-RS 2.x
Quick introduction of asynchrnous processing in JAX-RS 2.x on both server-side and client-side.
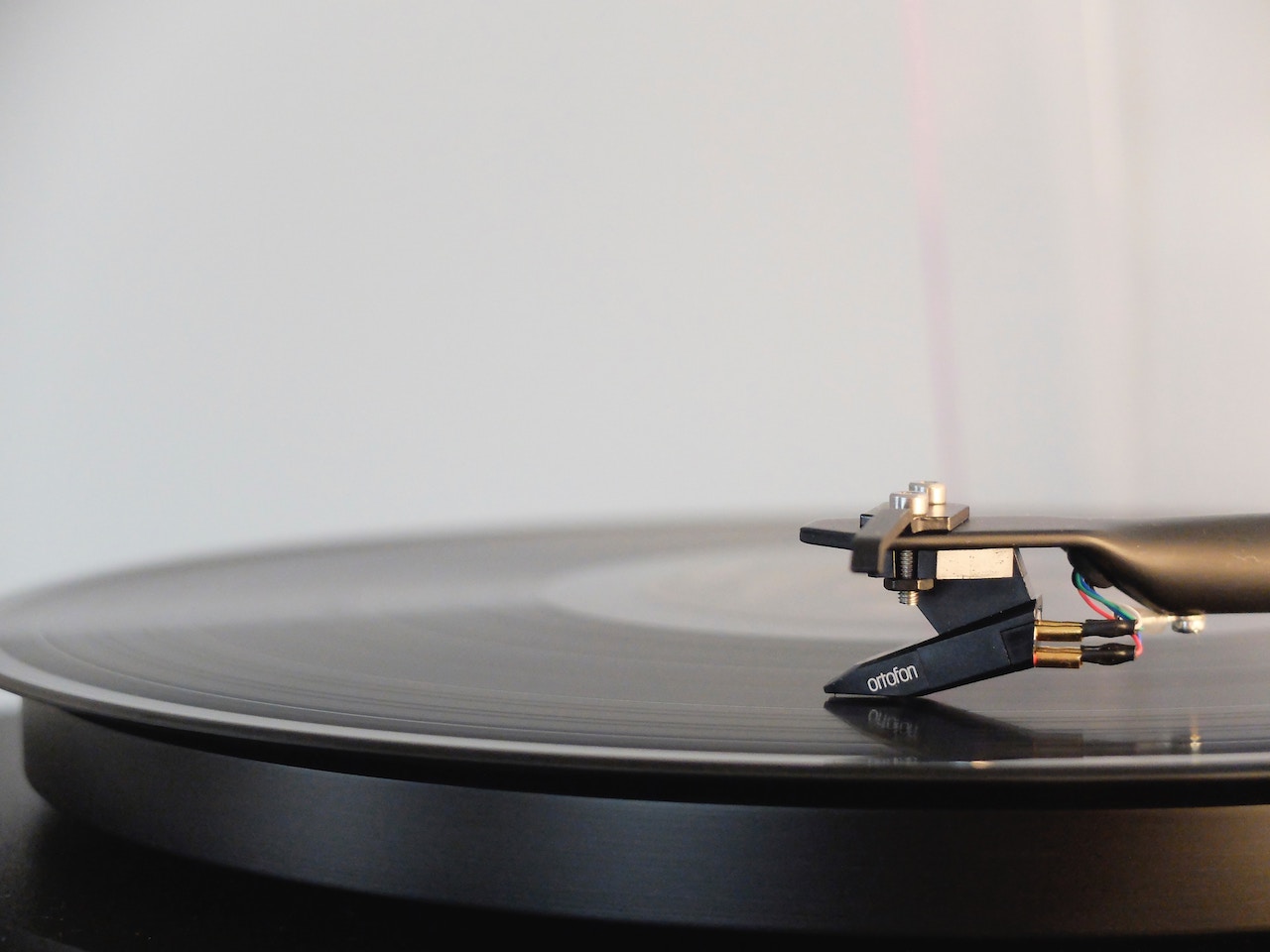
Logback: Test Logging Event
Capture SLF4J + Logback logging events and test them in unit tests using ListAppender.
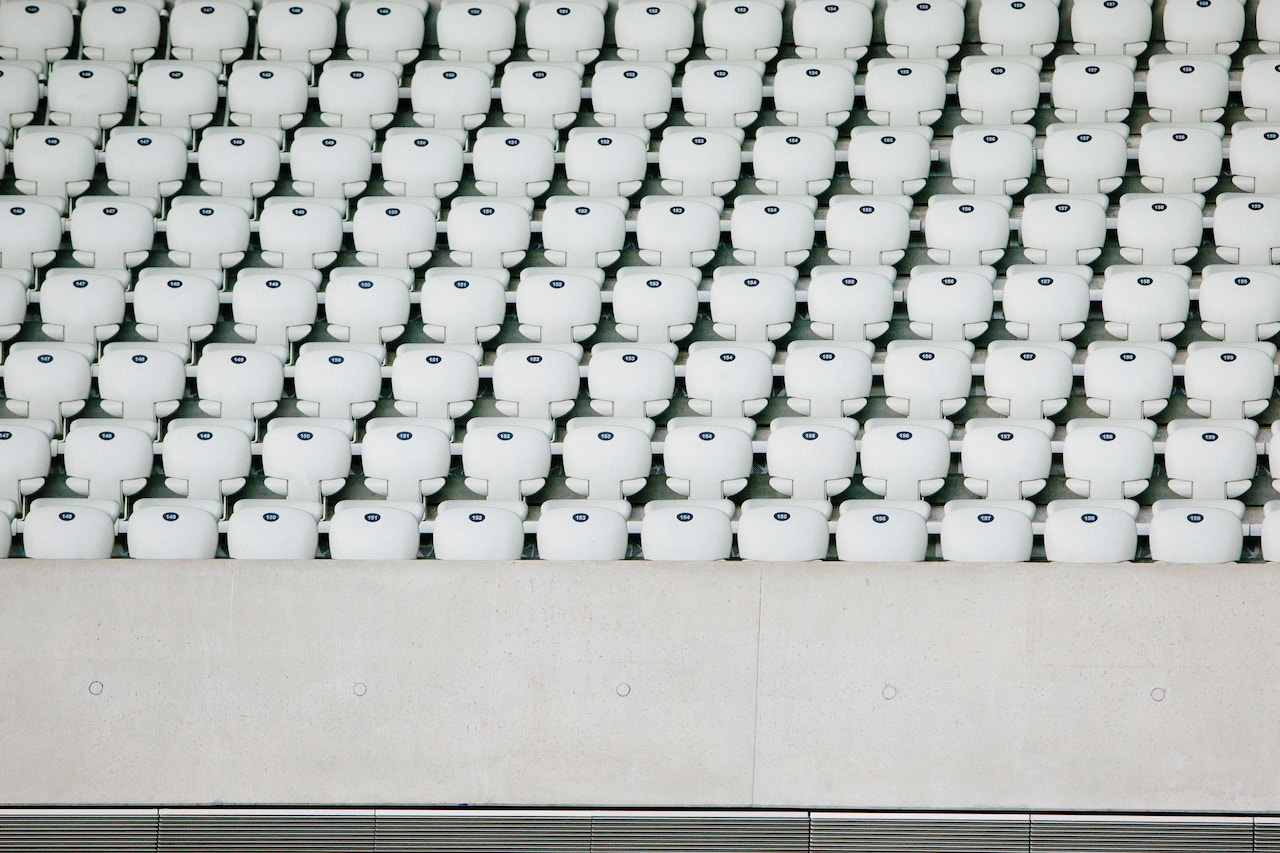
Elasticsearch: Scroll API in Java
Elasticsearch Scroll API sample written in Java, useful for retrieving large numbers of results (or even all results) from a single search request.
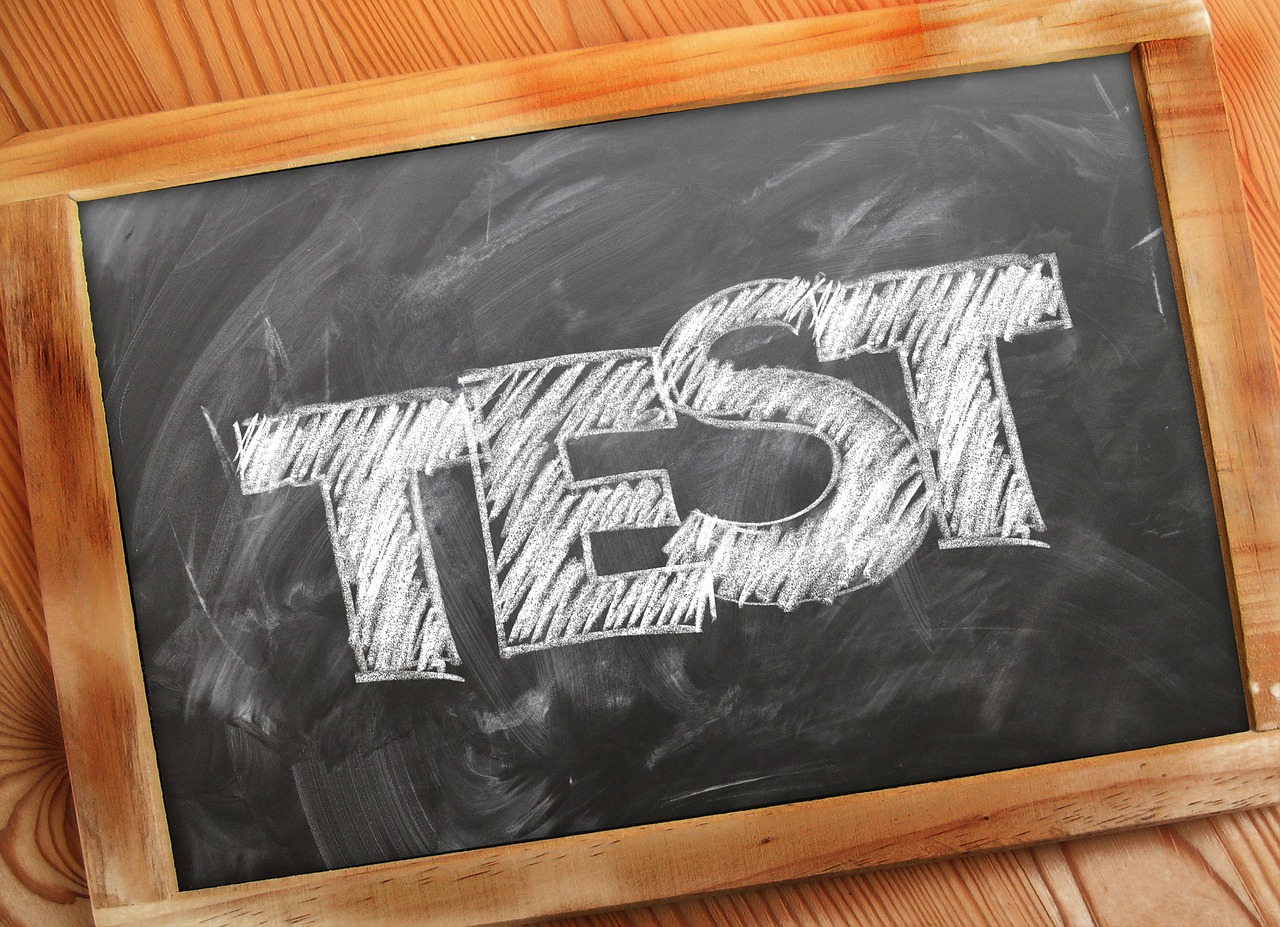
Akka: Testing Actor with TestActorRef
Unit testing Akka actor with "TestActorRef", which gives you access to underlying actor instance and runs the logic synchronously in a single-threaded environment.
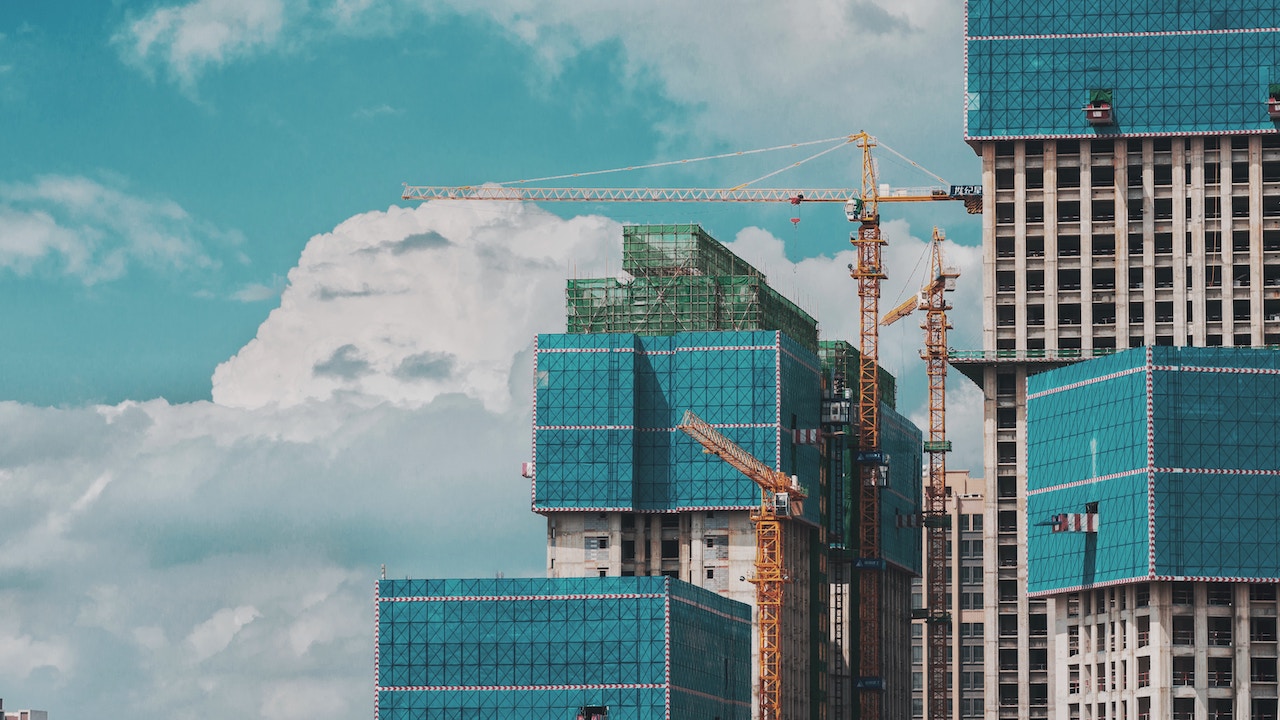
Build Helper Maven Plugin
How to set up additional source directory (src/it/java) and resource directory (src/it/resources) for integration tests in Maven project using Build Helper Maven Plugin.
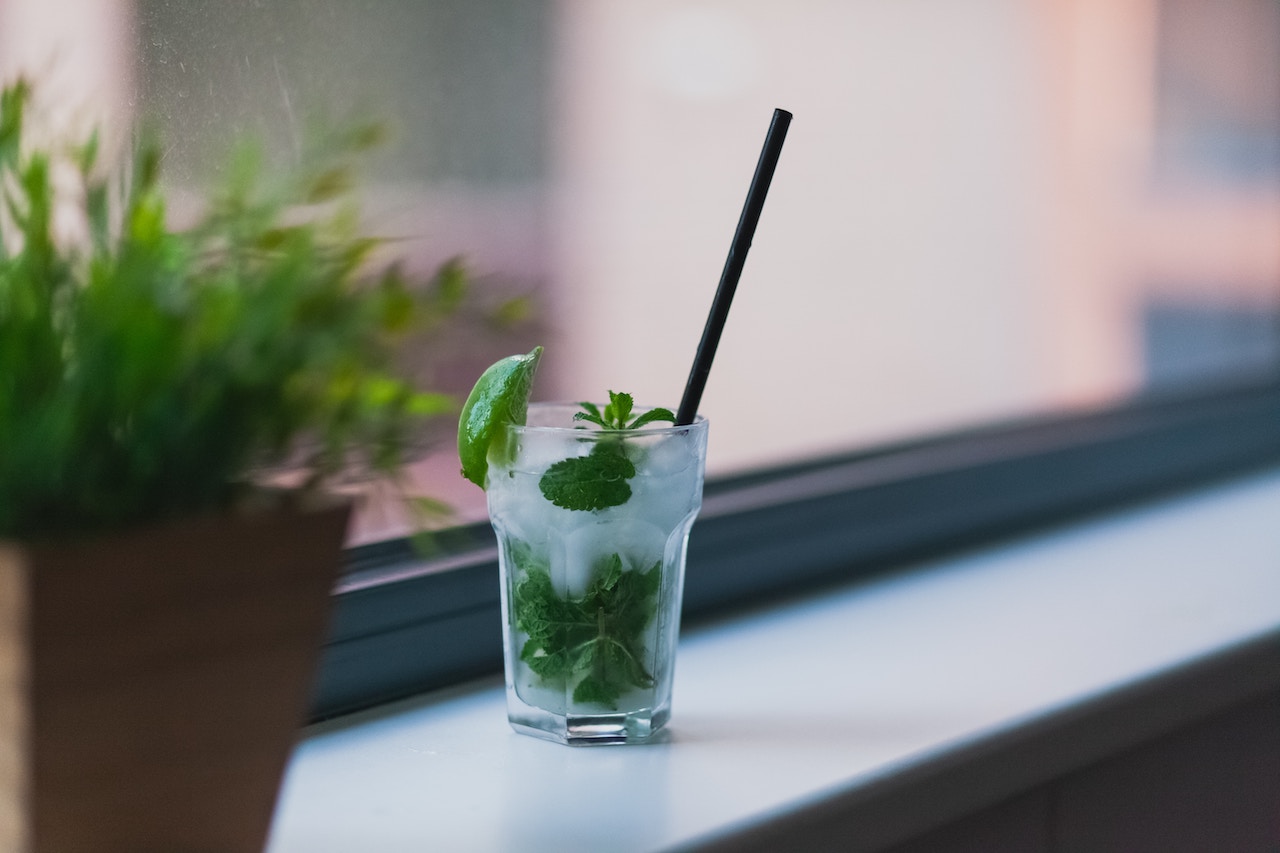
Mockito: ArgumentCaptor
Three ways to create ArgumentCaptor in unit tests (Mockito JUnit Runner, annotations, or factory method) and its different usage.
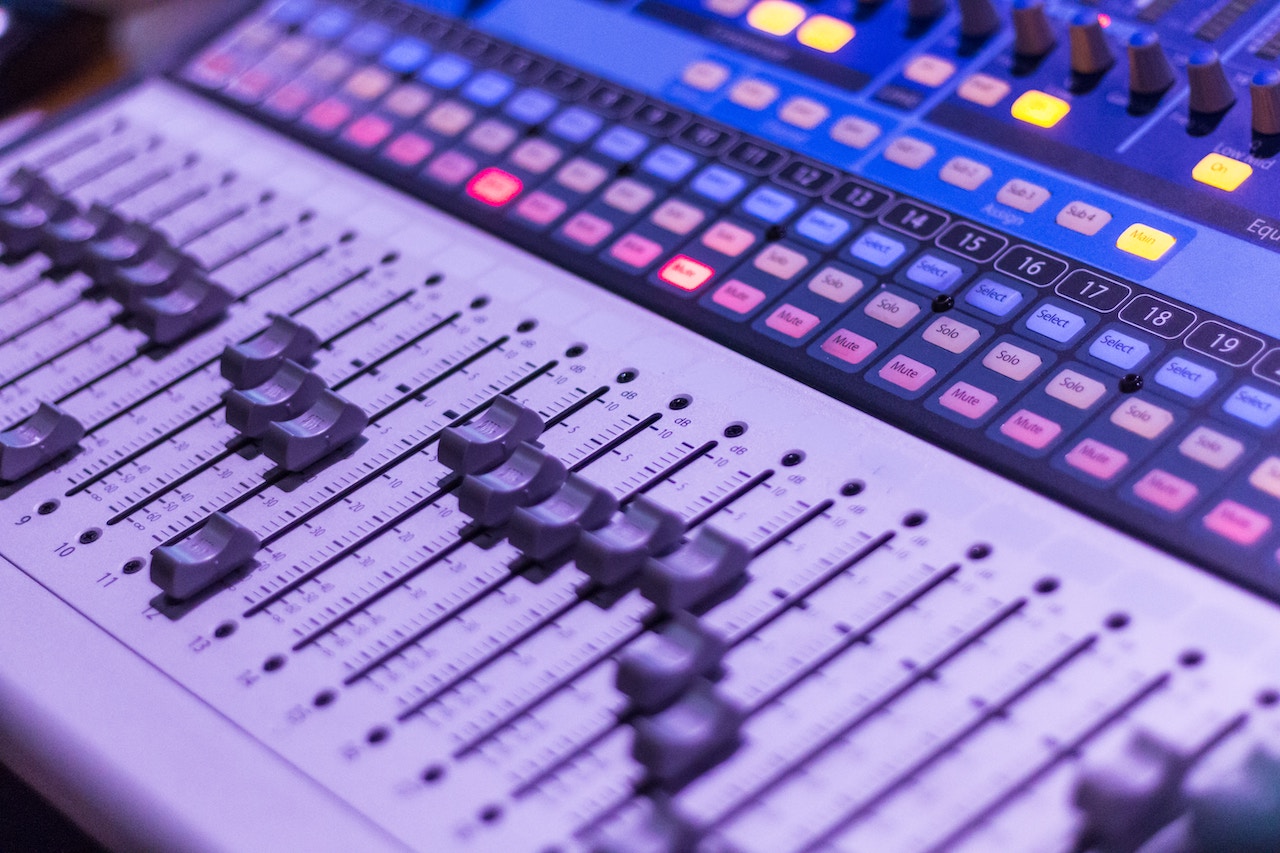
Typesafe Config Introduction
Typesafe Config library: its basic structure, loading mechanism, parsing, substitutions, merging, inclusion, unit format support, IDE plugin, and more.
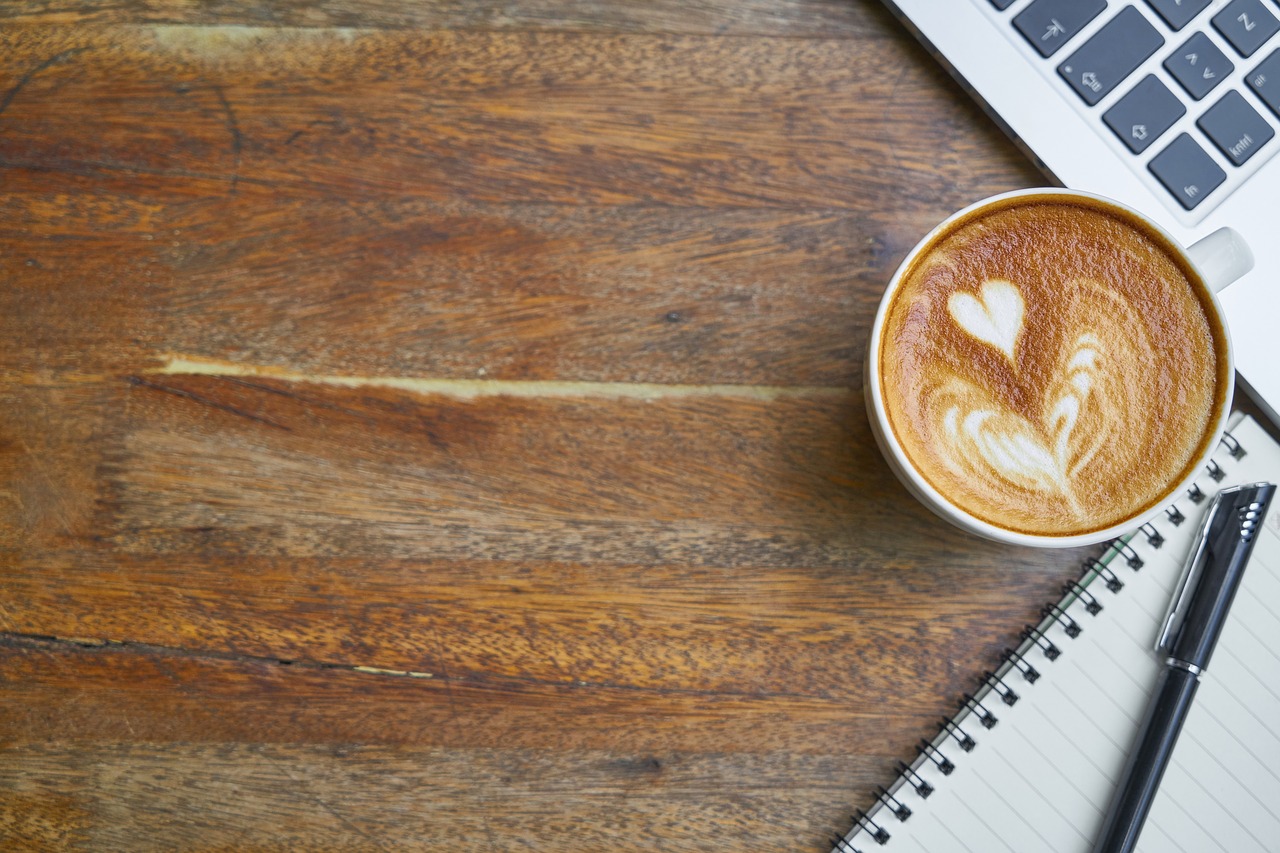
Indexing New Data in Elasticsearch
Initializing Elasticsearch client, sending a single index request or a bulk index request, index response, different content types, refresh policy etc.
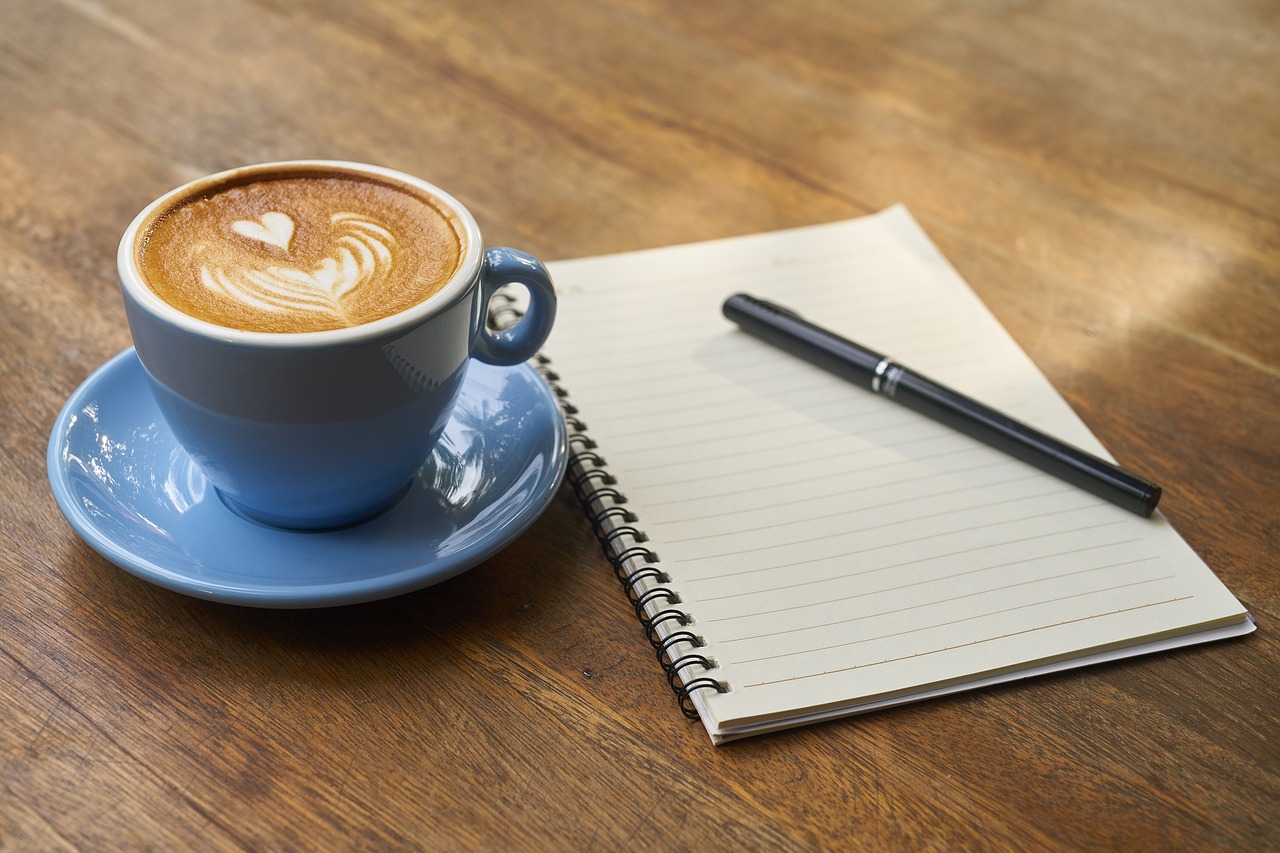
Testing Elasticsearch with ESSingleNodeTestCase
Writing unit tests for Elasticsearch using Elasticsearch Single Node Test Case (ESSingleNodeTestCase), a derived class of ESTestCase which simplifies the testing set up for you.
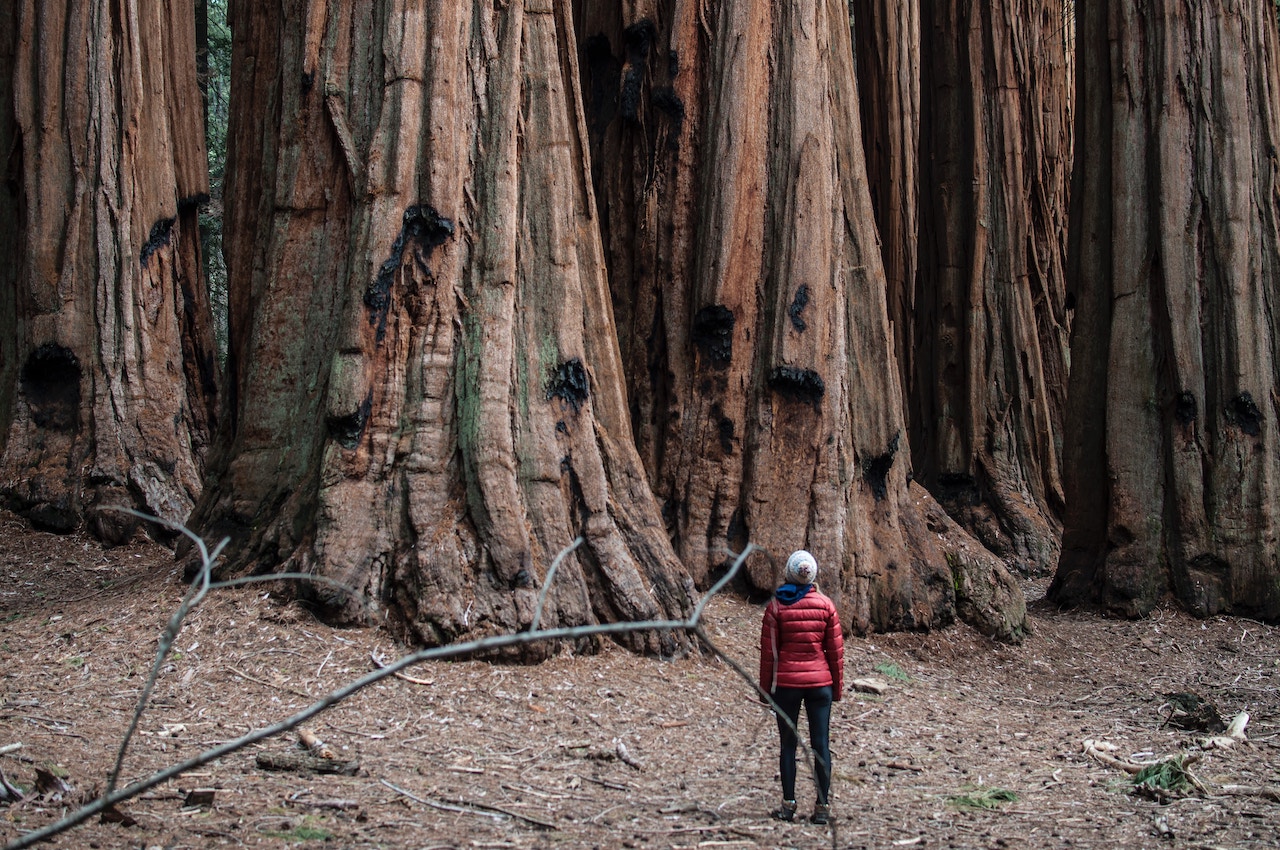
Inspect Maven Dependency Tree
Inspecting Maven dependencies via Maven Depenedency Plugin: dependency listing, dependency filtering, exporting result to file etc.
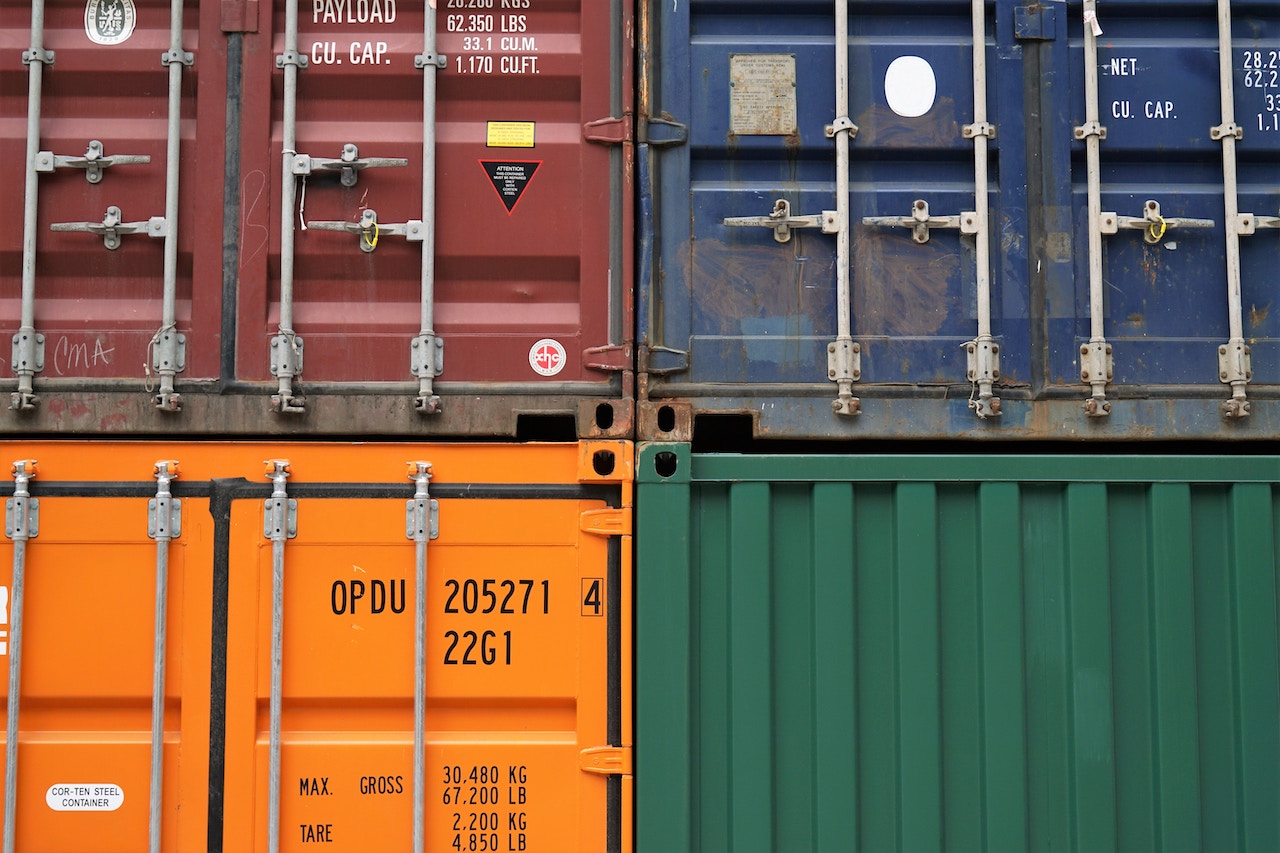
Docker Maven Plugin
Introduction to Docker Maven Plugin: how to build and run Docker images in Maven via samples from Debezium and Docker Maven Plugin itself.
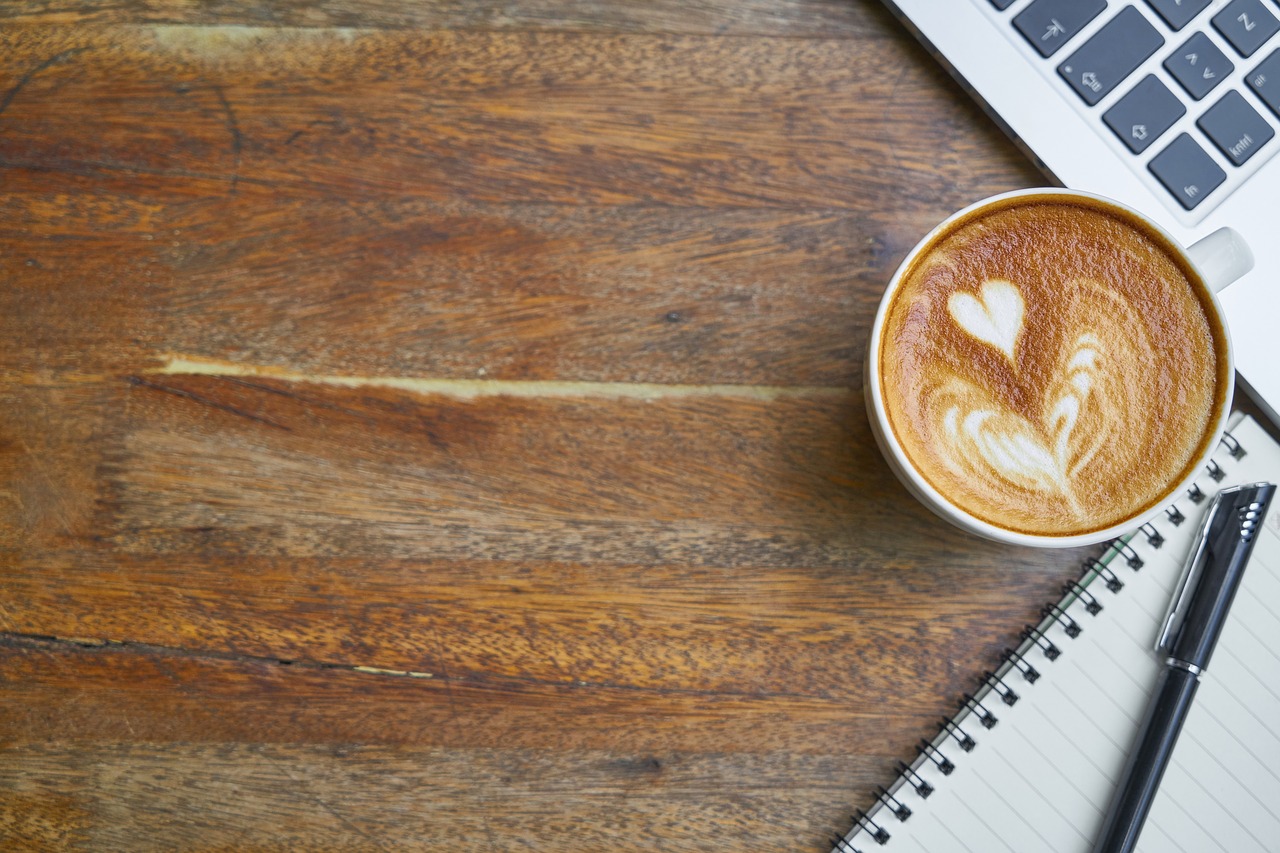
Unzipping File in Java
Unzipping zip file in Java 8 using builtin Java classes: ZipInputStream and ZipEntry. Implementation supports sub-directories as well.
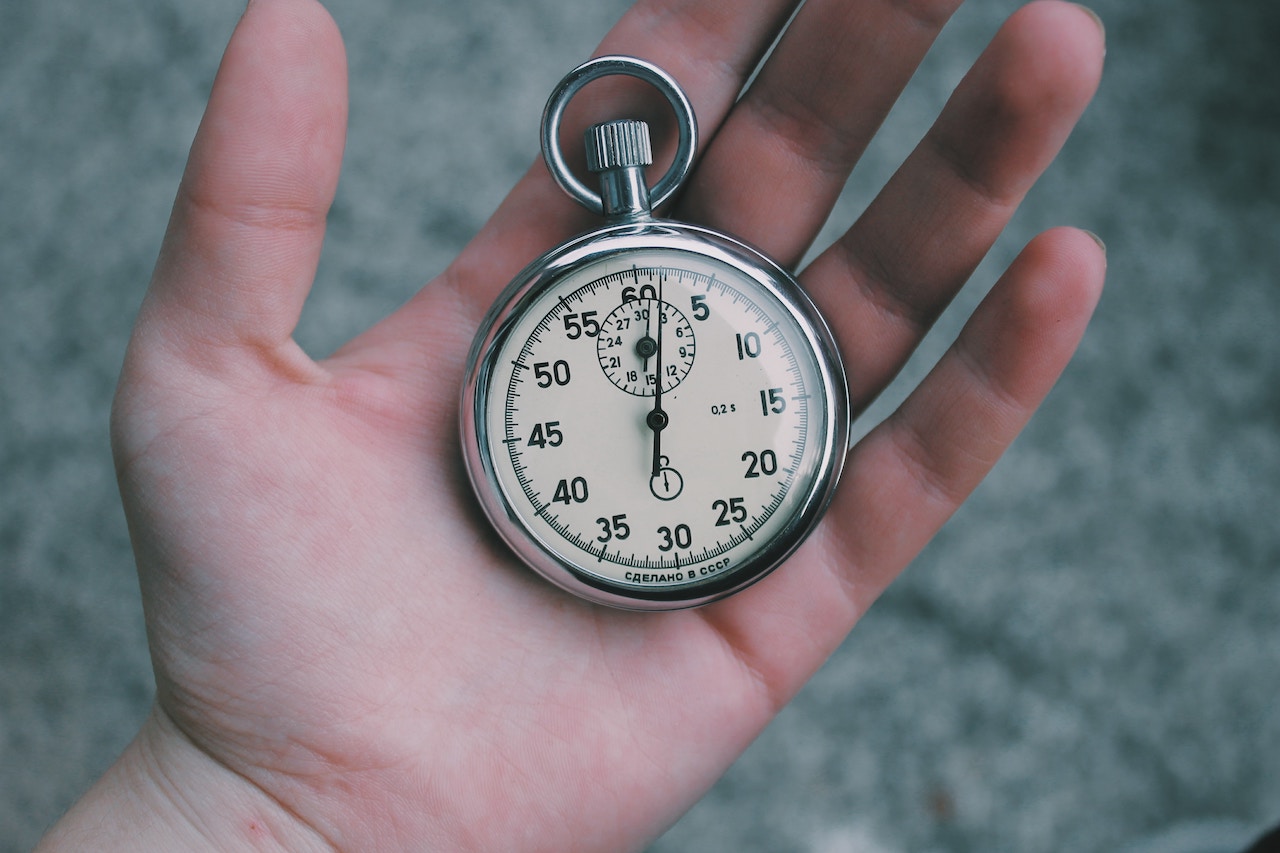
Performance Improvements on Nuxeoctl
How NOS team improves the performance of Nuxeoctl, Nuxeo Server's command line, recently.
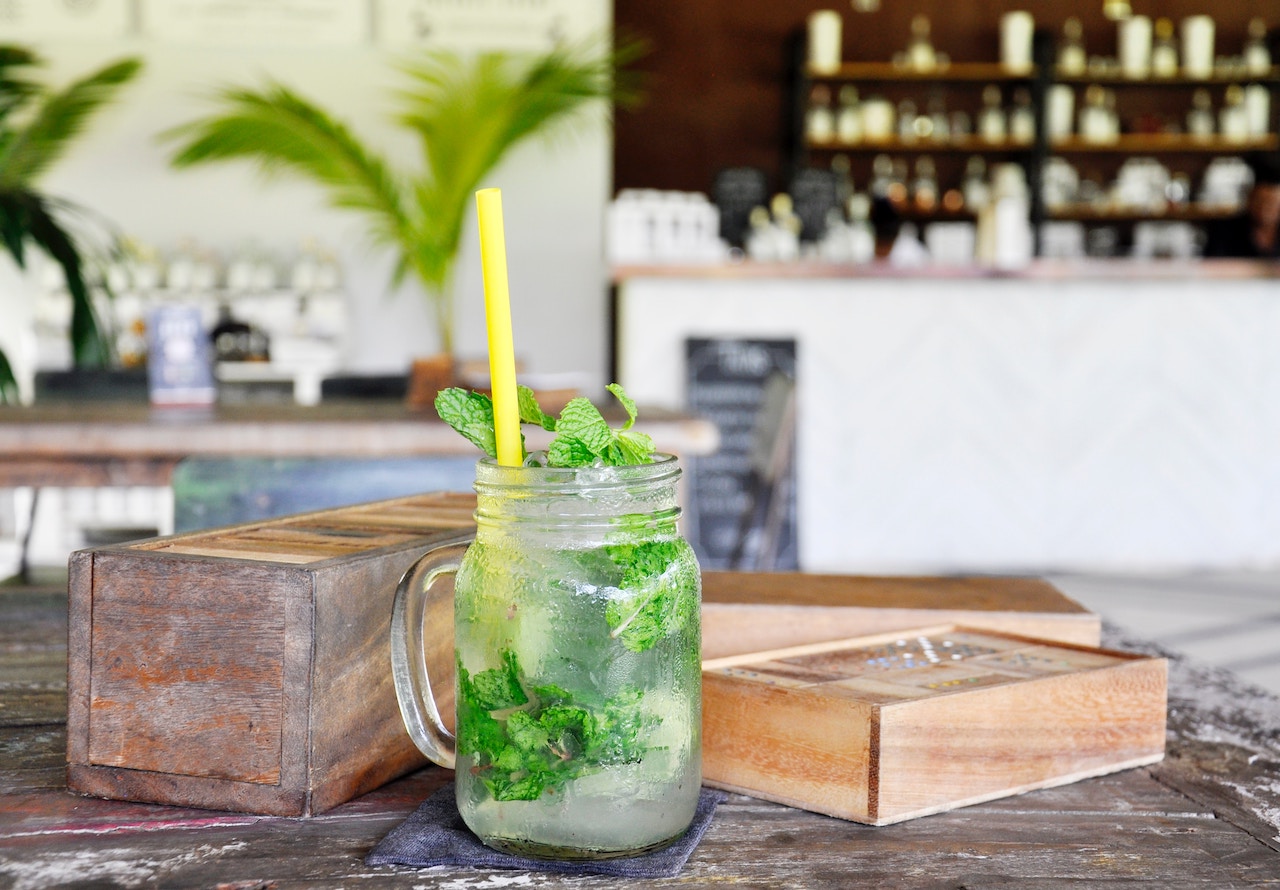
Mockito: 4 Ways to Verify Interactions
Verify interaction with mock objects with verify(), verifyZeroInteractions() verifyNoMoreInteractions(), and inOrder().
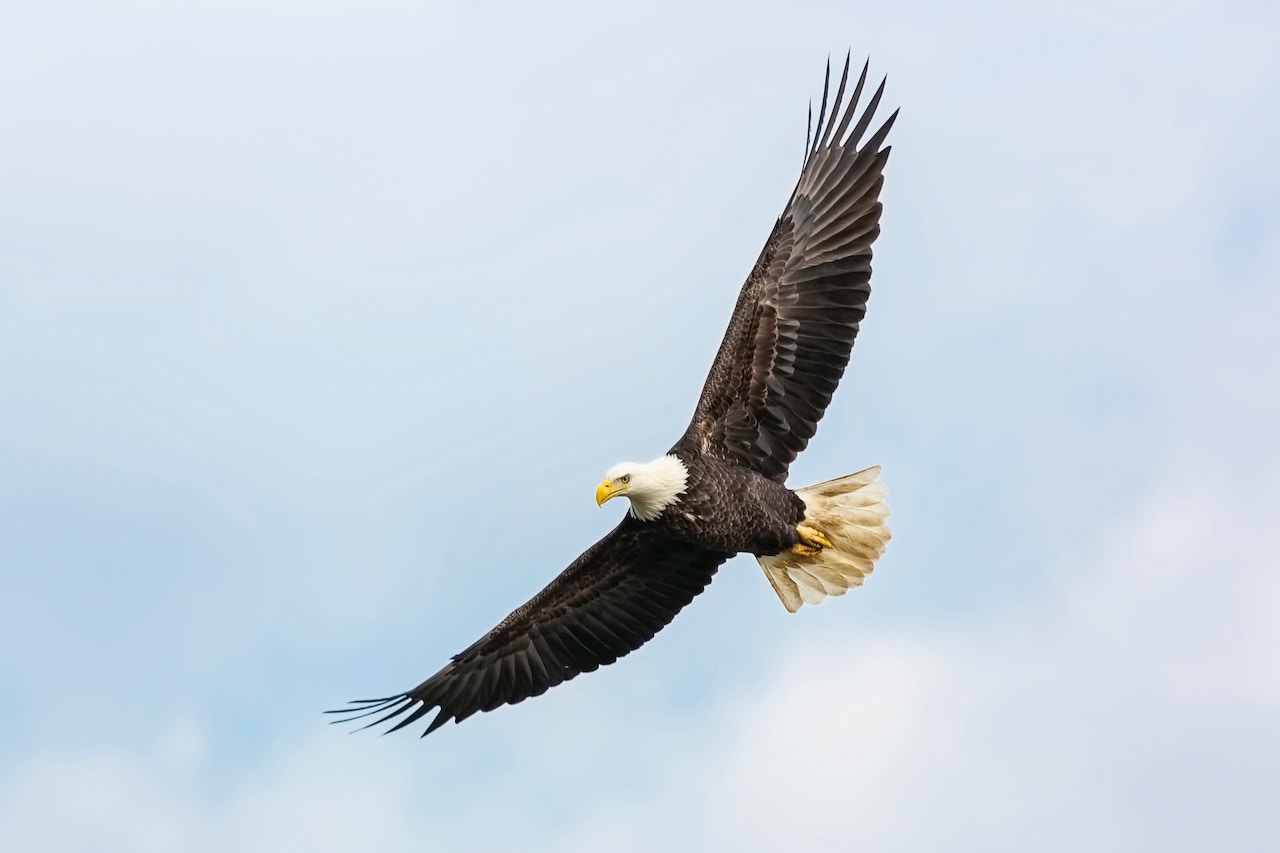
Project: NOS Test
NOS Test is a QA project consists of 3 parts: RESTful API, browser, and command line. In this article, I will explain how I implemented it.
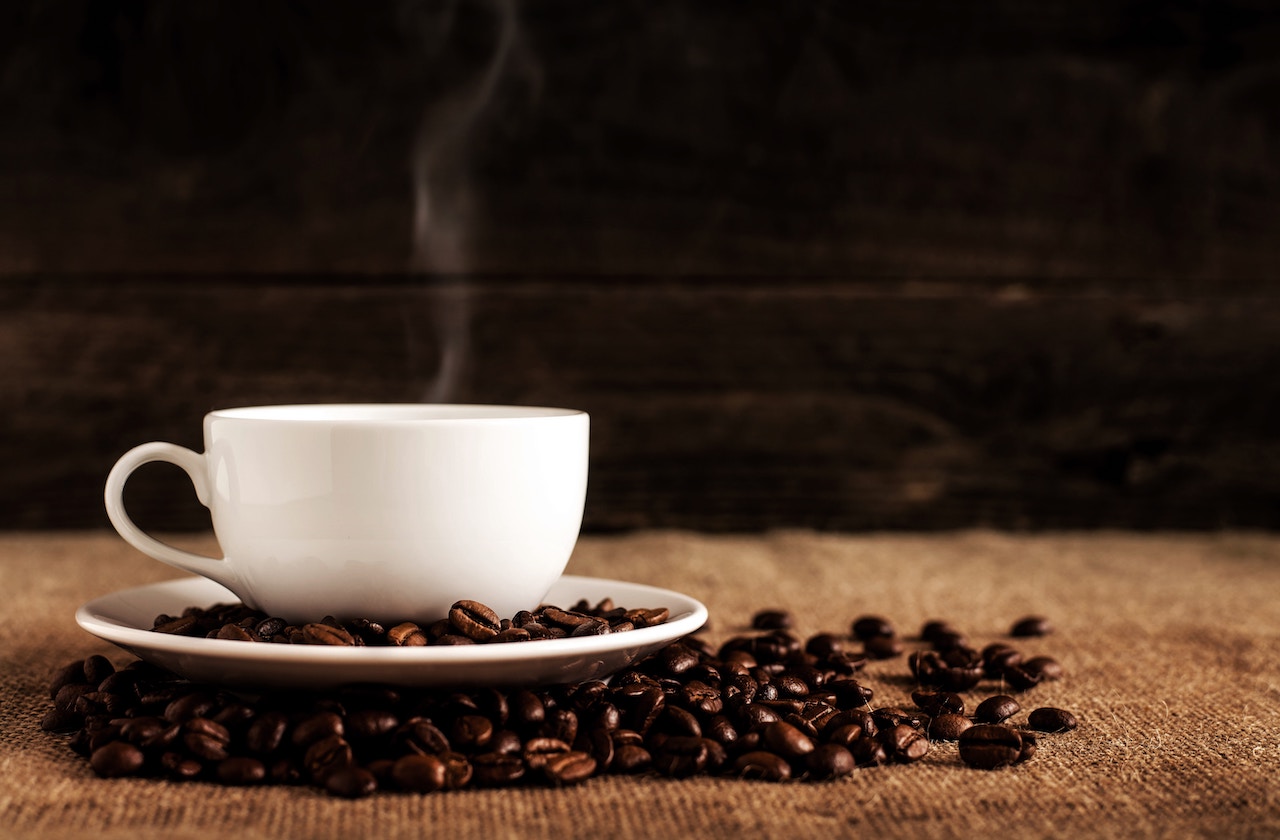
Mockito: 3 Ways to Init Mock in JUnit 4
Initialize Mockito mock objects in JUnit 4 using MockitoJUnitRunner, MockitoAnnotations#initMocks, or Mockito#mock.
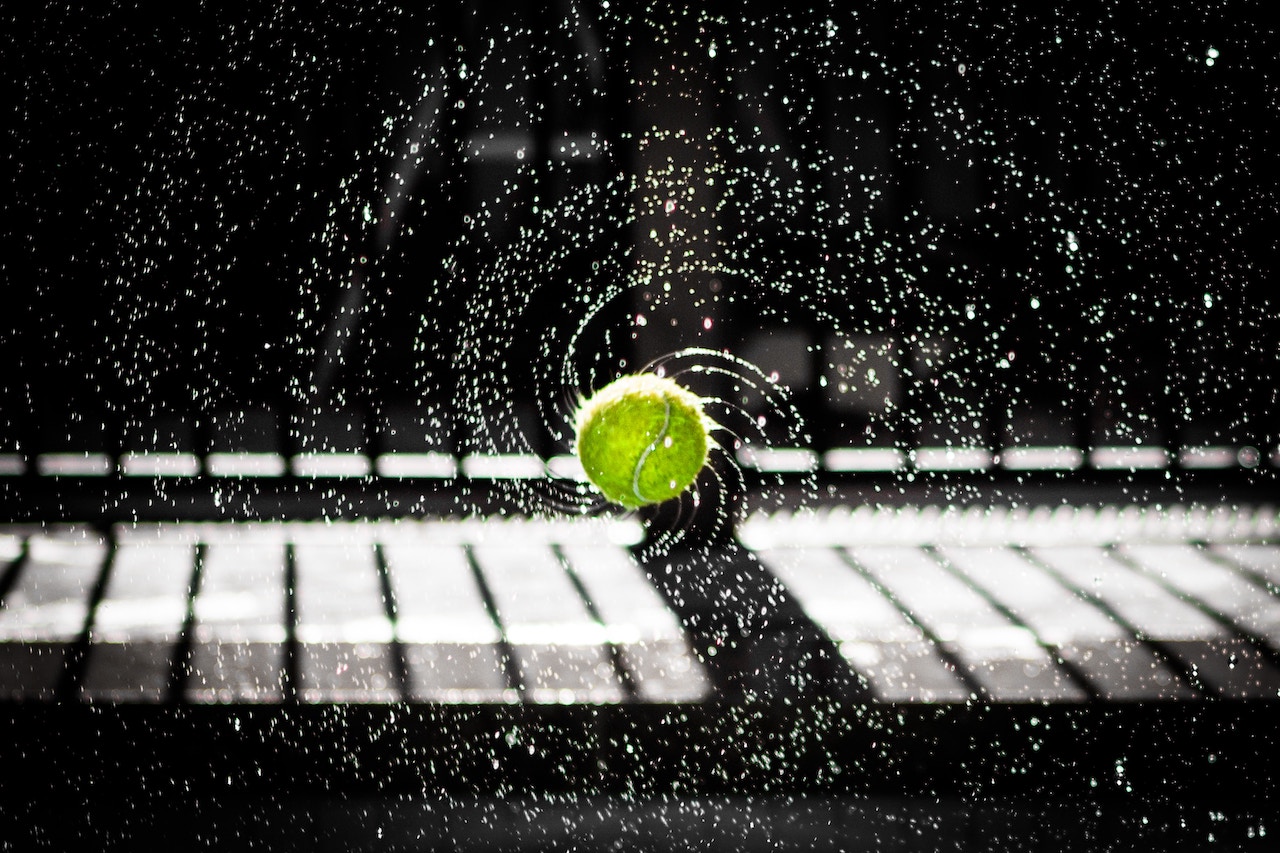
JGit: Config Loading Optimization
How JGit optimizes internally the configuration loading process using file snapshots and reduces unnecessary file I/O?
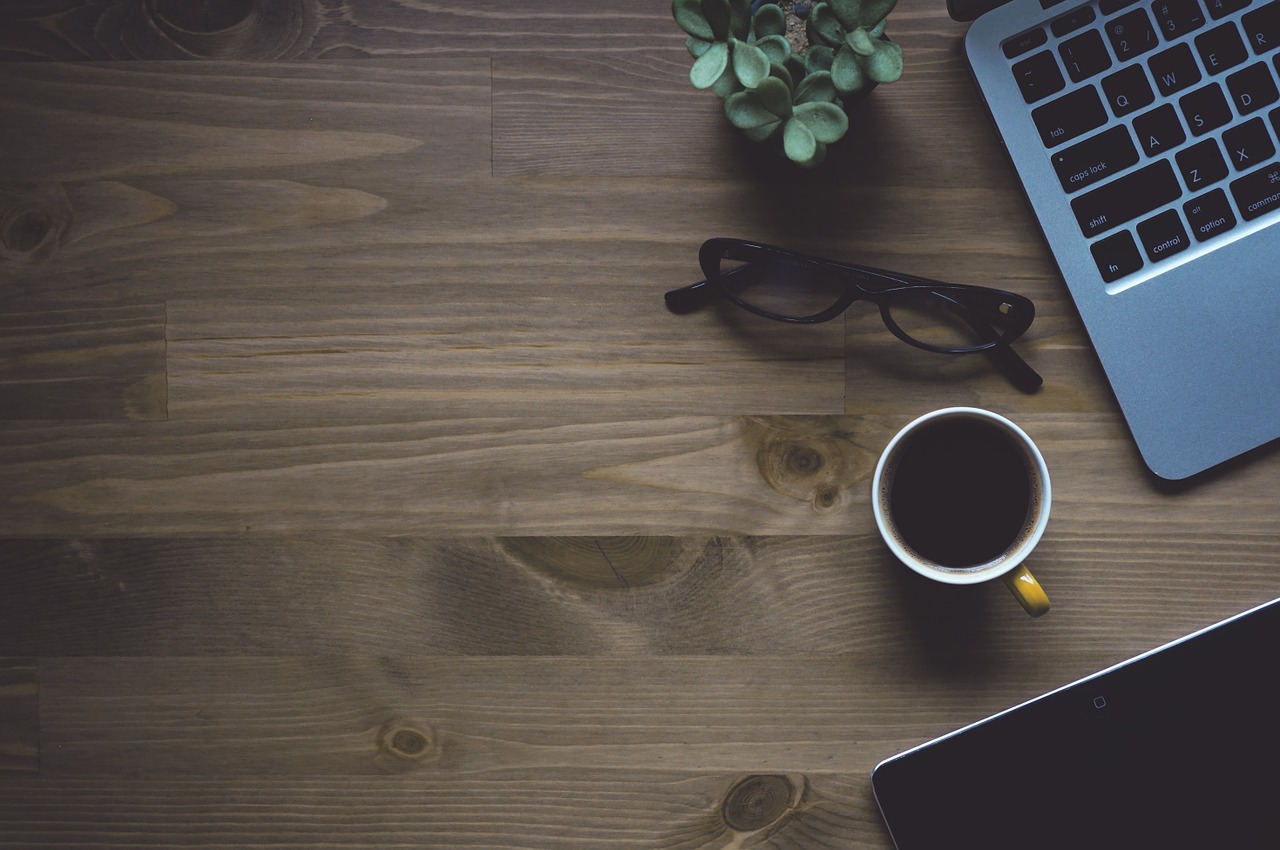
Checkstyle CustomImportOrder
Fix import order in Java class using Checkstyle's CustomImportOrder module. This article explains how I did it for Nuxeo Online Services.
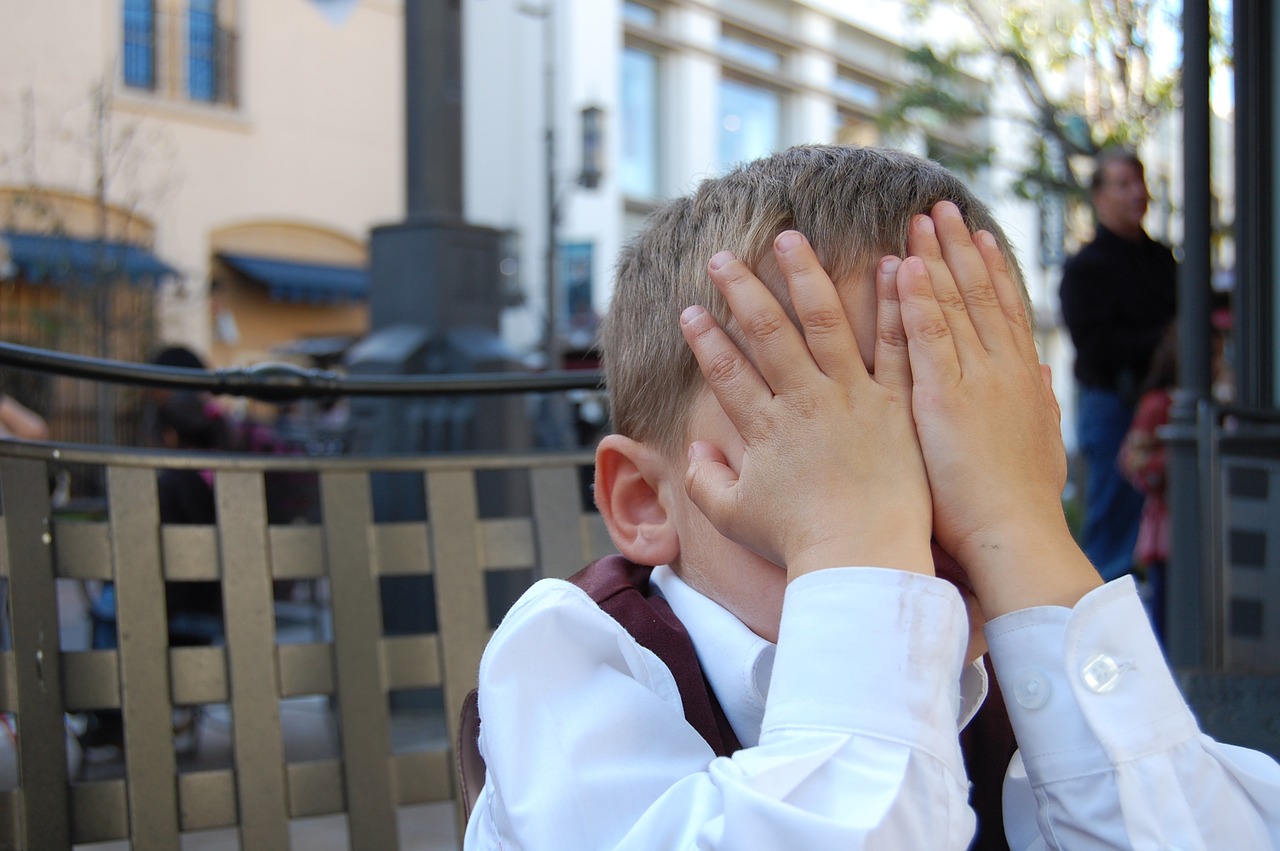
6 Tips for Fixing Bugs with Legacy Frameworks
In my daily work, I have to deal with legacy frameworks. Here are 6 tips that I summarized for bug-fixing, including documentation, searching, testing, patching library and more.
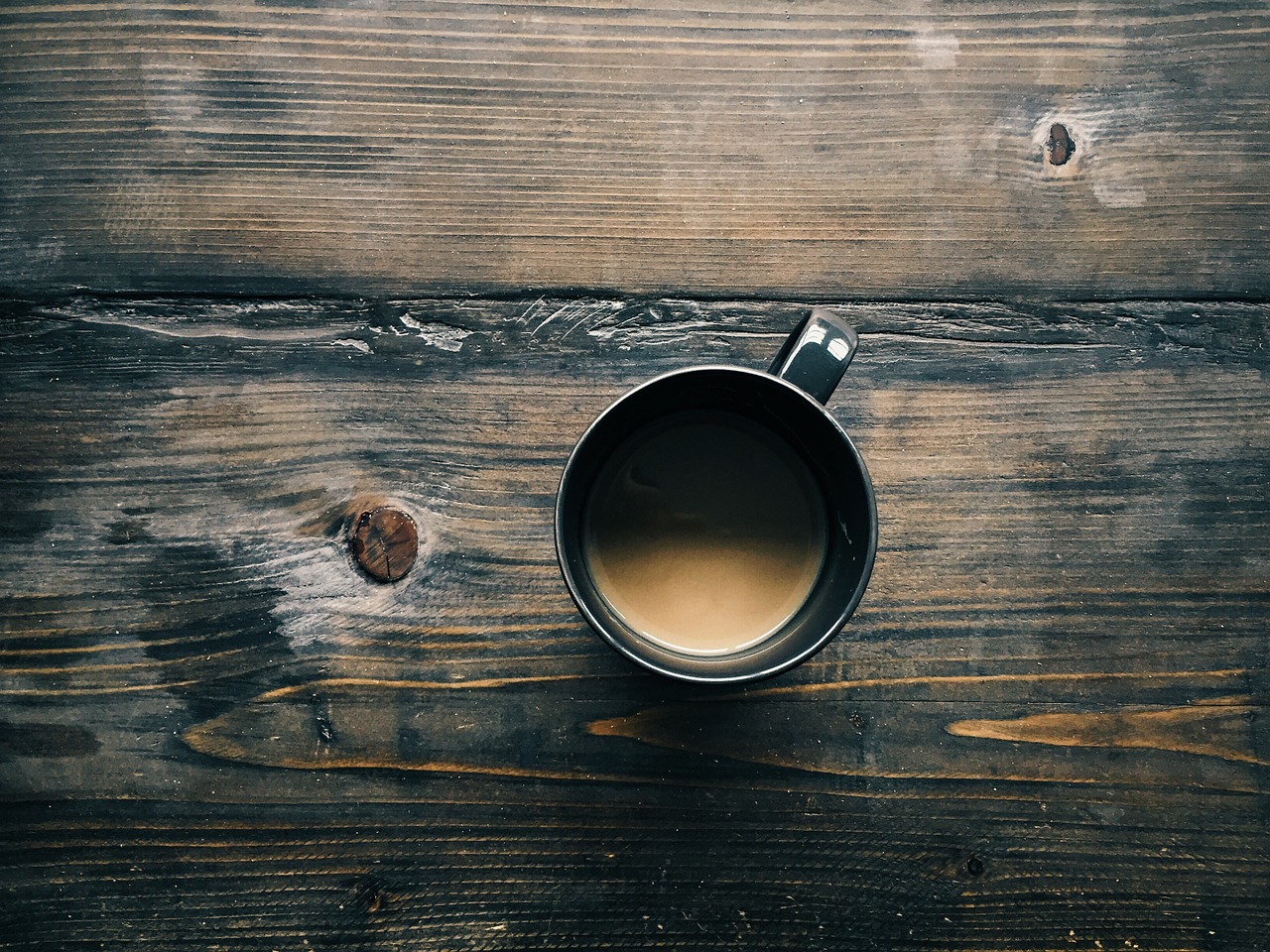
Introduction to Commons CLI
Simple but common use-cases: create options, create option, parse command line arguments, print usage, and more using Apache Commons CLI.
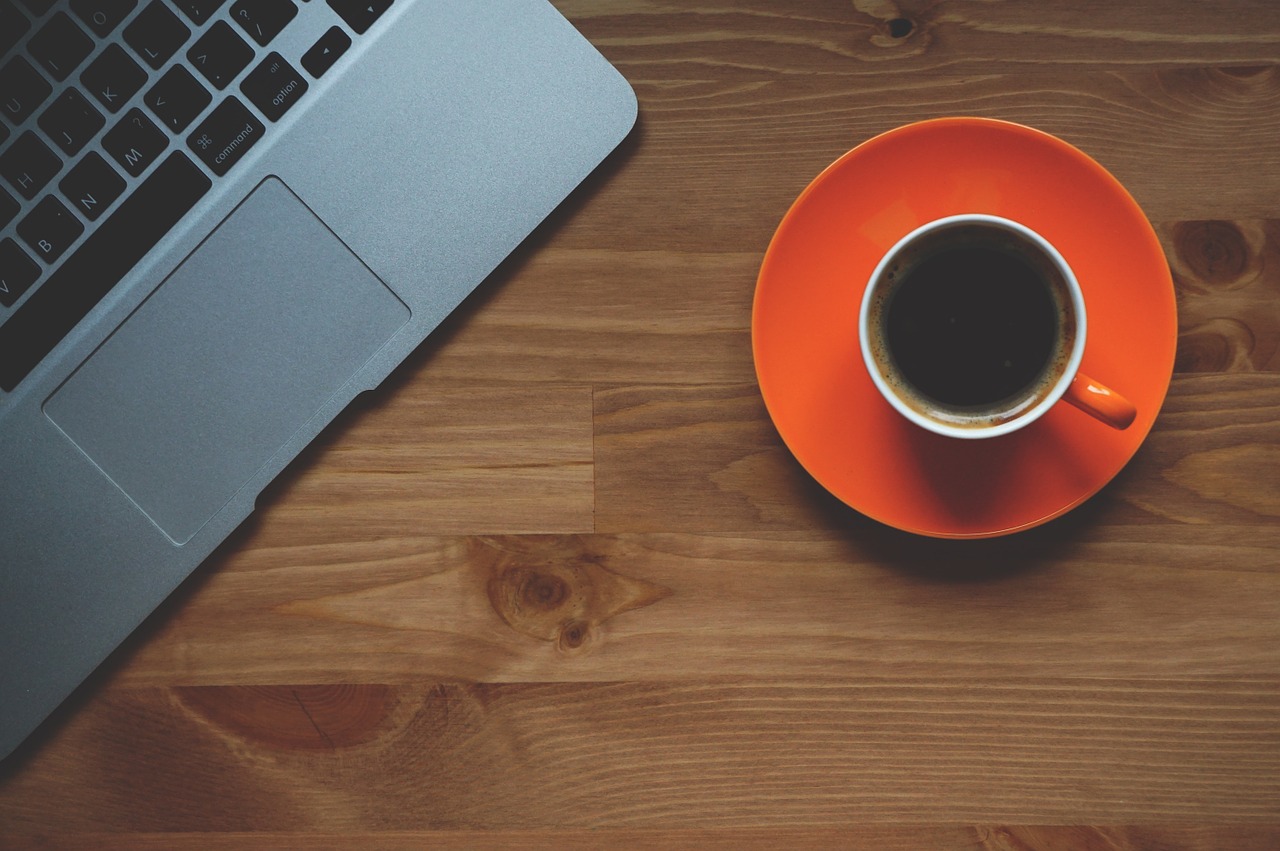
Fixing Comparator
Fun experience on fixing a custom comparator by identifying the sub-problems, testing different combinations, and finally fix it.
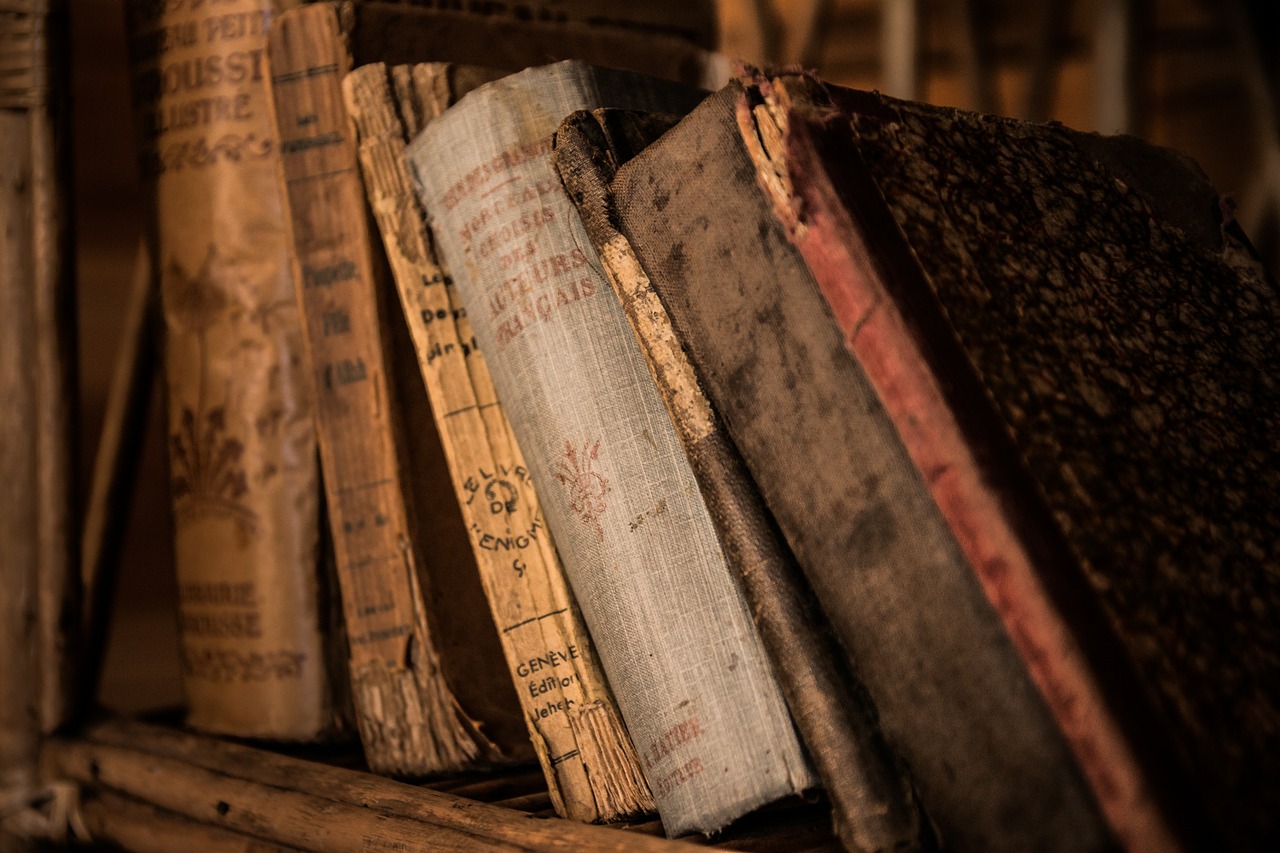
Specifying Maven Local Repository From CLI
Use option "maven.repo.local" to specify the Maven local repository path.
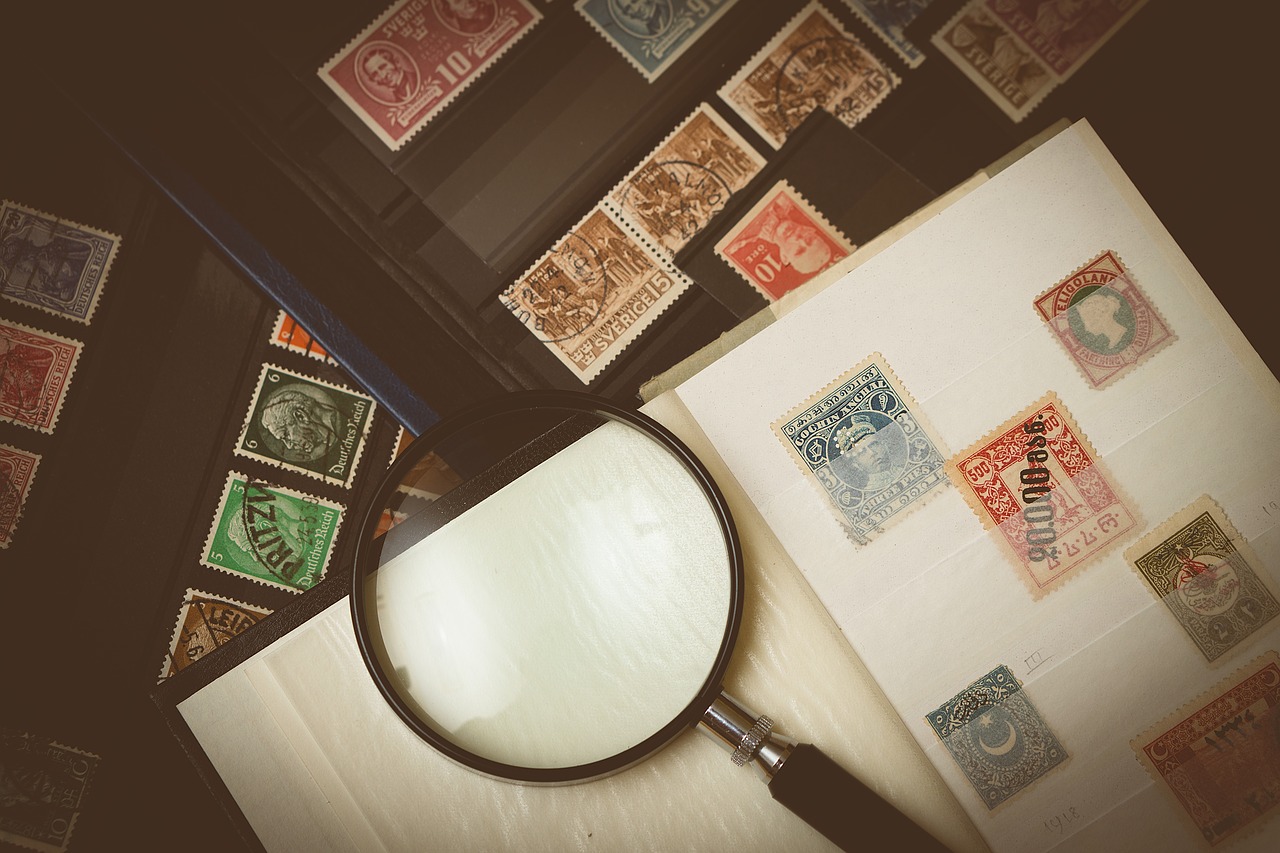
Viewing the Contents of JAR
Listing files inside a JAR or displaying content of a single file using different commands: "jar", "unzip", or "vim".
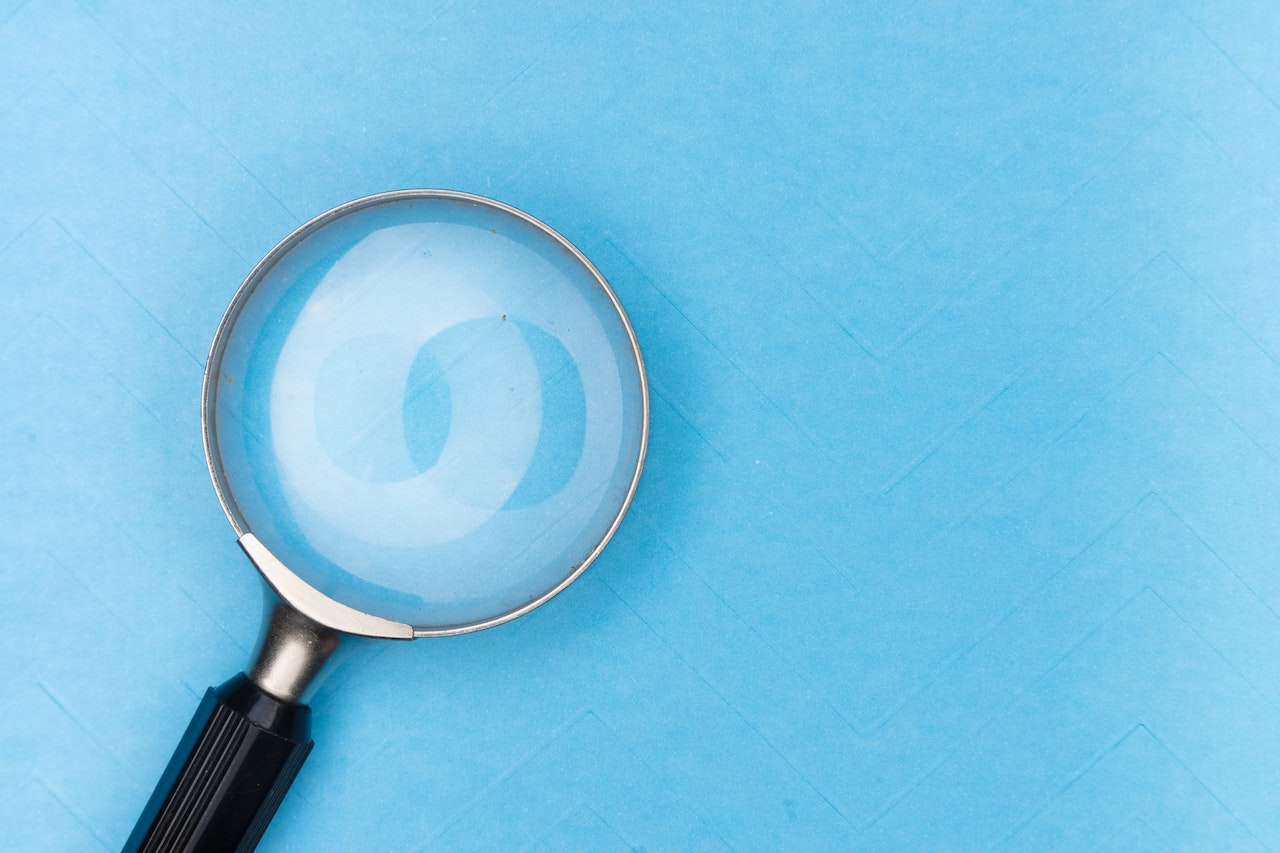
Glob Expression Understanding
Glob expression syntax, and its usage in Java through Path Matcher and Directory Stream.
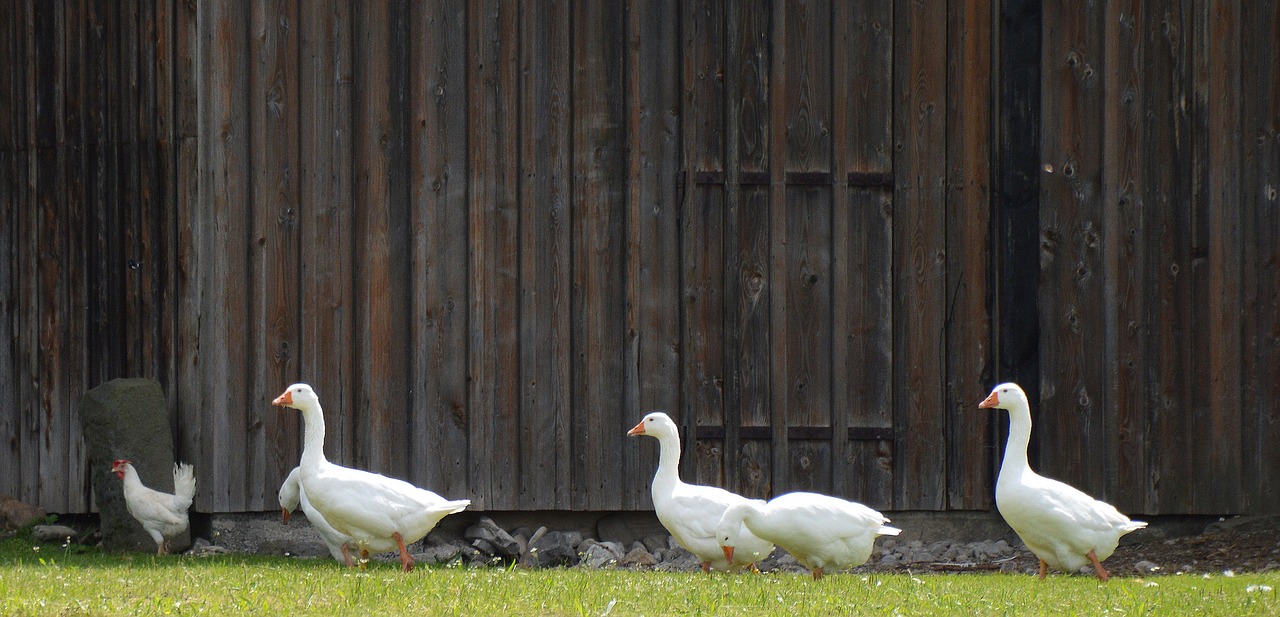
Understanding ISO-8859-1 / UTF-8
Character mapping between ISO-8859-1 / UTF-8, decode and encode data between string and bytes, and file I/O operations including MIME encoding detection. All examples are written in Java and Python 3.
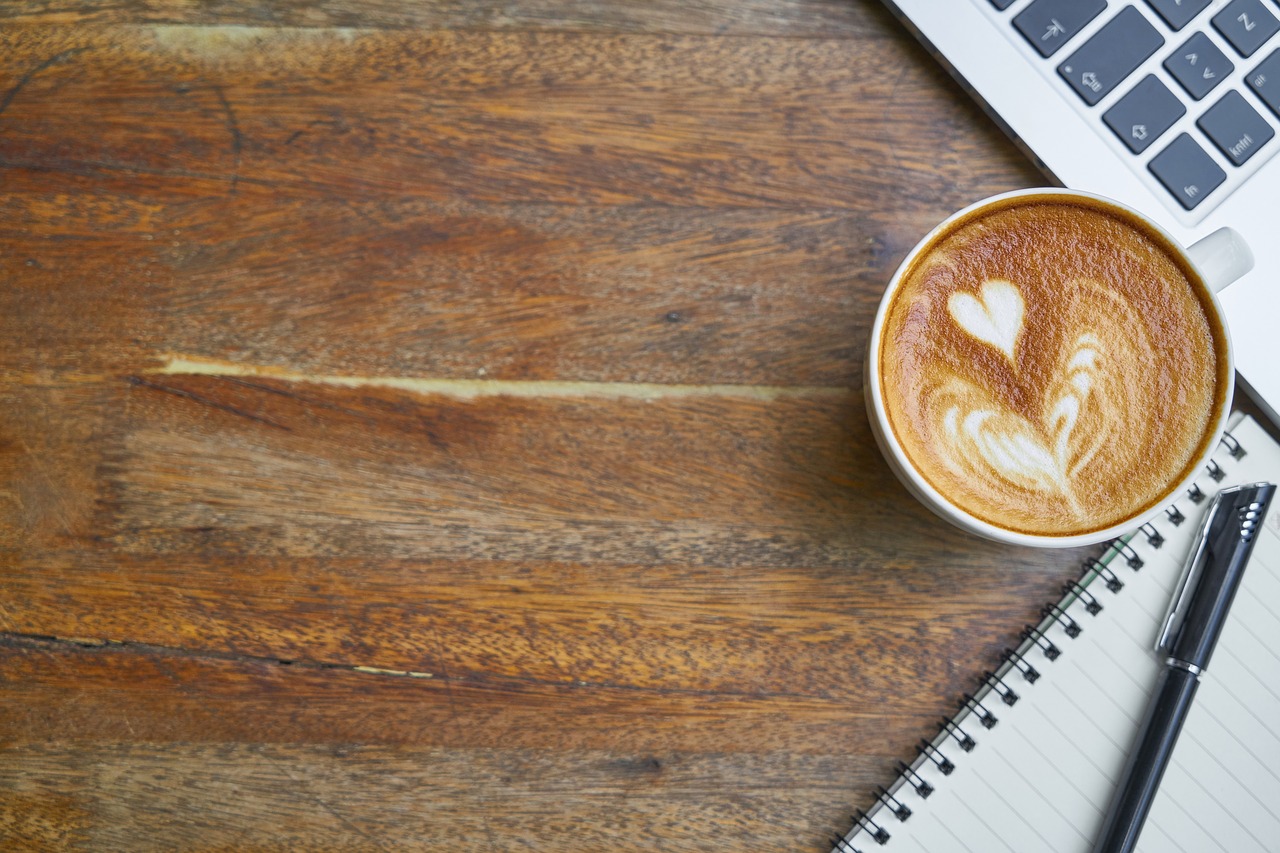
Jackson XML Mapper
How to do Java / XML mapping using Jackson XML Mapper. This article explains the annotations used for root element, property, and collection mapping. Also, the basic configuration of Jackson XML Mapper.
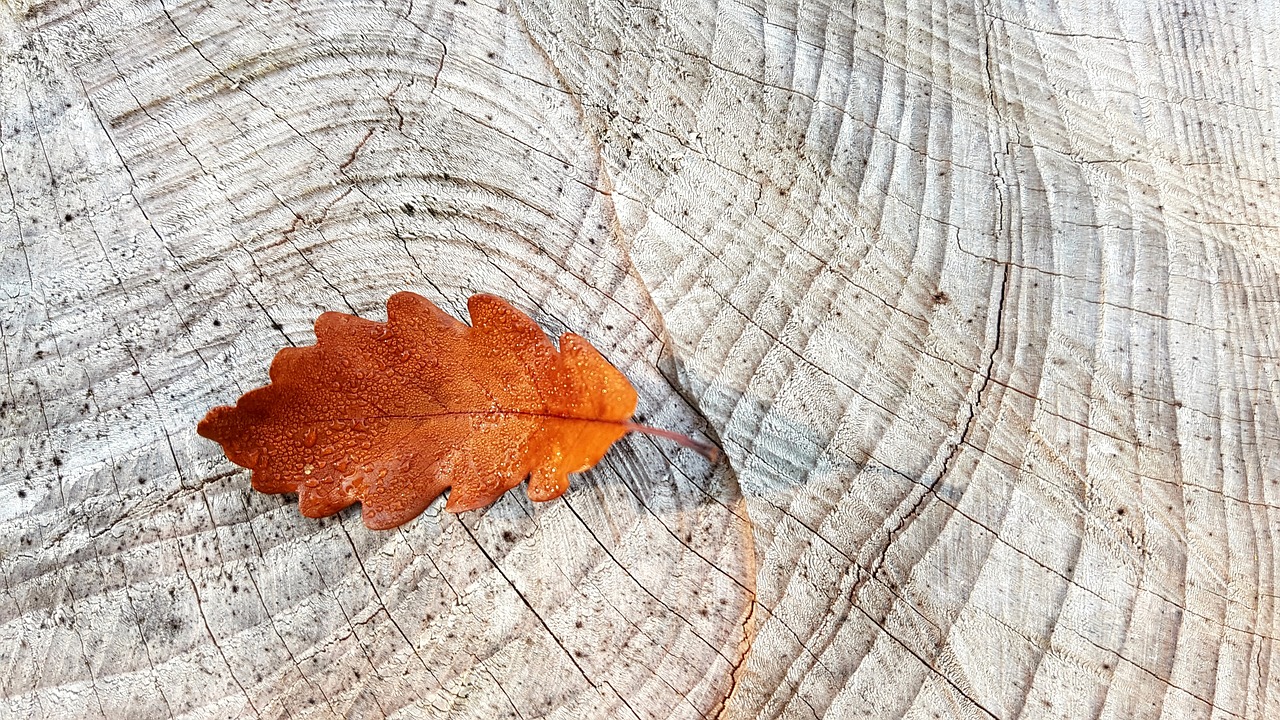
SLF4J Understanding
Simple Logging Facade for Java (SLF4J) reduces the coupling between application and logging framework. It support various logging frameworks as bindings. In this article, I will explain what is SLF4J, why and how to use it.
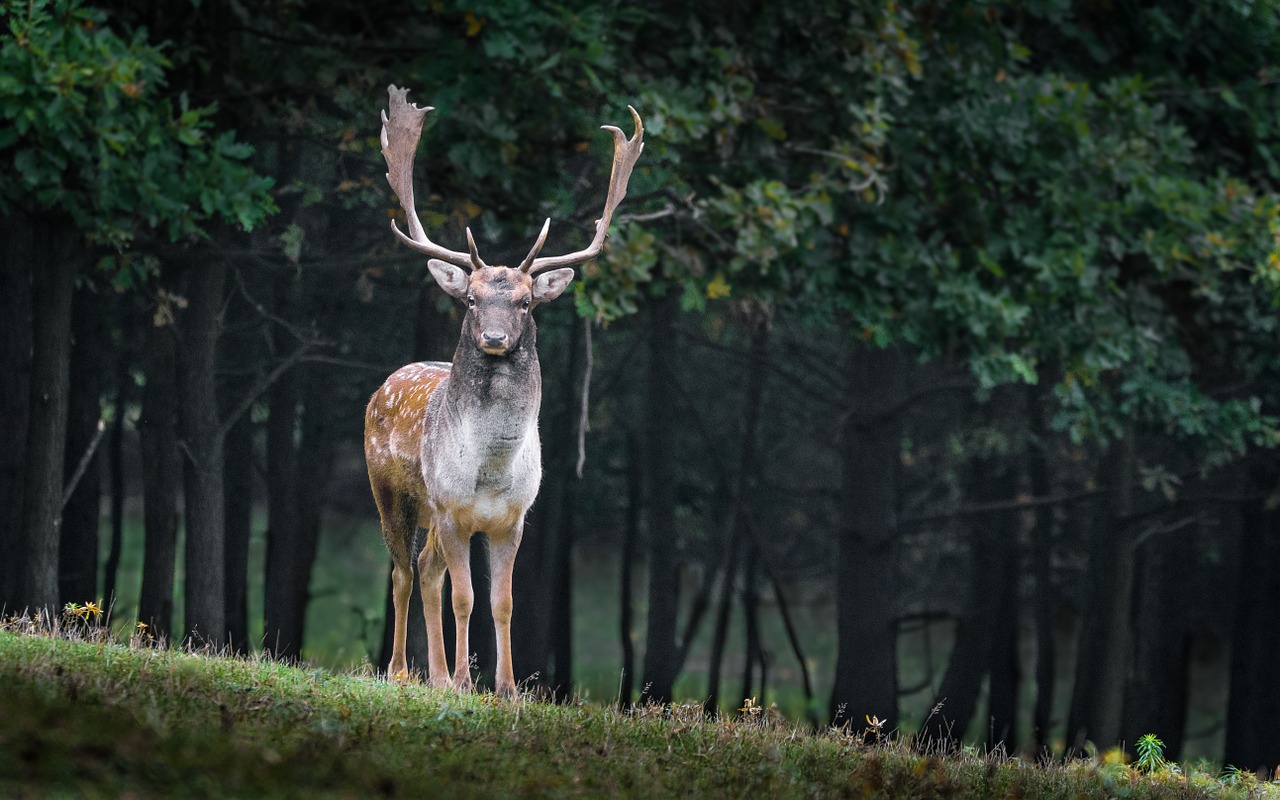
What I Know About Logs
The information inside a log event, the advantages of having a log platform (aggregate, enrich, search, analysis, monitor), the sources of logs, and log analytics.
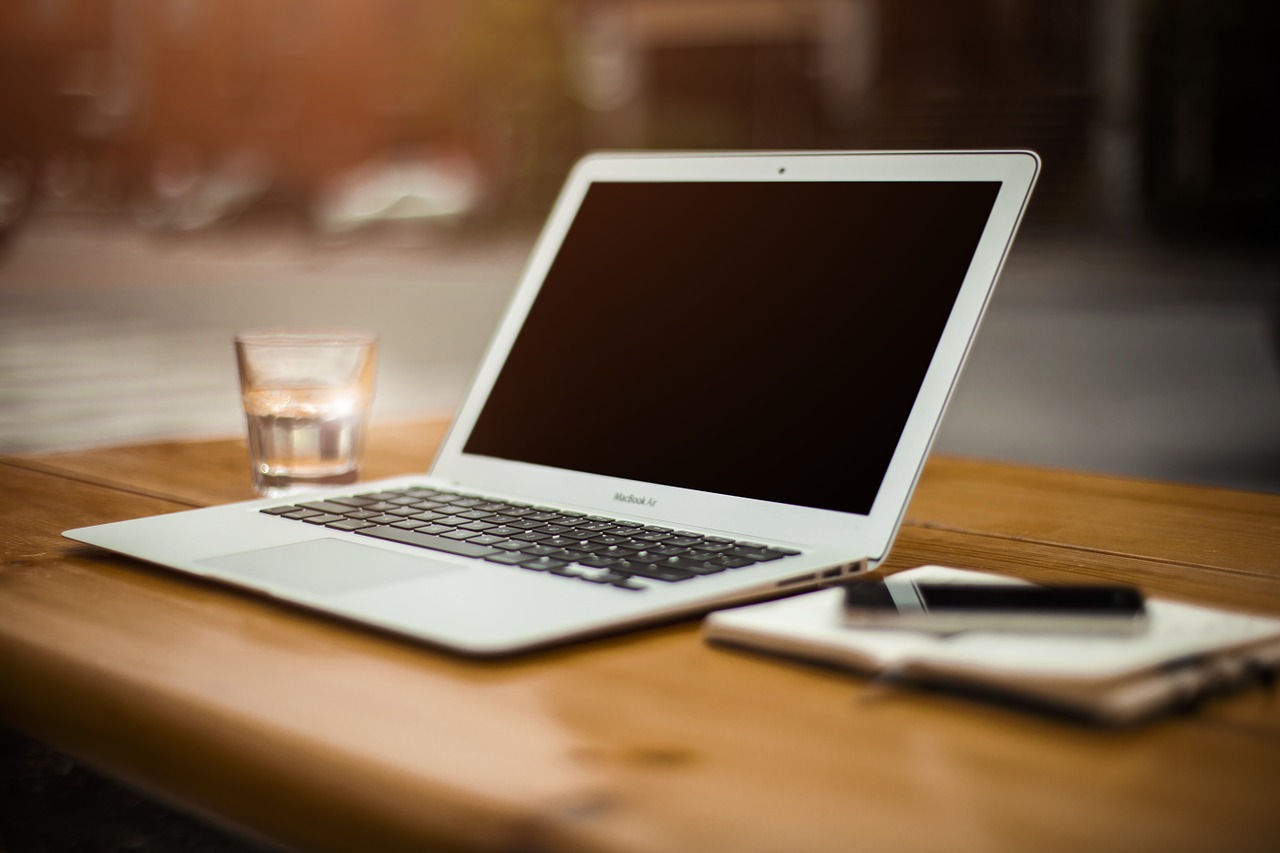
Project qWatch
Quality Watch (qWatch) is a data aggregator for code quality, based on different metrics.
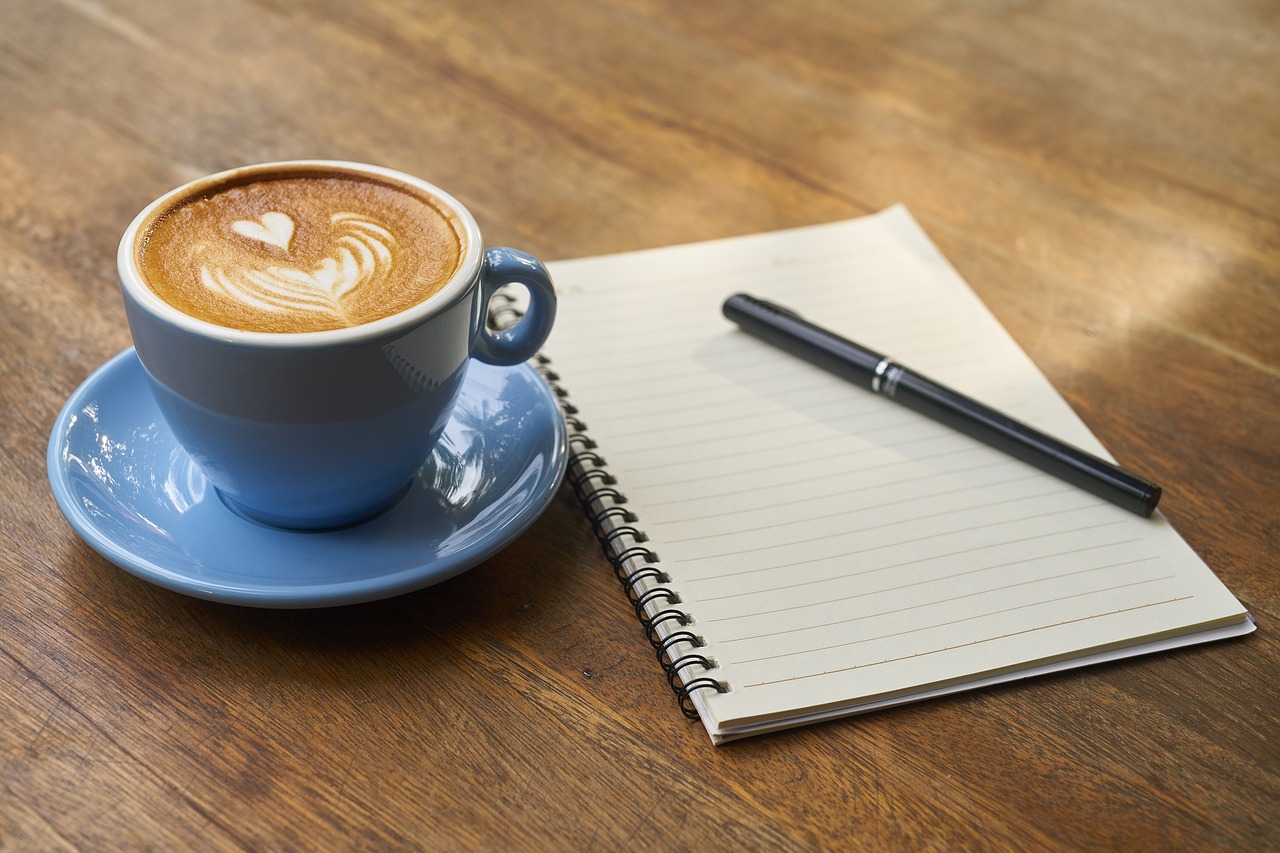
Design Pattern: Static Factory Method
Understand static factory method pattern in Java with concrete examples from Selenium WebDriver, Jackson JSON object mapper, and SAX reader for XML.
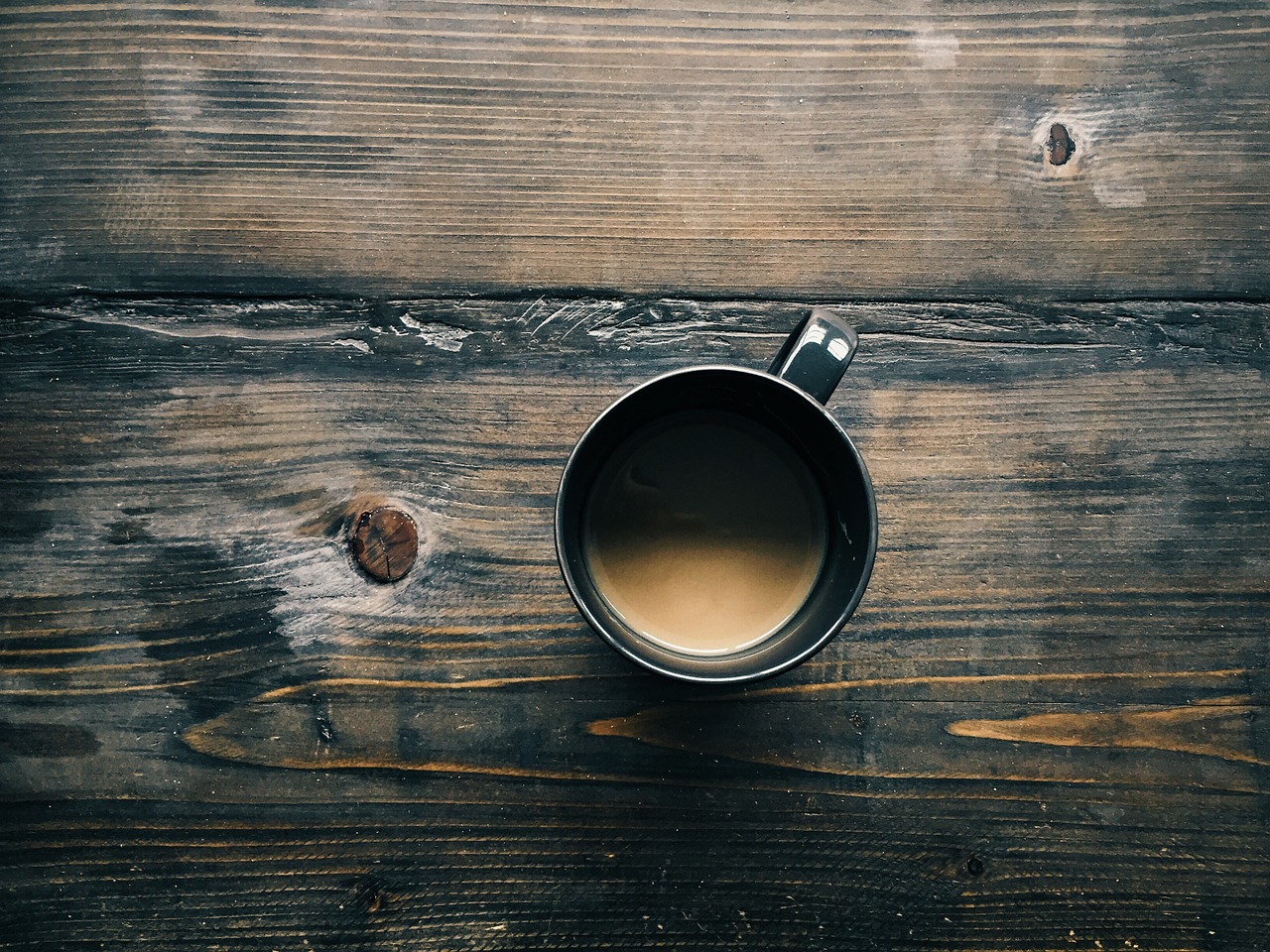
Vavr List vs Java List
Difference between Vavr List and Java List? I'll compare them via CRUD operations, immutability, performance, streaming, and thread safety in this article.
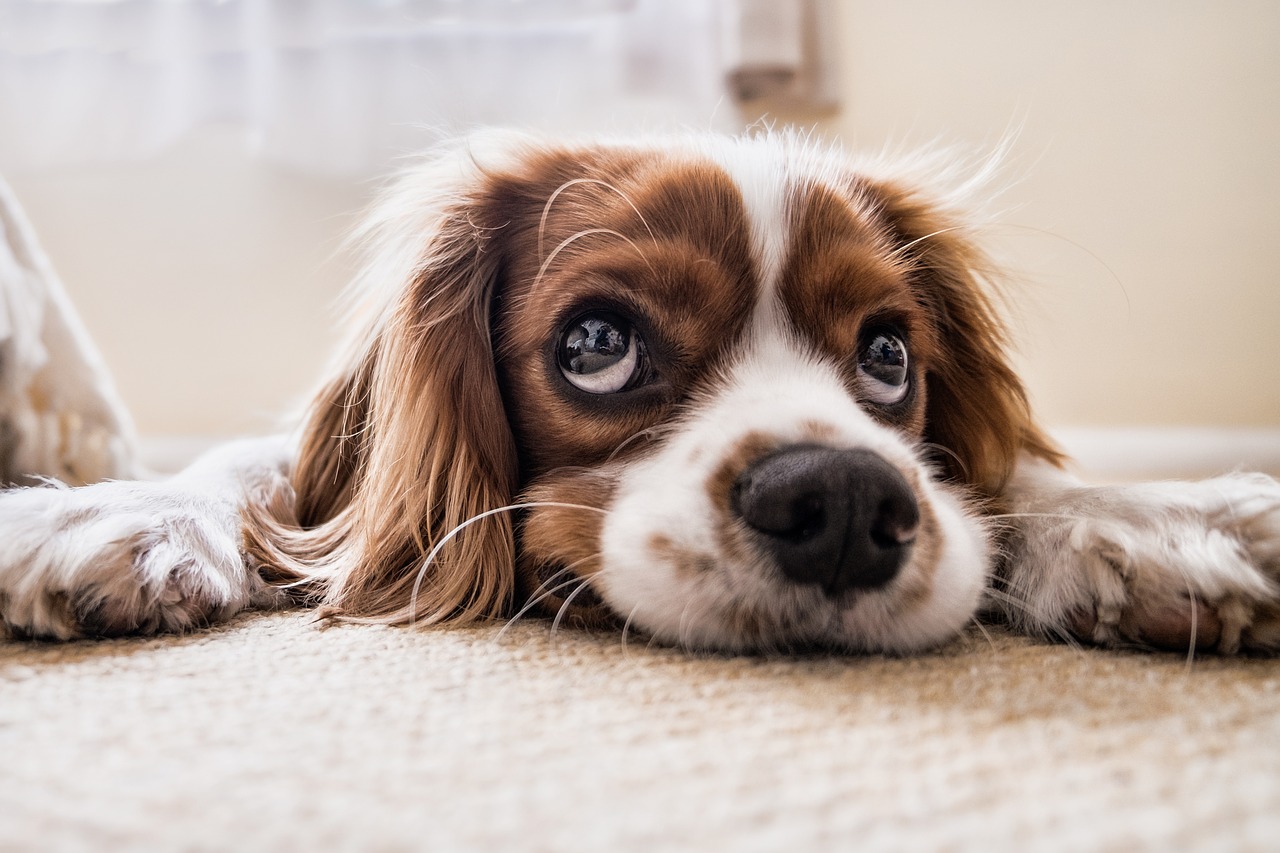
TDD: After 3 Months' Practice
I started TDD in all my personal projects 3 months ago. Here're some thoughts about it, including architecture, IDE, methodology, execution speed up, legacy code, and limits.
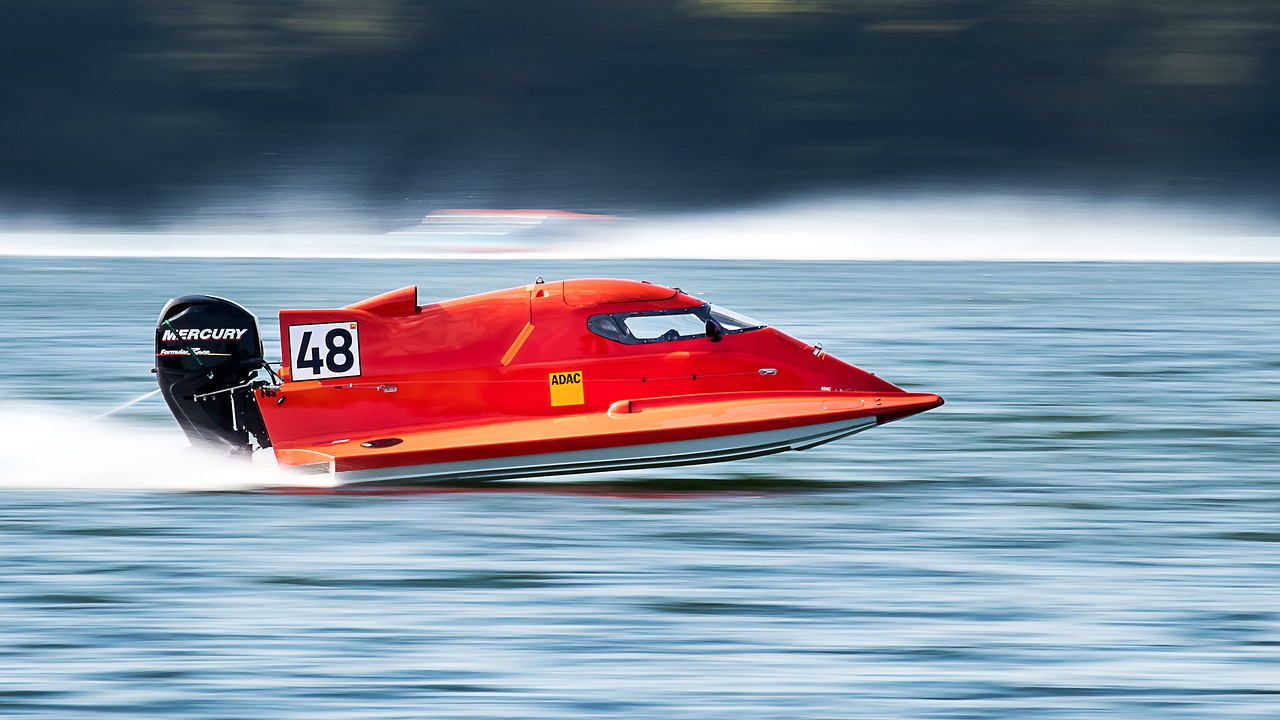
ExecutorService.invokeAll()
Using 100% CPU effortlessly in Java: submit all your tasks to thread pool and wait for completion.
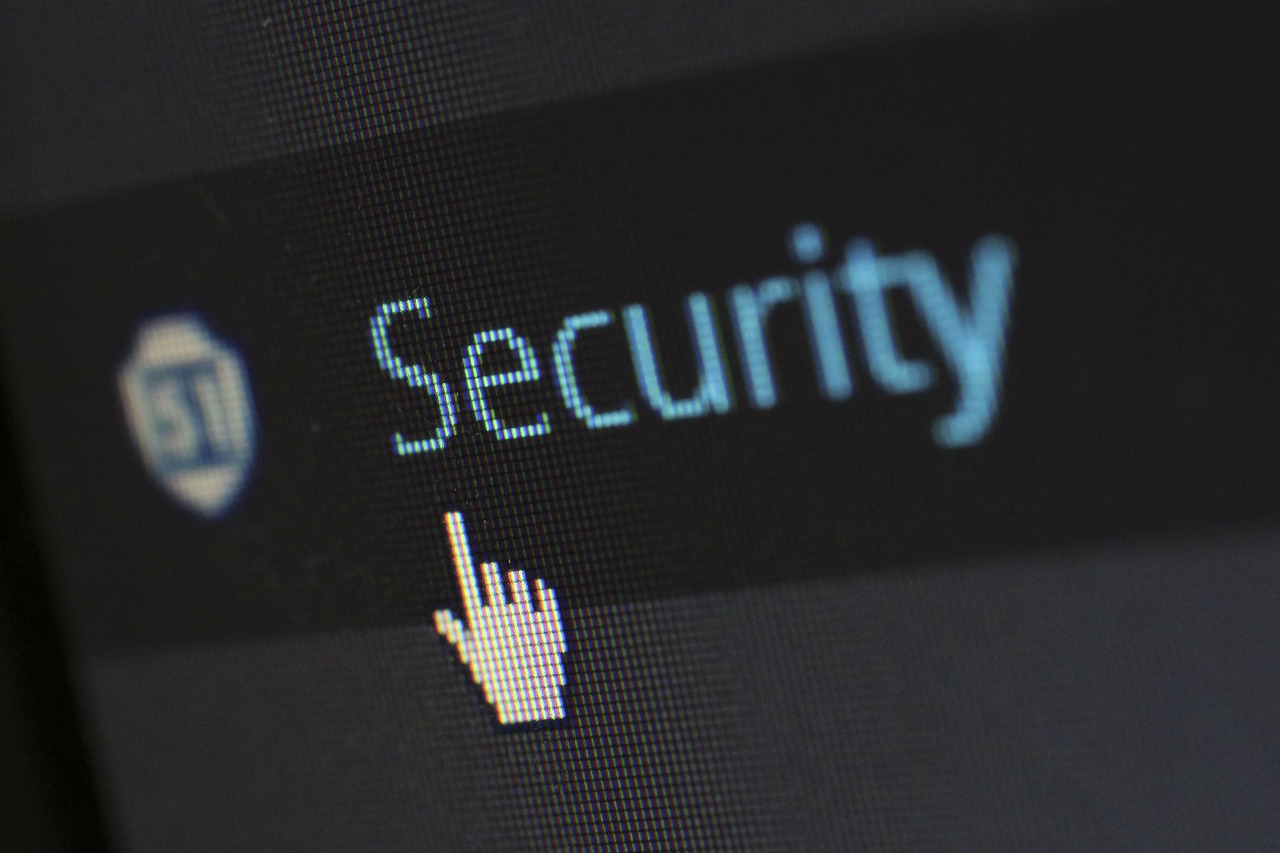
Security Training Day 1
My study notes of security training (Day 1), including web thread landscape (Java in particular), security tools, and some Juice shop training answers.
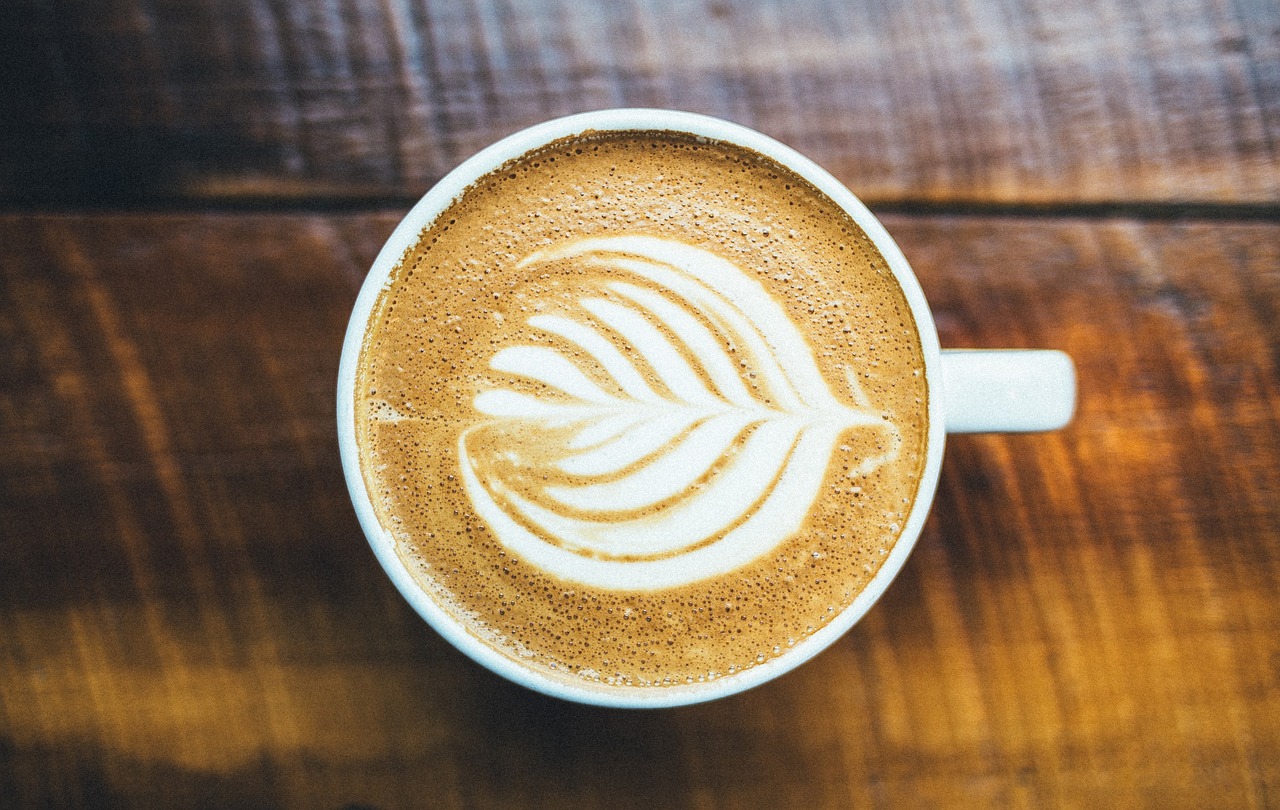
VAVR HashMap vs Java HashMap
What is the difference between VAVR Collection API and Java Collection API? Why should you give it a try? Today, we will start comparing them via Map and HashMap, including the map creation, entries iteration, streaming, and the side effect.
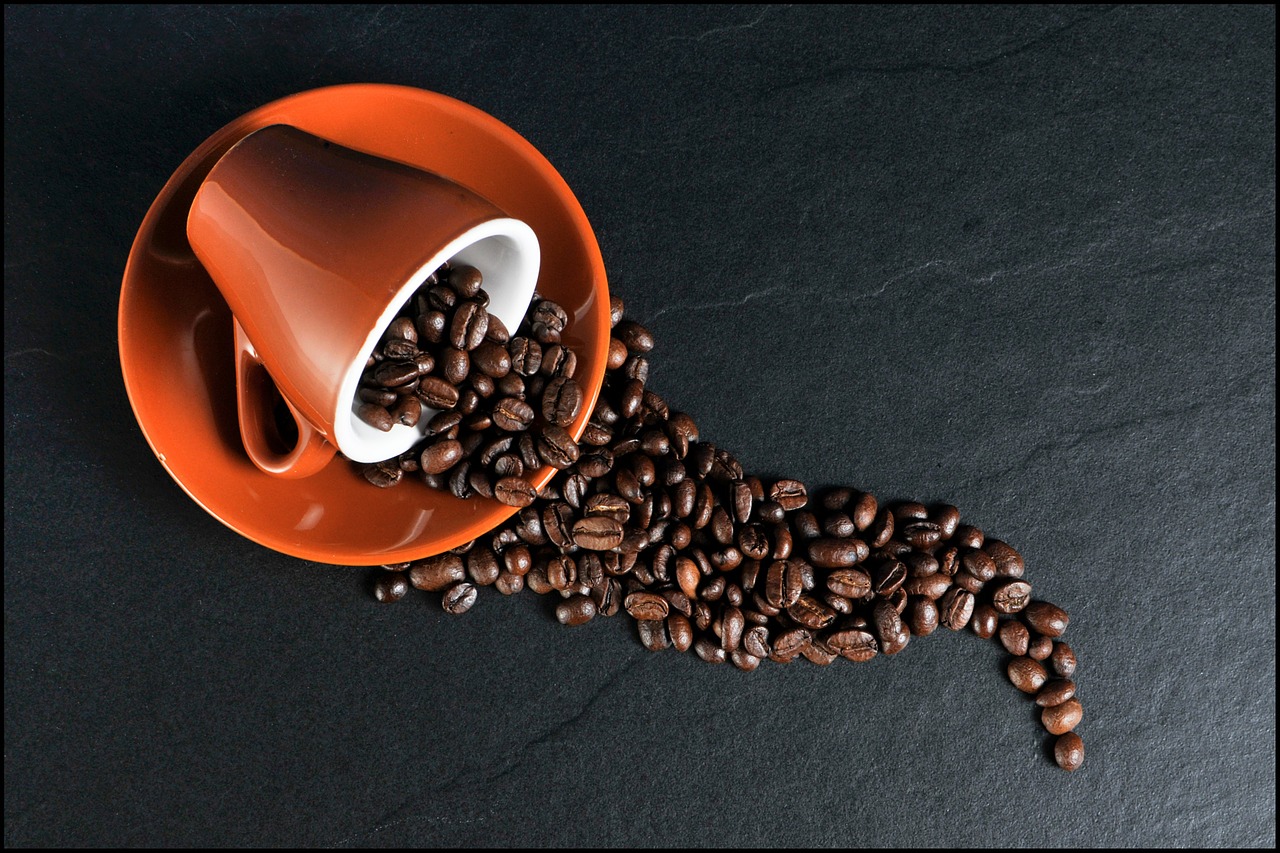
Testing JAX-RS Resources
This article explains how to set up and tear down a Grizzly Server for testing JAX-RS resources, how to create a HTTP request and assert the response using JUnit 4. And finally, the limits of testing API in reality.
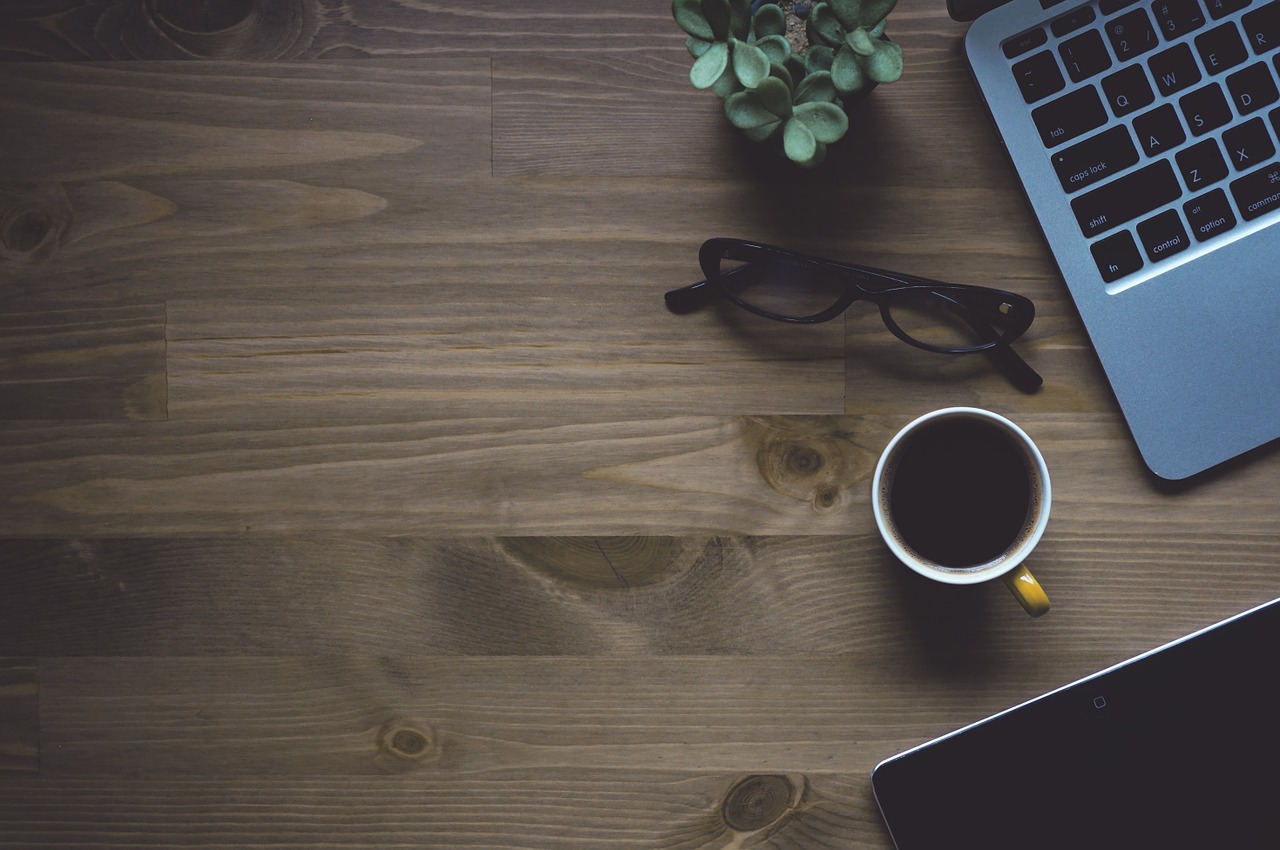
JAX-RS Client API
This post explains what is JAX-RS Client API and how to use it via Jersey Client API. We will talk about the Maven dependencies, Client, WebTarget, and HTTP response.
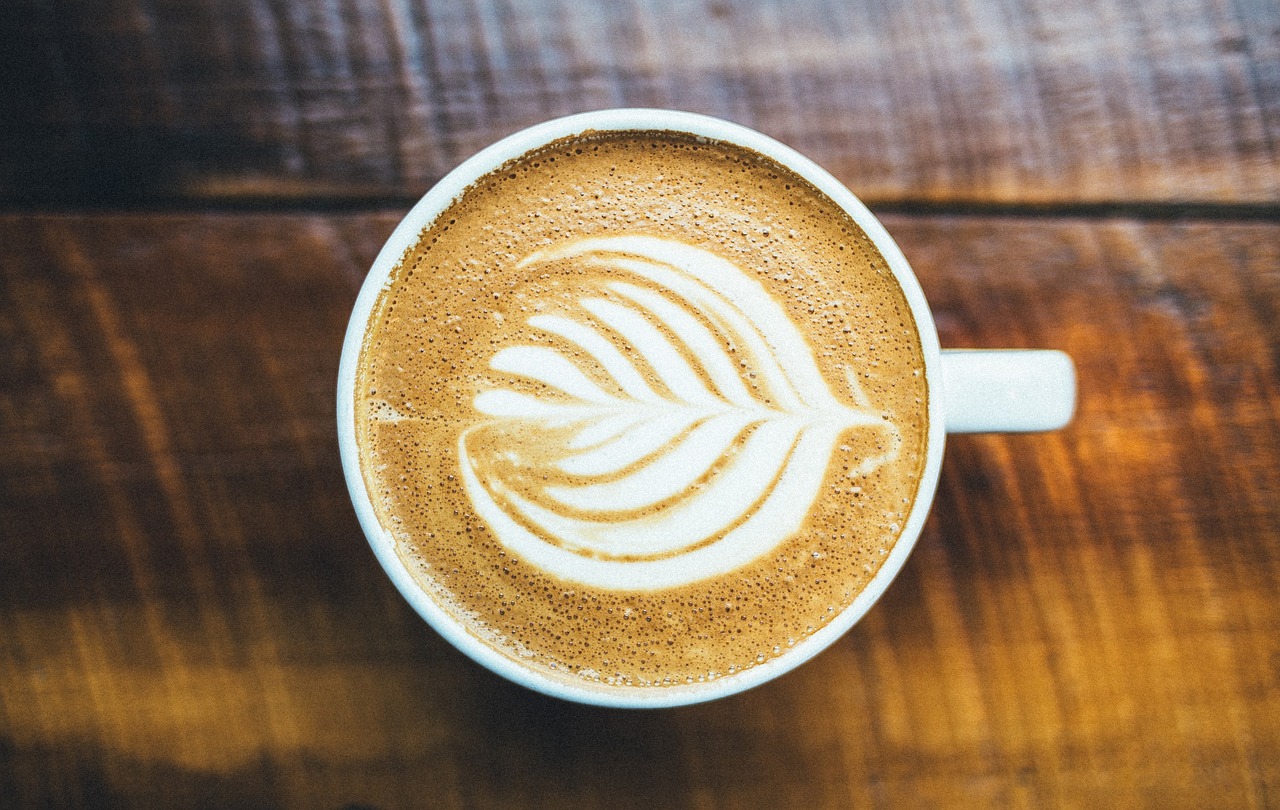
Exception Handling in JAX-RS
This post explains exception mapper, how to register it in JAX-RS application programmatically or via annotation, the exception matching mechanism (nearest-superclass), and more.
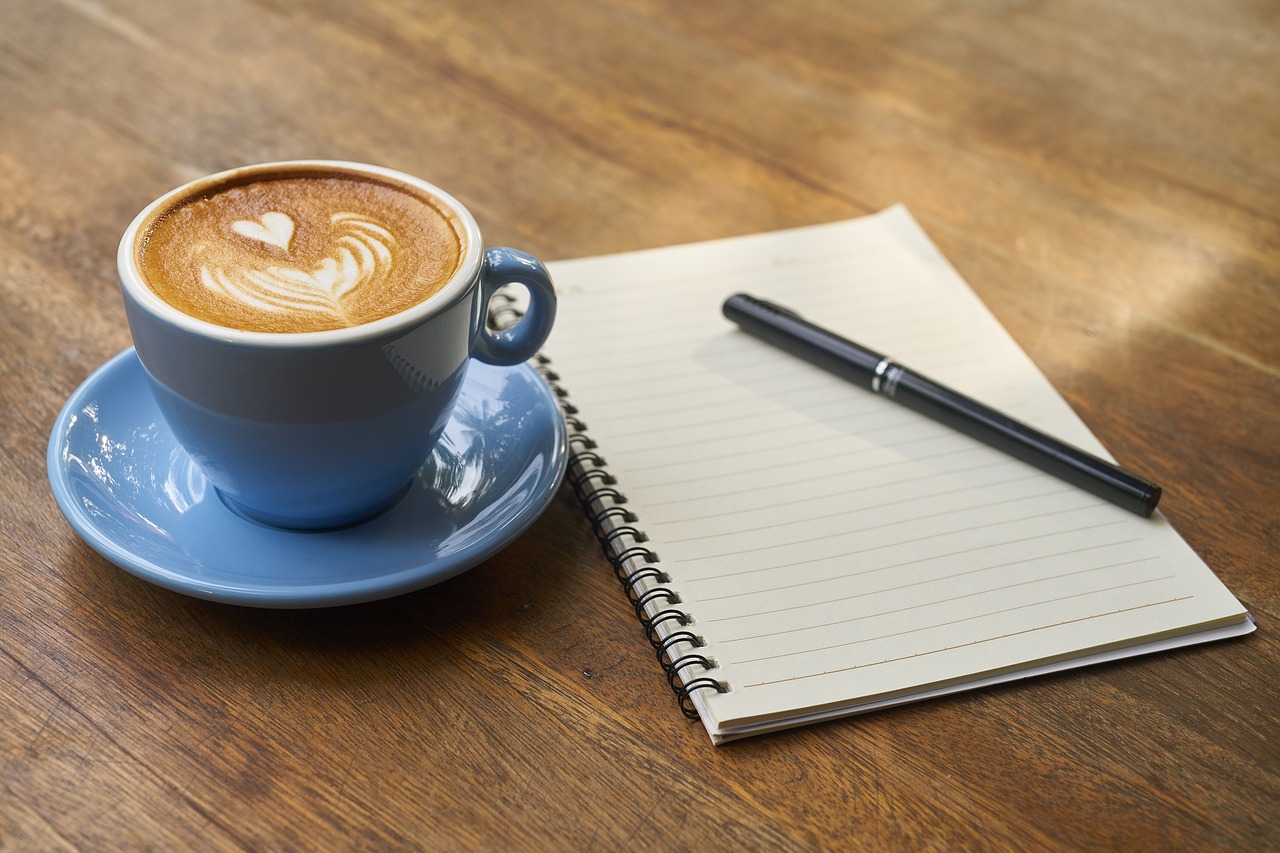
JAX-RS Param Annotations
This post explains different param annotations in JAX-RS 2.1 and their use-cases, including @QueryParam, @MatrixParam, @PathParam, @HeaderParam, @CookieParam, @FormParam and @BeanParam.
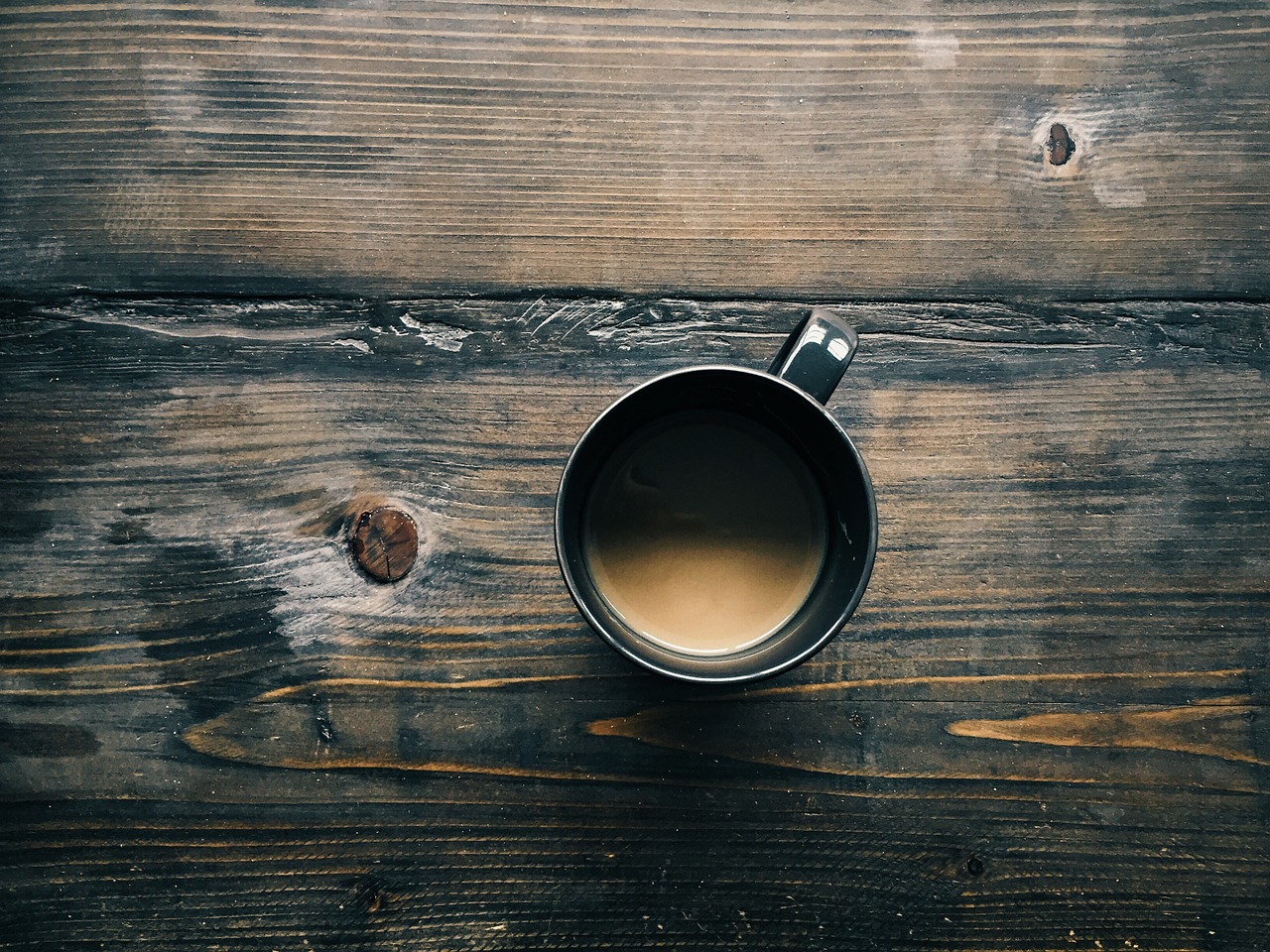
HTTP Methods in JAX-RS
This article explains the common HTTP methods in JAX-RS: annotation @GET, @POST, @PUT, and @DELETE.
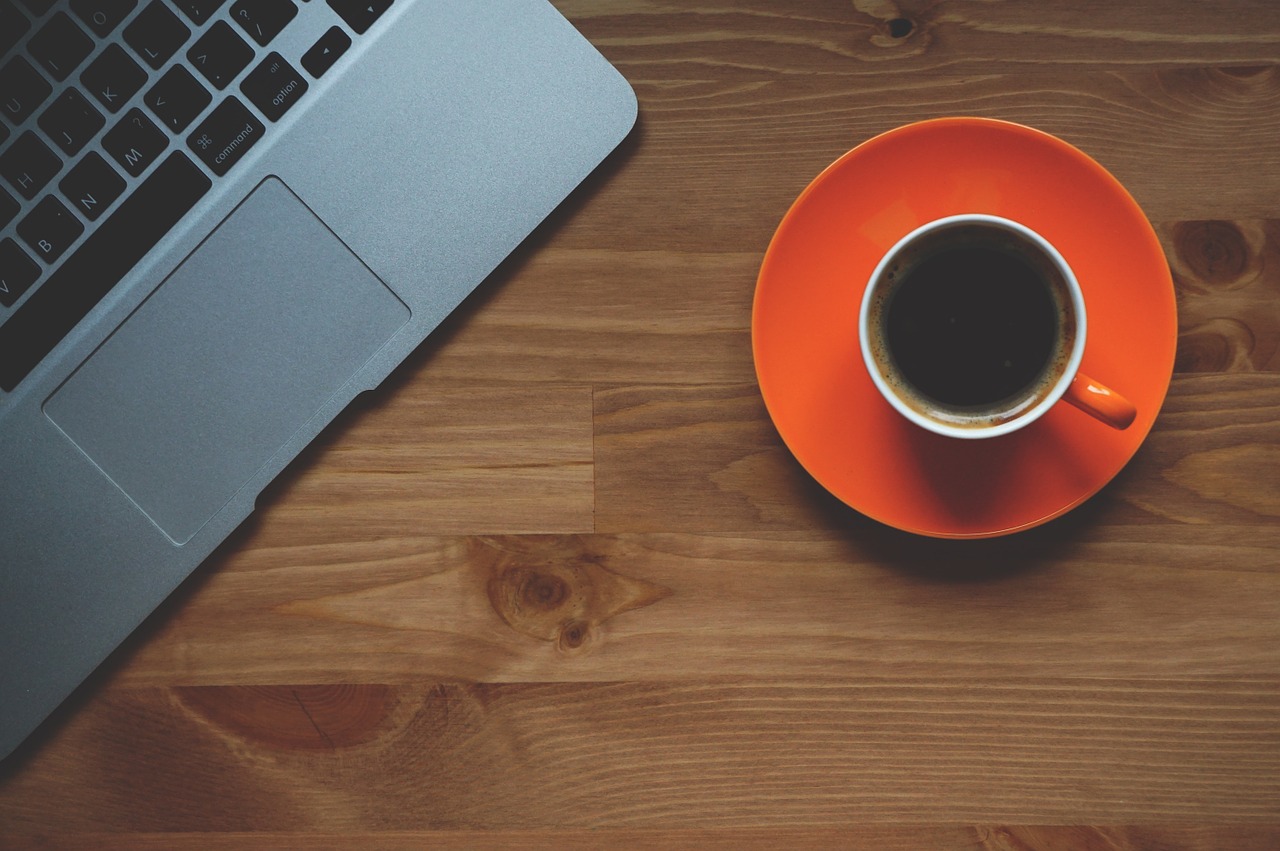
Simple REST Demo With JAX-RS
A quickstart demo for creating REST service in Java using JAX-RS 2.0. The sample is implemented by Jersey, the reference implementation of JAX-RS.
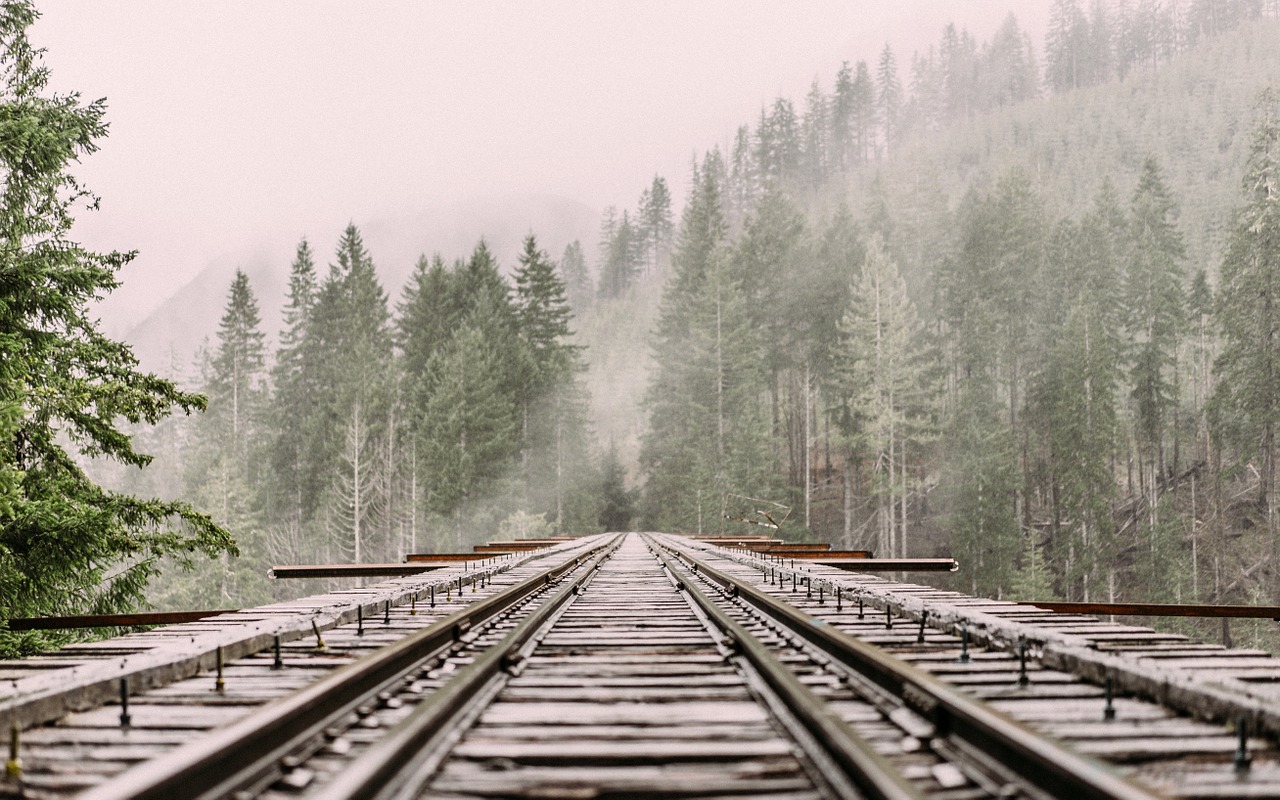
Merging Git Repositories
Engineering story: how I merge two Git repositories and what are the hidden tasks you didn't think about.
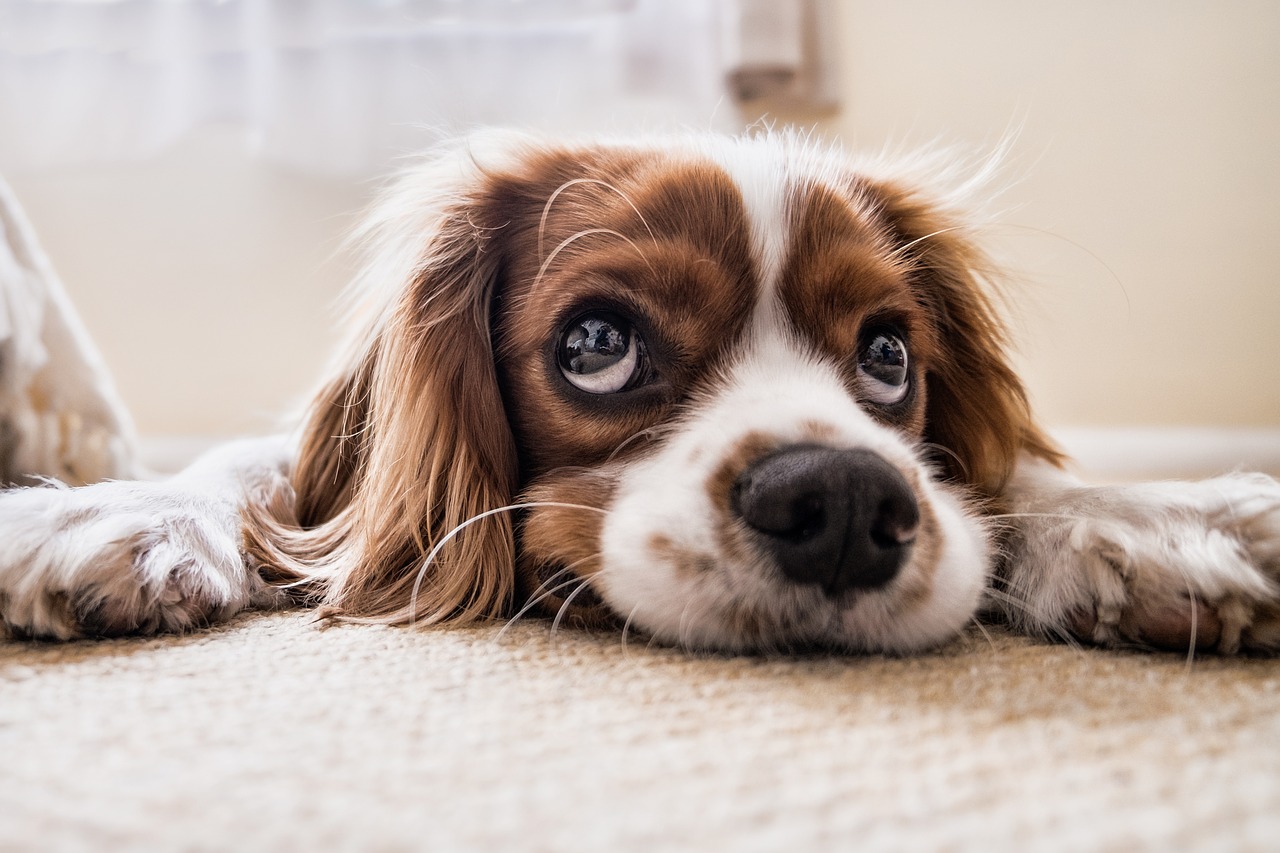
Speed Up The Maven Build
How to speed up a Maven project by using different tips.
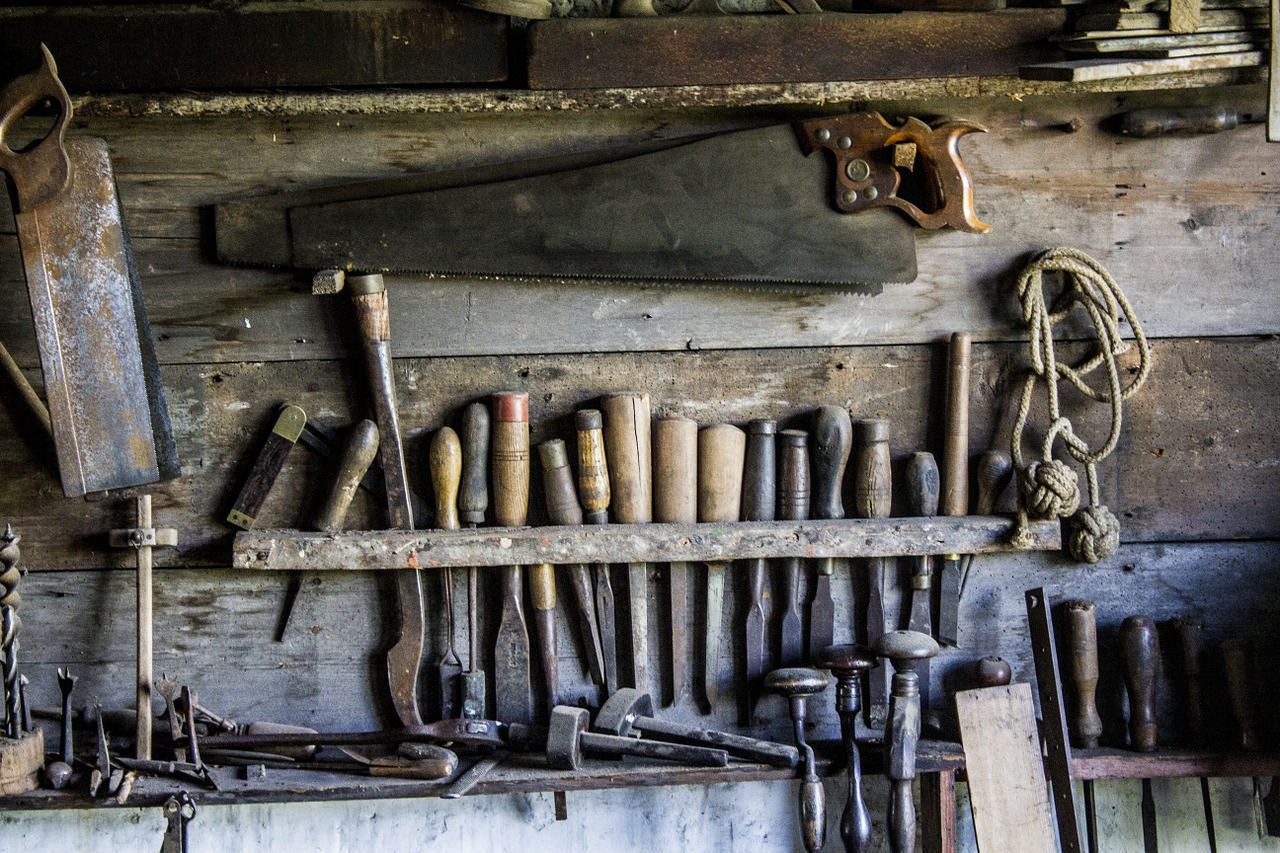
Maven Failsafe Plugin Understanding
Maven Failsafe Plugin runs integration tests for your Maven project. In this article, we will see what is Failsafe plugin and its common use cases.
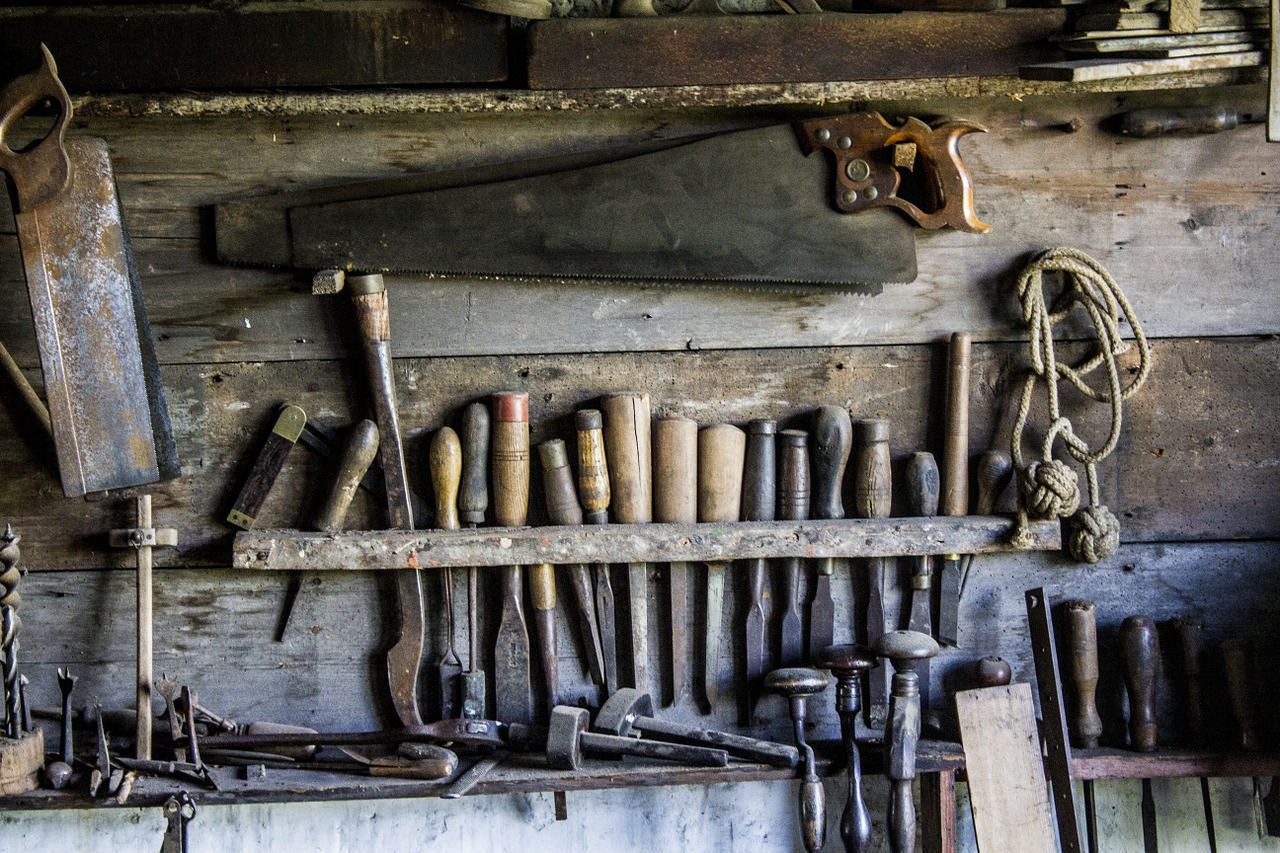
Maven Surefire Plugin Understanding
Maven Surefire Plugin is used during the "test" phase of Maven build lifecycle to execute unit tests. It can be used with JUnit, TestNG or other frameworks. This article explains what is Surefire plugin and its common use cases.
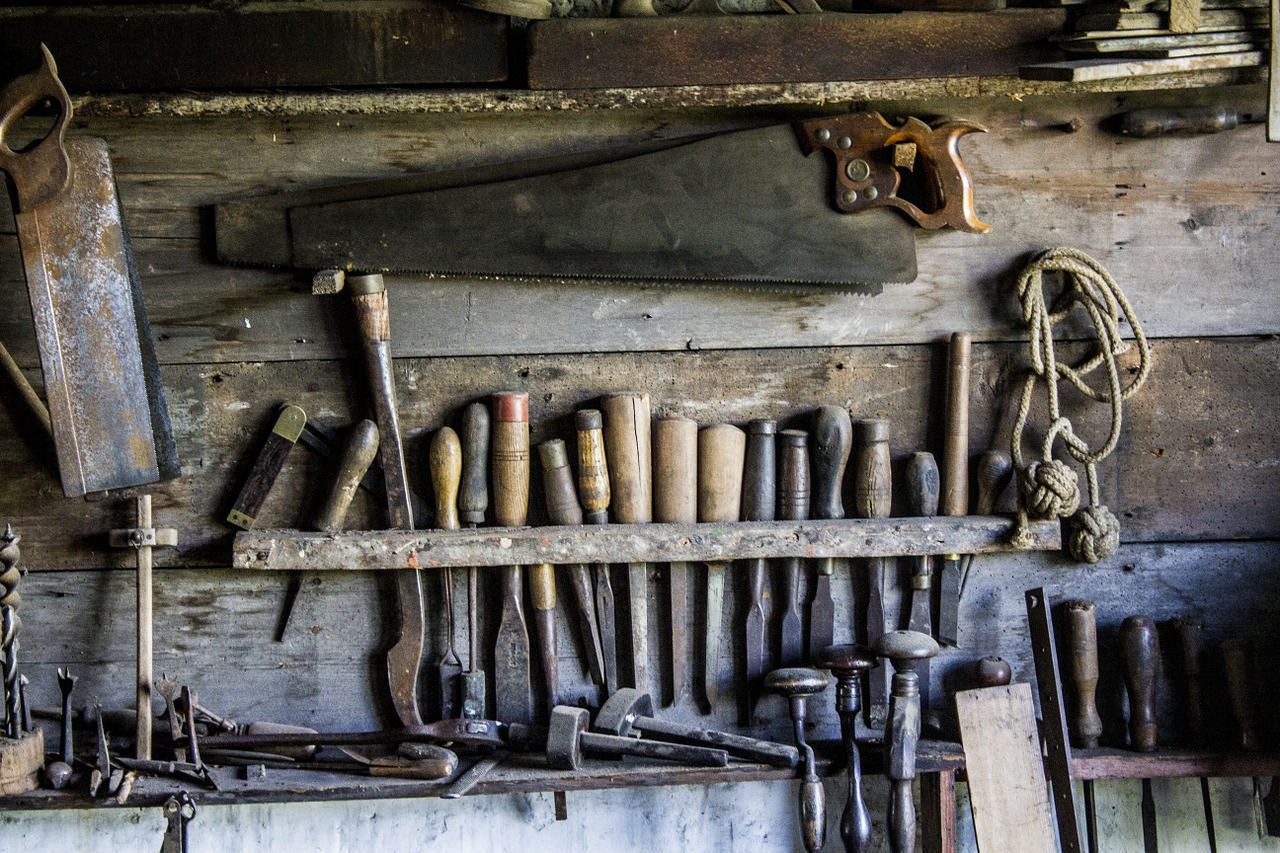
Maven Resources Plugin Understanding
Maven Resources Plugin is part of the core Maven plugins, which handles resources copying to the output directory. It has 3 goals: "resources", "testResources", and "copy-resources". In this post, I'll show you some common use-cases of this plugin.
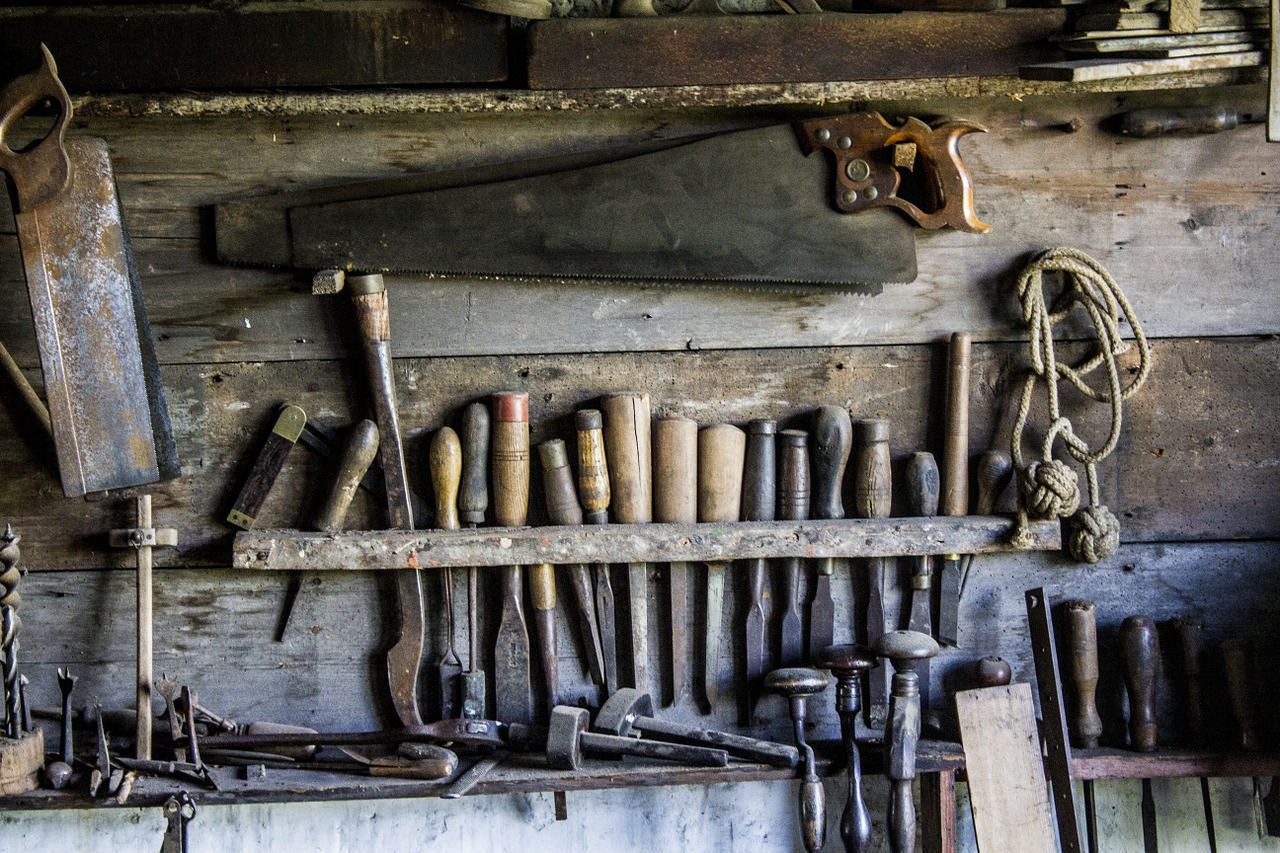
Maven JAR Plugin Understanding
A step-by-step guide for understanding Maven JAR Plugin in Java 8 and Java 11.
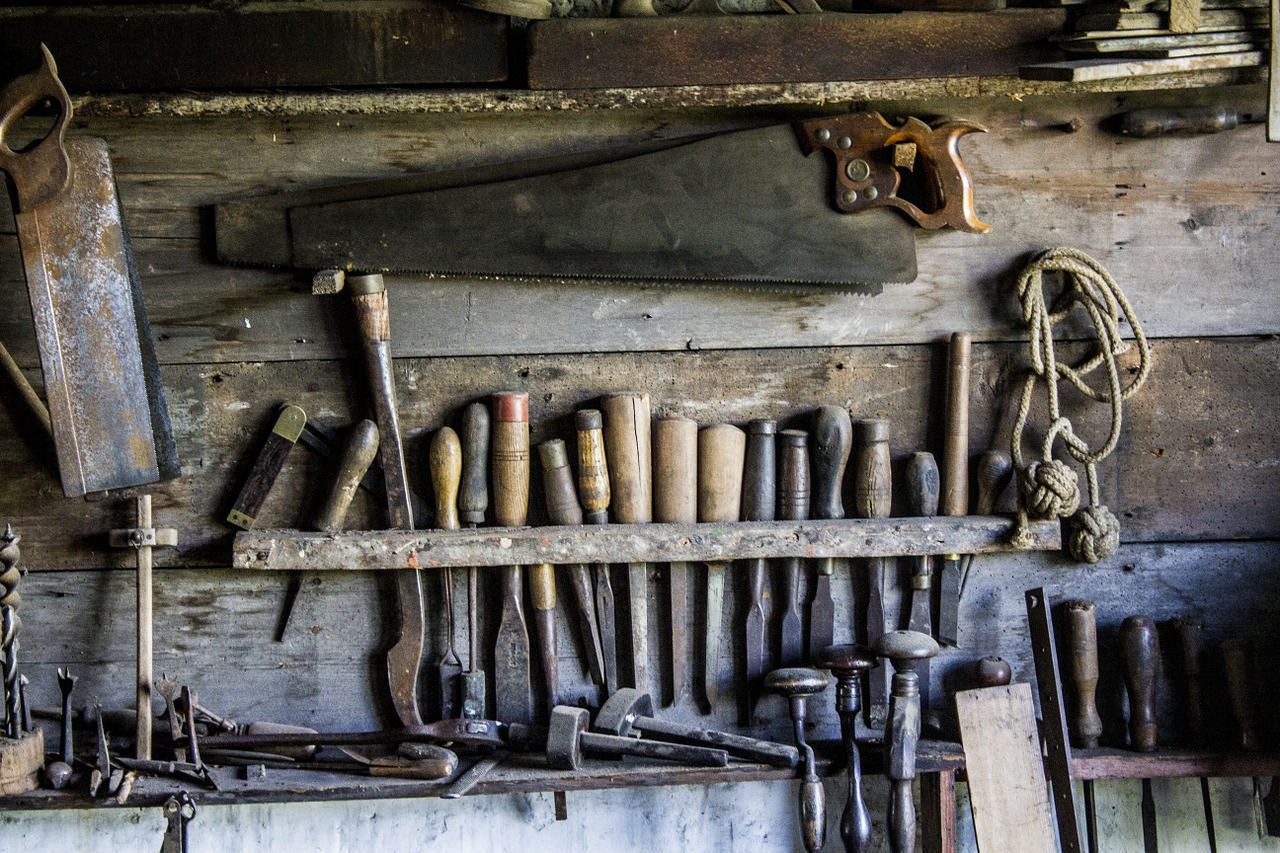
Maven Compiler Plugin Understanding
A step-by-step guide for understanding Maven Compiler Plugin in Java 11.
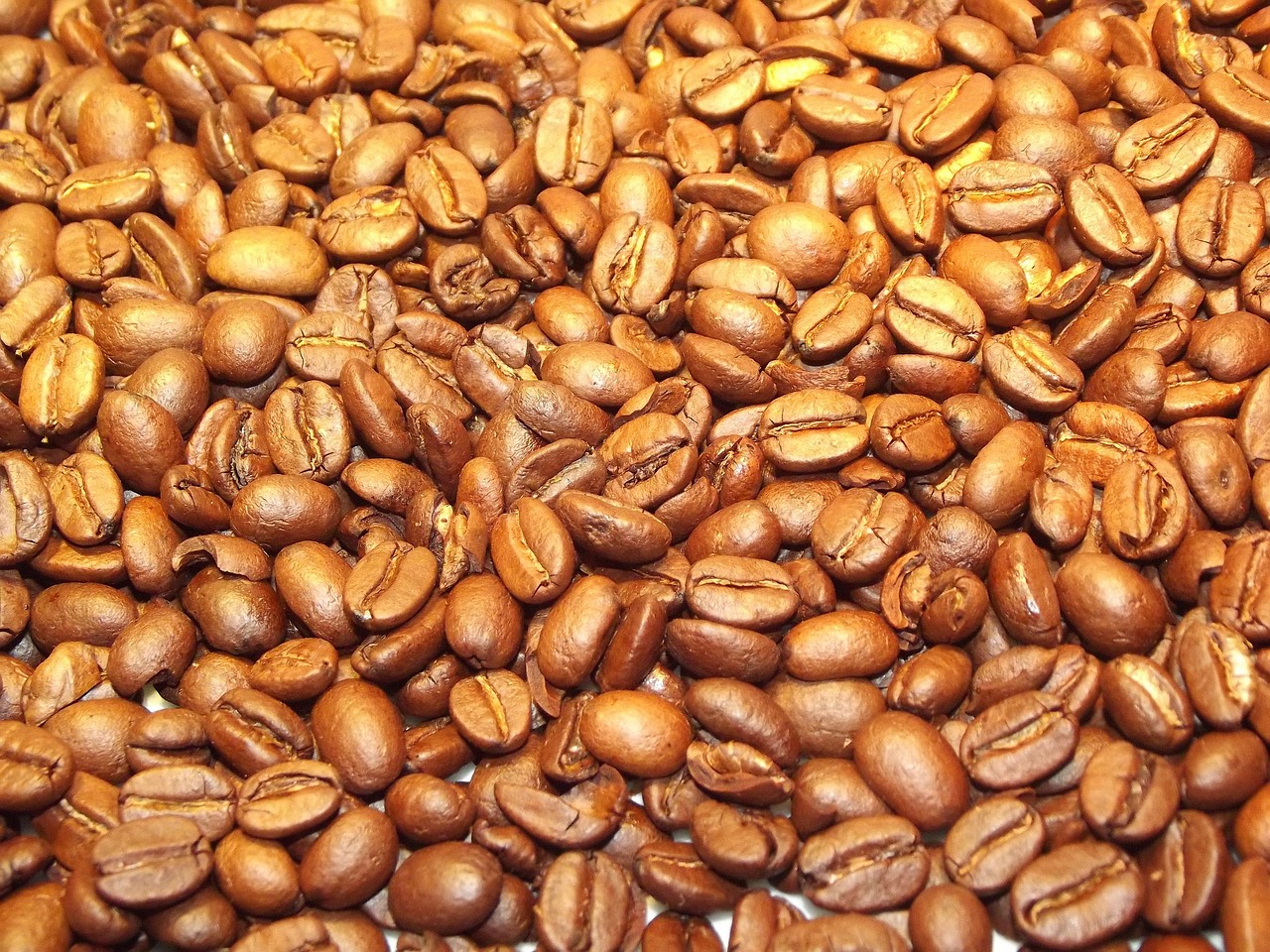
Why You Should Use Auto Value in Java?
Auto Value generates immutable value classes during Java compilation, including equals(), hashCode(), toString(). It lighten your load from writing these boilerplate source code.
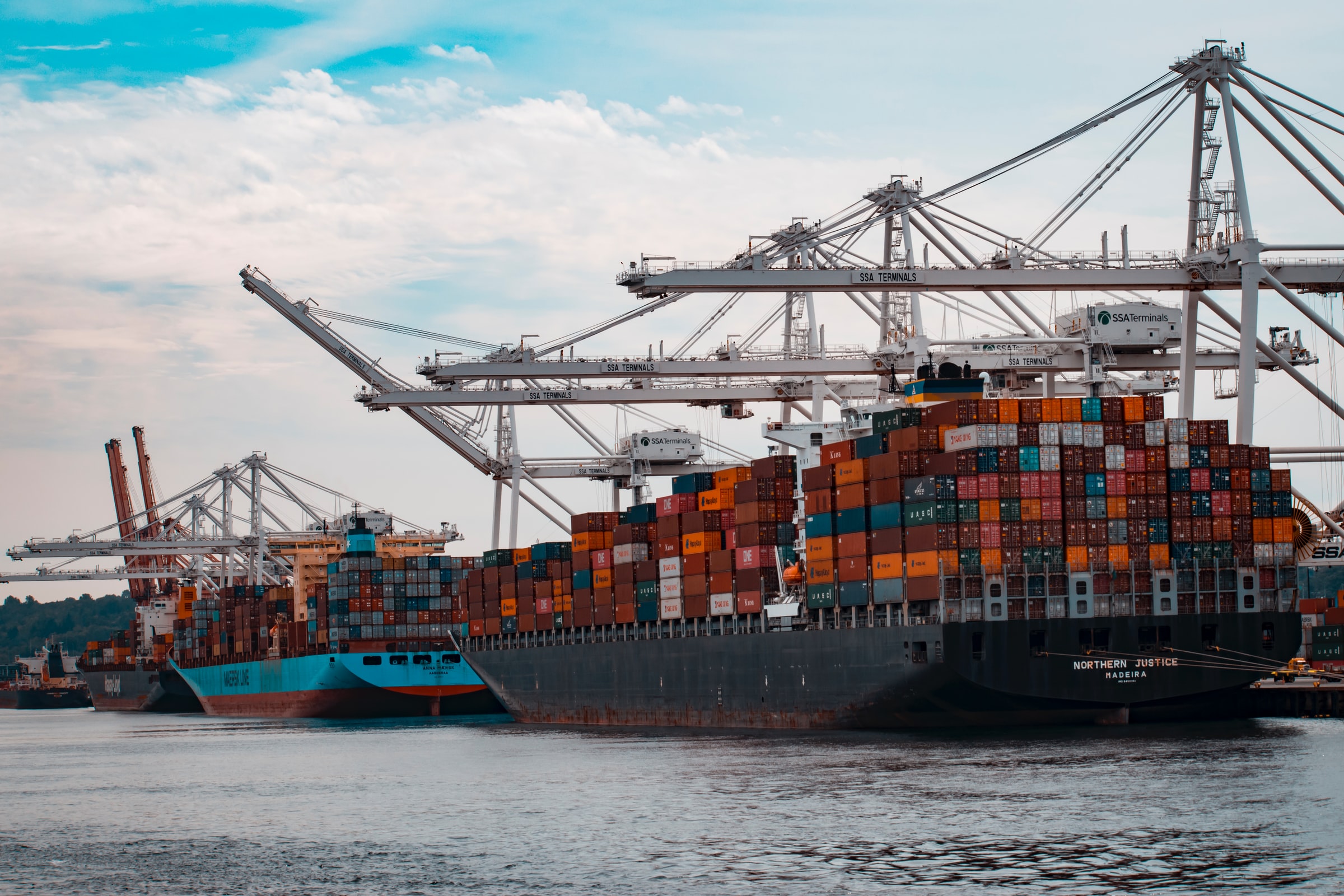
Maven: Deploy Artifacts to Nexus
Declare Maven deploy plugin in the parent POM. It's the same no matter your project is a single module project or a multi-modules project. Then, define the Nexus repository id and url in distributionManagement. After that, add your credentials in ~/.m2/settings.xml. Finally, execute command `mvn deploy` to deploy your artifacts.
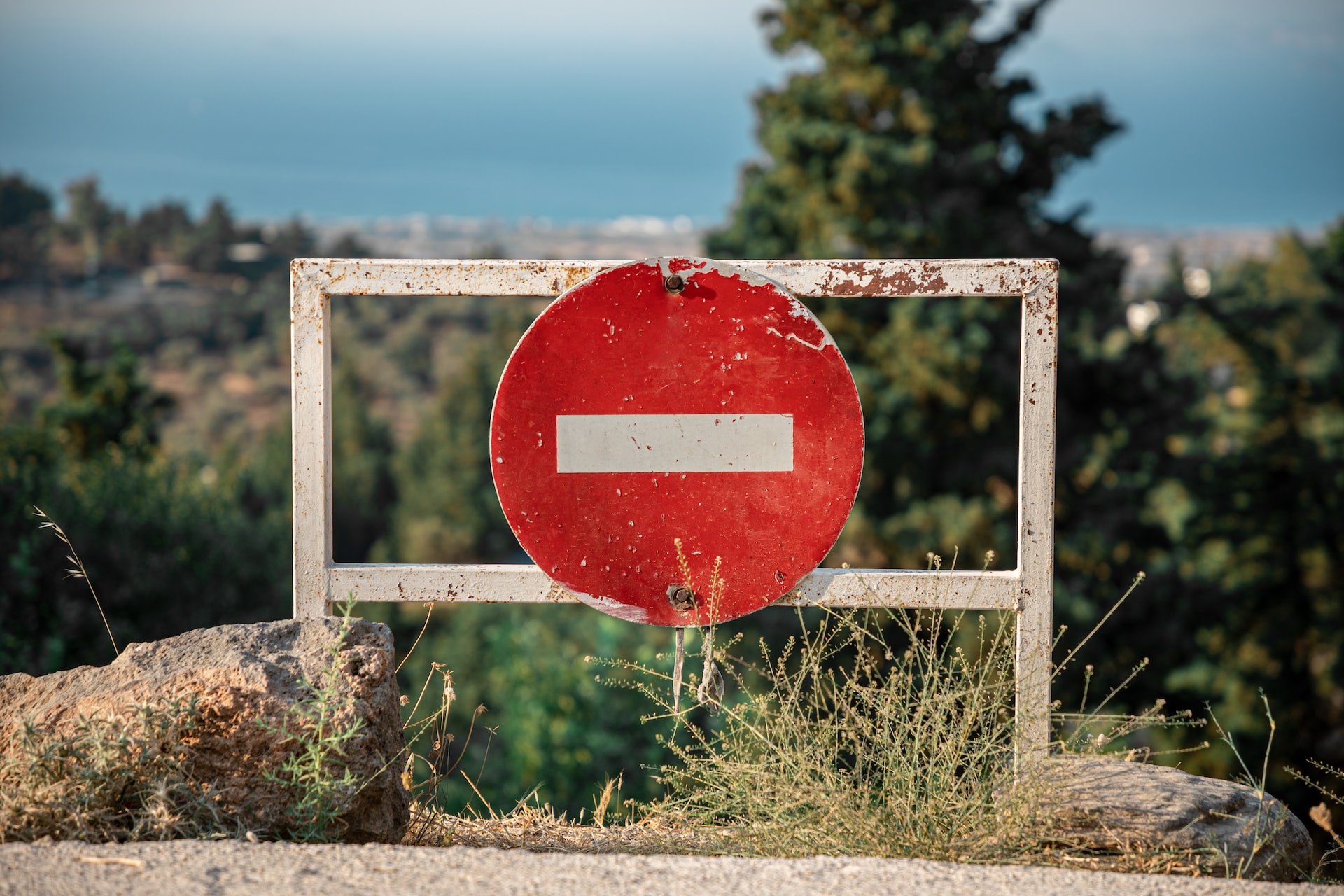
JGit: Protect Branches on Git Server
If you're using JGit for your Git server, you can combine Git config (.git/config) and a customized pre-receive hook to protect branches on a specific Git repository.
Java Server on Raspberry Pi
A step-by-step guide for installing Java server on Raspberry Pi: install Raspbian OS, install JRE, configure SSH, transfer data, and setup Java server with Systemd.
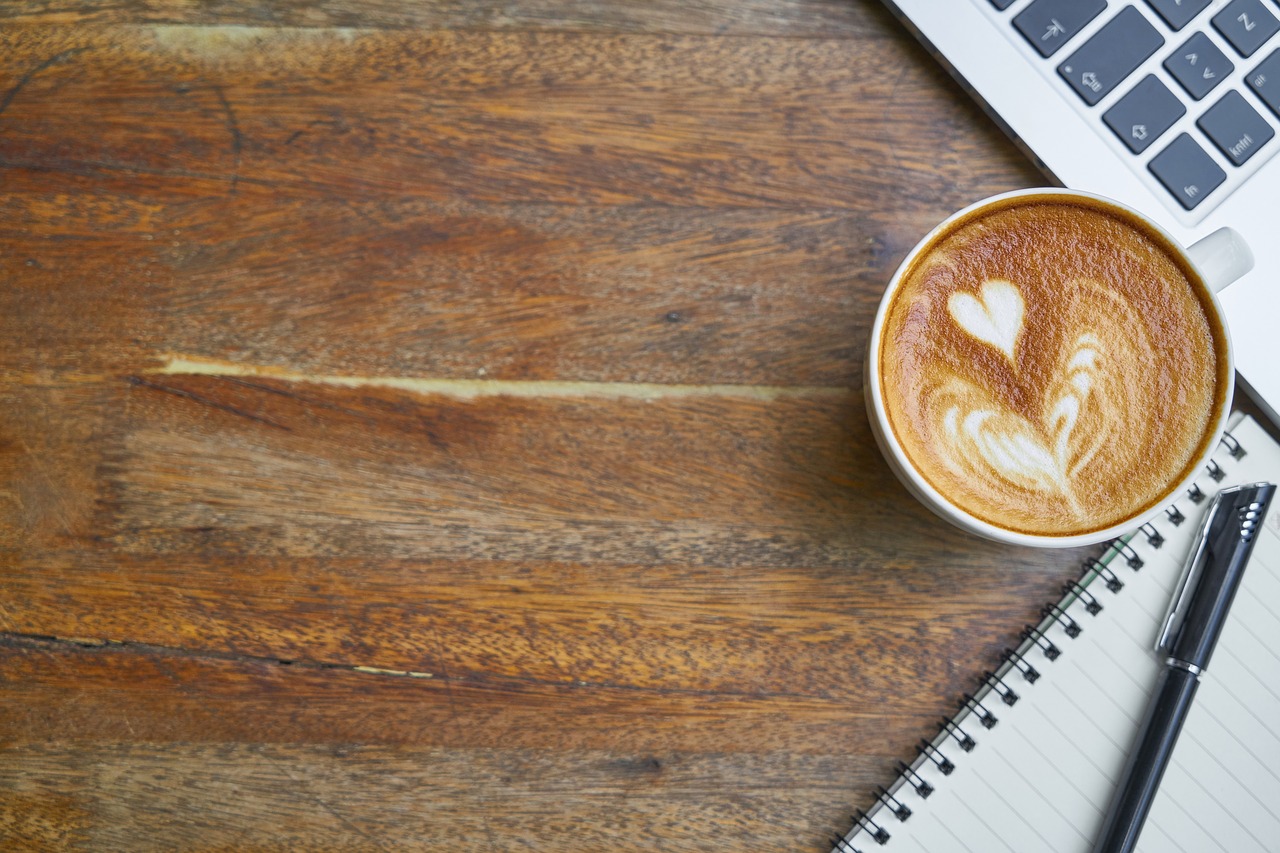
Create Systemd Unit File for Java
This post explains how to create a systemd unit file for Java, so that you can run your Java application as a service in Linux. It also explains the structure of a service file, and tells your the useful commands after service's creation.
Method Execution In Multithreading
Today I met some multithreading problems. Many Java syntax become very confusing in a multithreading environment. So I created a mini Java program, and wrote this study note to clarify the unclear methods. This blog post will go through the following steps:
- Source code and execution
- Understand
Thread#join()
- Understand logic sharing
- Understand variable sharing
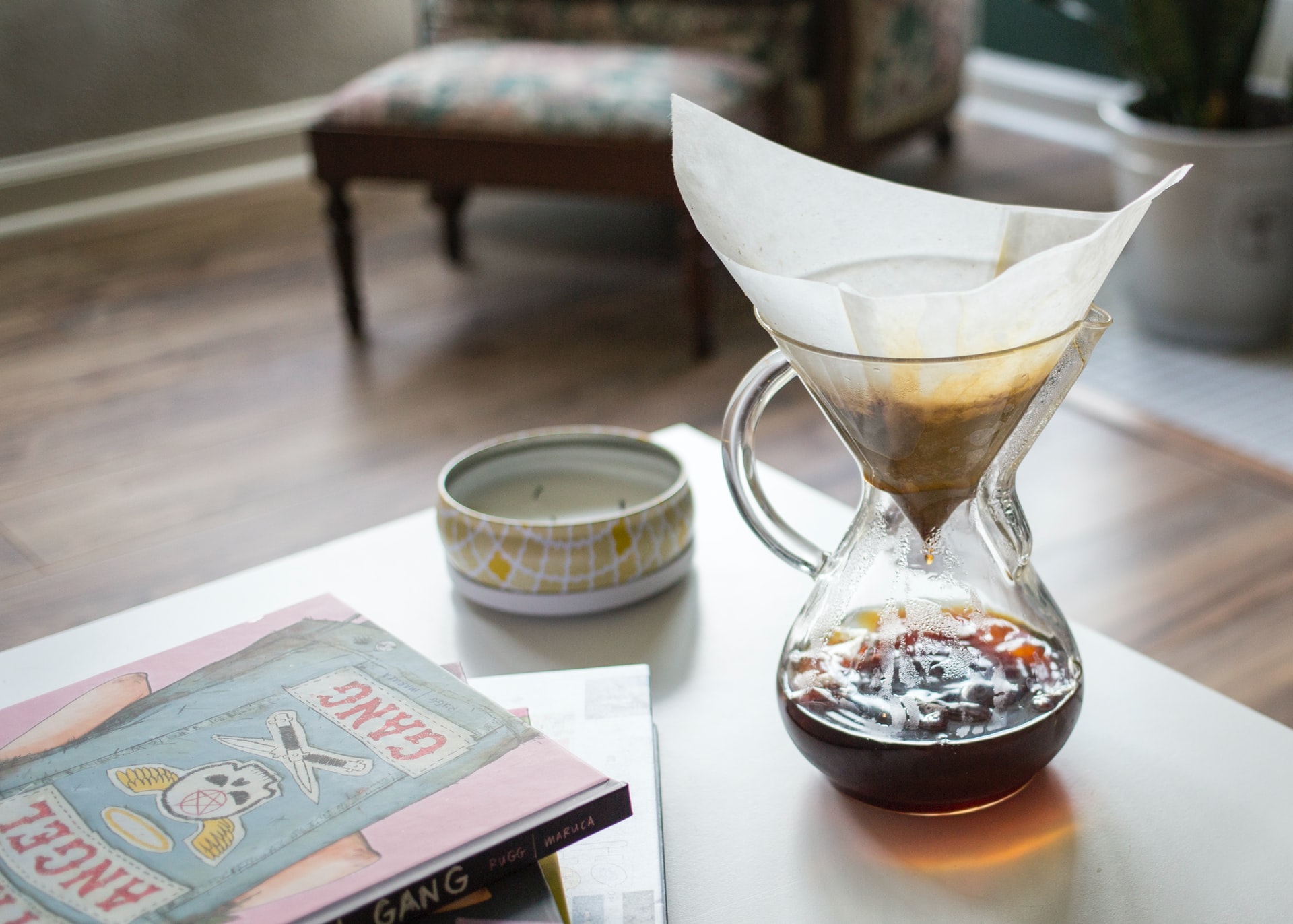
JGit: Customize Git references using RefFilter
This post explains how to apply a Git reference filter to your Git server in Java. It allows you to customize Git references before sending data to clients (upload-pack).
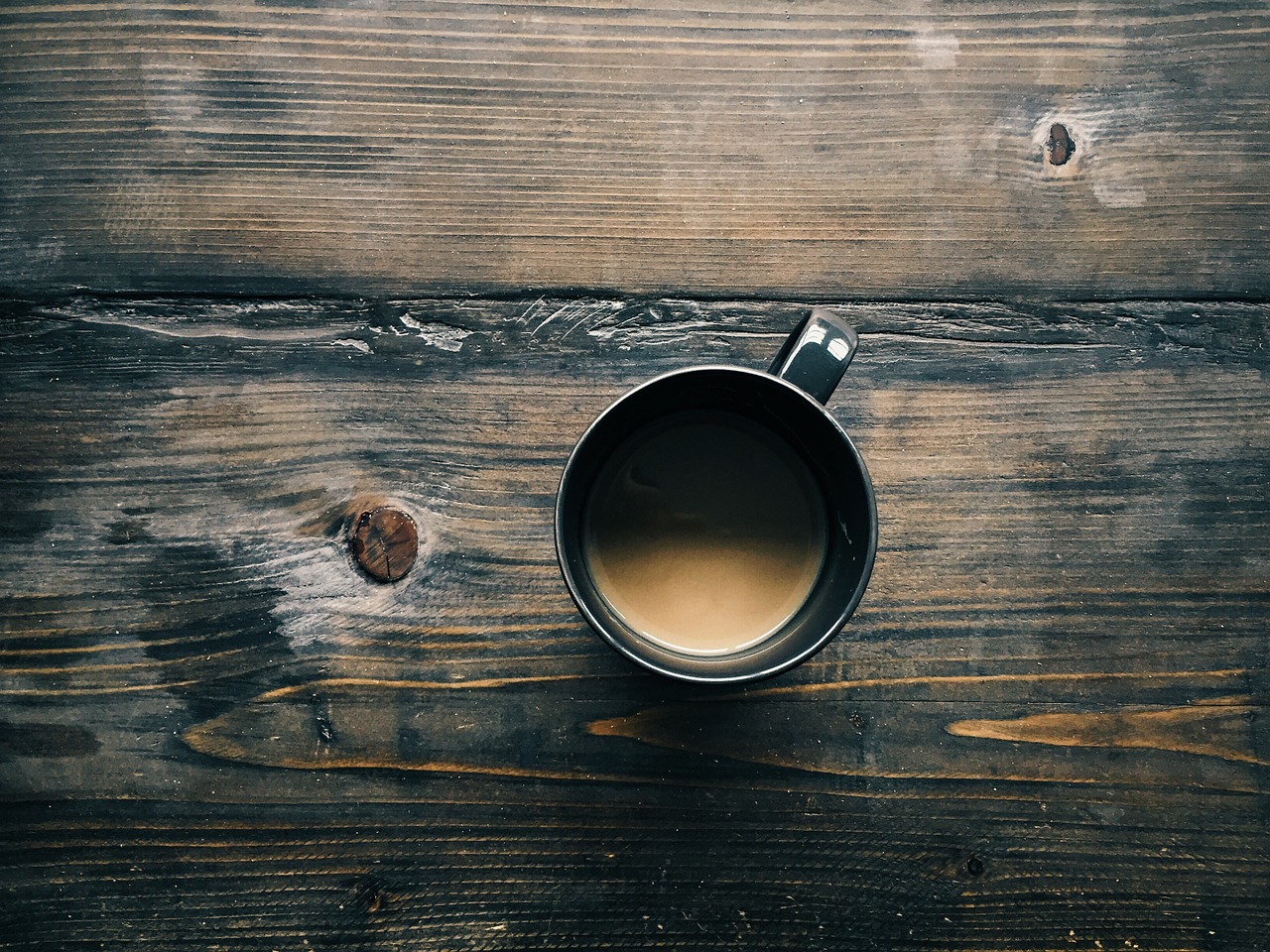
Use Auto Value and Jackson in REST API
This post explains what is Google Auto Value and why it is the best alternative for Data Transfer Objects (DTO) in your web service. It shares how to apply Jackson annotations on top of Auto Value classes. There're also some advanced configurations about this topic.
My Java Code Style
My Java code style: a collection of Java good practices.
Servlet and Filter
Today, I’d like to talk about the famous HttpServlet
and Filter
, in
Tomcat 8.5 server.
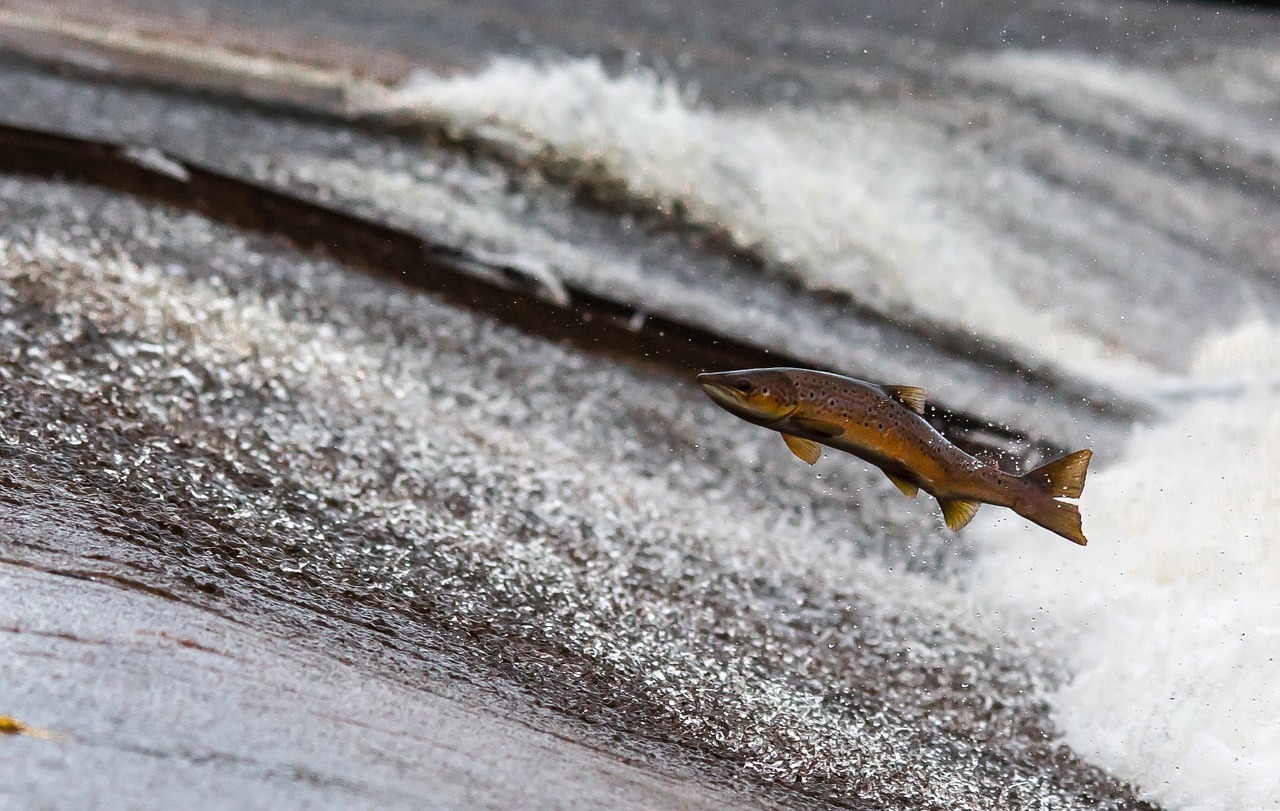
Git: Upstream Tracking Understanding
A quick introduction about Git upstream tracking: set upstream with git-push or git-branch, the internal mechanism inside Git config (.git/config), unset upstream, and related implementation in Java (JGit).
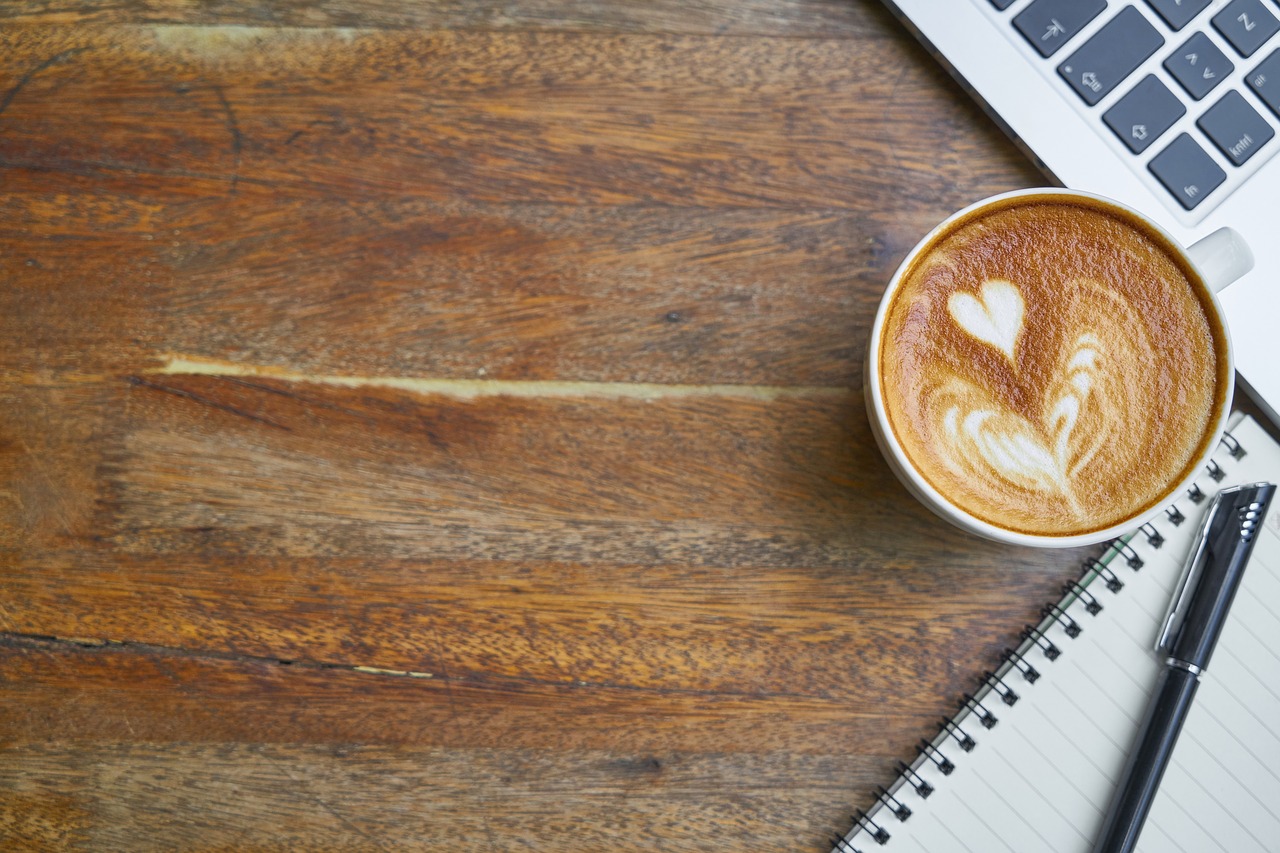
Learning HashMap
Understand java.util.HashMap in Java 11: bitwise operations, hash computation using hash code, index calculation, and more.
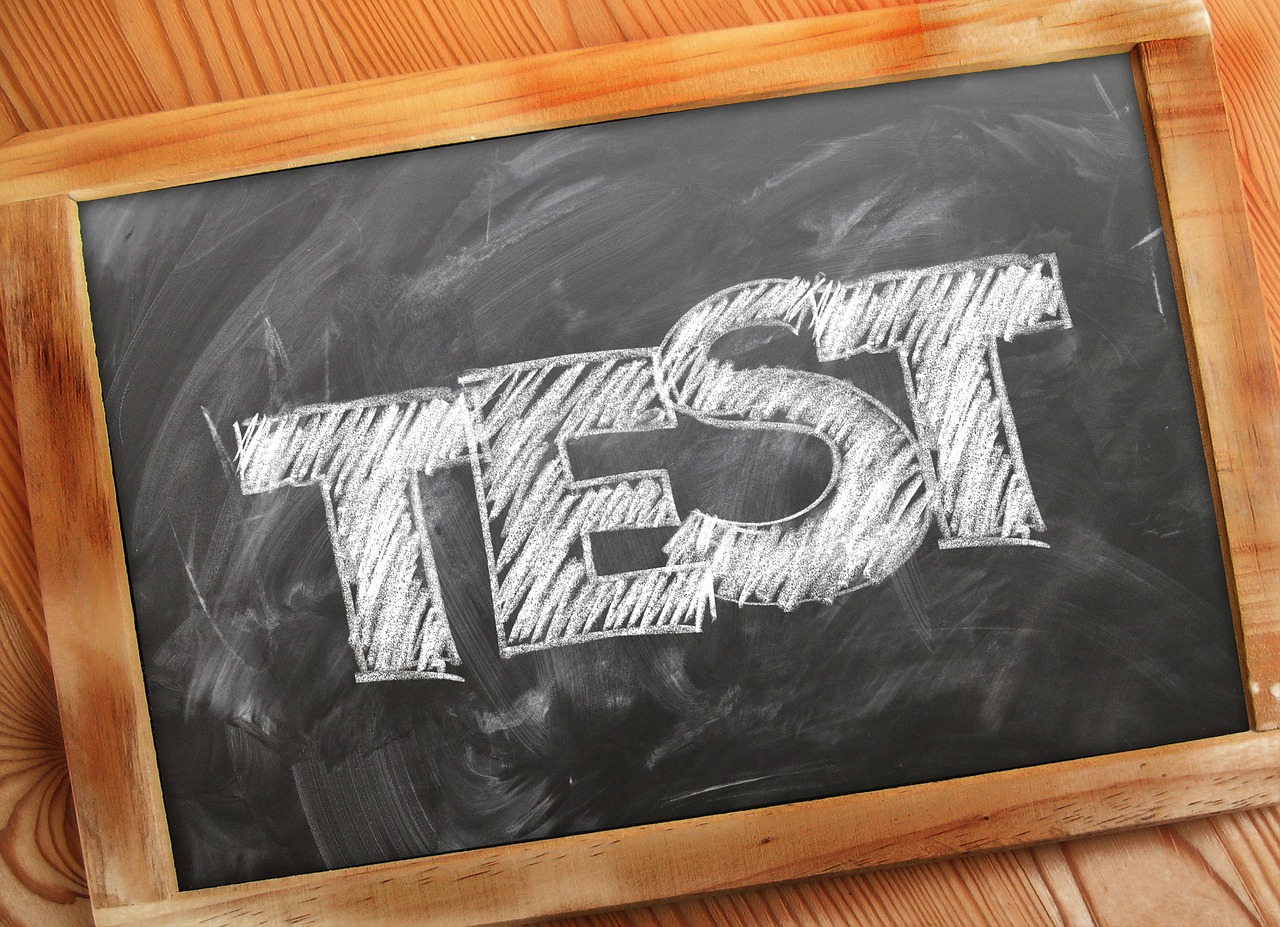
Introduction to Selenium WebDriver
A quick introduction to Selenium WebDriver, a practical tool for running functional tests and browser automation. The sample is written with Firefox 58 and GeckoDriver 0.20.
GWT SafeCSS Internal
Recently, I helped the GWT community for porting module gwt-safecss to GitHub. I think it’s also a good opportunity to learn more about GWT SafeCss. That’s why I’m writing this study note.
JGit Internal: Reference and RevObject
Today, I want to discuss Git internal mechanism with you: How Git resolves references? How JGit, a pure Java implementation of Git, resolves references in Java? Then, how to use them via class RevObject and its subtypes.
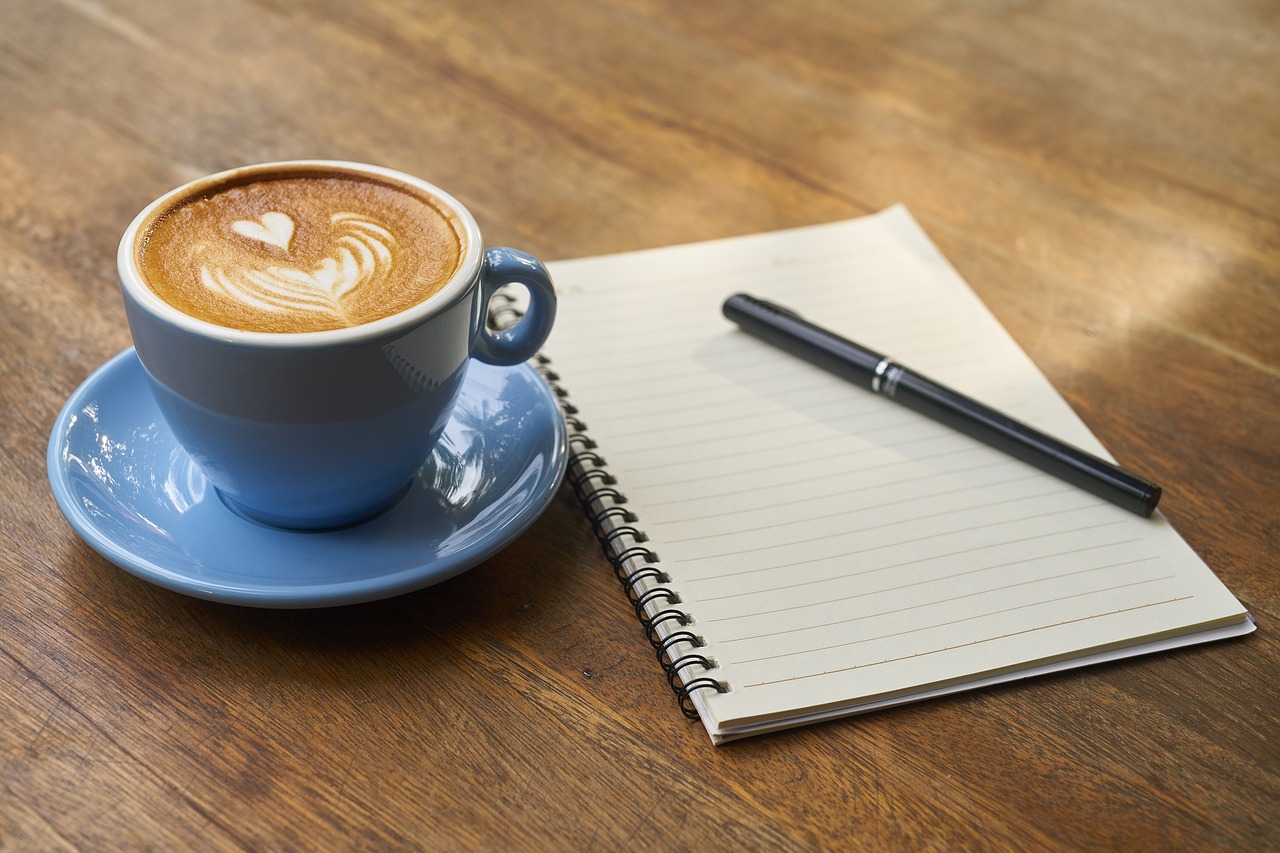
Java 9 Migration
Java 9 migration for Maven project. It consists Java 9 installation, IDE update, Maven project update, CI update, and fixing tests. This article is written using macOS and IntelliJ IDEA.
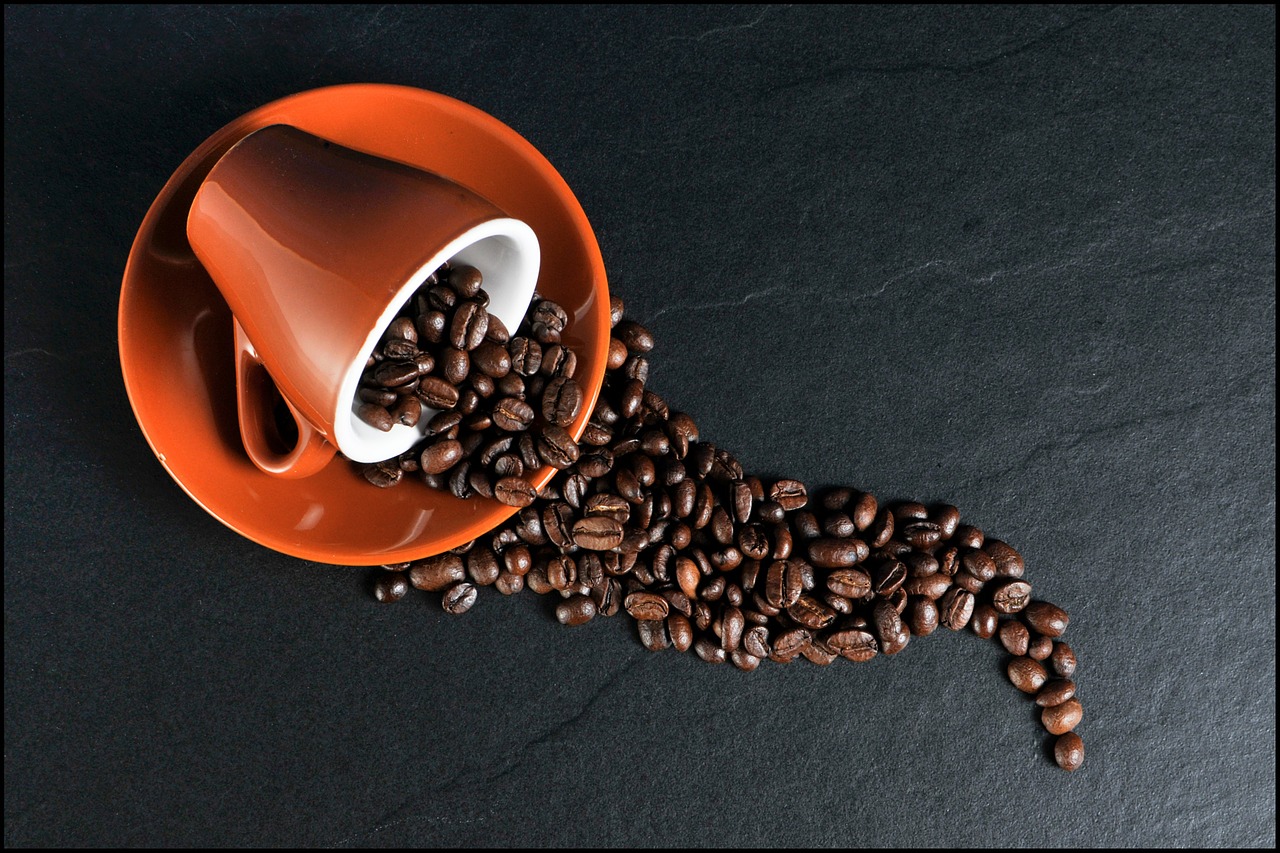
Learning Checkstyle
Checkstyle is a development tool to help programmers write Java code that adheres to a coding standard. By default it supports the Google Java Style Guide and Sun Code Conventions, but is highly configurable. It can be invoked with an ANT task and a command line program.
Learning JNI
Today I would like share my very first experience about JNI—Java Native Interface. The Java Native Interface (JNI) is a programming framework that enables Java code running in a Java Virtual Machine (JVM) to call and be called by native applications and libraries written in other languages such as C, C++ and assembly. This post uses a Hello-world example to demonstrate the communication between Java and C on Mac OS X.
Learning GWT with Maven
Today, I want to share how to learn GWT 2.8 with Maven GWT Plugin. I wrote this post because the official GWT tutorial has some inconvenience: source code and resources are stored as GWT standard structure, commands must be launched from Apache Ant, JARs and classpath must be handled explicitly etc. I found it more comfortable to start with Maven, the tool which many Java developers are familiar with.
OCP Java 8 Review Notes
Here're some review notes before my Oracle Certified Professional Java SE 8 exam. They're highly inspired by the following books: OCP Java SE 7 Certification Guide and Java 8 in Action. They are excellent resources for learning Java, which I highly recommend.
Sonar Integration with Maven
A concret Sonar integration example for your Java project, using Maven + JaCoCo + SonarCloud + Jenkins.
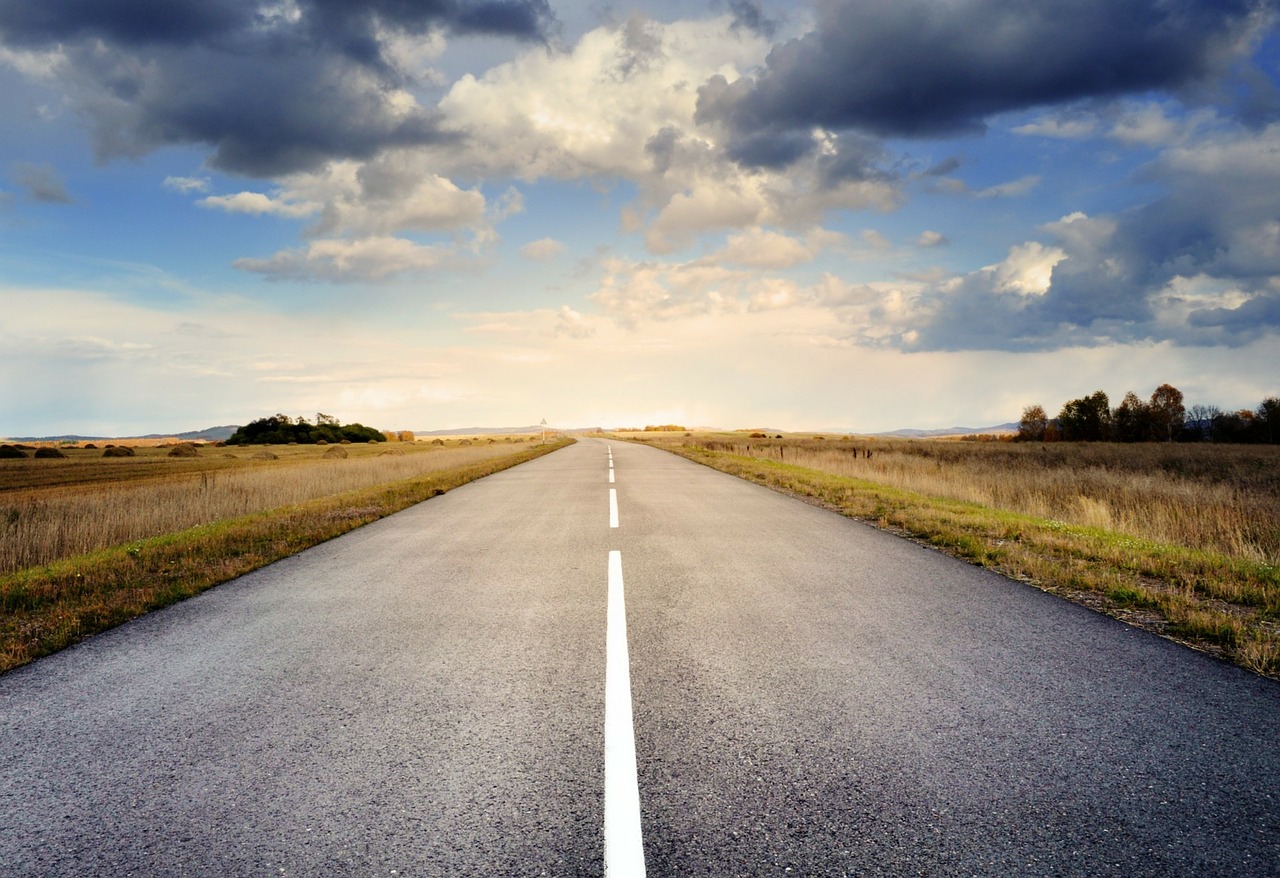
Highlight 2017
Today, I'd like to share with you the review of year 2017.
Introduction to Threads
Today, I want to share with you an introduction to multithreading in Java. In order to make the blog post more fun, I'll use the roles of "Game of Thrones" in my examples: white walkers and the Night King. After reading the blog post, you'll understand: How to create and run a thread, use wait(), notify(), notifyAll() and join().
Test a POM-Packaging Maven Module
Recently, I need to write tests for resources inside a Maven module and I met
some technical issues. The context of the situation is that I need to test some
resources located in a Maven module, where its packaging value is pom
. I
think it’s worth to take some notes, so I wrote them down and share with you.
How to Use Safe HTML in GWT
Today, I would like to share with you about how to secure your GWT application
by using package com.google.gwt.safehtml
. After reading this post, you’ll
understand how to:
- Secure HTML using
SafeHtml
- Secure URI using
SafeUri
- Secure CSS using
SafeStyles
OCA Review 8 - Notes
Today, I want to write blog post to summarize all the important review notes for Oracle Certification Associate (OCA) - Java SE 8 Programmer. This article will be separated into several subjects: Java basics, Java data types, methods and encapsulation, core APIs, flow control, inheritance, and exception handling.
OCA Review 7 - Online Test Chapter 5
Today, I’m going to review my online test, chapter 5 of Oracle Certified Associate (OCA), provided by SYBEX. If you need to access to this online resources, you need to buy their book first. See https://sybextestbanks.wiley.com/.
OCA Review 6 - Online Test Chapter 4
Today, I’m going to review my online test, chapter 4 of Oracle Certified Associate (OCA), provided by SYBEX. If you need to access to this online resources, you need to buy their book first. See https://sybextestbanks.wiley.com/.
OCA Review 5 - Online Test Chapter 3
Today, I’m going to review my online test, chapter 3 of Oracle Certified Associate (OCA), provided by SYBEX. If you need to access to this online resources, you need to buy their book first. See https://sybextestbanks.wiley.com/.
OCA Review 4 - Online Test Chapter 2
Today, I’m going to review my online test, chapter 2 of Oracle Certified Associate (OCA), provided by SYBEX. If you need to access to this online resources, you need to buy their book first. See https://sybextestbanks.wiley.com/.
OCA Review 3 - Online Test Chapter 1
Today, I’m going to review my online test, chapter 1 of Oracle Certified Associate (OCA), provided by SYBEX. If you need to access to this online resources, you need to buy their book first. See https://sybextestbanks.wiley.com/.
OCA Review 2 - Java Core APIs
I’m preparing the OCAJP: Oracle Certified Associate Java SE 8 Programmer. Here’s
the second review of the certification training. In this review, I’ll talk about
the Java Core APIs, including operations of String
, StringBuiler
, Arrays,
ArrayList
, and Java Time in Java 8.
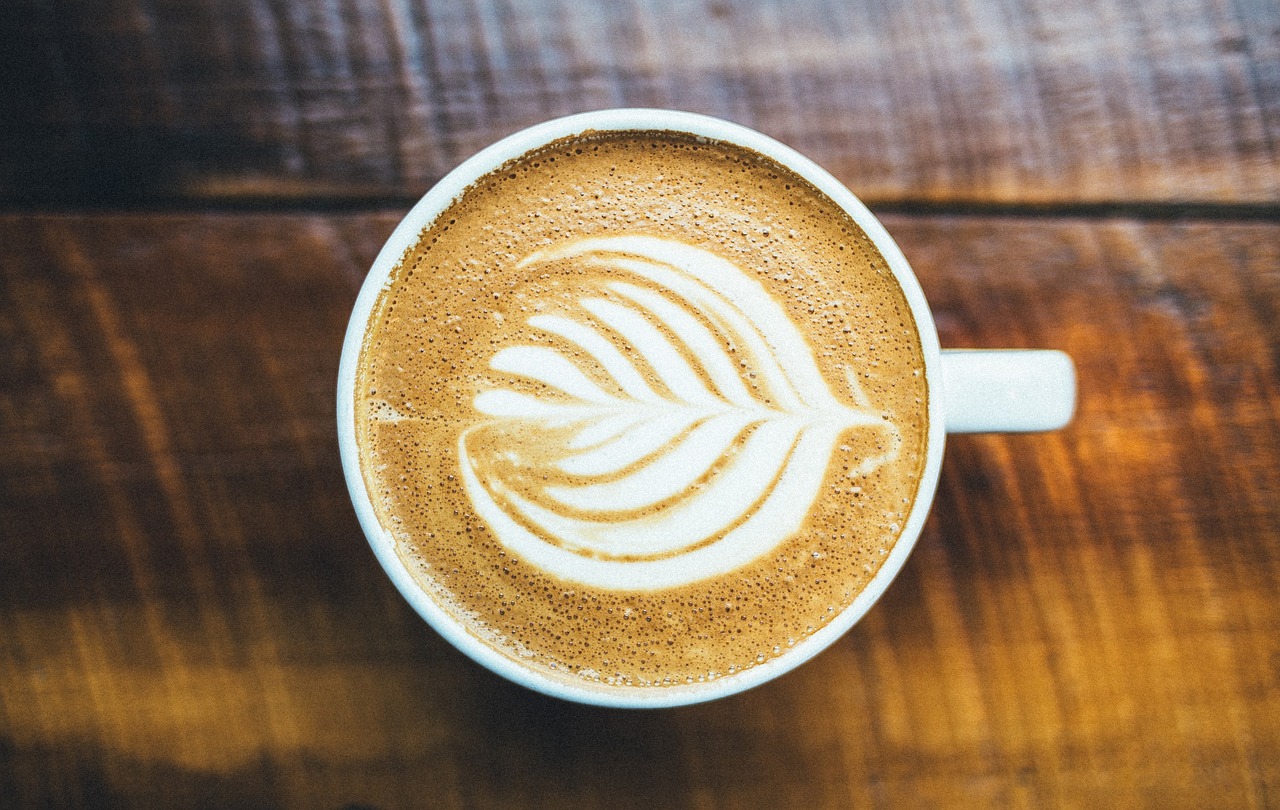
OCA Review 1 - Java Basics
I’m preparing the OCAJP: Oracle Certified Associate Java SE 8 Programmer. Here’re something interesting that I learned from the study guide, chapter 1 and chapter 2. They’re rarely used in our daily mission, but I just wrote them down for fun.
Convert Date to ISO 8601 String in Java
Convert Java dates to ISO-8601 string: this post explains how to convert java.util.Date, java.util.Calendar, java.time.ZonedDateTime to string.
New repo: Java Examples
I’ve been learning different Java frameworks since my last intern in Beijing. Today, I want to share my new GitHub repository: Java Examples. This repository is built on Maven, modularized and extensible.
Add listener to Android ListView
How to add listener to Android ListView.